Understanding JUnit and AssertJ
Introduction :#
JUnit and AssertJ are both popular libraries in the Java ecosystem for writing and managing unit tests, but they serve different purposes and complement each other well.
Add dependency#
For testing, we have already added the spring-boot-starter-test
dependency to your application.
Try pressing Ctrl + click on the dependency to navigate to it. Inside this dependency, you will find many additional dependencies: assertj-core and junit-jupiter and mockito-junit-jupiter.

JUnit#
JUnit is a framework for writing and running tests in Java. It provides the basic structure for test cases, including annotations to define test methods and assertions to check expected outcomes.
Common Junit Annotations
- @Test
- Marks a method as a test method.
- JUnit will execute this method when running tests.
- Example
- Output
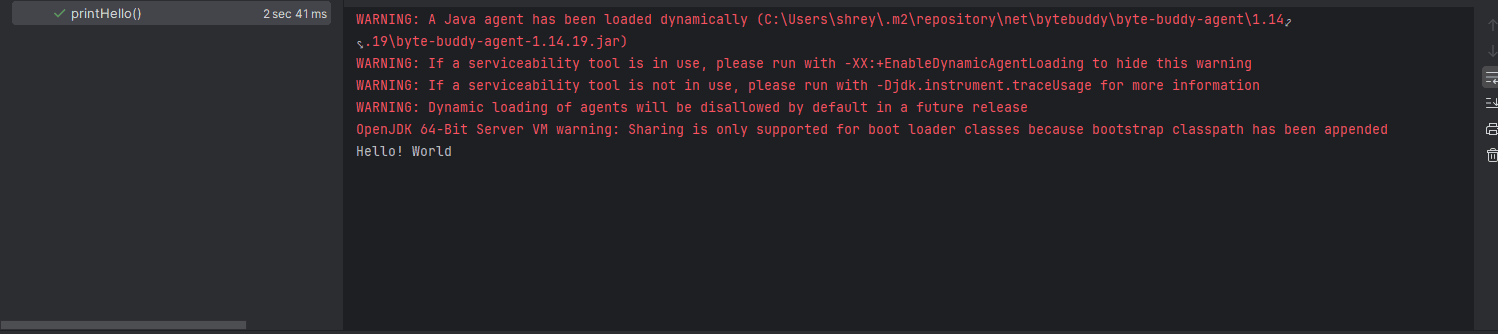
If you choose to remove @SpringBootTest, you can do so. However, the output structure will be affected as follows.

