Task Scheduling in Spring Boot
Introduction#
Tasks are scheduled in Spring Boot for the execution of specified tasks or methods, either once or repeatedly, at certain times or intervals, without human intervention. The feature is vital for automating background processes and optimizing application performance.
Spring Boot supports task scheduling through the **@Scheduled
**annotation along with the TaskScheduler
interface. Using this support, the execution of tasks can be scheduled easily.
Typical Applications of Task Scheduling#
- Log user activity on a separate thread.
- Send notifications (emails/SMS) asynchronously after some user actions.
- Perform parallel processing of image uploads, database queries, or API calls.
- Clean up expired records from the database.
- Archive logs at prescribed rates
- Fetch data from external APIs at specified intervals
- Carry out health checks on external systems or services.
@Scheduled Annotation#
This annotation performs the task of running processes at given intervals or times.
How It Works:#
- Enable Scheduling
In the configuration class, you have to specify @EnableScheduling
to turn on the scheduling service.
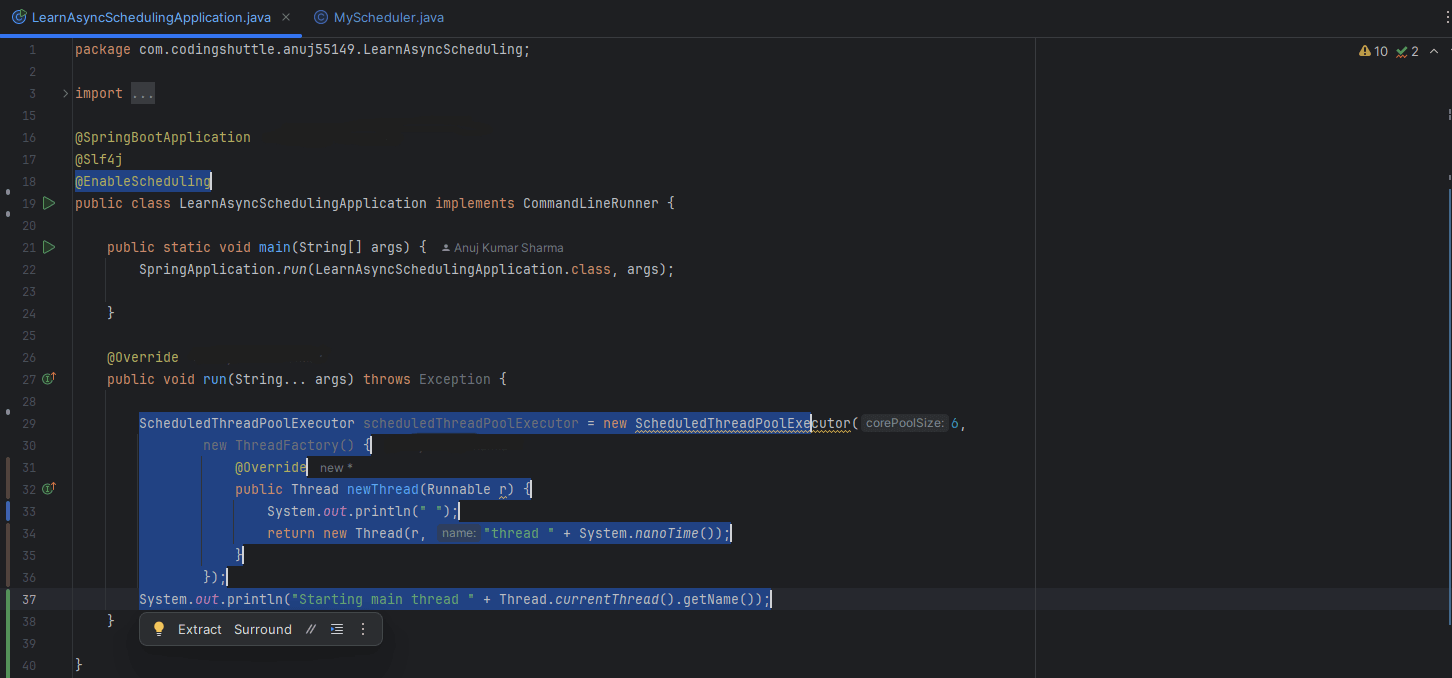
- Annotate the Methods
It applies the @Scheduled
annotation to any method that is to be scheduled. The method must satisfy:
- Has no return type (
void
) - No parameters are passed to it (no arguments)
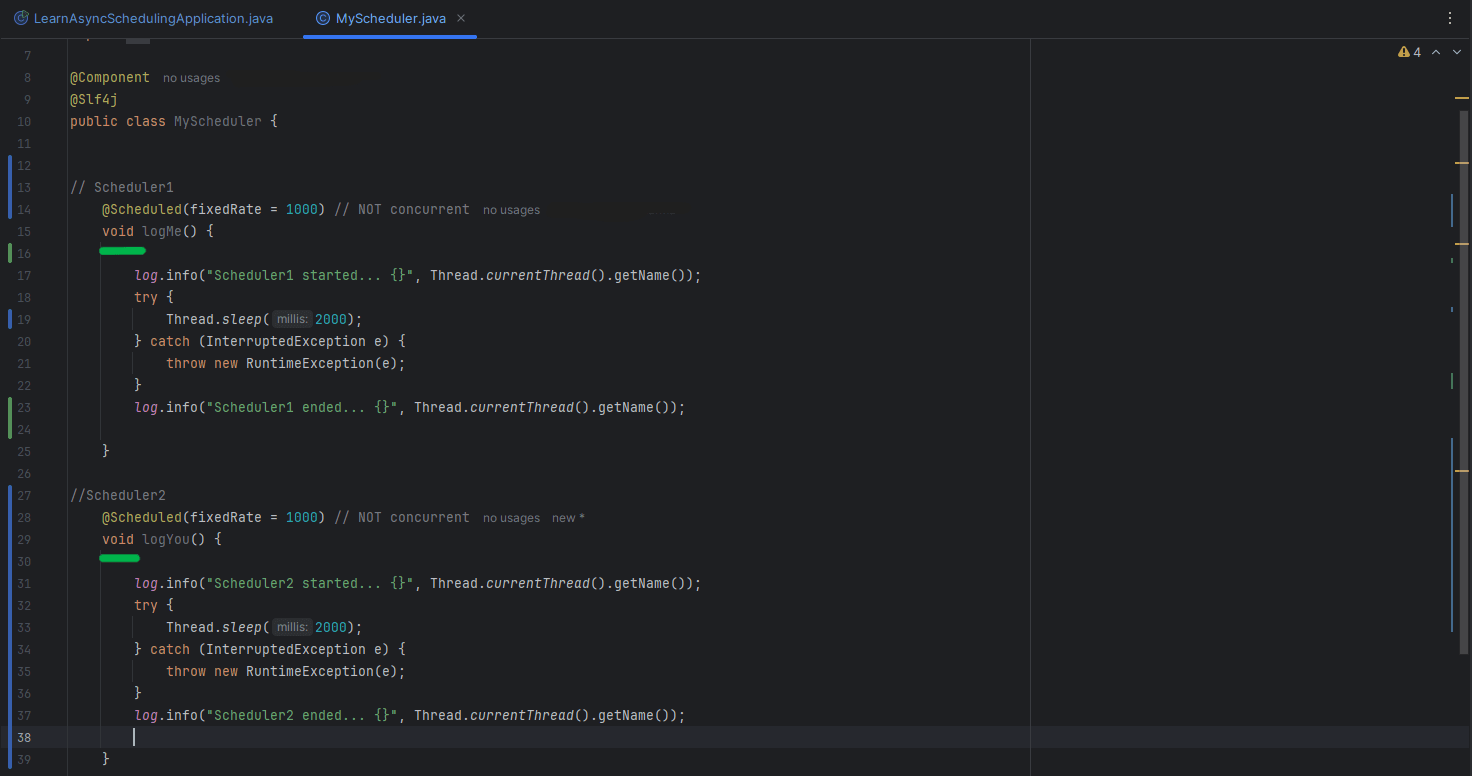
@Scheduled
Annotate the MethodsKey parameters of @Scheduled#
This annotation,@Scheduled
, makes it possible for Spring Boot to define any given task to be scheduled using a variety of timing options:
fixedRate
→ The defined interval between the start of two successive executions is not affected by the duration of the task.
