Spring Boot Default Caching
Introduction:#
Spring Boot Caching allows for easier integration and configuration of caching mechanisms. Spring Boot provides a Cache Abstraction API to integrate various caching solutions (e.g., Ehcache, Redis, Caffeine). It simplifies caching with annotations like:
@Cacheable
: Stores method results in the cache.@CacheEvict
: Removes entries from the cache.@CachePut
: Updates the cache with a new value.@EnableCaching
: Enables caching in the application.
You can configure cache providers via application.properties
(e.g., Ehcache, Redis). Caching improves performance by reducing redundant data processing and database calls.
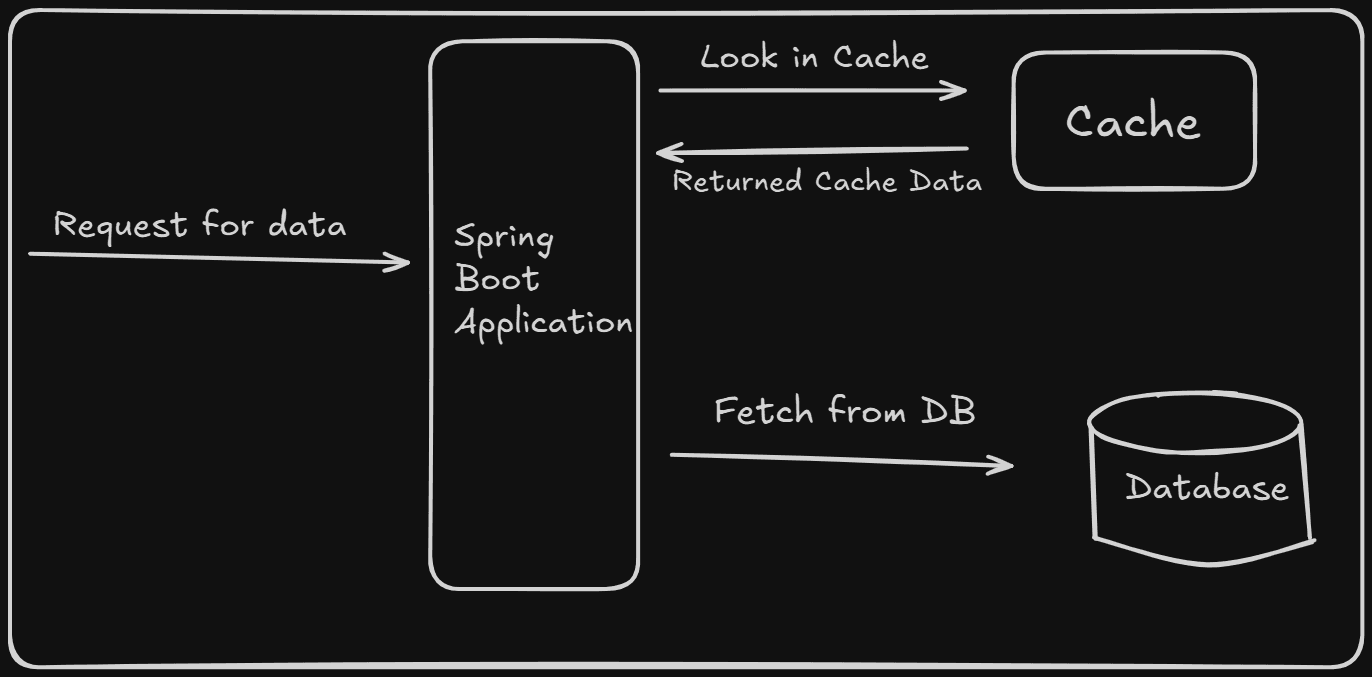
Spring Boot Caching Annotations#
@EnableCaching
- Description: Enables caching in the Spring Boot application.
- Scope: Class-level annotation (usually on the main application class or you can create a configuration class and apply on it).
- Behavior: Configures a default
CacheManager
and uses an in-memory cache (based onConcurrentHashMap
) by default.
@Cacheable
- Description: Used at the method level to cache the result of the method. If the same method is called with the same parameters, the cached response is returned without executing the method again.
- Key Attribute:
cacheNames
: Specifies the name of the cache to store the result (in this case,"employees"
).key
: Defines the key used to store and retrieve the cached value (#id
means the method parameterid
is used as the cache key). If you have multiple keys then you can write them like thiskey = "{#id,#name,....}"
Behavior:
If the cache already contains the result for the given id
, the method will not execute, and the cached result is returned.
If the result is not in the cache, the method executes and stores the result in the cache.
Execution Flow#
- Method Call: When
getEmployeeById(Long id)
is called:
- Spring intercepts the call due to the
@Cacheable
annotation.
