Internal Working of Spring Security Basic
Introduction :#
Spring Security is a robust framework that enhances Java EE applications by adding essential security features. It acts as a collection of filters that manage authentication (verifying user identity), authorization (controlling access to resources based on permissions), and protection (safeguarding applications against common security threats). This library ensures that applications are secure, user identities are verified, access is properly controlled, and vulnerabilities are mitigated effectively.
Authentication
Authentication is the process of verifying who you are. In a Spring Boot application, this involves checking your credentials (like a username and password) against the stored data, and if they match, you're granted access to the system, much like being allowed into a mall after showing a valid ID.
Imagine :
You're logging into any of your social media apps. Here's how the authentication process works:
- You open the app and enter your username and password.
- This is like providing your credentials to the application, which the system will use to verify your identity.
- After entering your credentials, you click the "Login" button.
- The app sends your username and password to the server for verification.
- The server checks if the provided credentials match the stored records.
- If the credentials are correct, the server confirms your identity.
- Once verified, you gain access to your account and can start using the app.
- The server grants access, allowing you to interact with different features like viewing your profile or messaging friends.
- If the credentials are incorrect, the server denies access and might show an error message like "Invalid username or password.”
- You remain unauthenticated and are not allowed to proceed into the app.
Authorization
Authorization is the process of determining what you can and cannot do within the app after you've logged in. Even though you're authenticated and allowed into the app, your permissions decide whether you can access certain features or areas, like editing your settings or accessing the admin panel. Authorization ensures that users can only interact with the parts of the application they have the rights to, safeguarding sensitive information and features.
Imagine:
You've successfully logged into your favorite social media app. Now, you want to do different things within the app, like viewing your profile, editing settings, or accessing admin features. Here’s how authorization comes into play:
- You click on the "Profile" button to view your personal information.
- The app checks if you're authorized to view this section.
- Since it’s your profile, you have the necessary permissions, so the app displays your profile details.
- Next, you decide to change your account settings, like updating your email address.
- The app checks if you have permission to edit these settings.
- You have the correct permissions (because it’s your account), so you’re allowed to make the changes.
- Out of curiosity, you try to access the admin panel, which is reserved for administrators.
- The app checks your role and sees that you're not an administrator.
- Since you don’t have the necessary permissions, the app denies access to the admin panel and might display an "Access Denied" message.
- You attempt to view another user's private messages.
- The app checks whether you're authorized to view this sensitive information.
- Since you’re not authorized to access another user's private data, the app blocks this action.
Adding Spring Security :#
To enable Spring Security support, we need to add the spring-boot-starter-security
dependency in our Spring MVC application.
Internal Working of Spring Security:#
In a Spring Boot application, SecurityFilterAutoConfiguration automatically registers the DelegatingFilterProxy filter with the name springSecurityFilterChain. Once the request reaches to DelegatingFilterProxy, Spring delegates the processing to FilterChainProxy bean that utilizes the SecurityFilterChain to execute the list of all filters to be invoked for the current request.
SecurityFilterAutoConfiguration:
Automatically registers the DelegatingFilterProxy
filter under the name springSecurityFilterChain
.
DelegatingFilterProxy:
This filter intercepts incoming HTTP requests and delegates their processing to the FilterChainProxy
.
FilterChainProxy:
The FilterChainProxy
bean manages a list of security filters defined in the SecurityFilterChain
. It determines which filters should be applied to the current request.
SecurityFilterChain:
This component contains the filters that will be executed in sequence for the request, handling various security aspects like authentication, authorization, etc.
