Microservice Advance: Introduction to the API Gateway Filters
Introduction#
The concept of an API Gateway is known as a single point of entry into a system for routing management and request securing under a microservices architecture. The API Gateway Filters develop the functionality of modifying incoming and outgoing traffic while allowing security, performance, and scalability. The filters help with authentication, rate-limiting, logging, request transformation, and response handling. Using API Gateway Filters, enterprises more efficiently manage the API, control flow directed toward the API Gateway, and enforce security policies. Consequently, they are considered an eminent part of microservices infrastructure.
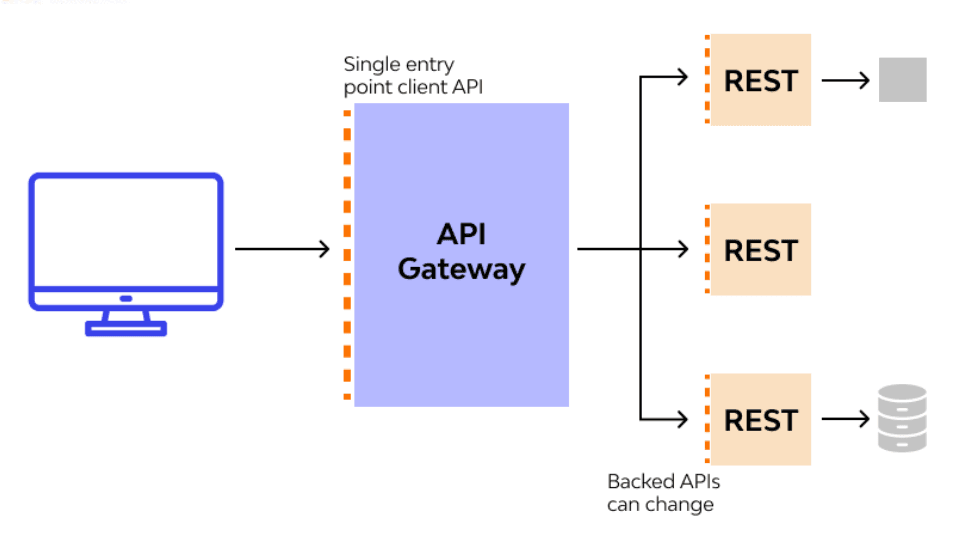
Real-Life Analogy#
An API Gateway is analogous to an airport security checkpoint where requests are controlled and managed before they reach backend microservices.
Global Filters (Universal Security across the Airport):
This means that they apply to all requests, in a way similar to passport checks and baggage screening for all passengers.
For example, JWT authentication, logging, and rate limiting—all ensure every request is secure and monitored.
Route-Specific Filters (Precaution for Specific Flights):
They apply only to selected API routes, almost similar to how some flights mandate checks on visas or access for business class passengers.
An instance is added security for payment APIs, file-size limits for uploads, and priority processing for VIP users.
In as much as airports enforce security while allowing freedom, API Gateways ensure security, efficiency, and customizability using global and route-specific filters.
API Gateway Filters:#
API Gateway Filters intercept, modify, and enhance API requests and responses, enabling authentication, logging, rate limiting, and request transformation at a centralized entry point before reaching microservices.
Types of API Gateway Filters:#
- Global Filter: Applicable to all requests; engages security, logging, and analytics.
- Route-Specific Filter: Engages specific API traffic for transformations, authentication, and throttling.
What Are API Gateway Filters?#
An API Gateway filter is a middleware component in an API Gateway used to control how the API requests and responses are processed. They are interceptors that alter requests before reaching the backend services and responses before they are returned to the clients.
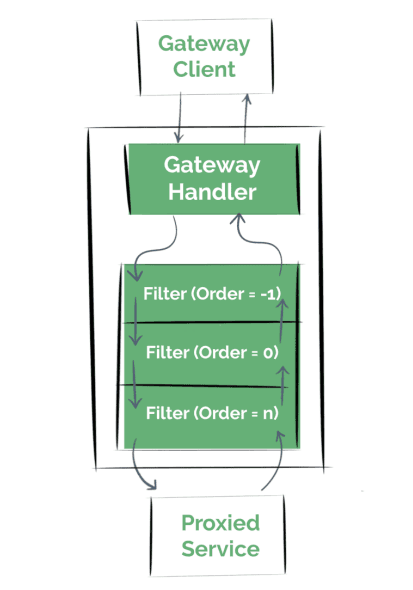
Key Functions of API Gateway Filters:#
- Security: Where authentication and authorization mechanisms are deployed: JWT, OAuth2, etc.
- Traffic Management: Make sure the API does not get overloaded through proper moderation.
- Request and Response Transformation: Changing headers, body, or parameters into one format for sufficient normalization.
- Logging and Monitoring: Even though API performance, request patterns, and security threats are tracked separately elsewhere.
- Load Balancing and Caching: Response times are reduced in some APIs through data that have been cached regularly.
