Introduction to Testing in Spring Boot
Introduction :#
Testing in Spring Boot is essential for ensuring that your application works as expected. Spring Boot simplifies testing by providing tools and annotations that integrate well with Spring's ecosystem. The testing framework focuses on two main types of tests: Unit tests and Integration tests.
Types of Tests#
- Unit Tests: Focus on testing individual components or methods, typically without involving the Spring context or any external services (e.g., databases). The goal is to isolate the code and ensure that each unit behaves as expected.
- Integration Tests: Involve the Spring context and external components, such as databases, messaging queues, or APIs, to test how various components work together in a more realistic environment.
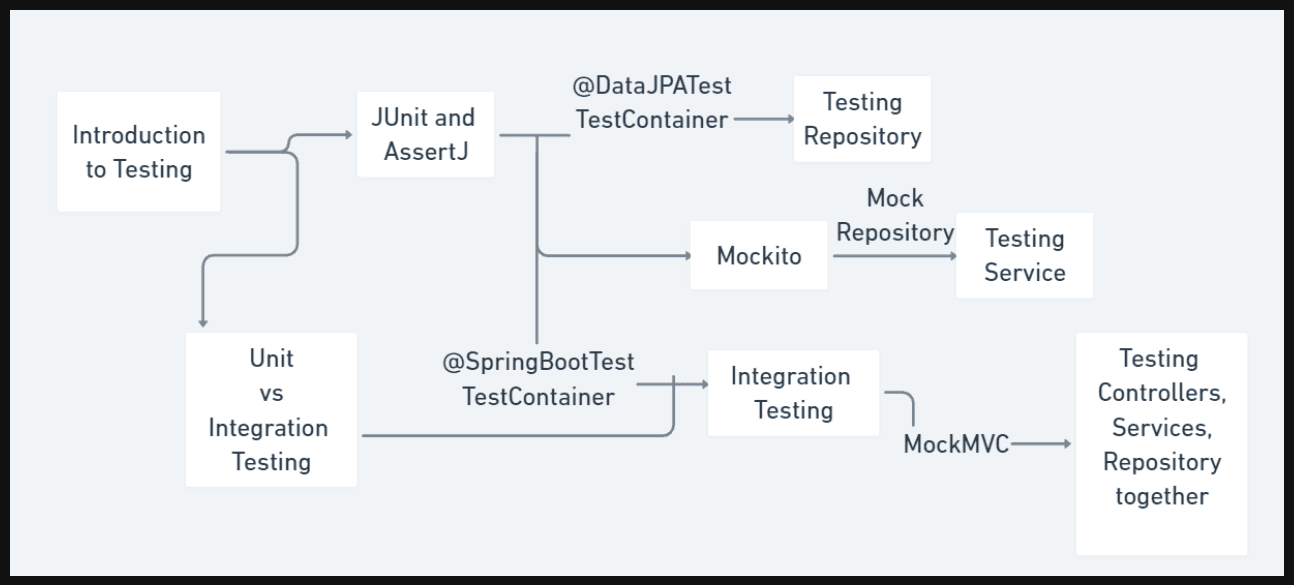
Spring Boot Test Example#
- When you open the Application file inside the ‘src’ folder, you will see the ‘test’ package.