Output:
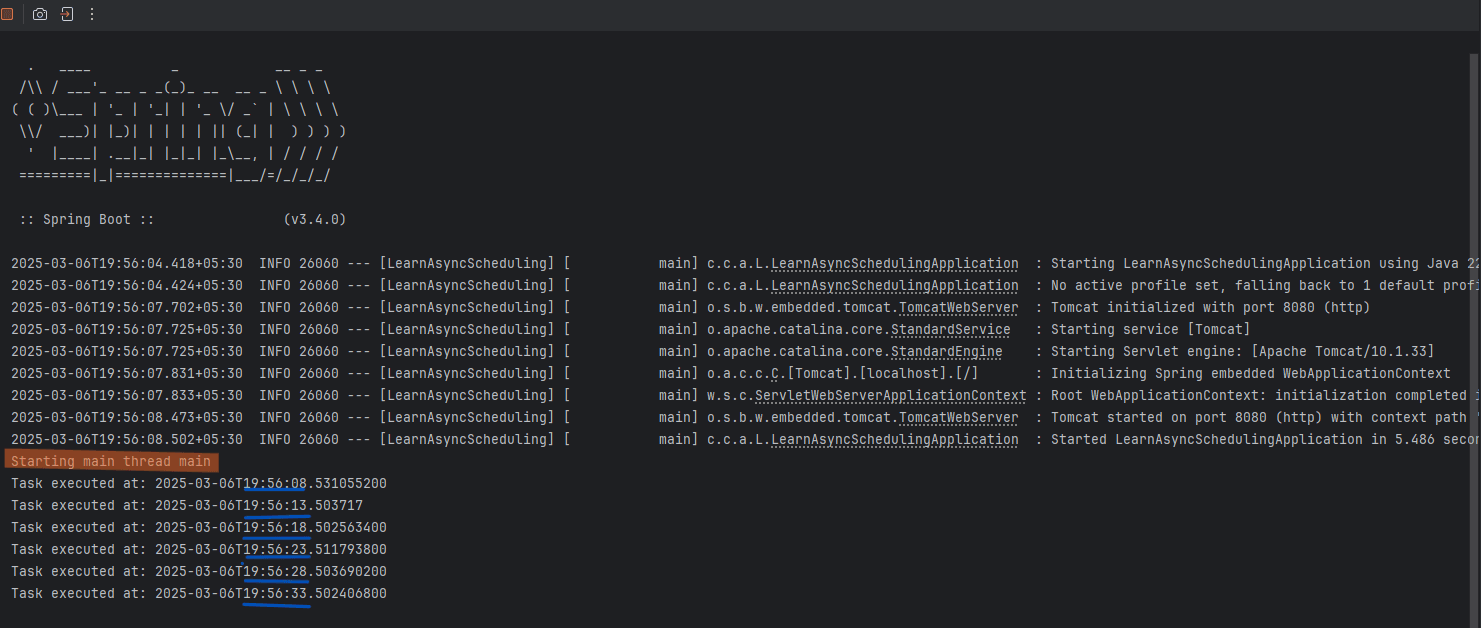
fixedDelay
→ The amount of time the task is to wait before being scheduled again from the end of the last execution.
Output:
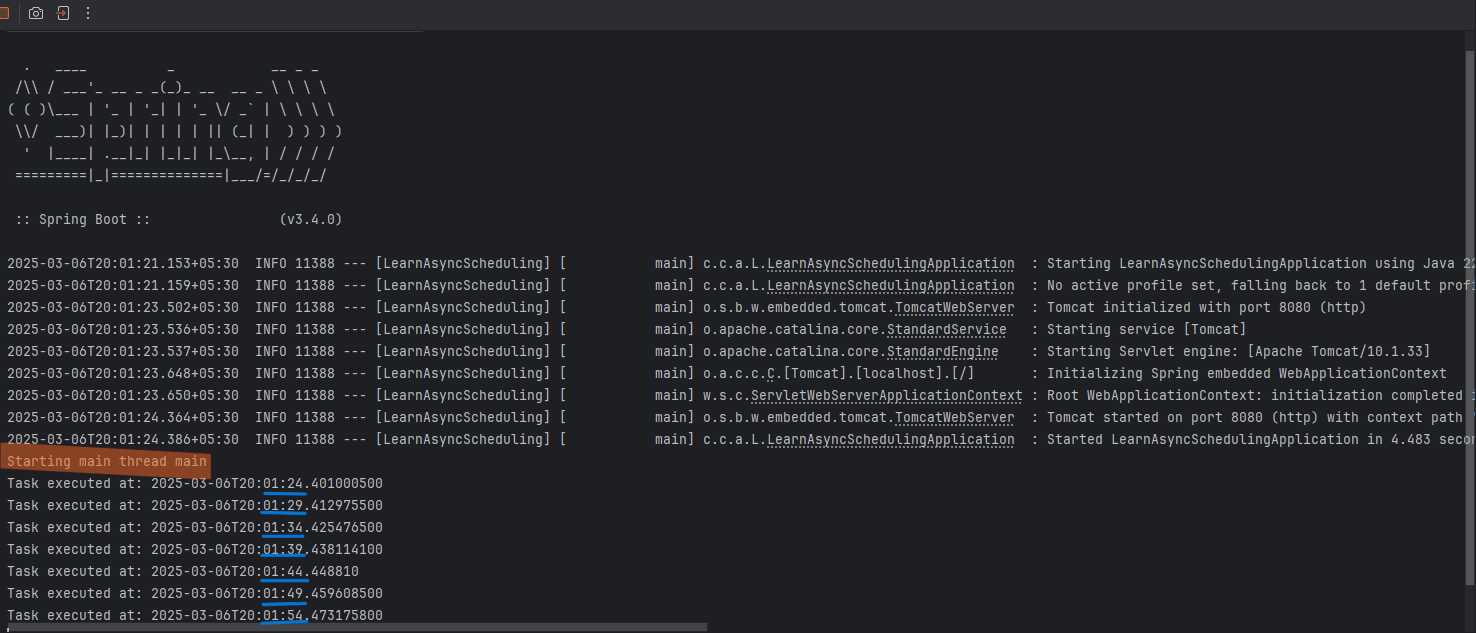
fixedDelay:
Runs 5 seconds after the last task completesinitialDelay
→ The first execution of the task is delayed (using this property) and then continued according to either fixedRate or fixedDelay.
