Microservice: Service Registration and Service Discovery with Eureka
Introduction#
Service communication is crucial in a microservices architecture for scalability and dependability. Service Registration and Discovery are important operations in dynamically managing service instances so that communication is transparent without explicit setup. A strong service registry tool developed by Netflix, Eureka, supports microservices to register and discover other services dynamically. This eliminates hardcoded service URLs, enhances fault tolerance, and allows for transparent load balancing. Using Eureka, microservices can have greater flexibility, robustness, and scalability within a distributed environment.
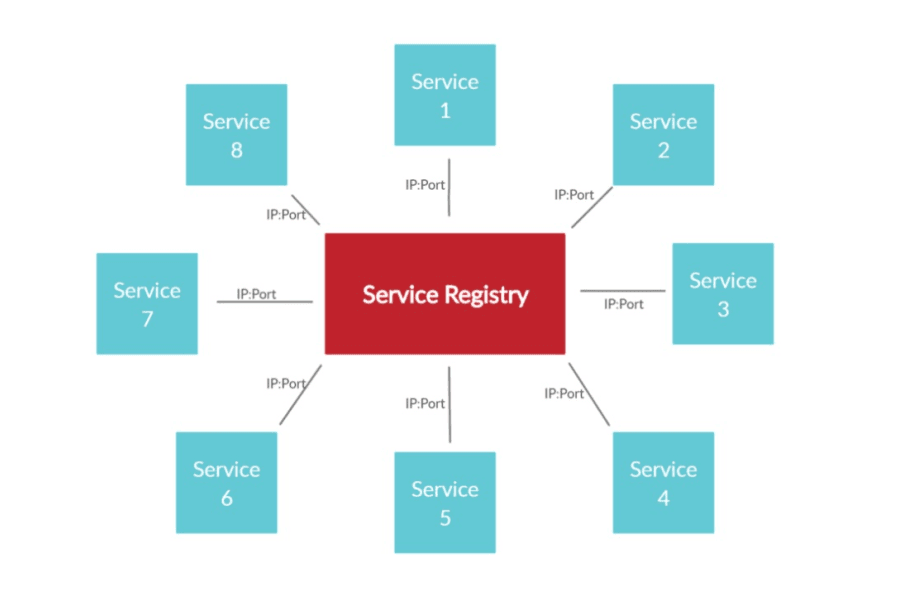
What is Service Discovery?#
In a microservices architecture, a microservice is an independent application with dedicated business functionality. Because these microservices have to interact with one another to act as a whole system, they require a way to dynamically discover each other. Service Discovery addresses this problem by keeping a list of currently running service instances and their network addresses. It allows microservices to discover and talk to each other without depending on hardcoded configurations, providing flexibility, scalability, and fault tolerance in distributed systems.
Service Discovery Types#
- Client-Side Discovery: The client asks for the service registry and chooses an available service instance directly. Example: Netflix Eureka + Ribbon.
- Server-Side Discovery: A load balancer (e.g., AWS ALB, Kubernetes Service) acts between the client and services, directing requests to the correct instance.
Why is service discovery important?#
- Removes manual service management.
- Allows for dynamic scaling and fault tolerance.
- Allows for load balancing and high availability.
- Enhances resilience in a microservices architecture.
Spring Cloud Eureka#
Eureka is a REST-based registry for services, built by Netflix and extensively utilized in Spring Cloud for service registration and discovery within a microservices system. Eureka facilitates dynamic registration of microservices and their discovery without hardcoding configurations.
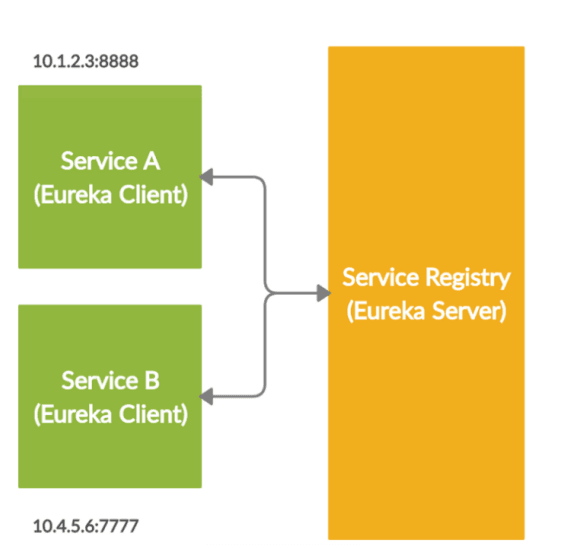
Eureka Components#
Eureka Server: Central service registry to keep details about all registered microservices. It serves as a discovery service.
Eureka Clients: Microservices that register themselves with the Eureka Server and ask it to discover other services for communication.
How It Works#
Service Registration: A microservice, when it is started, registers itself with the Eureka Server.
Service Discovery: Other microservices ask the Eureka Server to retrieve the location of necessary services.
Heartbeat Mechanism: Eureka clients periodically send heartbeats to confirm their availability; the failure of a service results in it being ejected from the registry.
Why Use Eureka?#
- Manual configuration was removed by dynamic service discovery.
- Highly available through redundancy and auto-healing.
- Load balancing in conjunction with Ribbon.
- Smooth integration with Spring Boot & Spring Cloud.
How Eureka Works?#
Eureka uses a formal procedure for handling service registration, discovery, and health checks in a microservices environment.
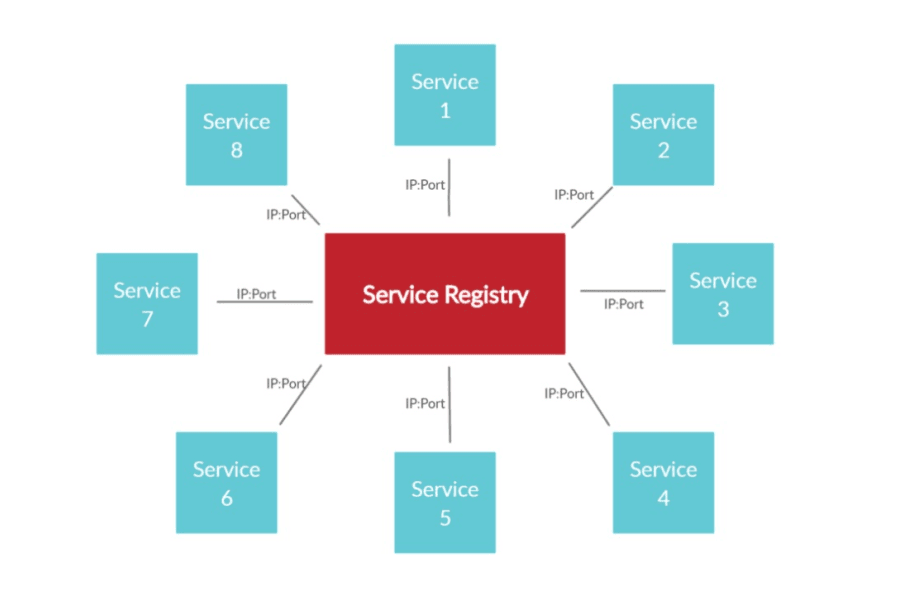
Service Registration#
During bootup, a microservice (Eureka Client) registers with the Eureka Server by issuing a registration request. The details of this service (IP address, port, metadata) are stored by the Eureka Server in its registry.