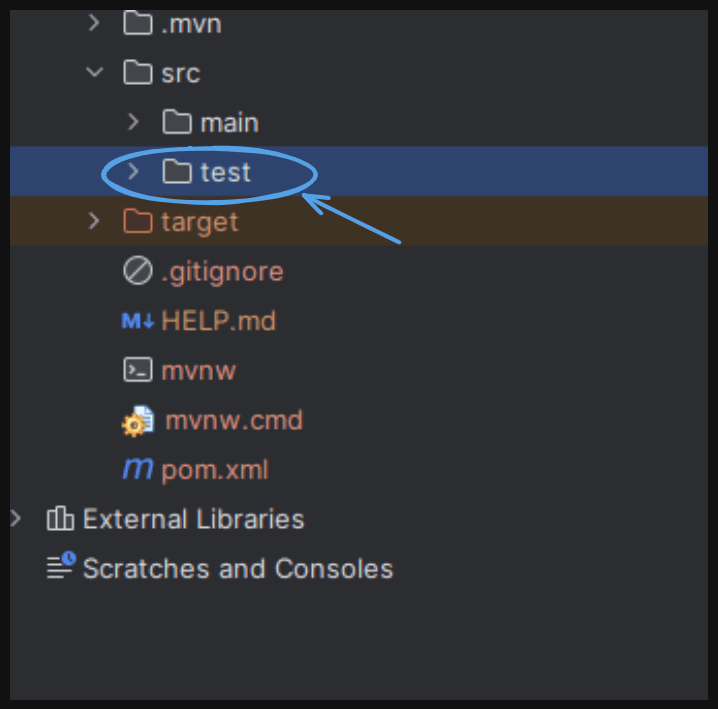
- Go to the ‘test’ package inside the ‘java’ directory, where you will find the test class for the application.
- When you go to the ‘test’ class you can see the
@SpringBootTest
. - In this test class, you can test various functionalities of the application.
SDLC :#
Software Development Life Cycle (SDLC) is a structured process followed for the development of software applications. It consists of a series of phases that guide teams in planning, creating, testing, and deploying software efficiently and with high quality. SDLC models ensure that the software meets business requirements, is delivered on time, and operates correctly and efficiently in production.
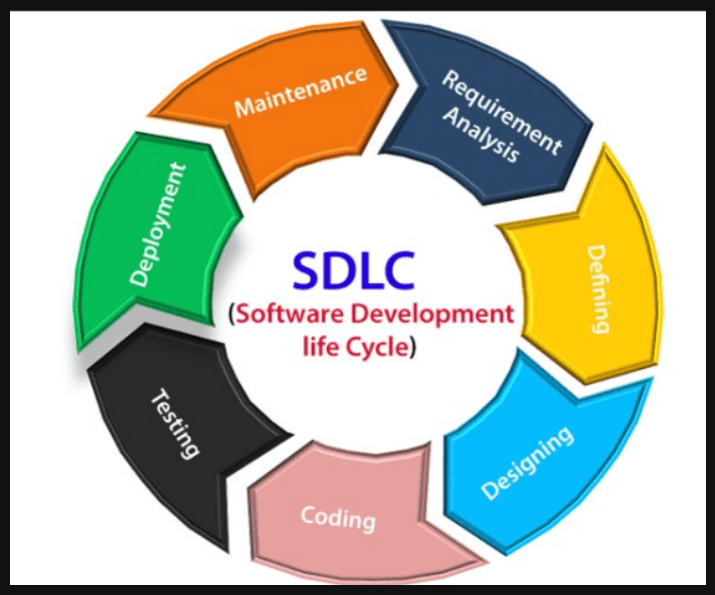
Key Phases of SDLC:#
Requirement Gathering and Analysis
- In this phase, stakeholders and business analysts collect the functional and non-functional requirements of the system.
- The goal is to understand what the software should do and document it thoroughly.
- Outcome: Requirement Specification Document (e.g., Software Requirements Specification - SRS).
System Design
- Based on the requirements gathered, the system architecture and design are created.
- This includes high-level design (HLD) for the overall architecture and low-level design (LLD) for the finer details of each component.
- The goal is to create blueprints for the system that outline how the software will be structured, including databases, UI, APIs, etc.
- Outcome: Design documents (e.g., UML diagrams, system architecture diagrams).
Implementation (Coding)
- This phase involves the actual coding or programming of the software.
- Developers write code according to the system design and specifications.
- Coding standards and guidelines are followed to ensure the quality and maintainability of the code.
- Outcome: Source code and executables.
Testing
- After development, the software is tested to find defects or bugs and ensure the product meets the specified requirements.
