Spring boot Dev tools
Introduction#
Spring Boot DevTools is a development toolset designed to enhance the productivity of developers by providing features like automatic restart, live reload, and property overrides. It simplifies the process of testing and tweaking applications during development by automatically applying changes without requiring a manual restart. DevTools also provides helpful development-time features such as disabling caching and enabling detailed debugging, making it easier to iterate quickly and see results immediately. It’s ideal for speeding up the feedback loop in local development environments.
Installing DevTools#
Add DevTools Dependency:
Make sure you have the spring-boot-devtools dependency in your pom.xml or build.gradle file.
After this, set the IntelliJ settings to allow restarting.
Ide Setting#
- In IntelliJ IDEA, you can enable the "Build project automatically" option by going to Settings > Build, Execution, Deployment > Compiler. Checking this option allows the IDE to automatically compile your project whenever changes are made, which works well in conjunction with Spring Boot DevTools to trigger the automatic restart feature. Make sure to also enable "Allow auto-make to start even if developed application is currently running" under the same settings for optimal functionality.
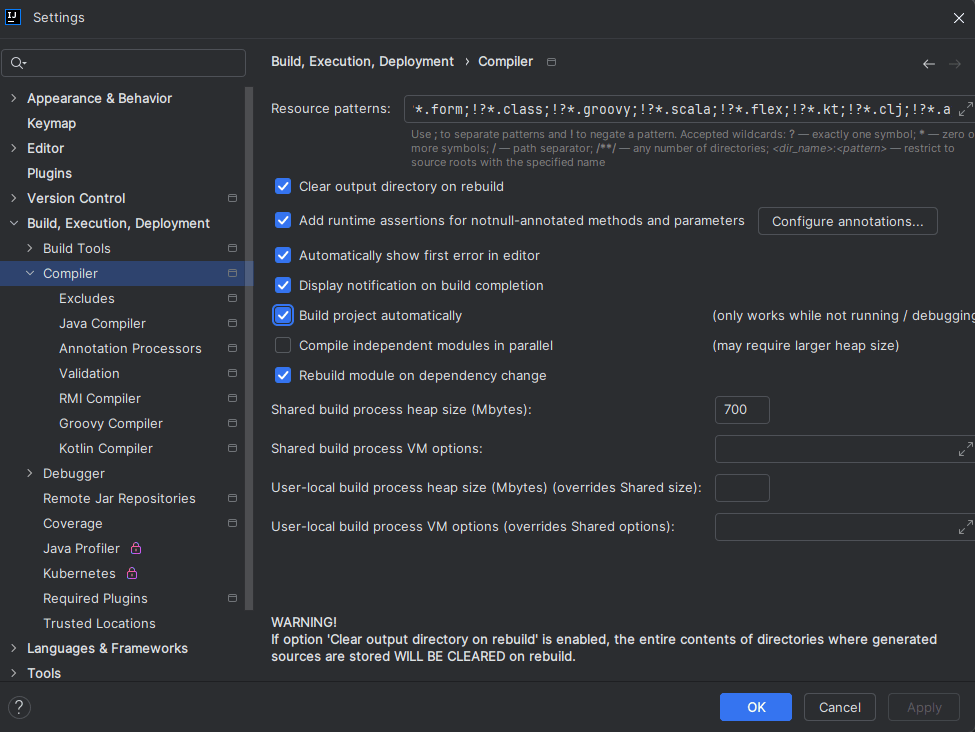
- After that, go to Advanced Settings and check whether the option 'Allow auto-make to start even if developed application is currently running' is enabled. If it's not, enable it and then press OK.