Heartbeat Mechanism#
Registered services issue heartbeat requests to the Eureka Server at regular intervals. These heartbeats extend the lease on the service so that Eureka is aware that the service is up and running.
Service Discovery#
If a microservice must talk to another service, it asks the Eureka Server to obtain the address (IP & port) of the target service. This avoids hardcoded service addresses and makes the system dynamic and scalable.
Health Checks#
Eureka can do health checks to ensure that a registered service is still up. If a service is not passing the health check, it is designated as unavailable in the registry.
Eviction Mechanism#
If a service is not sending heartbeats and its lease has expired, the Eureka Server evicts the service from the registry. This avoids routing traffic to non-available instances, thus making the system more stable.
Generate Discovery Service#
- Go to https://start.spring.io/ and generate a service (add only Eureka Server Dependency).
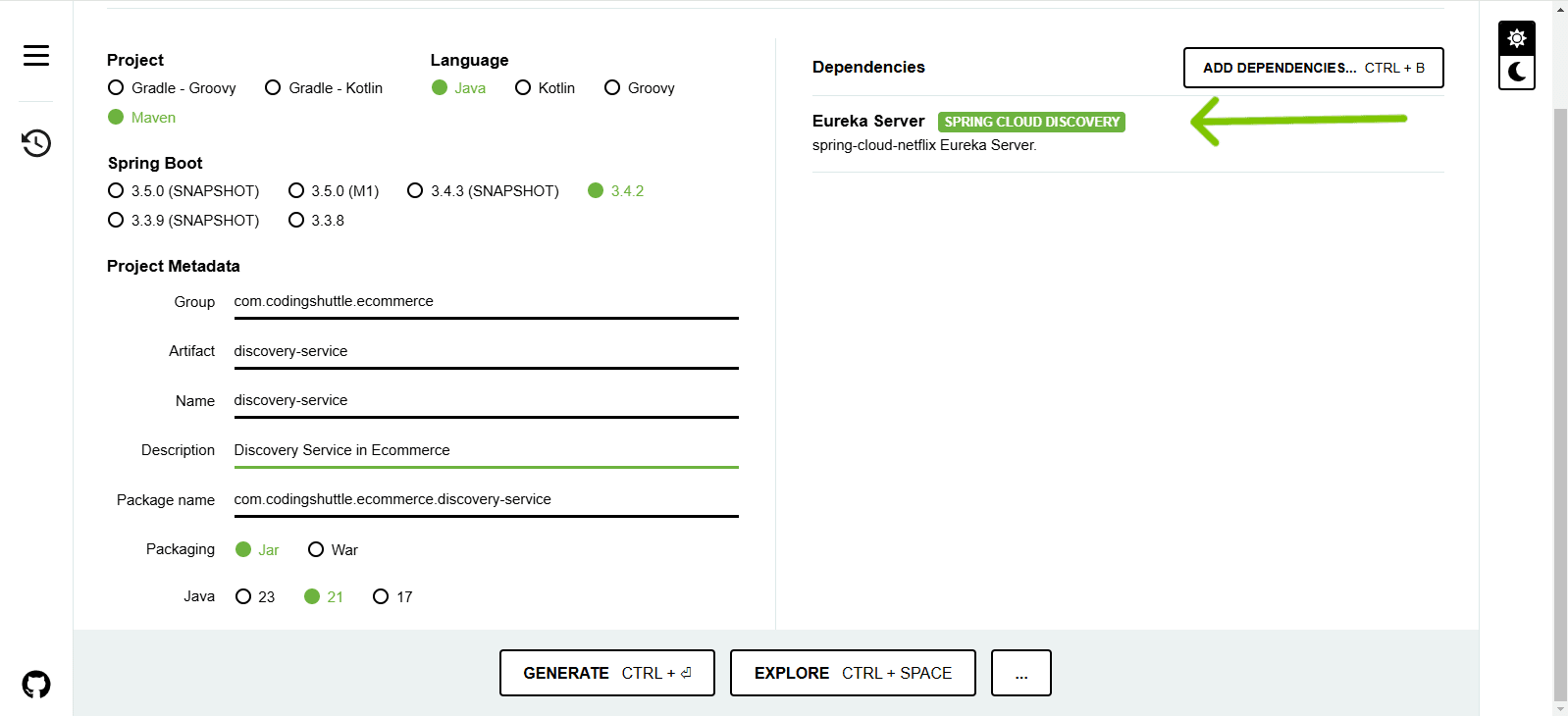
Extract it inside the ecommerce folder.
