Declaring Pointcuts in AOP
Introduction:#
A pointcut in Spring Boot AOP (Aspect-Oriented Programming) is a predicate expression that identifies specific join points, or points during the execution of a program, where an aspect's advice (cross-cutting concerns like logging, security, etc.) should be applied. It allows developers to define conditions based on method signatures, annotations, parameters, or class types, enabling the separation of cross-cutting concerns such as logging, validation, and transaction management from the core business logic. Pointcuts act as a filter to target specific methods or actions within an application, allowing for flexible and reusable application of advice (such as @Before
, @After
, or @Around
).
Imagine you’re a director working on a movie. The movie has multiple scenes, but you want to add some special effects or commentary at certain moments. Instead of applying these special effects to the entire movie, you define the pointcuts — these are the specific scenes (or moments) where the effects or commentary will be applied.
The pointcut acts like the selection of specific scenes based on certain criteria. For example, you might choose to add effects only to action scenes or commentary only to emotional moments.
The advice is the special effect or commentary that gets applied when the pointcut matches. So, when the action scene starts, your special effects kick in; when the emotional moment happens, the commentary begins.
Dependency:#
When you use spring-boot-starter-data-jpa
in a Spring Boot project, it includes Spring AOP as a transitive dependency. This means you don't need to explicitly add spring-boot-starter-aop
to your dependencies if you're already using JPA.
However, the ability to "Ctrl + click" or navigate to see dependencies depends on your IDE or build tool configuration.
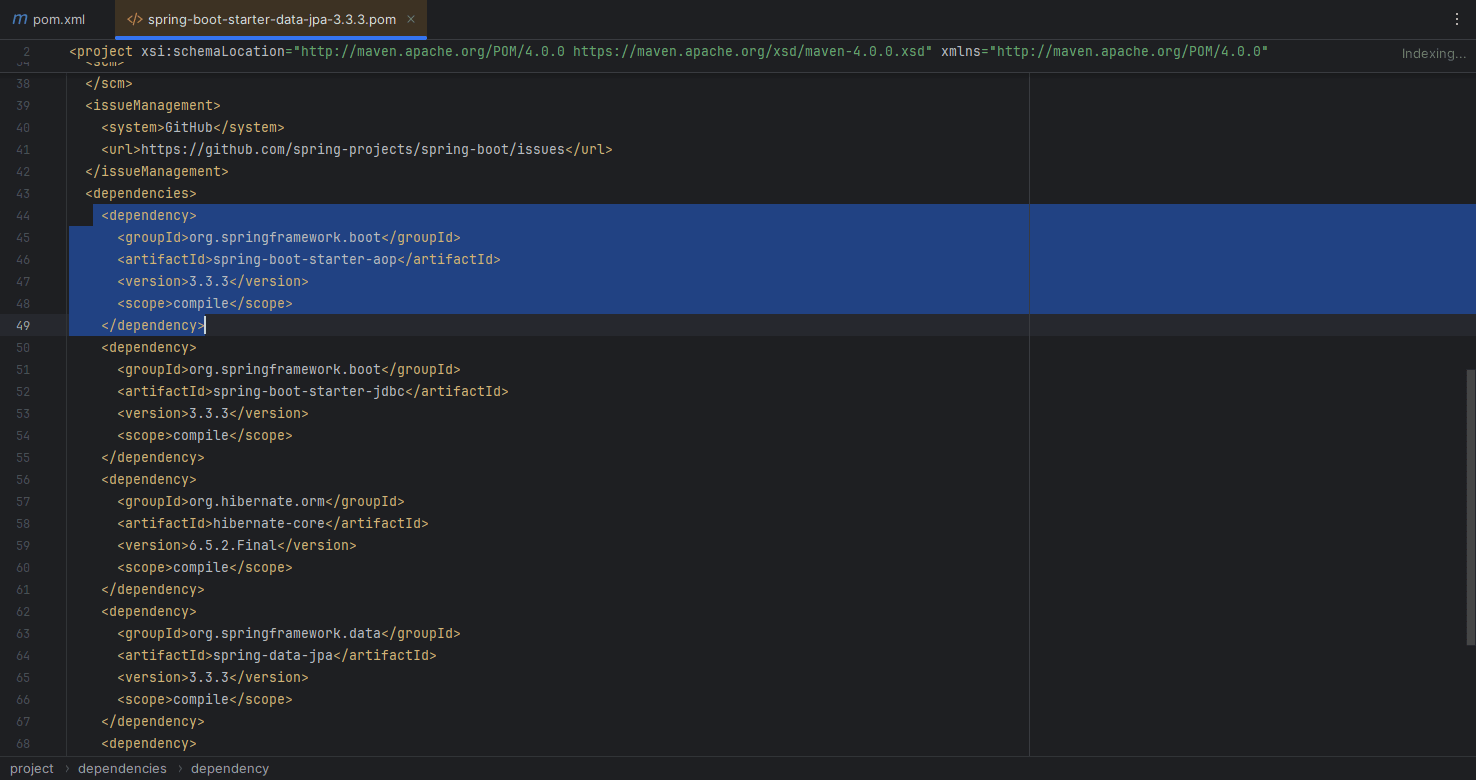
However, if your project does not include JPA, you must explicitly add the Spring AOP dependency:
Using pointcuts in Aspect-Oriented Programming (AOP) allows you to modularize cross-cutting concerns by targeting specific methods, classes, or behaviors. This modularization improves code readability, maintainability, and reusability.
Custom Classes Code For Pointcuts Test:#
Service Class Code From Custom Service Package:
Test Class Code For Testing Purpose:
Different kinds of pointcuts in Spring AOP with examples and explanations:#
1. Execution Kind Pointcut#
This is the most widely used pointcut expression. It targets method executions based on a specified pattern, such as the method signature, return type, method name, and parameter types.