- Cache Check:
- Cache Hit: If the
id
exists in the cache, the cachedEmployeeDto
is returned, skipping the repository call. - Cache Miss: If the
id
does not exist in the cache, the method executes.
- Repository Call: The employee is fetched from the database (or an exception is thrown if not found).
- Cache Update: The result is stored in the cache for future use.
- Return Result: The
EmployeeDto
is returned to the caller. @CachePut
- Description: Ensures the cache is updated after the method is executed. Unlike
@Cacheable
, the method is always executed, and its result is added to the cache. - Key Attribute:
cacheNames
: Specifies the cache where the result will be stored (employees
in this case).key
:- Defines the unique identifier for the cache entry (also known as the cache key).
- In this case:
#result
: Refers to the object returned by the method (i.e., the method's return value)..id
: Refers to theid
field of the returned object.- So, the cache key is set to the
id
of the object returned by the method.
- Description: Ensures the cache is updated after the method is executed. Unlike
Note: Why use
#result.id
?
- The
id
field uniquely identifies the object (e.g., an employee in the database).- Using
#result.id
ensures that each employee's data is cached under their unique identifier.- Future requests to fetch the employee by their
id
will quickly retrieve the data from the cache.
Execution Flow
- Method Call: When
createNewEmployee(EmployeeDto employeeDto)
is called:- The method executes, even if the cache has an entry for the employee.
- Database Interaction:
- Checks for existing employees with the same email.
- Saves the new employee to the database.
- Cache Update:
- After saving, the new employee is stored in the cache.
- Result Returned: The
EmployeeDto
object is returned to the caller. @CacheEvict
- Description: Used to remove entries from the cache. It can clear specific cache entries or all entries in the cache.
- Key Attributes:
value
: The cache name.key
: Specifies which entry to evict.allEntries
: Iftrue
, clears all entries in the cache.
Execution Flow
- Method Input: An
id
(e.g.,101
) is passed to thedeleteEmployee
method. - Check Existence:
- The
existsById(id)
method checks if an employee with the givenid
exists in the database.
- The
- Delete Operation:
- If the employee exists, the
deleteById(id)
method removes it from the database.
- If the employee exists, the
- Cache Eviction:
- The
@CacheEvict
annotation ensures the cache entry forid
is removed from the"employees"
cache.
- The
Spring Boot Caching Internal working#
Imagine This Scenario:#
You are a student preparing for exams, and you’ve created sticky notes for each subject to quickly review important topics (this is your cache).
Now, let’s map the caching process step by step to your exam preparation:
1. When a Method Annotated with @Cacheable
is Called#
- Analogy: Suppose you are revising a topic like "Java Basics". Before searching through your book (the actual method), you check your sticky notes (the cache) to see if you’ve already written down the summary.
- Spring’s Role: Spring intercepts the call to check if the result for that topic (or method) is already cached.
2. The CacheManager
Retrieves the Cache#
- Analogy: You have a folder for sticky notes labeled "Programming Subjects". Spring, like you, first goes to the folder to check for the sticky note (cache entry) named "Java Basics".
- Technical Step: The
CacheManager
is like your folder organizer. It retrieves the cache for the name specified in the annotation, e.g.,"employees"
,"products"
.
3. Cache Hit or Miss#
Hit: The Key Exists#
- Analogy: If you find the sticky note for "Java Basics" in your folder, you don’t need to open your book. You simply read the summary and save time.
- Spring’s Role: If the key (method arguments) exists in the cache, it directly returns the cached result.
Miss: The Key Does Not Exist#
- Analogy: If you don’t have a sticky note for "Java Basics", you’ll need to open your book, study the topic, and then create a sticky note for future reference.
- Spring’s Role: If the key doesn’t exist, the method is executed to get the result.
4. Storing in the Cache (put
Method)#
- Analogy: After studying "Java Basics", you quickly write down a summary on a sticky note and put it in your folder for next time.
- Spring’s Role: After the method executes, Spring saves the result in the cache using the
put
method.
5. Return the Result#
- Analogy: Whether you read from the sticky note (cache) or the book (method execution), you now have the knowledge to answer questions about "Java Basics".
- Spring’s Role: Finally, the result (either from the cache or method execution) is returned to the caller.
Visualizing the Process#
- Calling Method: You need "Java Basics".
- CacheManager: Looks in the "Programming Subjects" folder.
- Cache Check:
- Sticky note exists → Read and return it.
- Sticky note doesn’t exist → Study the book, write a sticky note, and save it.
- Return: You now have the answer.
Conclusion#
This article explains Spring Boot's default caching mechanisms, focusing on annotations like @Cacheable
, @CachePut
, and @CacheEvict
to improve performance. It highlights how caching reduces redundant database calls and method executions, enhancing scalability and efficiency. The article also discusses the importance of configuring cache providers for optimized resource usage.