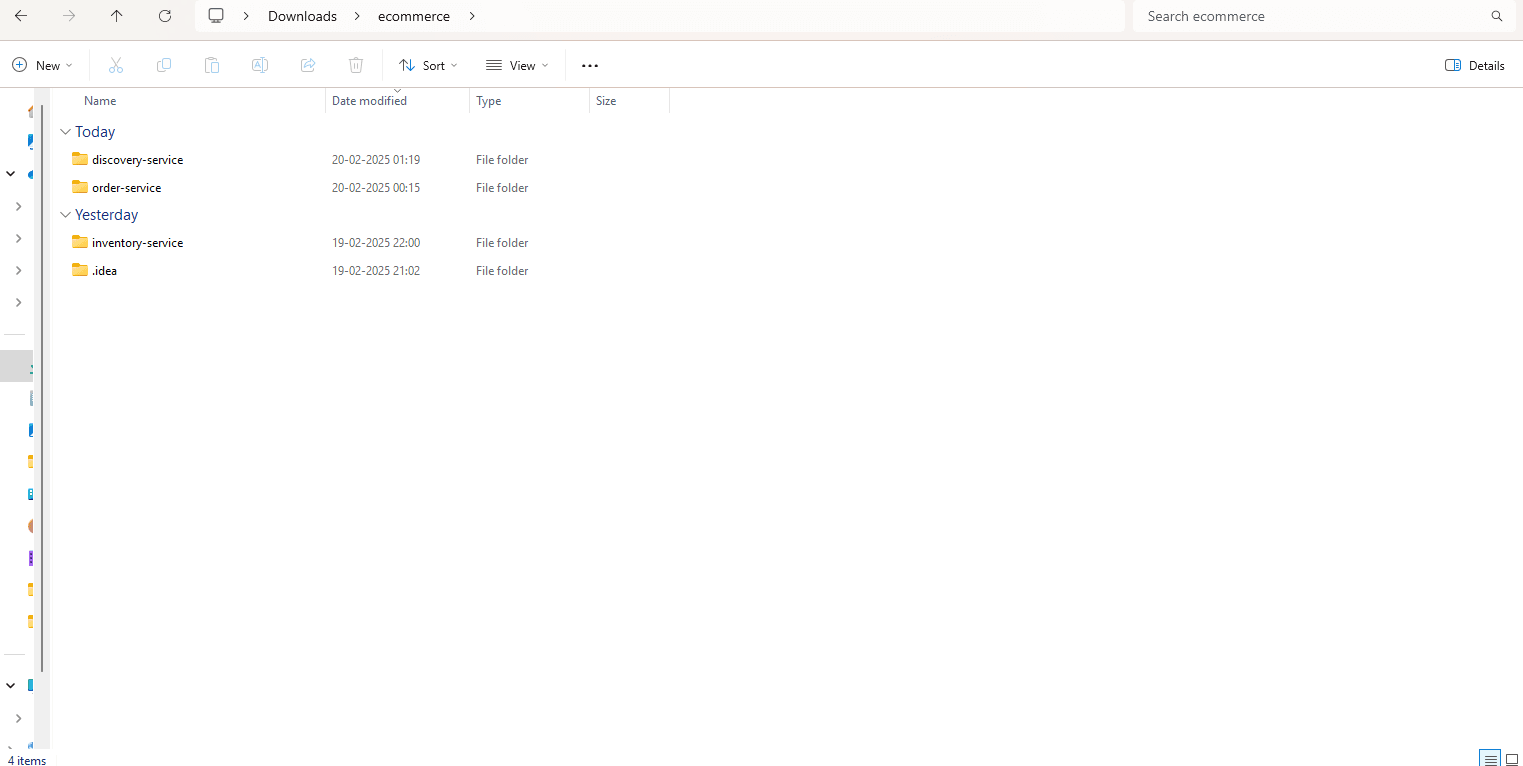
- After open it inside your ide, you have to add it as a maven project.
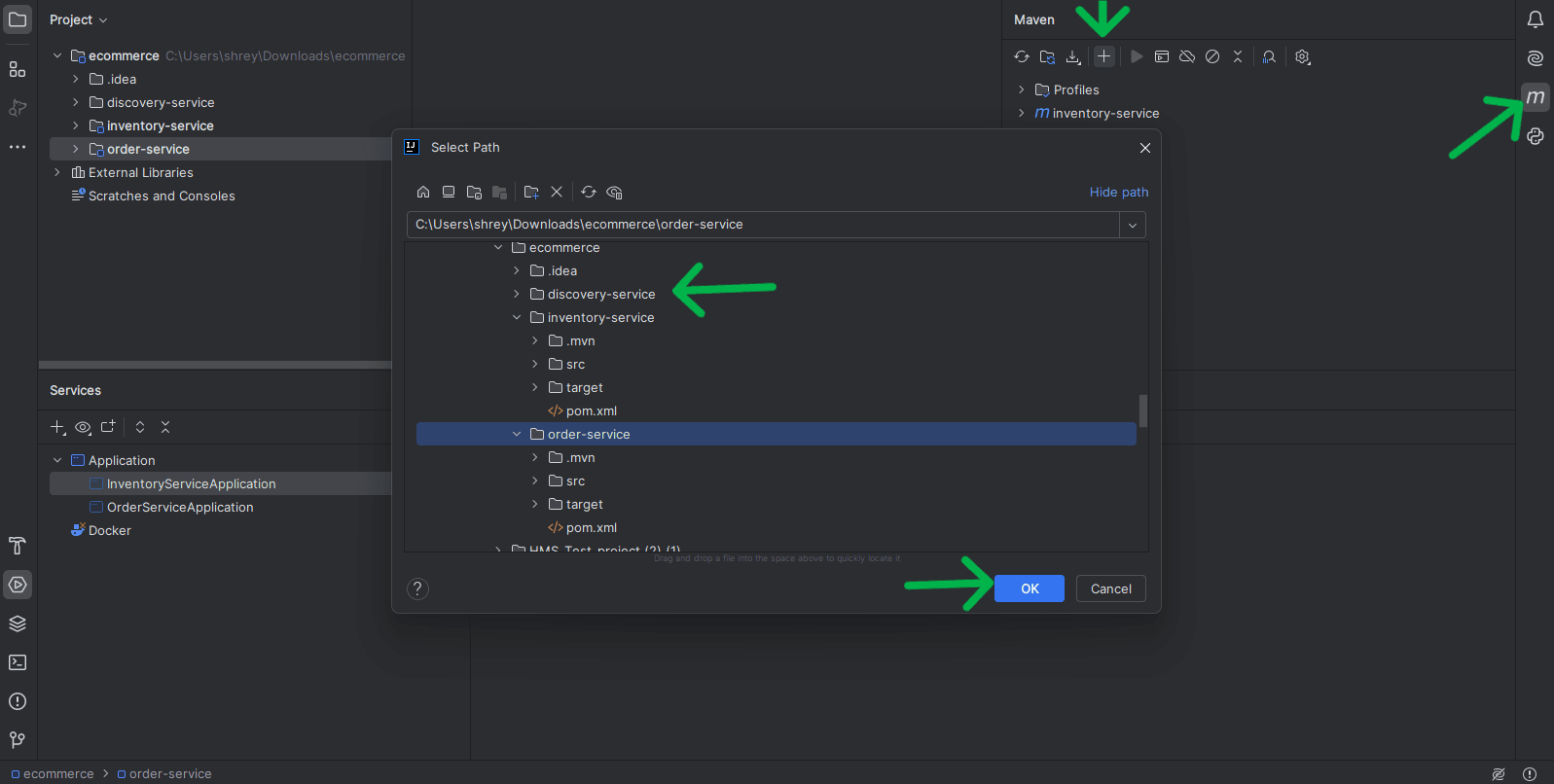
- Enable eureka server annotation inside main application class.