- Error Handling and Routing: Redirects traffic and allows such failures to be dealt with gracefully.
The more popular API gateways, such as Spring Cloud Gateway, Kong, Apigee, NGINX, and AWS API Gateway, have well-integrated features to improve API management and security.
Why Use API Gateway Filters in Microservices?#
It can be a challenge to realize stringent requirements on security, performance, and monitoring from an API perspective due to a microservices architecture, which disperses APIs across various services. API Gateway Filters help address these issues because they implement a centralized approach to API traffic control.
Some of the main advantages of using an API Gateway Filter are:#
- Improved Security: Securing APIs through authorization, IP whitelisting, and encryption.
- Improved Performance: Introducing caching, compression, and rate limiting for reduced response latency.
- Centralized API Governance: Unifying policies across all microservices.
- Transparent Routing of Traffic: Easily redirect, load balance, and control requests to your API.
- Better Developer Experience: Provides backend service developers with the leeway to focus on core business logic with lessened burden related to cross-cutting concerns.
Thus API Gateway Filters ensure the implementation of a mission of API reliability and compliance while improving the user experience in its entire microservice ecosystem.
Global Filter#
In Spring Cloud Gateway, a Global Filter is applicable for all requests in passing API Gateway. To create a custom global filter, the only requirement is to implement the Spring Cloud Gateway GlobalFilter interface and register it in the context as a bean.
Key use cases:#