- @DisplayName
- Sets a custom display name for the test class or test method.
- This name is used in test reports and IDEs to make test results more readable.
- Example
- Output
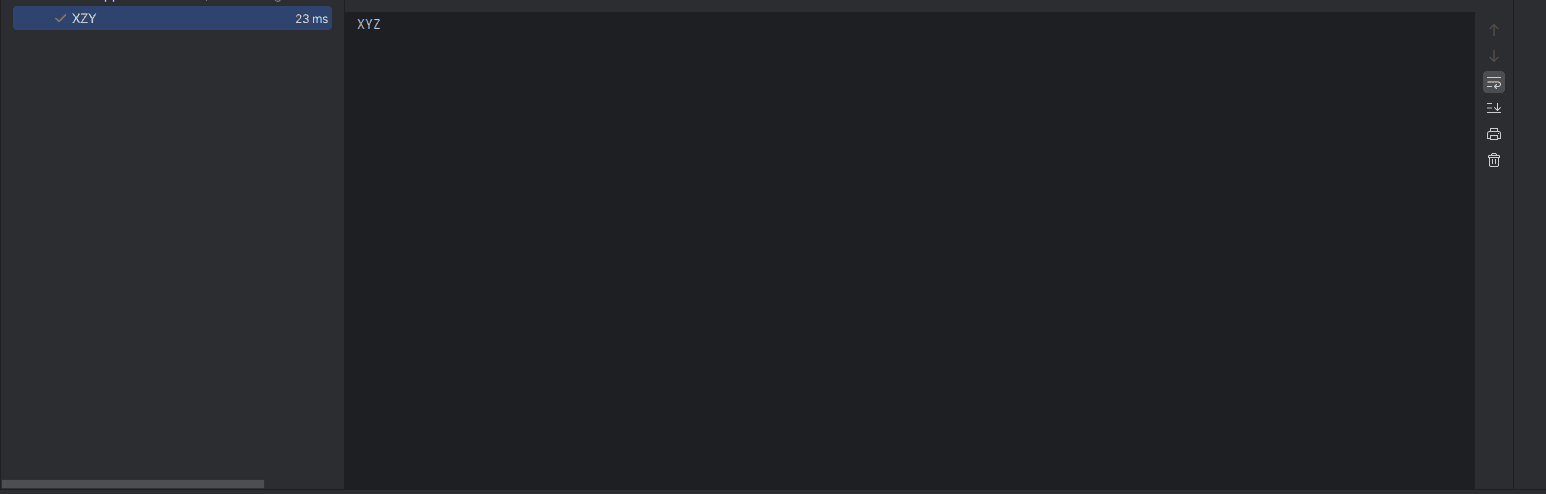
- @Disabled
- Disables a test class or test method, so it is not executed.
- Useful for skipping tests that are not relevant for a particular run or are known to be failing.
- Example
- Output
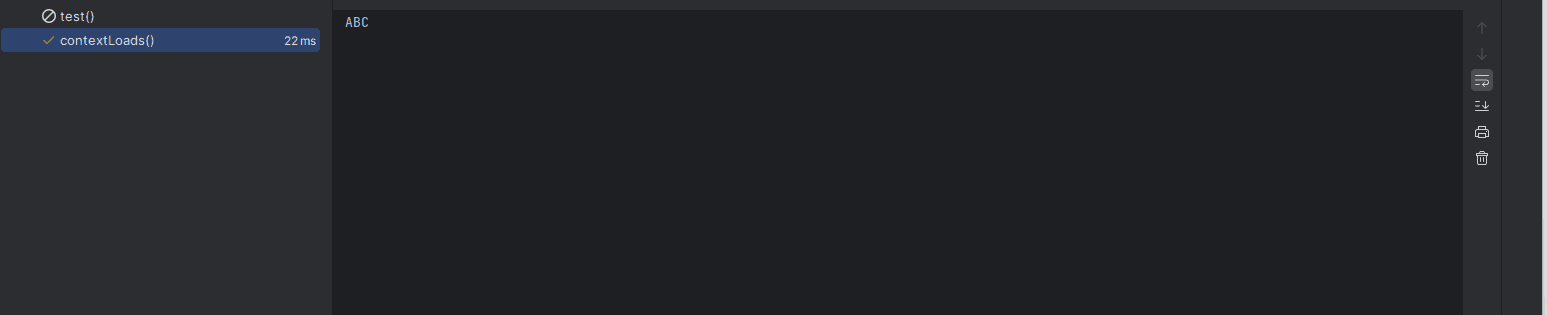
- @BeforeEach
- Indicates that the annotated method should be executed before each test method.
- Useful for setting up common test data or state before each test runs.
- Example
- Output

- @AfterEach
- Indicates that the annotated method should be executed after each test method.
- Useful for cleaning up resources or resetting states after each test.
- Example
- Output

- @BeforeAll
- Indicates that the annotated method should be executed once before all test methods in the class.
- The method must be static.
- Useful for expensive setup operations that are shared across all tests.
- Example
- Output

- @AfterAll
- Indicates that the annotated method should be executed once after all test methods in the class.
- The method must be static.
- Useful for cleaning up resources or performing final actions after all tests have run.
- Example
- Output

More JUnit Functionalities: Overview (JUnit 5.11.0 API)
Assertion with JUnit#
JUnit's basic assertions are essential for verifying test conditions. Here’s a detailed explanation of the ones you mentioned:
assertEquals(expected, actual)
:- Compares the
expected
value with theactual
value. - If they are not equal, the test fails.
- Useful for checking if a method returns the correct result.
- Here, you can use any type of datatype.
- Example:
- Compares the
- Output:

assertTrue(condition)
:- Checks if the given
condition
istrue
. - If it’s false, the test fails.
- Example
- Checks if the given
- Output

assertFalse(condition)
:- Checks if the given
condition
isfalse
. - If it’s true, the test fails.
- Example
- Checks if the given
- Output