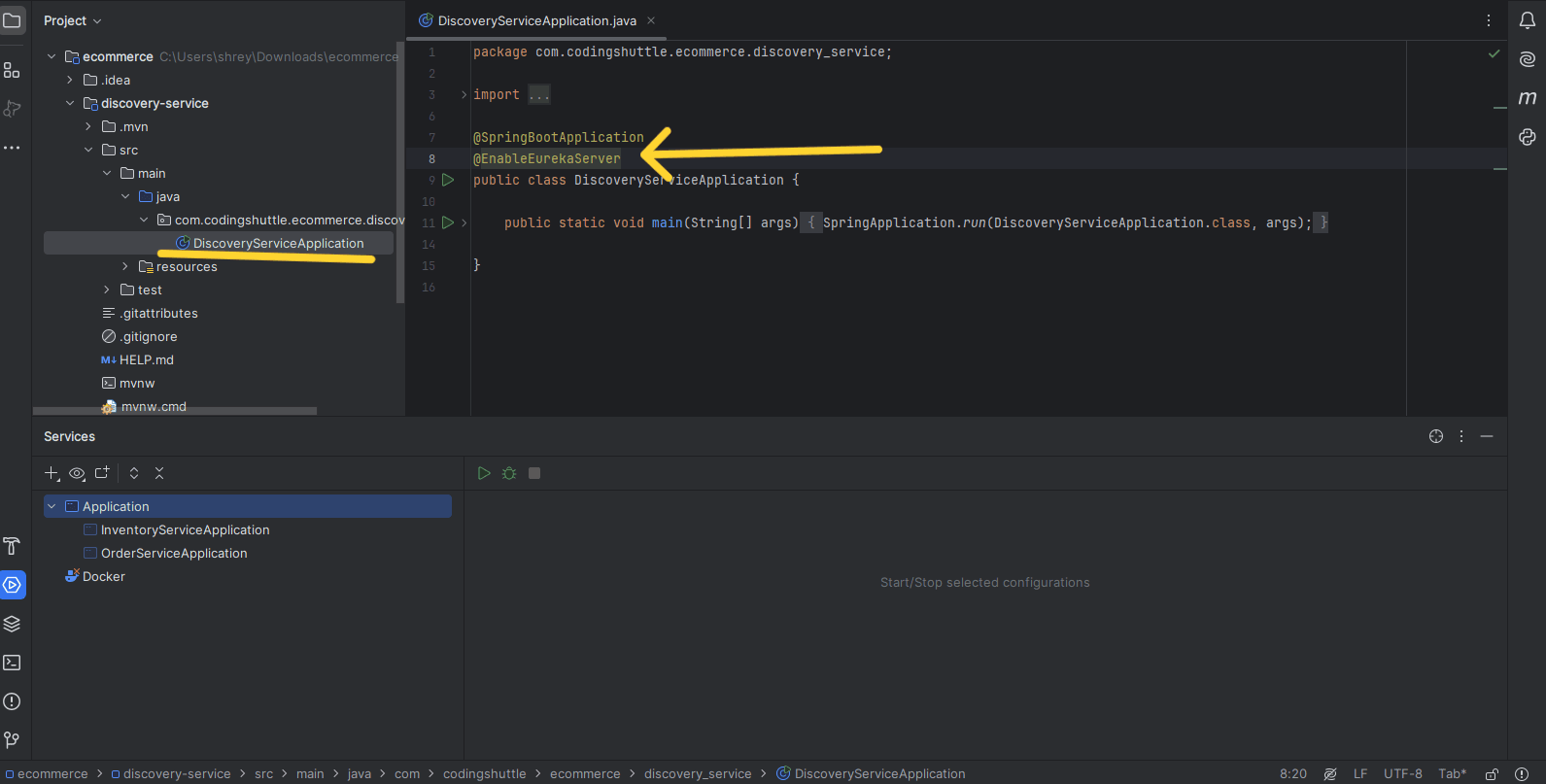
Configuring the Eureka Server#
- Add dependency of Eureka Server
- Inside application.properties/application.yml file you have to configure it.
spring.application.name=<Your application name>
server.port=8761
default port
eureka.client.register-with-eureka=false
eureka.client.fetch-registry=false
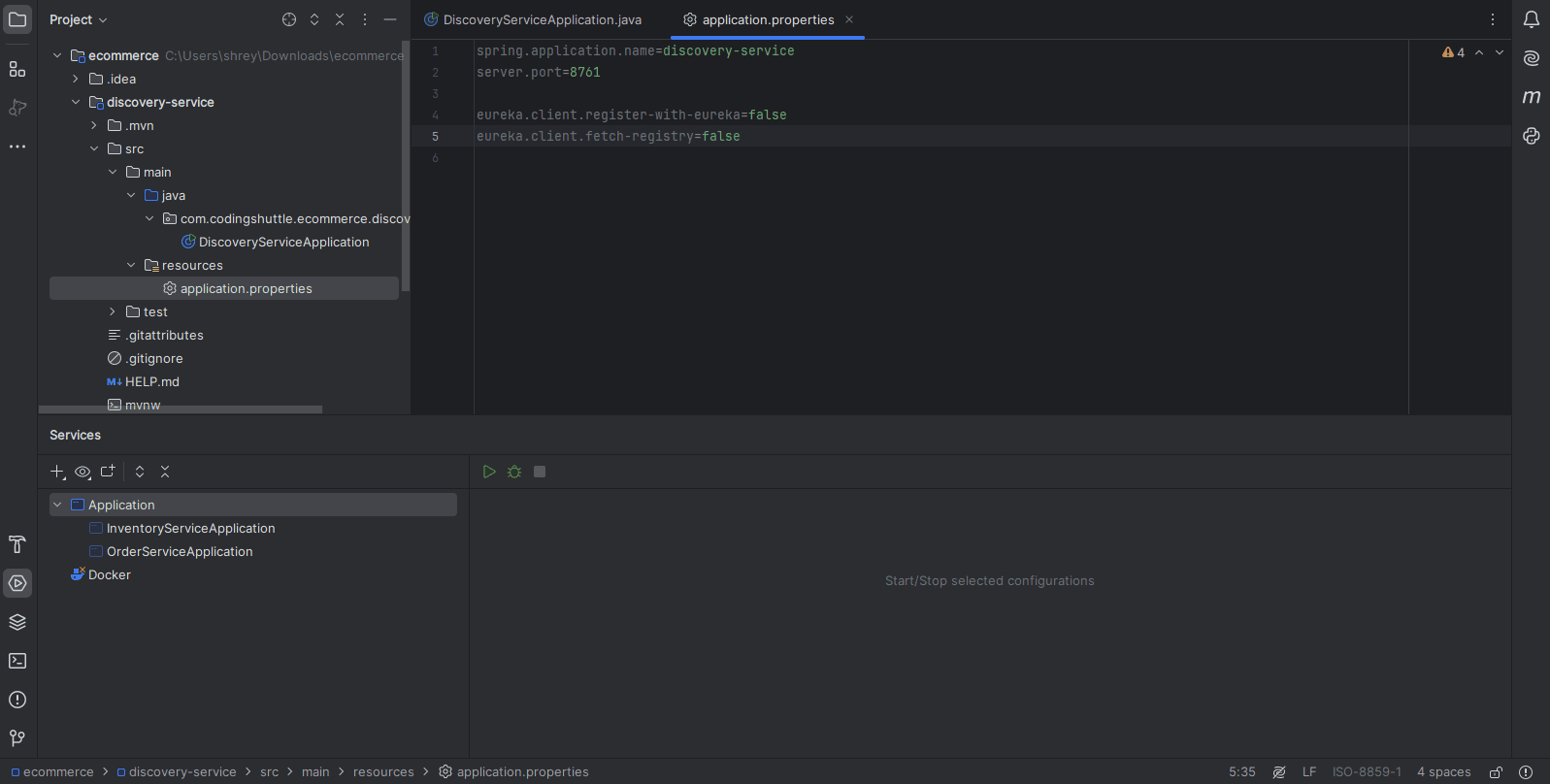