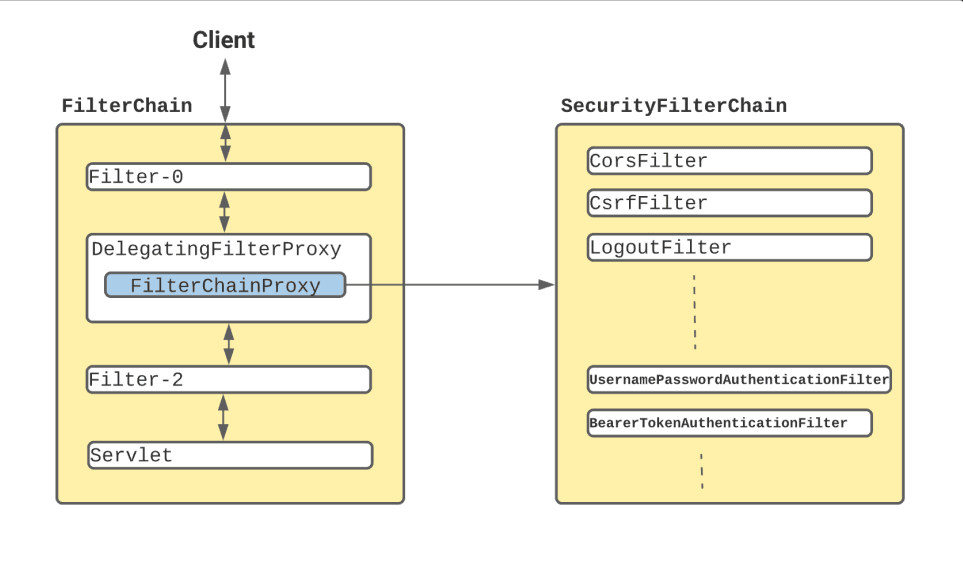
Imagine if This Situation :
Your Spring Boot application is like a mall. The mall has various sections, like stores, a food court, and a VIP lounge. Some areas are open to everyone, while others (like the VIP lounge) require special access.
- In this mall analogy, Spring Security’s
DelegatingFilterProxy
is the main security gate. - This gate ensures that every visitor is routed through the appropriate security checkpoints (filters) managed by the
FilterChainProxy
. - Each checkpoint in the
SecurityFilterChain
ensures that only authorized and authenticated visitors access restricted areas. - If you clear all the necessary checkpoints, you can freely explore the mall, including the restricted VIP areas.
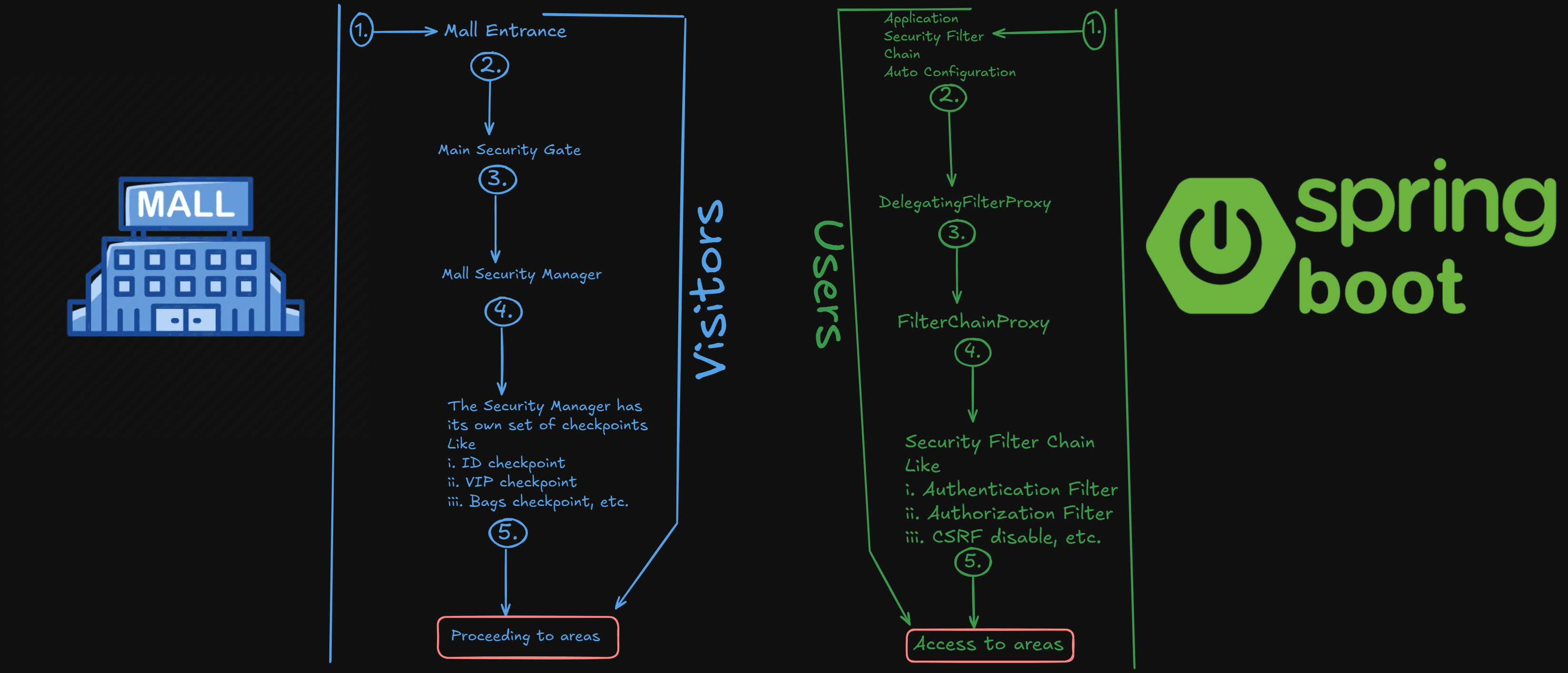
The Default Behavior of Spring Security :#
- Authentication: Spring Security defaults to form-based authentication and provides a basic login page. HTTP Basic Authentication is used for REST APIs.
- In-Memory User: An in-memory user with the username
user
and a generated password is created by default. - Authorization: All requests are secured by default, with access allowed only to login, logout, and error pages unless explicitly configured.