Output
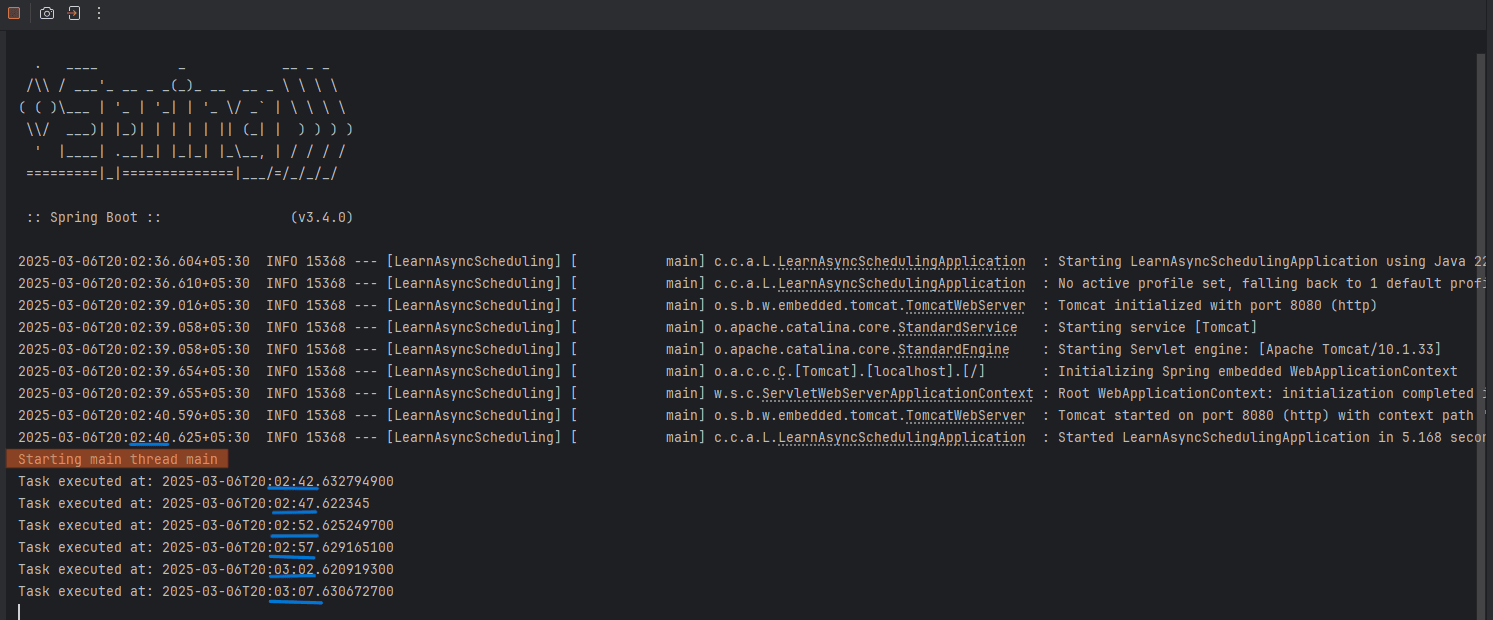
cron→ It uses a cron expression to have complex scheduling patterns (e.g., daily, weekly, or specific times).