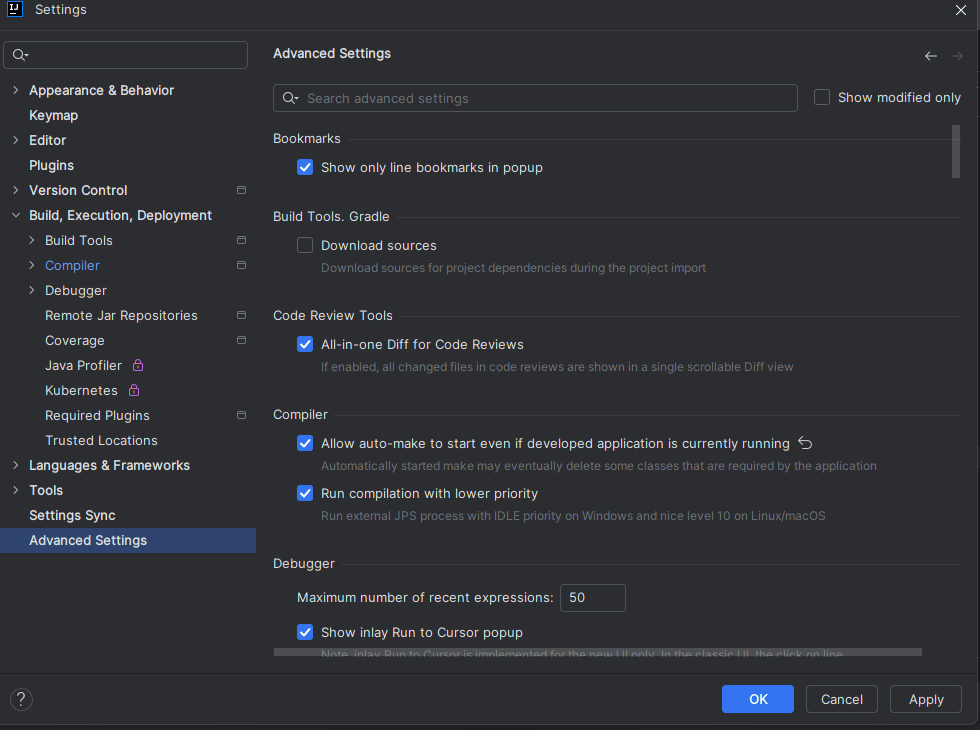
Implementations#
If we check our DTO class, we can see the ‘id’ attribute is present. After running the application, we can send a request using Postman.
Now, if we change the attribute from ‘id’ to ‘productId’ while the application is running.
And then send a POST request to create a product, you will see that the response still contains ‘id’ instead of ‘productId’.
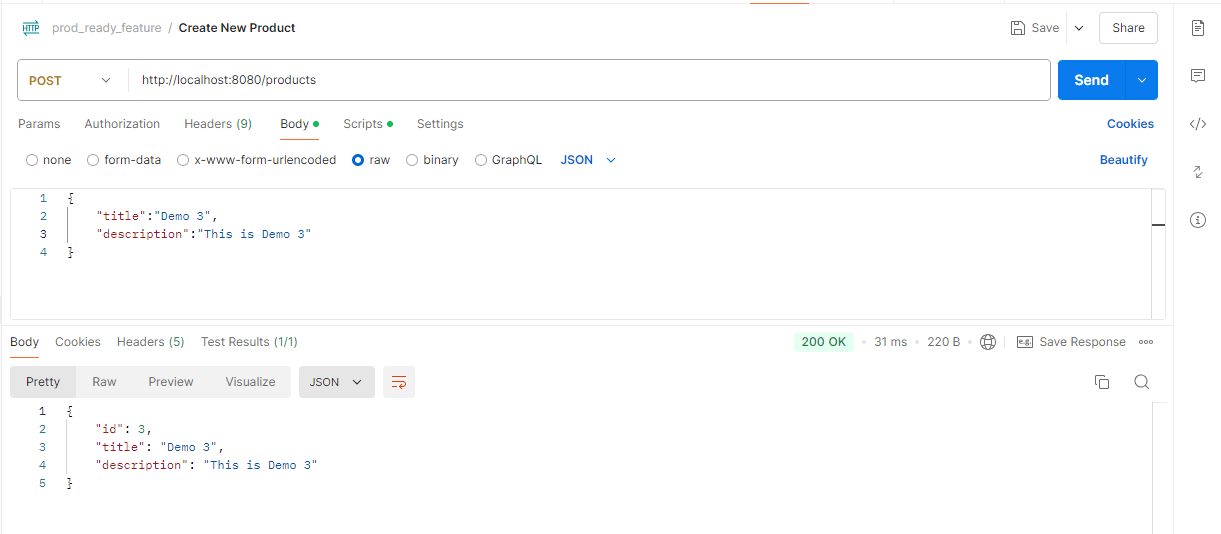
However, when you send a second request, the application automatically restarts, and the response will then include ‘productId’ instead of ‘id’. When working on it, ensure that spring.jpa.hibernate.ddl-auto=update is set in the application properties instead of create. If you set it to create, the productId will be null.