- CSRF Protection: CSRF protection is enabled by default for state-changing requests.
- Session Management: Sessions are managed with session fixation protection and a single session per user by default.
- Password Encoding: Passwords are encoded using
BCryptPasswordEncoder
. - Logout: A default logout endpoint is provided, which invalidates the session and redirects to the login page.
- Access Denied Handling: Unauthorized access attempts result in a 403 error or redirect to an access denied page.
Explanations of the Default Behavior of Spring Security:#
- Go to Chrome and hit **https://start.spring.io/**
- Create a maven application with validate dependencies (most important Spring security
spring-boot-starter-security
)
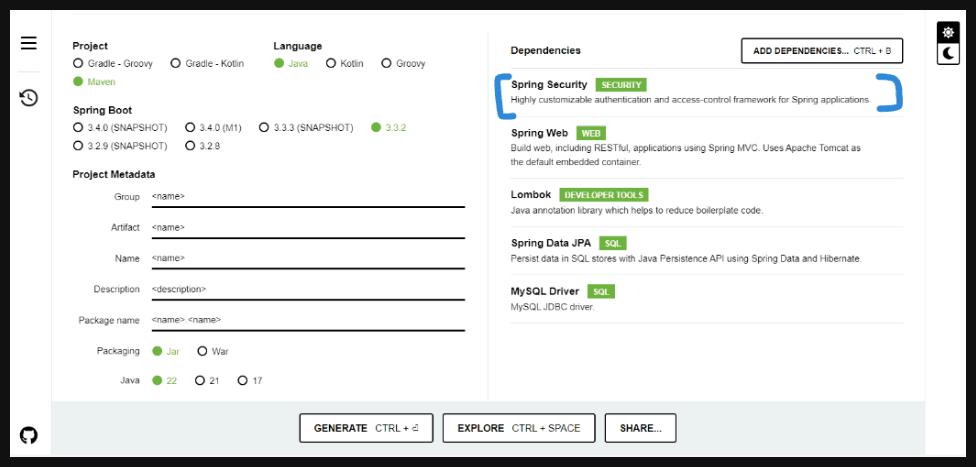
- If you run your application, in your run console you can see this application generates a default password and the username by default is ‘user’.

- If you have any controller and also have a ‘get’ request endpoint http://localhost:8080/<endpoint>) try to send a request in Chrome you can see there is a sign-in form generated and if you put ‘user’ as username and password then after hitting the “sign in” button
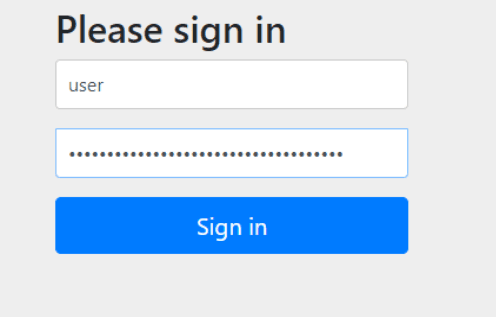
- you can see that an empty list.