Configuring the Eureka Client#
- Add dependency of Eureka Client inside client services(order-service, inventory-service, api-gateway, etc.).
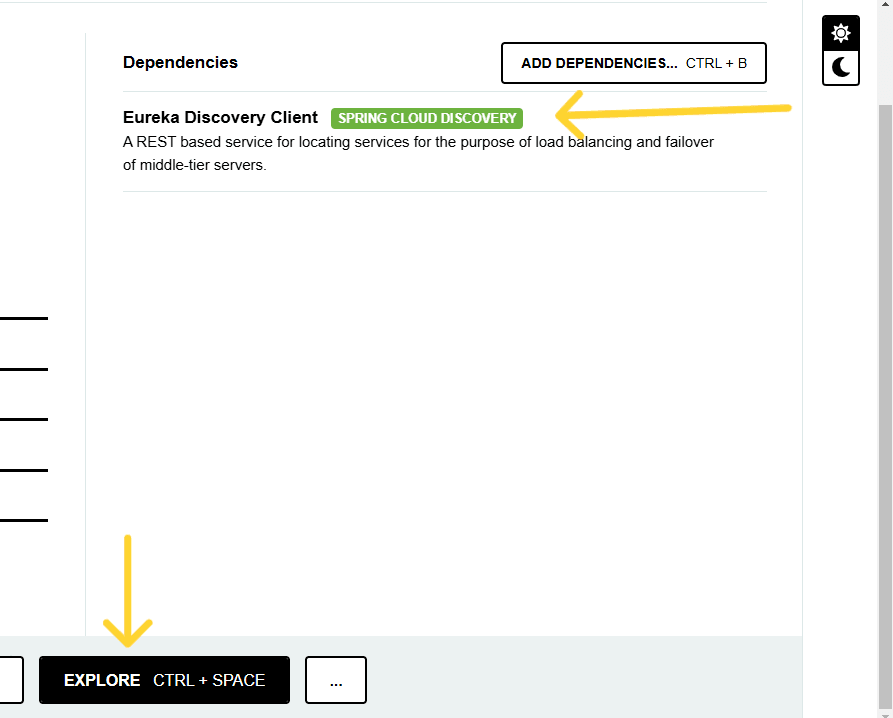
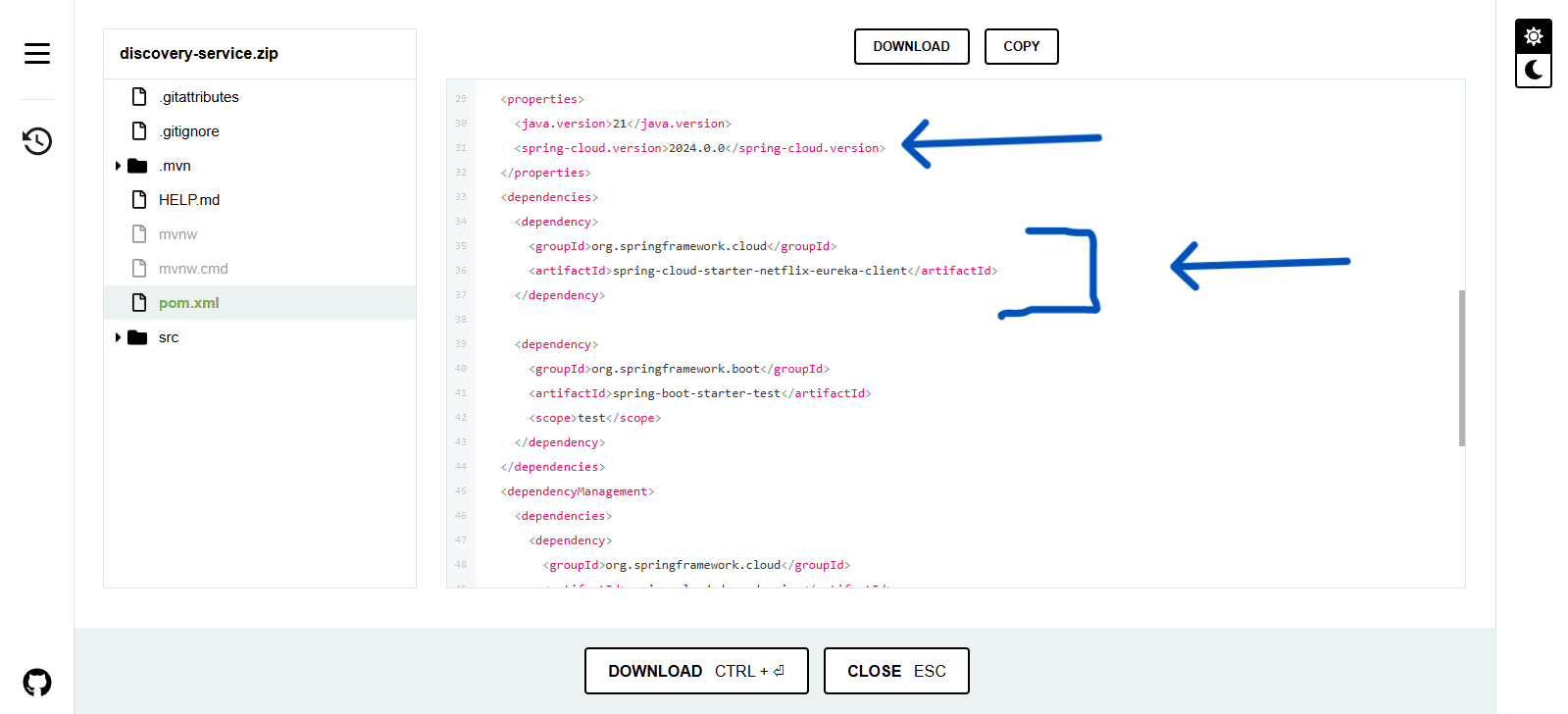
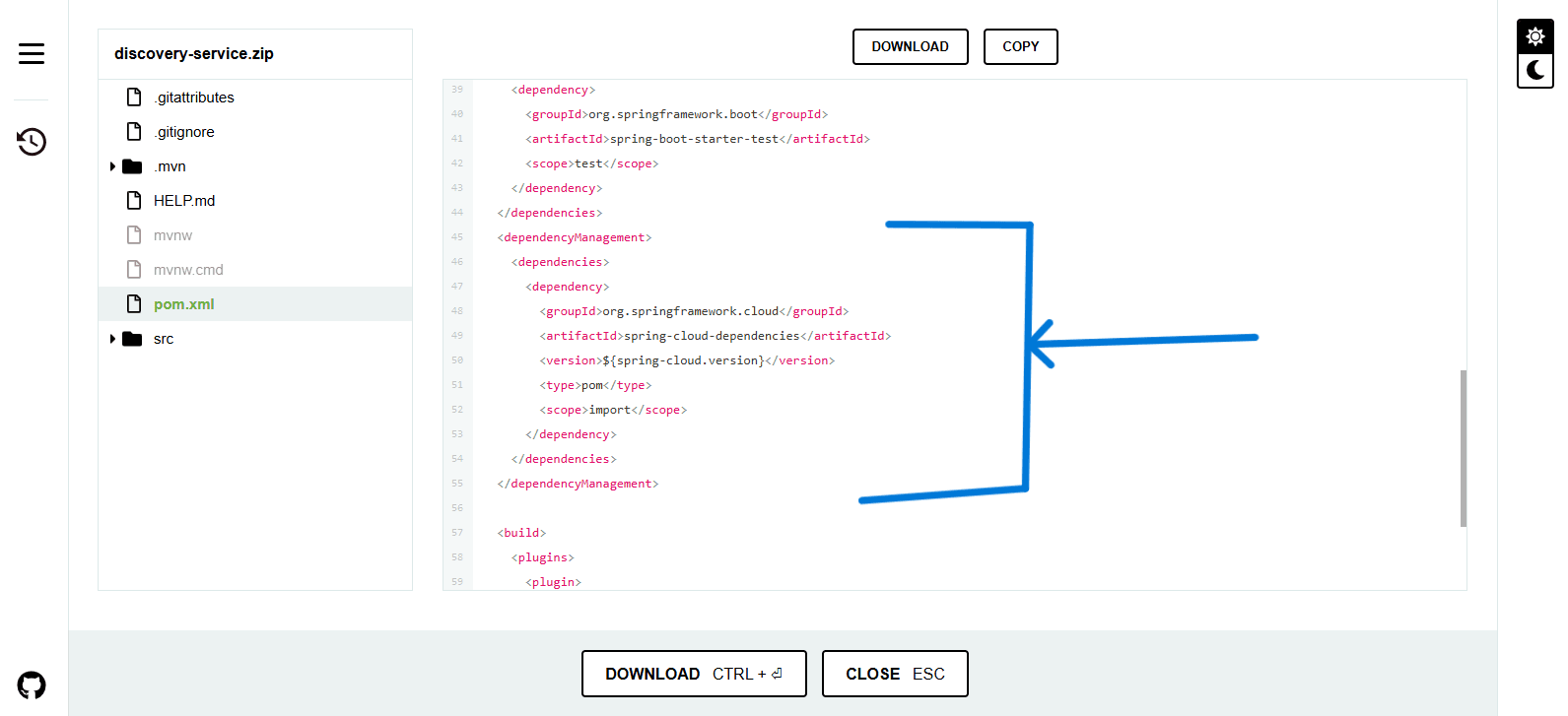
- Inside application.properties/application.yml file you have to configure it inside client