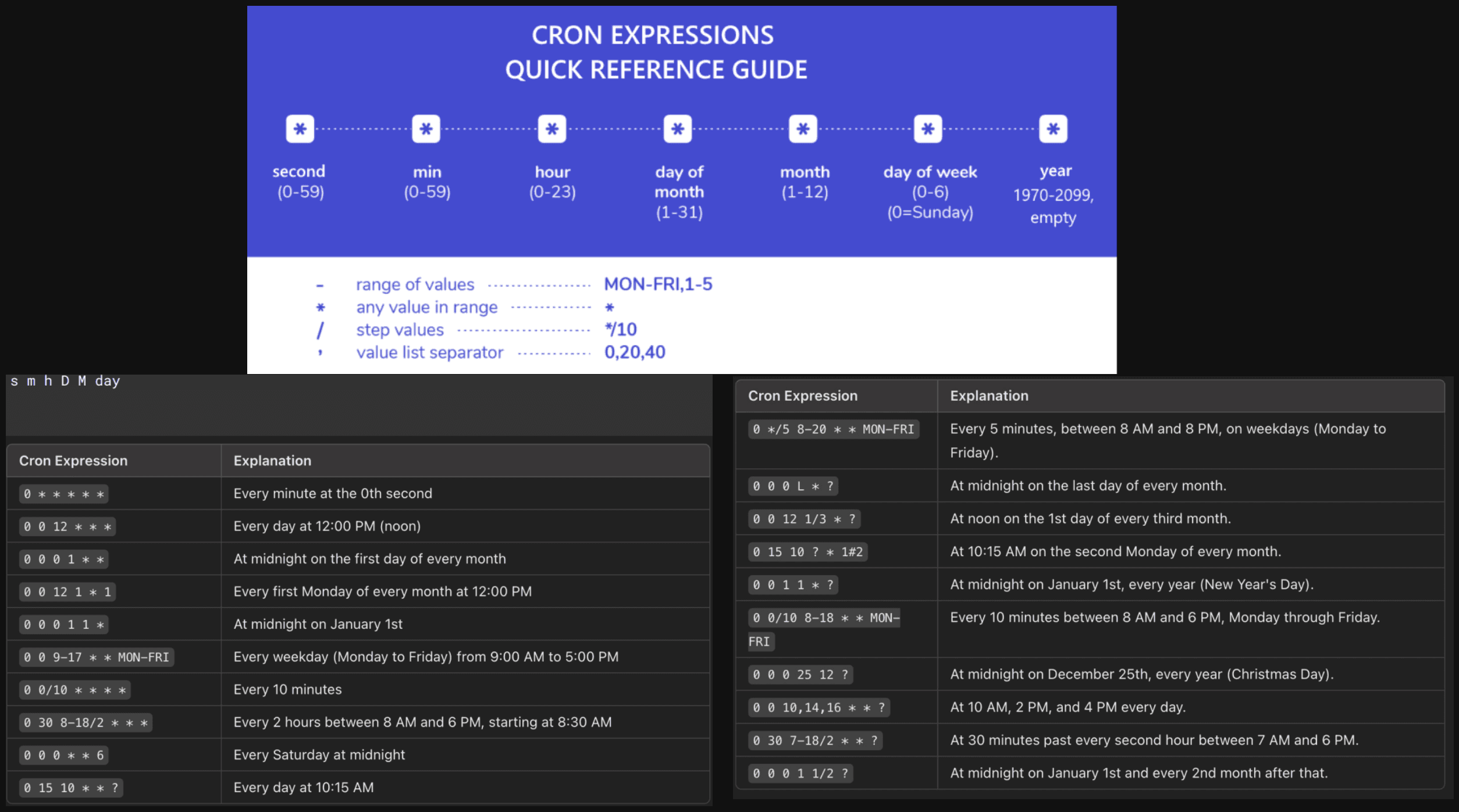
Output
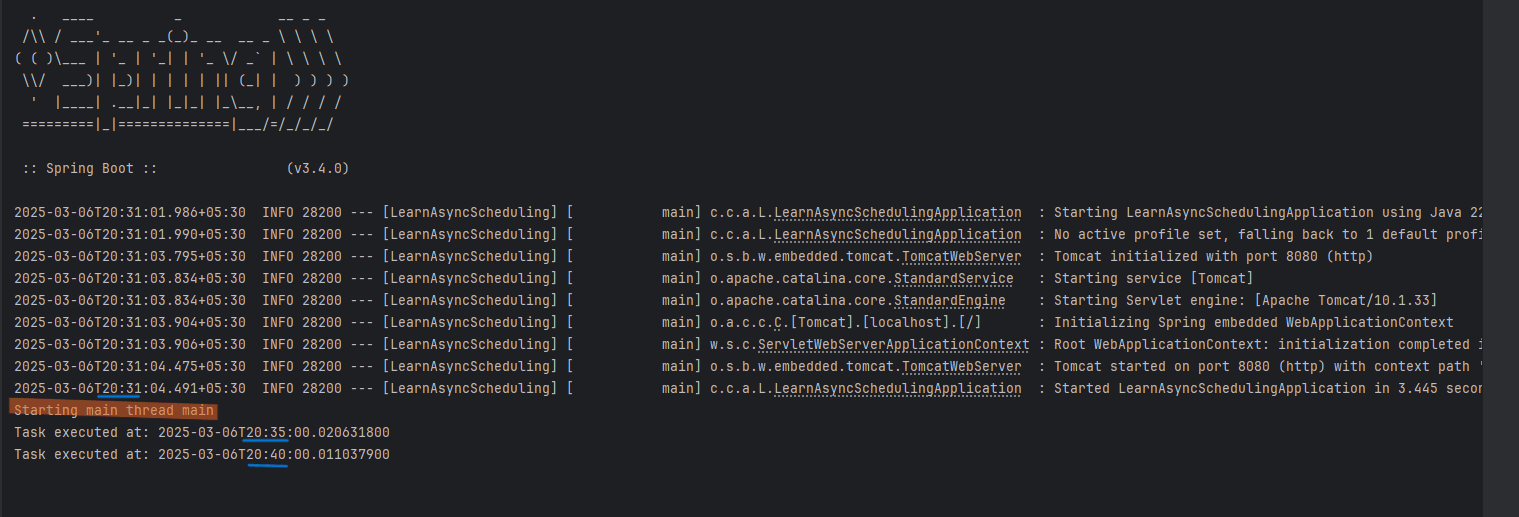
How @Scheduled works Inside Implementation?#
When a method is annotated with @Scheduled
, the following actions are performed by Spring:
- Register the method as scheduled tasks.
- Delegate the task execution to an implementing class of the
TaskScheduler
interface. - Lastly, schedule them by a thread pool (the default one is