- Types of testing include unit testing, integration testing, system testing, user acceptance testing (UAT), etc.
- This phase helps verify the software's correctness, performance, security, and compatibility.
- Outcome: Tested software, defect reports, and test cases.
Deployment
- Once the software has been tested and is free of critical defects, it is deployed to the production environment.
- This can be done in phases, such as alpha, beta releases, and final deployment, depending on the release strategy.
- Deployment may involve setting up hardware, databases, and configurations.
- Outcome: The software is made available to users.
Maintenance
- After the software is deployed, it enters the maintenance phase, where it is monitored for any issues.
- Bugs discovered after release are fixed, and updates or enhancements may be added over time.
- Regular updates are applied for security patches, performance optimization, and feature improvements.
- Outcome: Stable, updated software that continues to meet user needs.
Examples
Imagine you're building a house. The process you follow closely resembles the Software Development Life Cycle (SDLC), where each phase contributes to the final construction of a solid, well-functioning home. Here's an analogy comparing house building to software development:
1. Requirement Gathering and Analysis = Planning the House#
- Before you start building, you need to know what kind of house the owner wants. You sit down with the homeowners (stakeholders) to discuss their needs: How many bedrooms? What style? What’s the budget?
- In software, this is where you gather requirements and understand what the client wants the software to do.
- Once you understand the requirements, an architect creates a detailed blueprint showing the layout of the house, the materials needed, the structure, and utilities like plumbing and electricity.
- In software, this is where system architects and designers create the technical blueprints (system architecture and design) for how the software will function and interact with other systems.
- With the blueprint ready, the builders (developers) start laying the foundation, constructing the walls, installing plumbing, wiring electricity, and adding windows and doors.
- In software, developers write the actual code that makes up the application, based on the design documents created earlier.
- After the construction is complete, inspectors come in to check if everything is working correctly—if the plumbing doesn't leak, the electricity is functional, and the doors and windows open and close properly.
- In software, this is where testers perform tests to ensure the software works as intended, finding and fixing bugs before delivery.
- Once the inspection is passed and everything is fixed, the house is ready. You hand over the keys to the homeowners so they can move in.
- In software, this is the deployment phase where the completed and tested software is released to users for use in a live environment.
- Even after moving in, the homeowners might need repairs (like fixing a leaky faucet) or decide to add an extra room later. You remain available for maintenance and updates as needed.
- In software, this is the maintenance phase, where the development team addresses any issues that arise after deployment, and adds new features or updates over time.
Importance of SDLC
- Structured Workflow: SDLC provides a clear structure and roadmap for building software.
- Efficient Resource Management: Proper planning ensures that resources like time, money, and manpower are used optimally.
- Risk Mitigation: Early identification of risks and issues prevents costly errors later in development.
- Quality Assurance: SDLC includes phases for testing and validation, ensuring that the final product is of high quality.
- Documentation: SDLC promotes thorough documentation at each stage, which aids in communication, future reference, and maintenance.
Importance of Testing#
Identifies Bugs Early and Saves Money:
Catching defects early in the development process prevents costly fixes later on and reduces the chance of major issues in production.
Mitigates Deployment Risks and Ensures System Stability:
Testing ensures the software runs smoothly, reducing the risk of system crashes, failures, and other post-deployment issues.
Increases Productivity and Speeds Up Development:
Automated and frequent testing improves development efficiency by allowing developers to fix issues quickly and focus on creating new features.
Promotes Confidence among Developers and Builds Trust:
A well-tested application instills confidence in the development team, stakeholders, and users, ensuring that the system meets expectations and works as intended.
Test Writing Approaches#
- 1. Test-Driven Development (TDD):
- Analogy: Imagine baking a cake. First, you define what the cake should look and taste like (failing test), then you bake just enough to meet those expectations (minimal code), and finally, you tweak the recipe for better flavor and presentation (refactor).