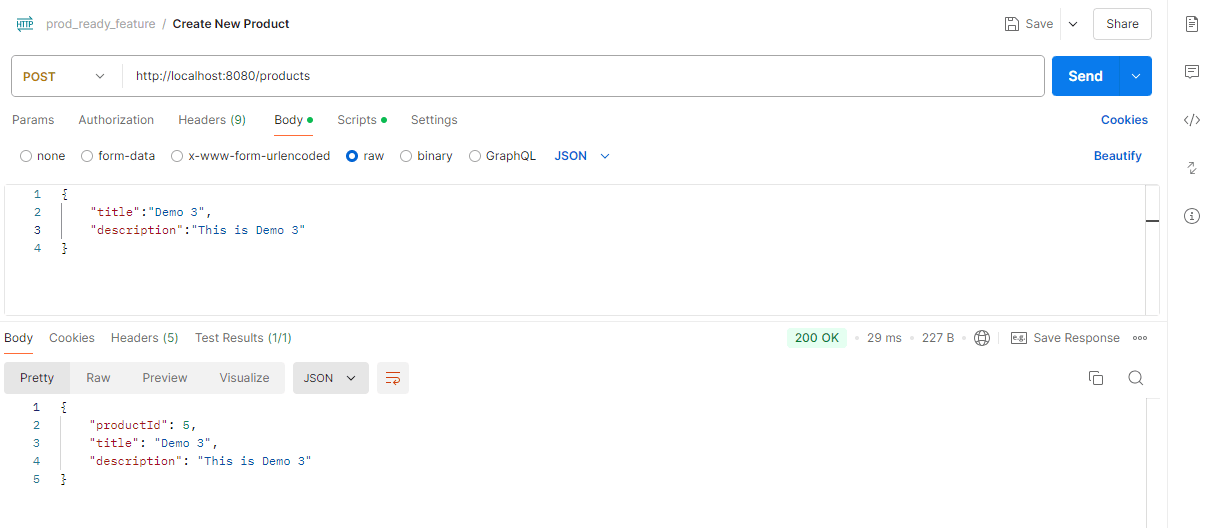
Automatic Restart#
Automatic Restart is a feature of Spring Boot DevTools that enhances developer productivity by automatically restarting the application when it detects changes in the classpath. Instead of manually stopping and restarting the application after every code change, DevTools monitors the project files for updates (such as changes in classes or resources) and triggers a restart automatically.
Key aspects of Automatic Restart:#
- It applies only to the classpath entries that belong to the project, meaning changes in external libraries won’t trigger a restart.
- Restarts are faster than a full application restart because Spring Boot only reloads the affected parts of the application, reusing the already loaded classes.
- It helps maintain the application state in a development environment by allowing quick feedback from code changes.
Useful configurations for Dev-tools#
spring.devtools.restart.enabled=false
: Disables automatic restarts. Useful when you want to manually control restarts during development.spring.devtools.restart.enabled=true
enables the automatic restart feature in Spring Boot DevTools. When set to true, the application will automatically restart when it detects changes in the classpath, such as modified classes or resources. This feature is particularly useful during development, as it allows for quicker testing of changes without the need to manually restart the application.spring.devtools.restart.exclude=static/**,public/**
: Specifies patterns for files or directories that should be excluded from triggering a restart. This helps avoid unnecessary restarts for static resources.- Navigate to a specific package in your project, right-click on it.