services(order-service, inventory-service, api-gateway, etc.).
eureka.client.service-url.defaultZone=http://localhost:8761/eureka
eureka.instance.prefer-ip-address=true
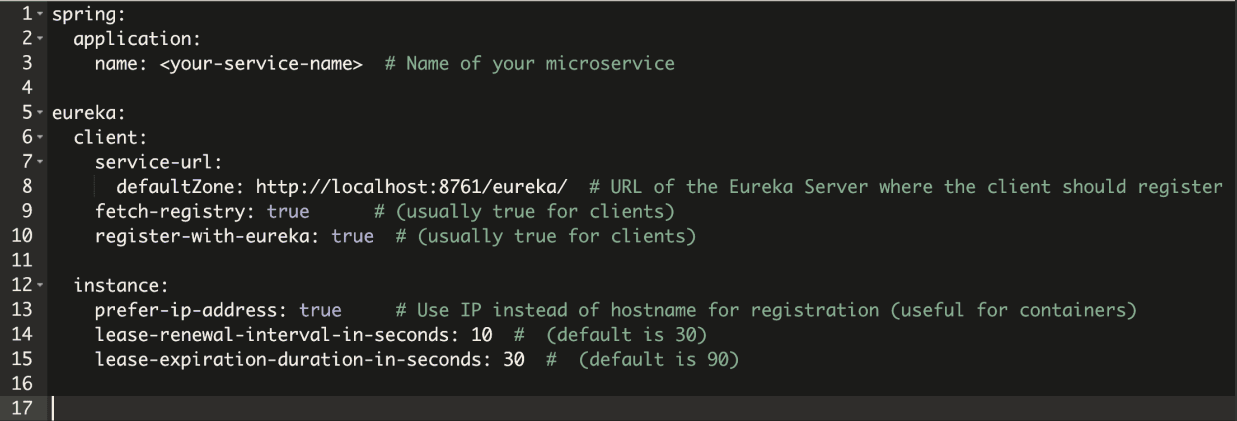
Run And Register All Services Sequentially#
- Run discovery service (Eureka Server)
- Run Eureka Clients (order-service, inventory-service, api-gateway, etc.)