- Security Enforcement (JWT validation and authentication): Implement security policies such as JWT verification for all APIs.
- Logging and Monitoring (audit logs and request tracing): Collect and analyze request/response data on all microservices.
- Rate Defining and Throttling (protection of APIs): Restrict API abuse by global rate limiting.
- Header Manipulation (modification of request/response headers): Add, remove, or modify headers and request payloads globally.
- Performance Optimization: Cache or compress any incoming or outgoing traffic.
Use Global Filters when the functionality is required across all services (e.g., logging, authentication).
Implementation:#
Here, I am implementing it inside the api-gateway application of the previous e-commerce project.
Global Filter Class:
@Slf4j
(Lombok Annotation) → Enables SLF4J-based logging (log.info(...)
) without manually creating a logger.
If you are not adding Lombok dependency you have to add it for @Slf4j
.
GlobalFilter
→ A Spring Cloud Gateway interface for defining global filters applied to all requests.
Ordered
→ Allows defining the execution order of the filter.
Component
→ Registers this class as a Spring-managed bean, making it available for dependency injection.
ServerWebExchange
→ Represents the web request & response during API Gateway processing.
Mono<Void>
→ A reactive type indicating that the filter logic runs asynchronously.
exchange
→ Represents the HTTP request and response.
chain
→ Calls the next filter in the chain.
then(Mono.fromRunnable(...))
ensures post-processing logic runs after the request is handled.