- Steps:
- Write a failing test (decide on cake requirements),
- Write the minimum code (bake enough to pass the test),
- Refactor (improve the cake design).
- 2. Behavior-Driven Development (BDD):
- Analogy: Planning a family dinner. You first talk with everyone to understand their preferences (define behavior), create a menu with different meal scenarios (write scenarios), and cook to meet those expectations (implement code).
- Steps:
- Define behavior (discuss food preferences),
- Write scenarios (create a menu),
- Implement code (cook meals).
- 3. Test-After Development:
- Analogy: Building furniture without reading the manual first. You assemble it (write code), then check the instructions later to see if you built it correctly (write tests after).
- Steps: Write the code first, then create tests after to verify functionality.
- 4. Simultaneous Development:
- Analogy: Writing a book while editing at the same time. You write a chapter (code) and immediately review/edit it (test) to make sure it fits the storyline.
- Steps: Develop code and write tests together to ensure both are aligned.
Here, we have already covered these aspects, which are highlighted in green in the image.
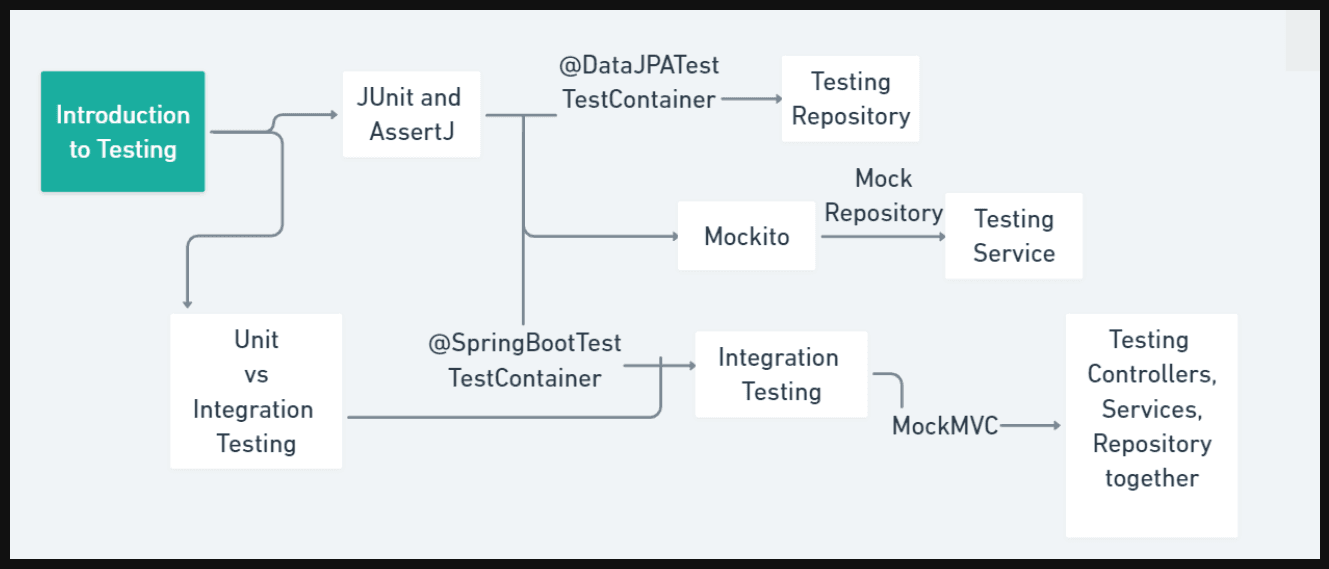
Introduction to Testing in Spring Boot
Conclusion#
This article highlighted the significance of testing in Spring Boot applications, focusing on unit tests, integration tests, and testing strategies like TDD and BDD. It emphasized the integration of testing throughout the Software Development Life Cycle (SDLC) to ensure quality and functionality. Structured testing methods help identify bugs early, reduce risks, and enhance system stability for more reliable software.