ScheduledExecutorService
).
Output
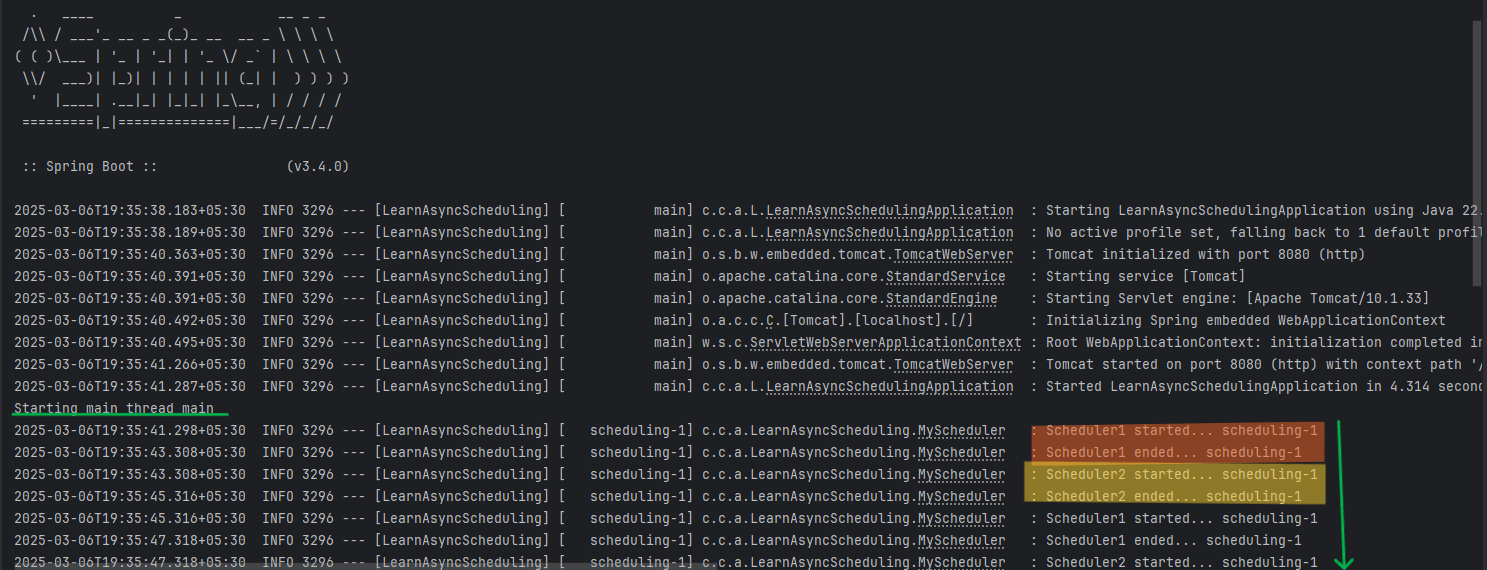
Default Behavior#
@Scheduled
runs the tasks on a single-threaded executor by default. If you schedule more than one task, they execute one after the other sequentially, not in parallel.
How to enable the concurrent execution#
To make them run simultaneously, define a ThreadPoolTaskScheduler
to be a custom TaskScheduler
for the thread pool.