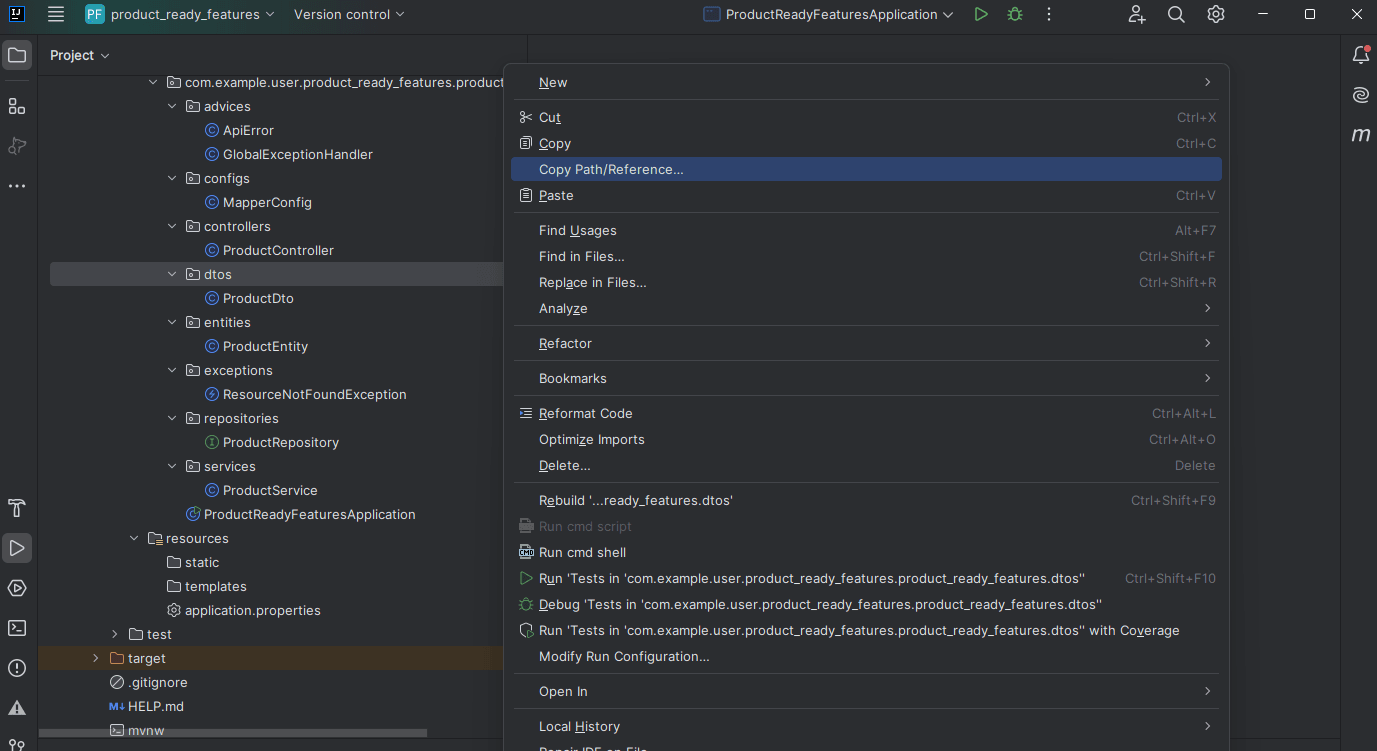
- And select Copy Path/Reference.
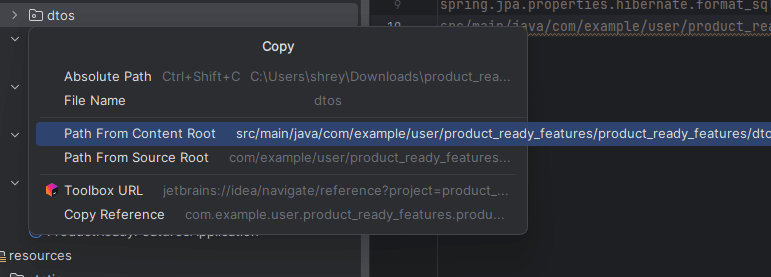
- Then, copy the content root path and paste it into the value of
spring.devtools.restart.exclude
. Like thisspring.devtools.restart.exclude=src/main/java/com/example/user/product_ready_features/product_ready_features/dtos/**
spring.devtools.restart.pollInterval=20
: Sets the interval (in milliseconds) for checking changes in the classpath. A shorter interval means faster detection of changes but may increase CPU usage.
spring.devtools.restart.quietPeriod=10
: Defines a delay (in milliseconds) before a restart is triggered after changes are detected. This helps avoid multiple rapid restarts when making several changes in quick succession.
Conclusion#
This article explores Spring Boot DevTools, a powerful toolset designed to boost developer productivity with features like automatic restart, live reload, and property overrides. It simplifies the development process by enabling seamless testing and iteration without manual restarts. Proper configuration ensures efficient workflows and quick feedback for code changes.