Spring Cloud Gateway's GlobalLoggingFilter logs incoming requests before being routed to the microservices and logs response statuses after processing. GlobalFilter is implemented to run across the board; Ordered is called to set the execution priority (5). This filter assists in centralized logging, which in turn aids monitoring, debugging, and analytics in such a microservices architecture.
Api-Gateway yml file:
StripPrefix=2
is a route-specific filter that removes the first two segments of the URL path before forwarding the request. Global filters, such as authentication and logging, are automatically applied to all routes and do not require additional configuration.
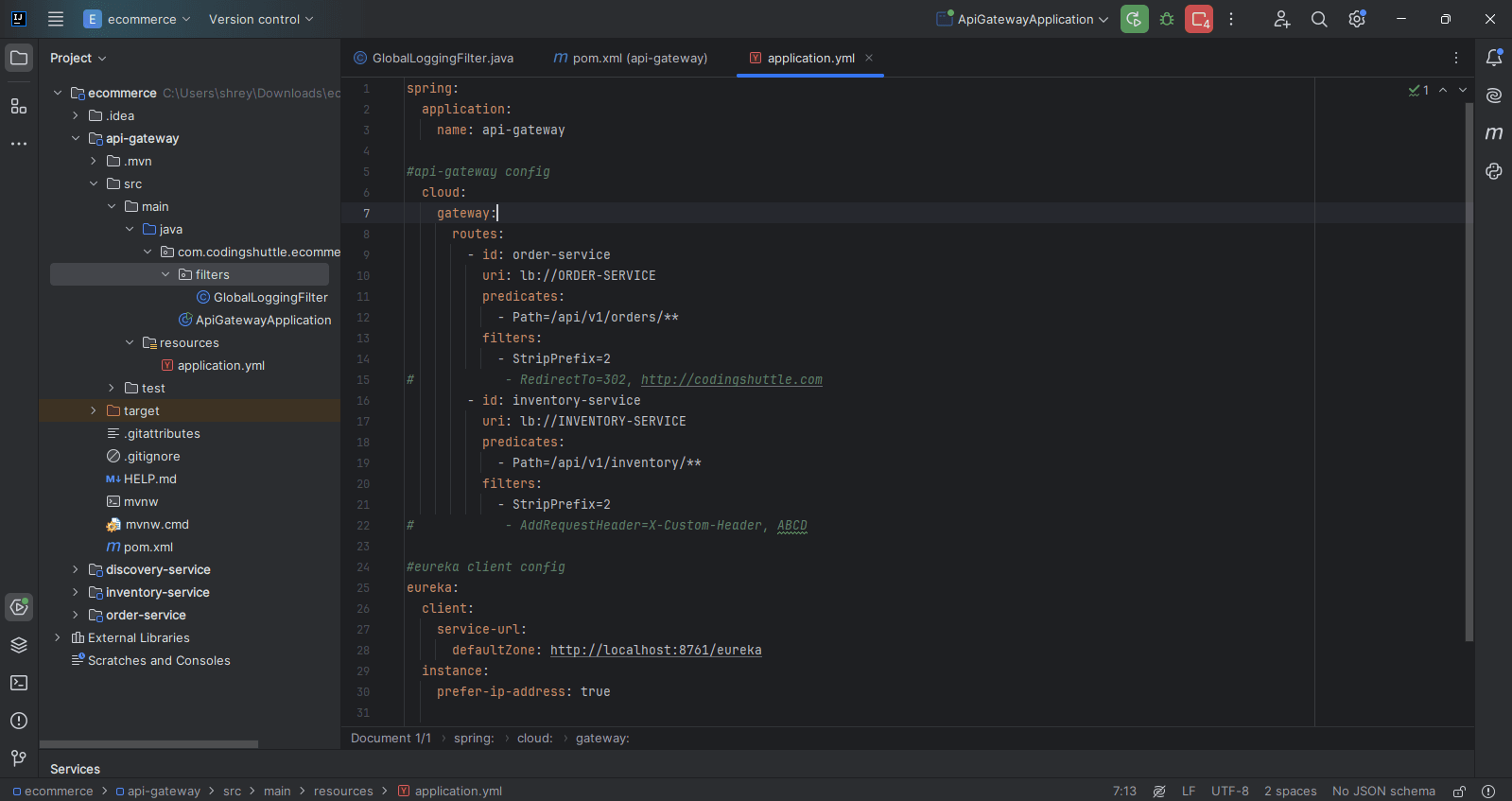
Output#
- Run all services:
After running all services (the sequence is 1. Run Eureka Server (Discovery Service) 2. Run other clients services. 3. Last run gateway service on default port 8080)
- Wait for all registered client services inside Eureka:
if your services are not fully registered before another service tries to communicate with them, you may encounter a "Service Unavailable" exception.
- Hit the url http://localhost:8080/api/v1/orders/core/helloOrders in the Browser or Postman with the request body:
After starting Eureka and all client services, test the API using a browser (for GET requests) or Postman (for all requests). If you are trying to fetch other services you can easily fetch it. Here, we are trying to fetch inventory service data by using api-gateway (port=8080). /api/v1
ignored because of using StripPrefix=2
.
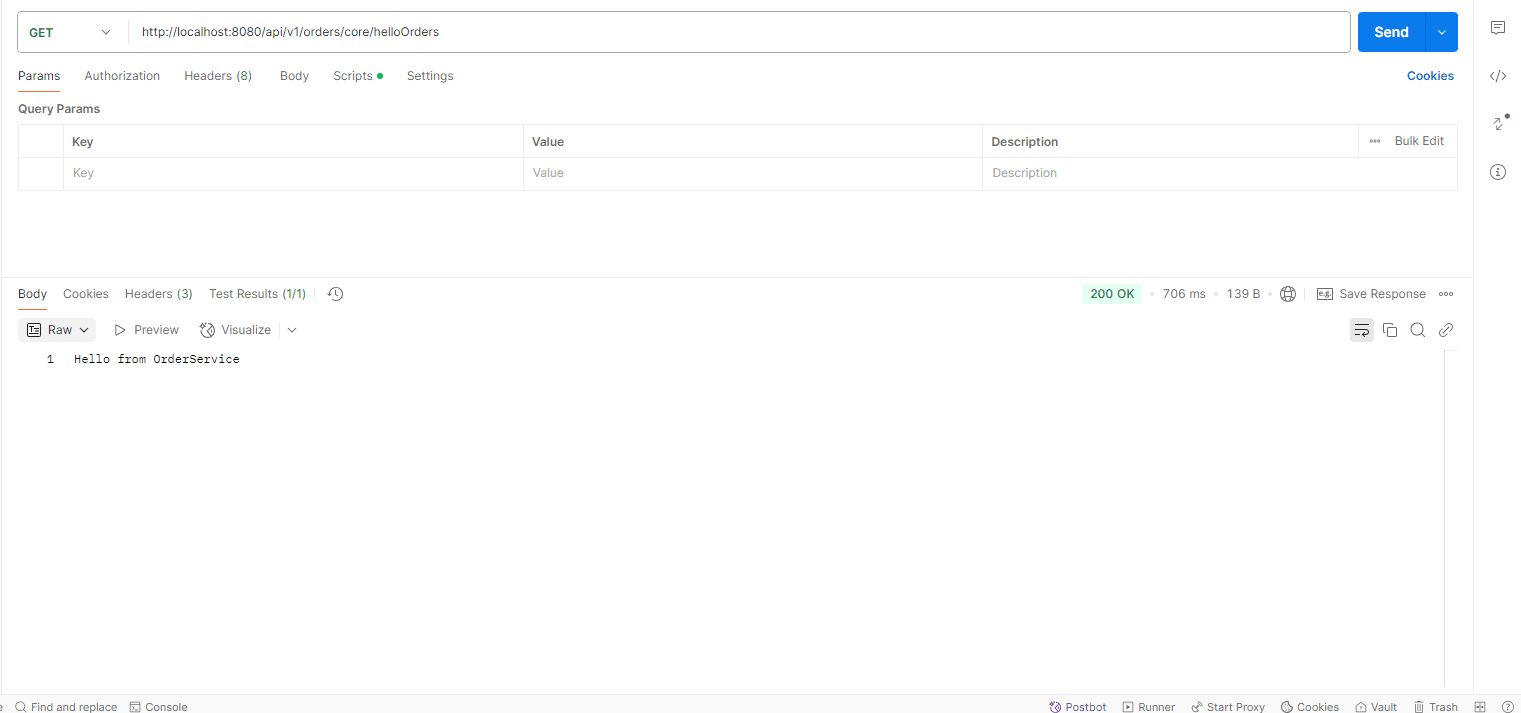
Ide console:
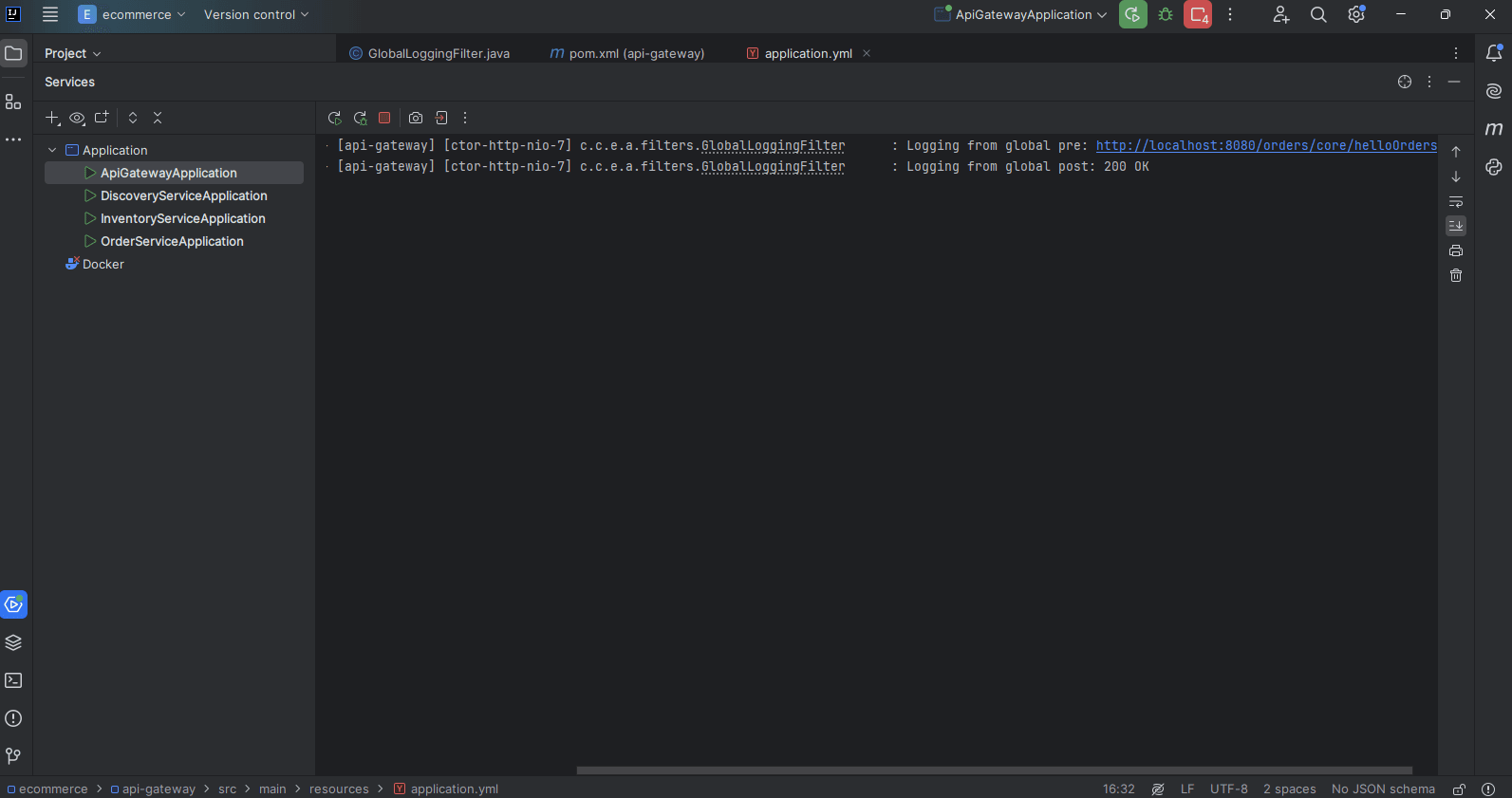
In Spring Cloud Gateway, the GlobalLoggingFilter applies to all APIs, logging the request URI before processing and the response status afterward. Centralized logging helps monitor, debug, and analyze across microservice applications.