Execution Flow#
@Scheduled
uses Spring's TaskScheduler to get that working.TaskScheduler
will now be a ThreadPoolTaskScheduler (Spring's default implementation) by default.ThreadPoolTaskScheduler
internally delegates their executions easily to the ScheduledExecutorService.
TaskScheduler#
An interface in the Spring scheduling framework that is capable of scheduling tasks dynamically at specific intervals or point-in-time will be TaskScheduler
.
Essential characteristics:#
- Dynamic Scheduling: Scheduling tasks programmatically with fixed rate, fixed delay, or cron specifications.
- Granular Control: Create, cancel, or reschedule a task from anywhere in the application.
- Cron Expression Support: Allows precise scheduling based on cron expressions.
- Thread Pooling: Integrates with**
ThreadPoolTaskScheduler
** to perform tasks in parallel rather than more efficiently.
Configuring TaskScheduler#
- Spring Boot makes an out-of-the-box provided default
TaskScheduler
throughThreadPoolTaskScheduler
, where this uses Java'sScheduledExecutorService
. - To allow concurrent executions, define a new
ThreadPoolTaskScheduler
bean with a configurable thread pool size.
- Tasks can be scheduled dynamically by using
TaskScheduler.schedule()
, which supports fixed delay, fixed rate, and cron expression.
Static Scheduling (@Scheduled
)
Dynamic Scheduling (TaskScheduler.schedule()
)
Output#
- Output Without Dynamic Scheduling (
@Scheduled
)- The task runs automatically at a fixed rate.
- It uses the default single-threaded
TaskScheduler
, meaning tasks run sequentially. - No manual control over task execution.
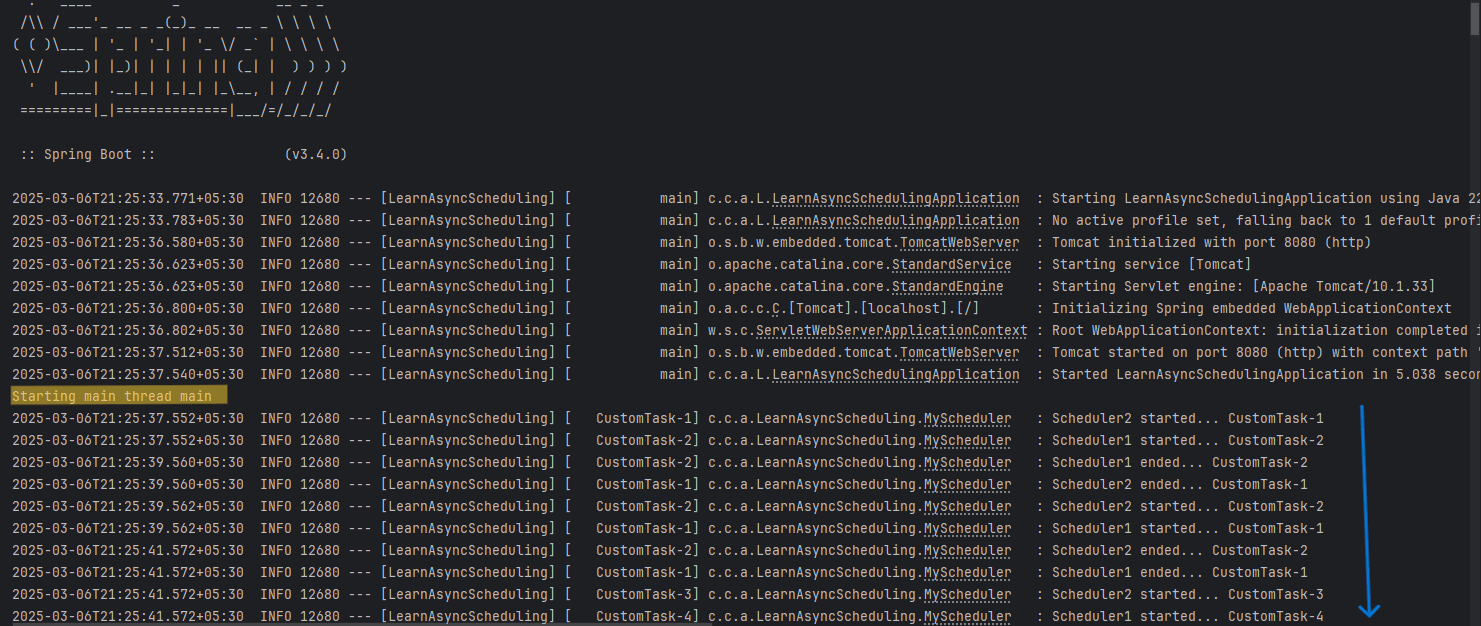
- Output With Dynamic Scheduling (
TaskScheduler.schedule()
)- The task executes only once dynamically at the scheduled time.