Go to http://localhost:8761/ url you can see all clients services are registered properly (after running properly) and if you stopped any of service, service are de-registered.
- Registered Services
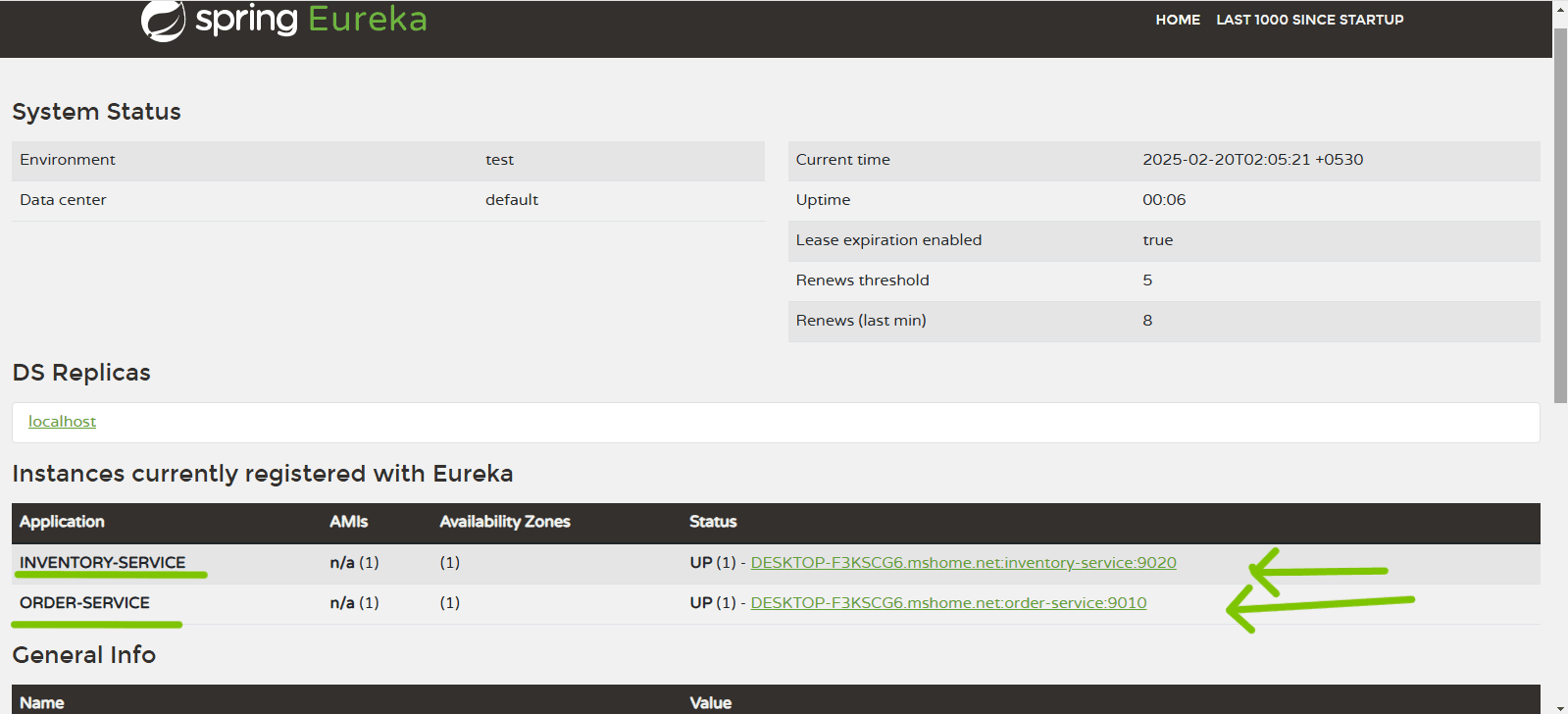
- De-Registered Service
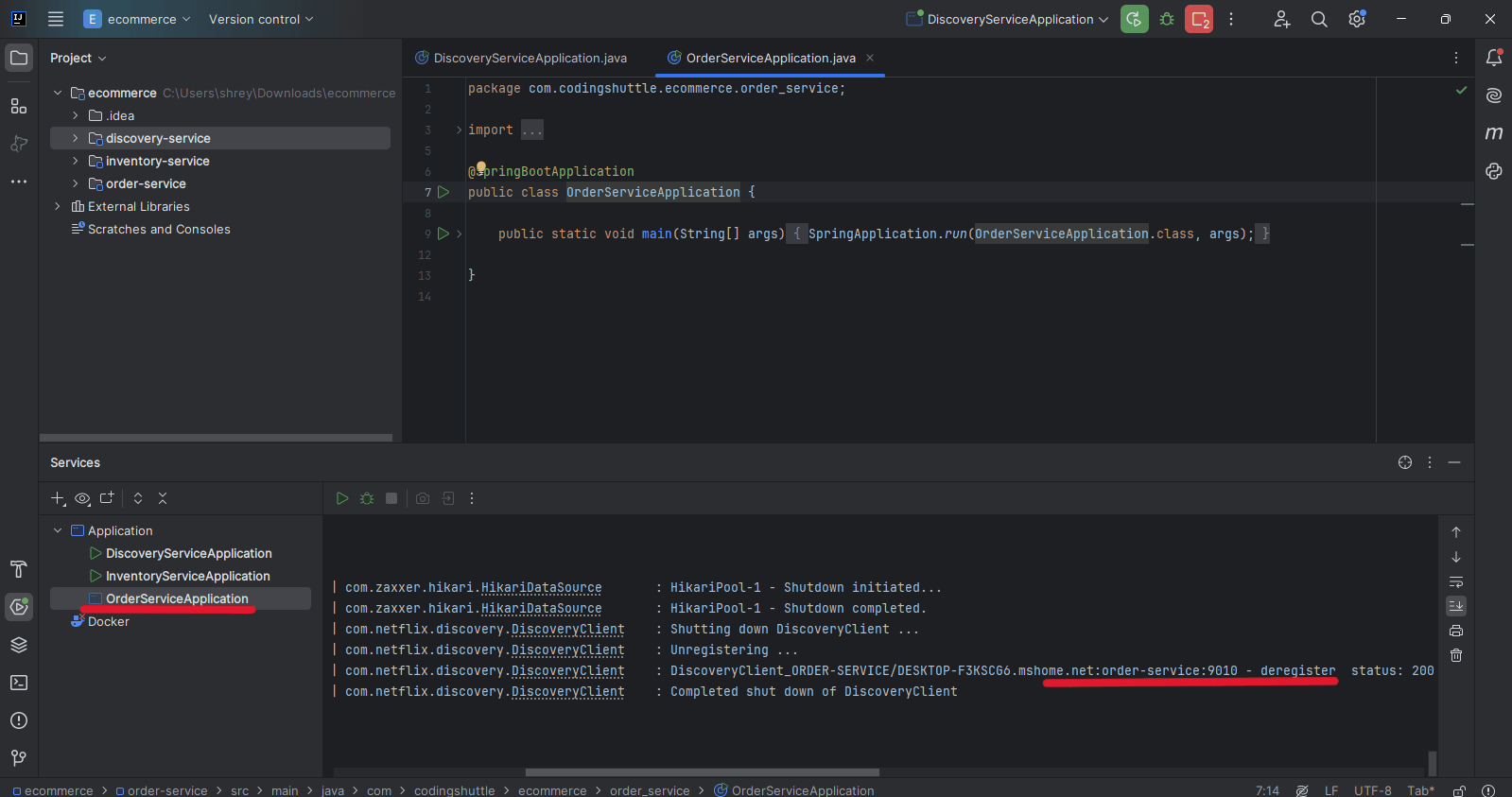
Examine How To Work All Services (Eureka Server and Eureka Clients)#
1. Create a Orders (Dummy api) inside order-service#
2. Create a Fetch (Dummy api) inside inventory-service#
3. RestClient configuration class#
4. Output#