Route Specific Filters#
Globally applicable filters provide blanket processing of all requests, but route-specific filters will allow the control of the processing of specific API routes. These filters will allow custom transformations, authentication, request modifications, and traffic shaping on selected endpoints.
Key use cases:#
- Granular Control: Only put filters where the need arises for performance optimization.
- Custom authentication and authorization (per-route security): Different services can have their own exclusive authentication/authorization logic.
- Request and response transformation (headers and payloads manipulation): Modify headers/parameters/payloads into a limited number of APIs.
- Rate limiting (traffic restriction for selected services): Put the traffic limitation only on specific services; the others remain unaffected.
- Dynamic routing and redirection (modification of destinations): Redirection or modification of traffic can be dynamic based on request conditions.
Use Route-Specific Filters when customization is needed for individual services (e.g., request transformation for a payment service).
Implementation:#
Here, I am implementing it inside the api-gateway application of the previous e-commerce project.
Route Filter Class:
This LoggingOrderFilter is a custom filter that belongs to Spring Cloud Gateway. It is responsible for logging the incoming request URIs just before they are passed along the filter chain. It extends AbstractGatewayFilterFactory and is therefore route-specific; that is, it applies to specific routes only as defined in the API Gateway configuration.
The constructor calls the super constructor with Config.class, an empty static class for configuration purposes. The apply() method contains the logic for the filter, which logs the request URI before passing on the request to the next filter in the chain for processing. Since it is a route-level filter, it gets executed before any global filters.
Spring Cloud Gateway executes specific route filters first, then global filters. Thus, the order of execution depends on whether the filter is global or route-scoped and on its assigned order value. The LoggingOrderFilter ensures that logging occurs early in the processing pipeline for a selective set of routes, thus giving fine control over request handling.
Api-Gateway yml file:
StripPrefix=2
is a route-specific filter that removes the first two segments of the URL path before forwarding the request. Global filters, such as authentication and logging, are automatically applied to all routes and do not require additional configuration. However, route-specific filters must be explicitly added per route in application.yml
or Java configuration.
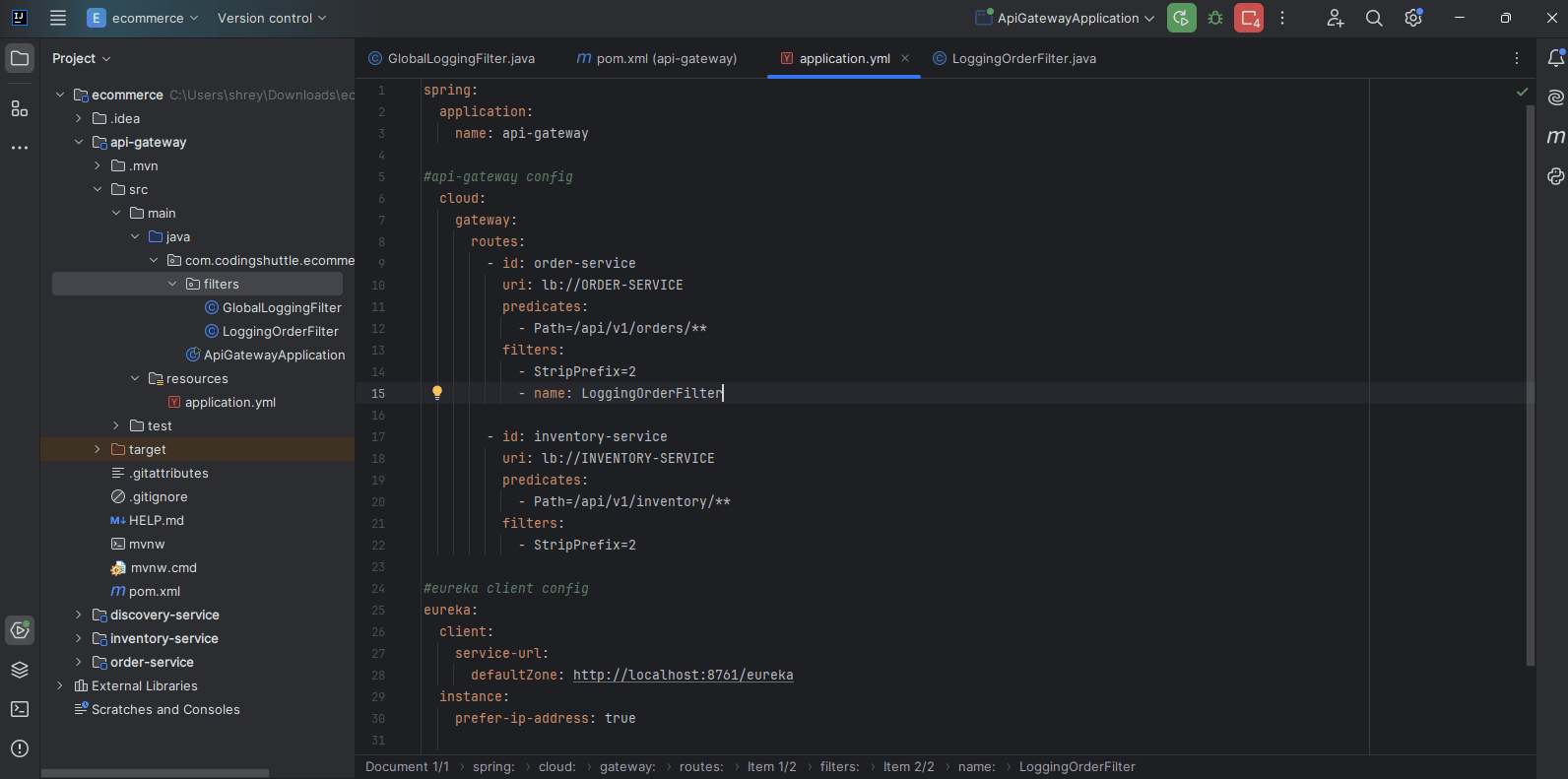
Output#
- Run all services:
After running all services (the sequence is 1. Run Eureka Server (Discovery Service) 2. Run other clients services. 3. Last run gateway service on default port 8080)