- It does not repeat automatically like
@Scheduled
. - Allows more flexibility (e.g., run at specific user-defined times).
- Uses a custom thread pool, enabling parallel execution.
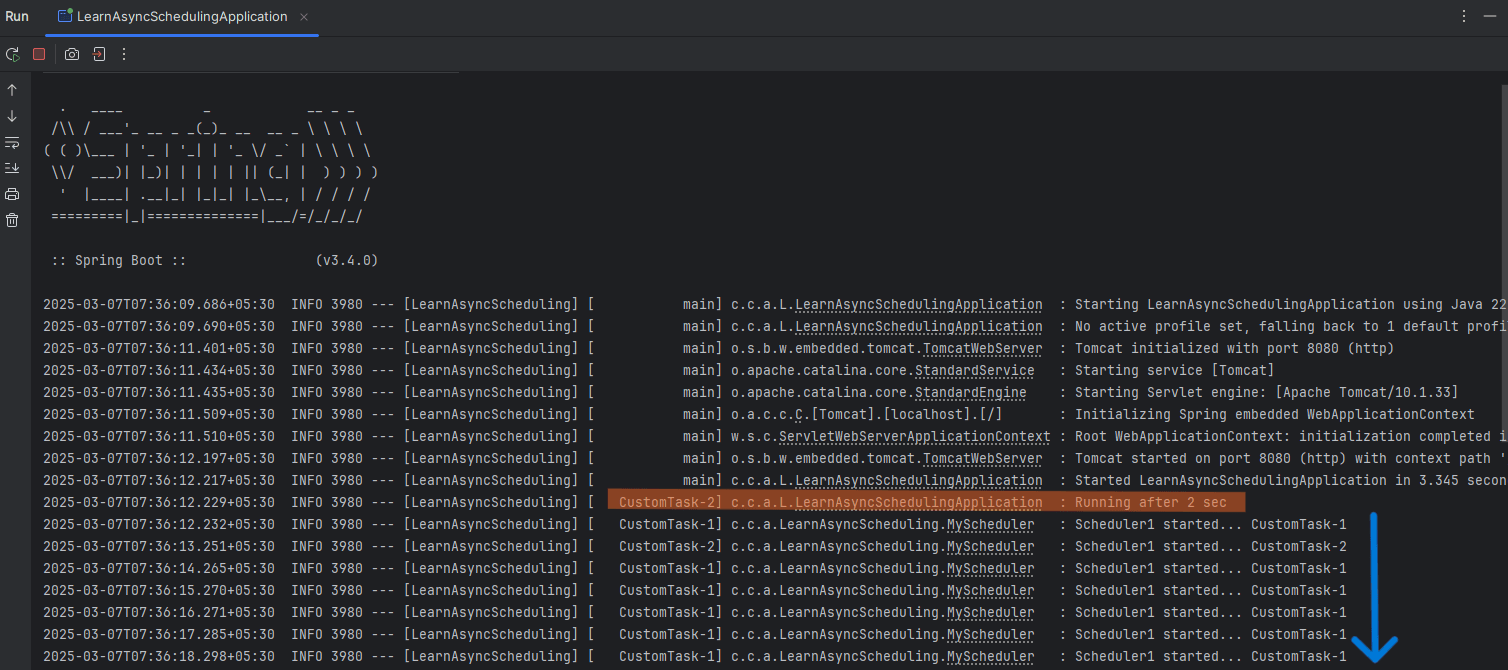
Key Benefits:#
- Supports concurrent execution through multiple threads.
- Supports dynamic scheduling, rescheduling, and canceling of tasks.
- It is much faster than the default single-threaded scheduler.
Final Comparison: Static vs. Dynamic Scheduling#
Feature | @Scheduled (Static) | TaskScheduler.schedule() (Dynamic) |
---|---|---|
Execution Type | Fixed interval | Manually scheduled |
Repetition | Yes, automatically repeats | No, executes once unless rescheduled |
Threading | Default single-threaded | Uses a thread pool (if configured) |
Use Case | Periodic tasks like log rotation or auto-backups | Dynamic tasks like delayed processing or user-scheduled jobs |
Conclusion#
This article elaborated on task scheduling in Spring Boot through the use of @Scheduled
and TaskScheduler
to perform task automation at specified intervals or otherwise. It also contains the important methods in scheduling, how to configure ThreadPoolTaskScheduler
for concurrent execution, and real-life use cases such as notification logging, data cleanup, and API polling, among others.