AssertJ#
AssertJ is a library that provides a rich and fluent API for writing assertions in tests. It offers more expressive and readable assertions compared to JUnit’s built-in assertions.
- Numbers:
- assertThat(5).isEqualTo(5).isNotEqualTo(10).isGreaterThan(4);
- You can chain multiple assertions for better readability and conciseness when working with numbers.
- Example
- Output

- String:
- assertThat("hello").startsWith("he").endsWith("lo").contains("ell");
- AssertJ provides powerful assertions for string operations like matching, checking substrings, and more.
- Example
- Output

- Boolean:
- assertThat(true).isTrue(); OR assertThat(false).isFalse();
- AssertJ makes assertions on boolean values more readable and intuitive.
- Example
- Output

- List/Array:
- assertThat(List.of("apple", "banana")).contains("apple") .doesNotContain("orange").hasSize(2);
- AssertJ provides a wide range of assertions for collections like lists and arrays.
- Example
- Output

- Exceptions:
- assertThatThrownBy(() -> { throw new IllegalArgumentException("Invalid argument"); }).isInstanceOf(IllegalArgumentException.class) .hasMessage("Invalid argument") .hasStackTraceContaining("ExampleTest");
- AssertJ offers a fluent API to check for exceptions thrown by code blocks, including checking the exception type, message, and stack trace details.
- Example
- Output

More AssertJ Methods: AssertJ - fluent assertions java library
JUnit vs AssertJ#
JUnit is one of the most widely used testing frameworks in the Java ecosystem. JUnit provides a simple and standardized way to write test cases, execute them, and report the results. AssertJ, on the other hand, is not a testing framework but rather a library that complements testing frameworks like JUnit. It focuses on providing fluent and expressive assertions, enhancing the readability and maintainability of your test code.
JUnit and AssertJ are both popular tools used in Java for testing, but they serve different purposes and have distinct features.
Here, we have already covered these aspects, which are highlighted in green in the image.
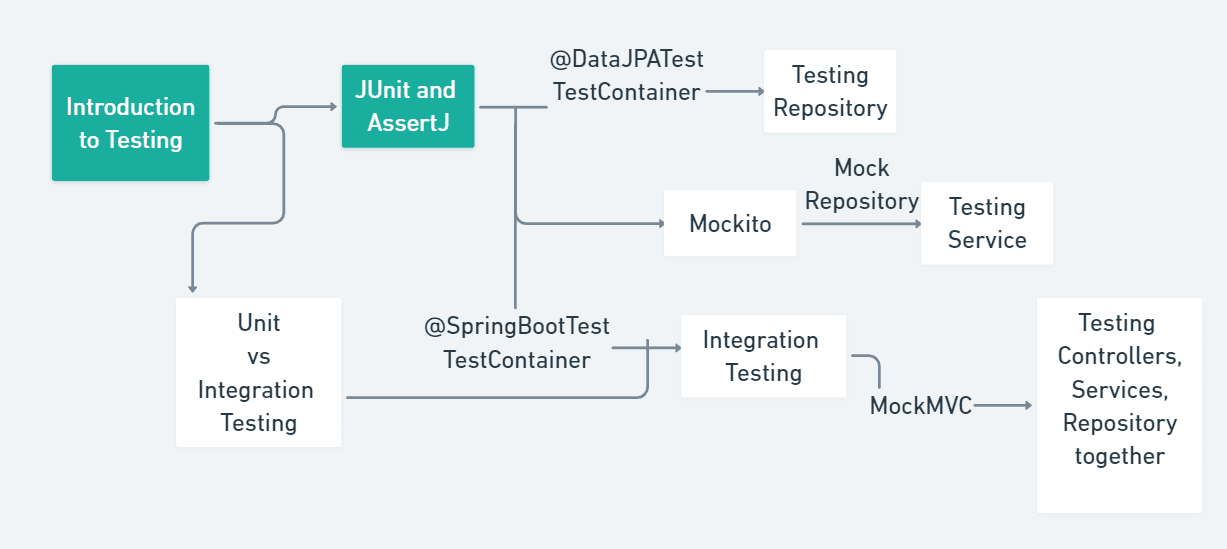
Conclusion#
This article highlighted the key roles of JUnit and AssertJ in Java testing. JUnit offers a framework with annotations and assertions for structuring tests, while AssertJ enhances readability with a fluent API for assertions. Together, they enable developers to write efficient, reliable tests, ensuring high-quality Java applications.