- Wait for all registered client services inside Eureka:
if your services are not fully registered before another service tries to communicate with them, you may encounter a "Service Unavailable" exception.
- Hit the url http://localhost:8080/api/v1/orders/core/helloOrders in the Browser or Postman with the request body:
After starting Eureka and all client services, test the API using a browser (for GET requests) or Postman (for all requests). If you are trying to fetch other services you can easily fetch it. Here, we are trying to fetch inventory service data by using api-gateway (port=8080). /api/v1
ignored because of using StripPrefix=2
.
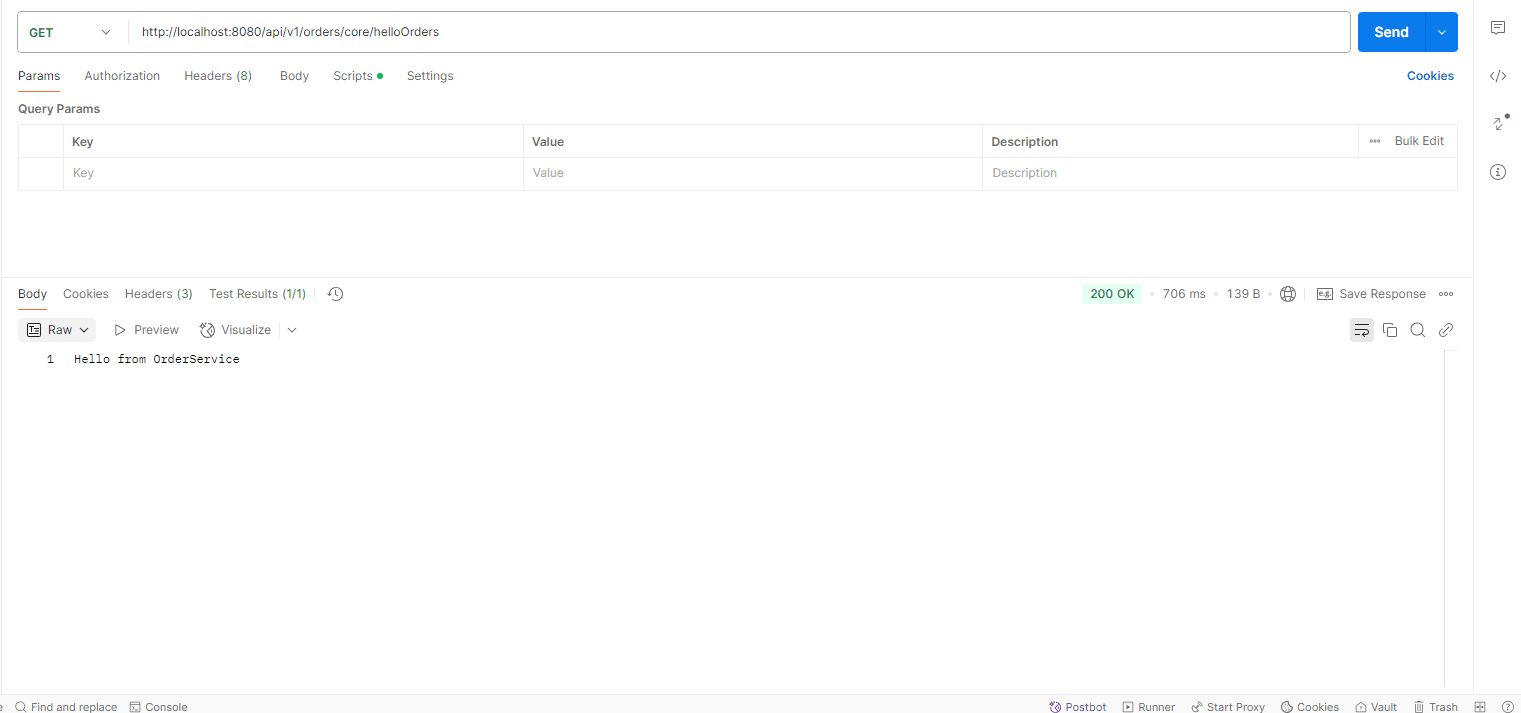
Ide console:
The execution of filters in Spring Cloud Gateway depends on their order. Filters with lower order values execute first, meaning route-specific filters (order = 2) will run before global filters (order = 5). This ordering ensures that more specific filters process requests before broader global filters.
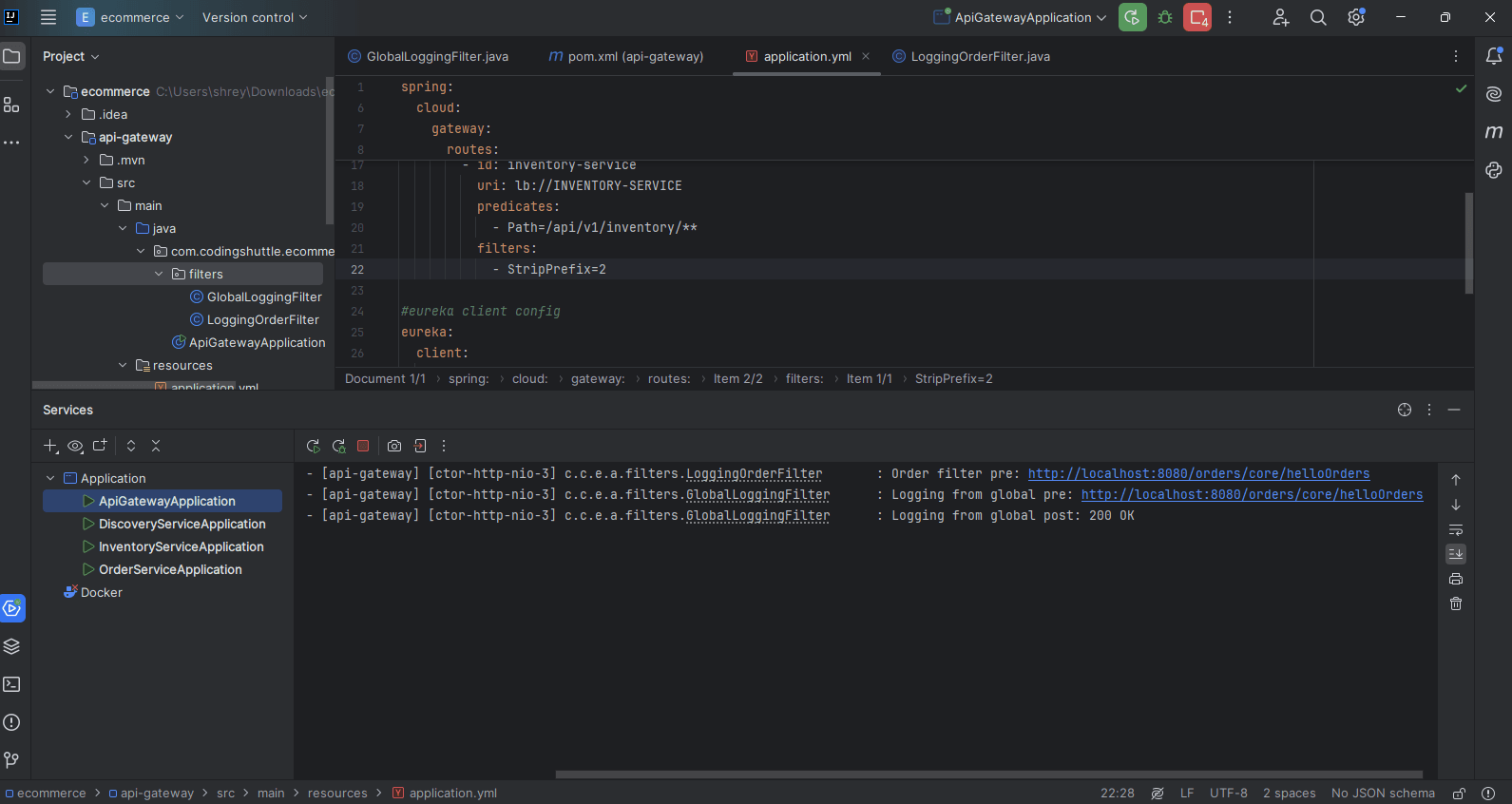
Execution of Filters:#
In Spring Cloud Gateway, filters execute based on priority. The image confirms that route-specific filters (order = 2) execute first, followed by global filters (order = 5). This means route-level filters handle requests before global filters process them, ensuring a structured execution order.