After running all services one by one (1. Discovery Service, 2. All clients services one by one), if you hit the inventory service’s (http://localhost:9020/api/v1/products/fetchOrder) url you can fetch the order.
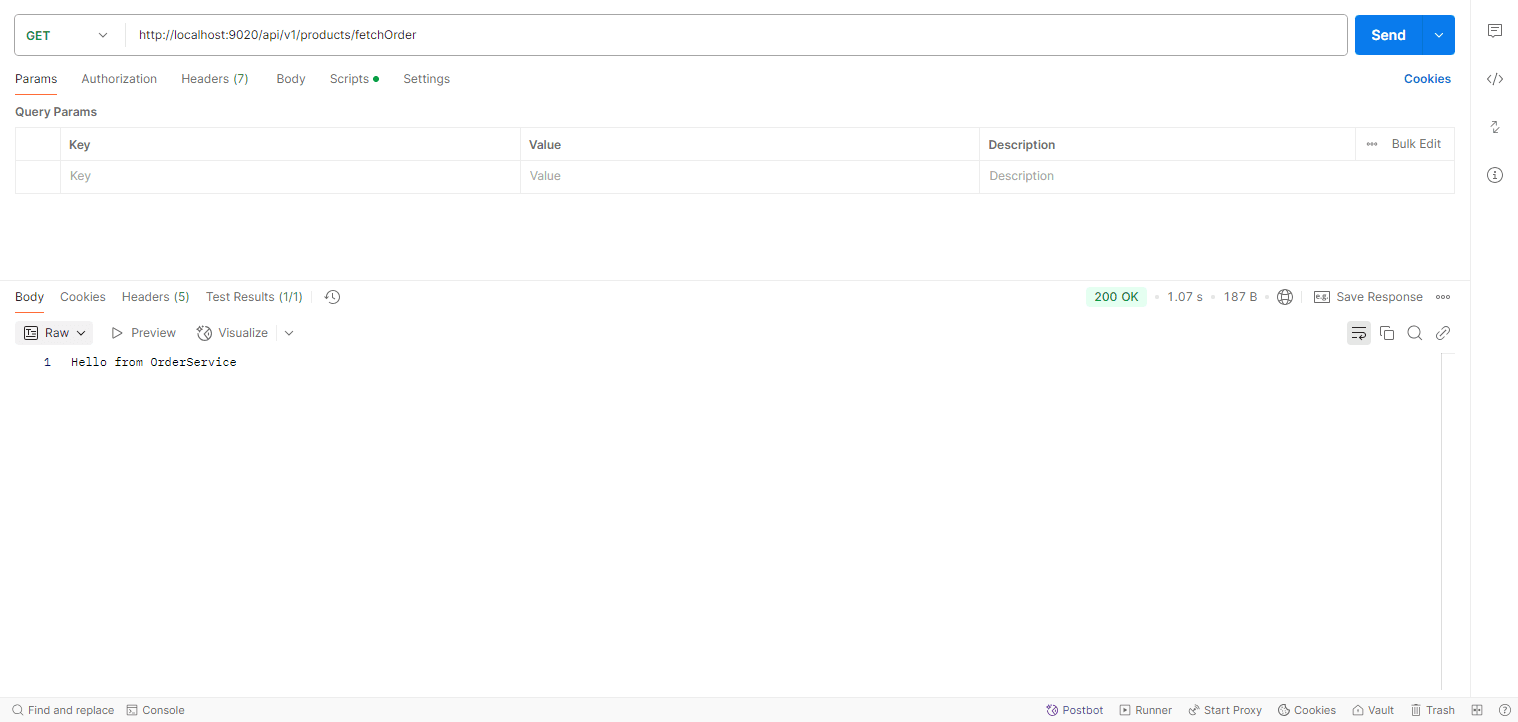
Conclusion#
This article describes how service registration with Eureka facilitates transparent microservices communication by enabling services to dynamically discover and register with each other. By using Eureka Server to manage services, it provides high availability, fault tolerance, and scalability for cloud-native microservices architectures.