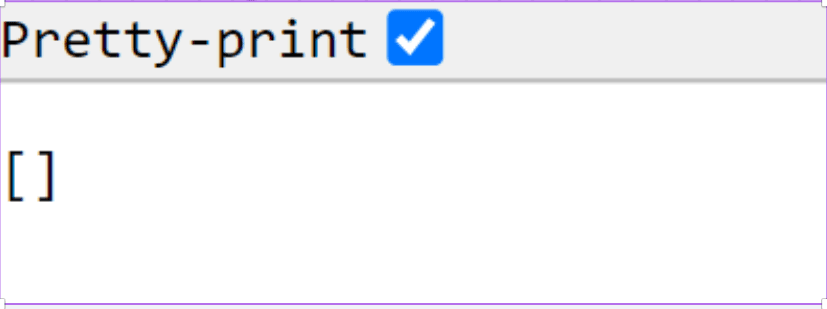
- If you put in the wrong username or password you will get a “Bad Credentials” error in your browser.
- The password and user are no valid longer because security has maintained a session for us all that is happening by default. If you want to look at the session just right-click on your browser and go to inspect > Application > Cookies. Inside your cookies, you will see one cookie has been generated for us with “JSESSIONID”. Until you log out this session ID will be there and this will be validated. Once you log out then the session id has been invalidated in the backend. This session ID is expired. If you go to inspect > Elements and inside the input field of the form field you can see the input name is “csrf” and the type is “hidden” and also see the “csrf token”. This token is passed by the server when the sign-in page is loaded. It was generated at the backend only.
- For logging out if you hit the “/logout” endpoint we will see a page “Are you sure you want to log out” and after hitting the “log out” button we will again go to the “sign in” page. The log-out process is here.
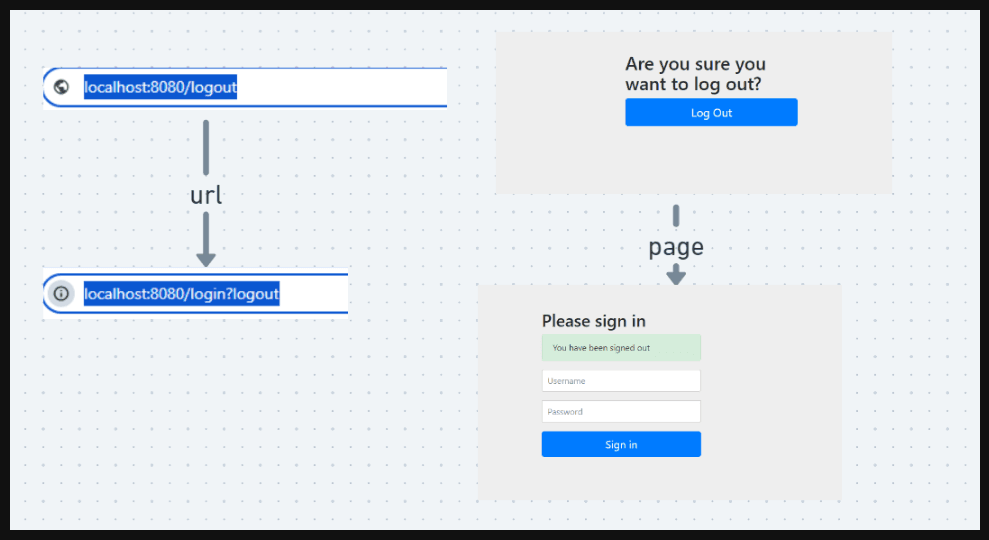
Instead of using the default username and password, you can also create your username and password in your “application.properties”.
Conclusion#
This article explains how Spring Security manages authentication and authorization in Spring Boot applications using filters like DelegatingFilterProxy
and FilterChainProxy
. It covers the default security behavior, including form-based authentication and session management. Developers can customize settings to enhance security while ensuring proper access control.