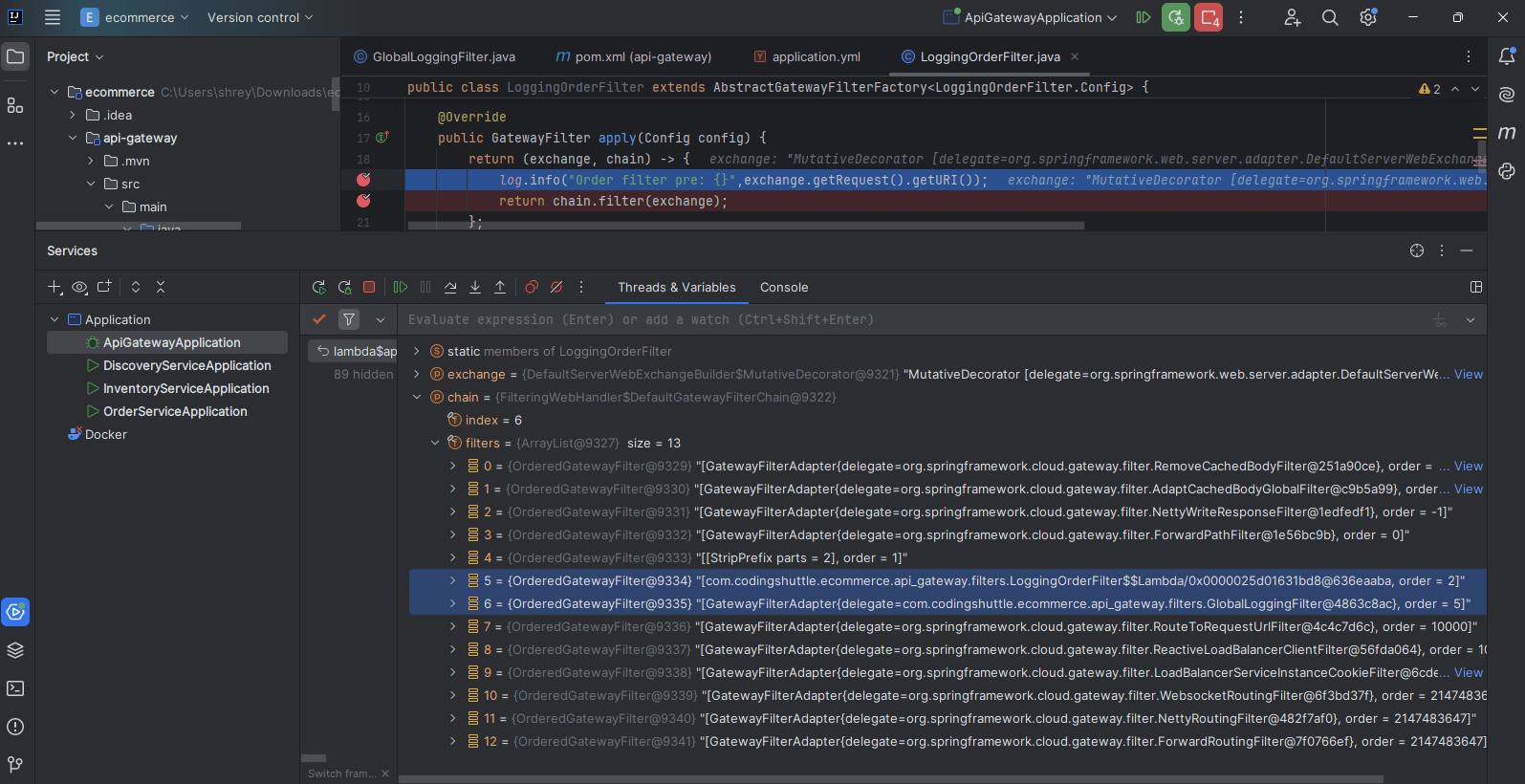
Conclusion#
This article states that API Gateway Filters facilitate microservices by ensuring security, mediating traffic, and processing requests. Global filters apply to everything. Route-specific filters offer custom control on each of the services. Implementing these filters leads to better scalability, security, and performance of API management.