Match All Methods in a Package
To match all methods in the com.example.service
package:
Match Specific Method#
To match the method findById
in com.example.service.UserService
:
Match All Public Methods#
To match all public methods in the application:
Pointcut Syntax Explanation#
General Syntax:#
Simplified Syntax:#
CODE:#
Logging Aspect Class Code From Custom Aspect Package:
JoinPoint: A point in the execution of a program where an aspect can be applied (e.g., method execution).
- Specific method with specific package and class.
- Any method of specific package with specific class.
- Try to find out orderPackage() method if it is present inside all classes of all impl packages.
- Any method of any class.
Output:#
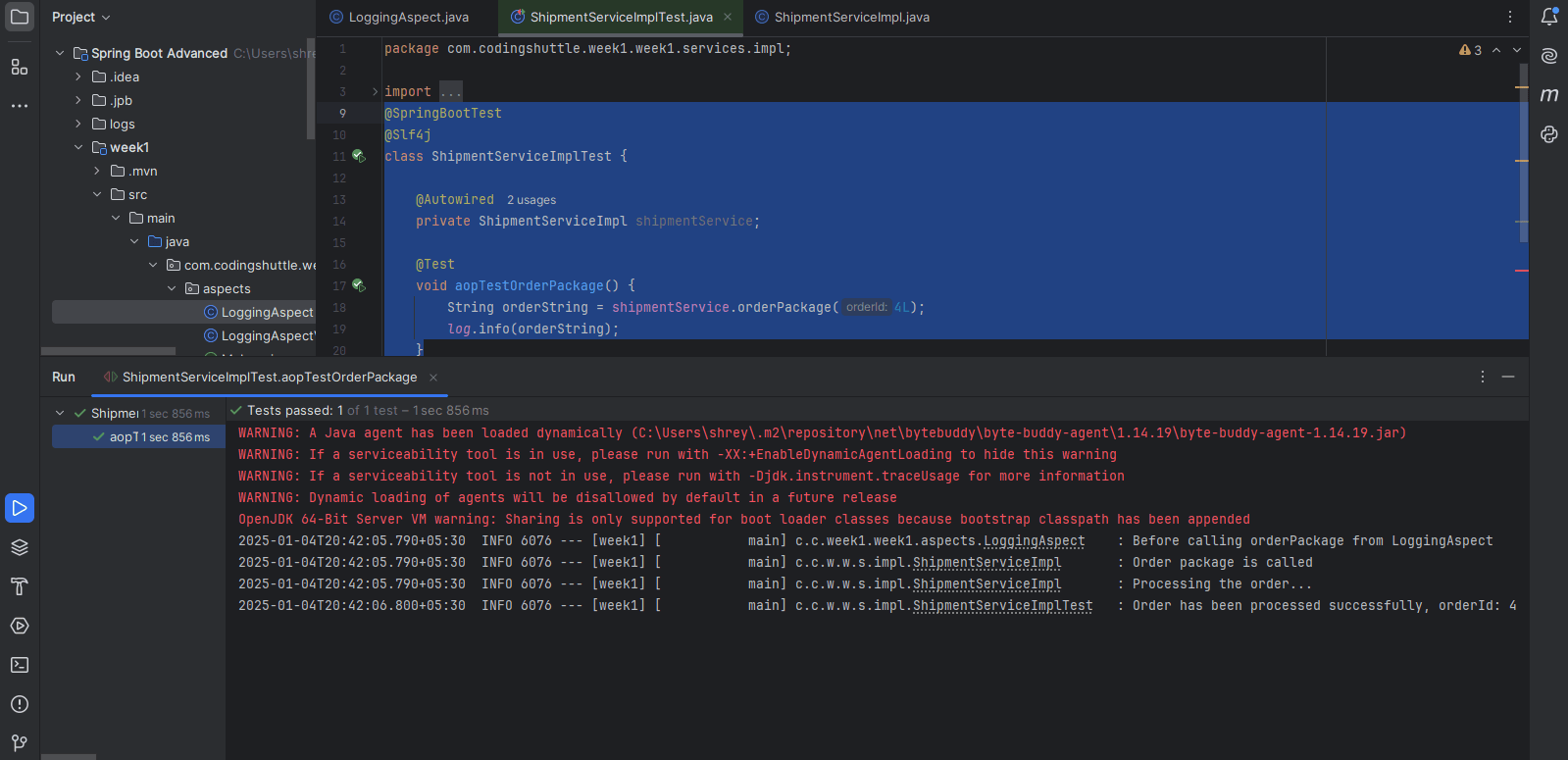
Explanation:#
execution
: Specifies that the pointcut targets method executions.return-type-pattern
: Defines the return type of the method ( matches any type).package.class.method-name
: Specifies the fully qualified name of the method ( matches any class or method name).param-pattern
: Matches method parameters. Use(..)
,(*)
, or specific parameter types.
2. Within Kind Pointcut#
The within()
pointcut allows you to limit the advice to a particular class or package, without focusing on specific methods. It applies to all join points (methods, fields, constructors) within the target class or package.
Syntax:#
- Class-level: Apply to all methods of a specific class.
- Package-level: Apply to all methods within classes in a package.
Apply Advice to All Methods in a Class:#
Apply Advice to All Methods in a Package:#
Applying Advice to All Methods in Subclasses:#
Applying Advice to All Classes and Subclasses:#
Explanation:#
within
: Specifies the target class or package where the advice should apply. It is often used to apply advice to all methods in a particular class or package without needing to define specific method signatures.- Scope: It applies advice to all join points (methods, constructors, fields) within the specified class or package.
Code:#
Logging Aspect Class Code From Custom Aspect Package:
Output:#
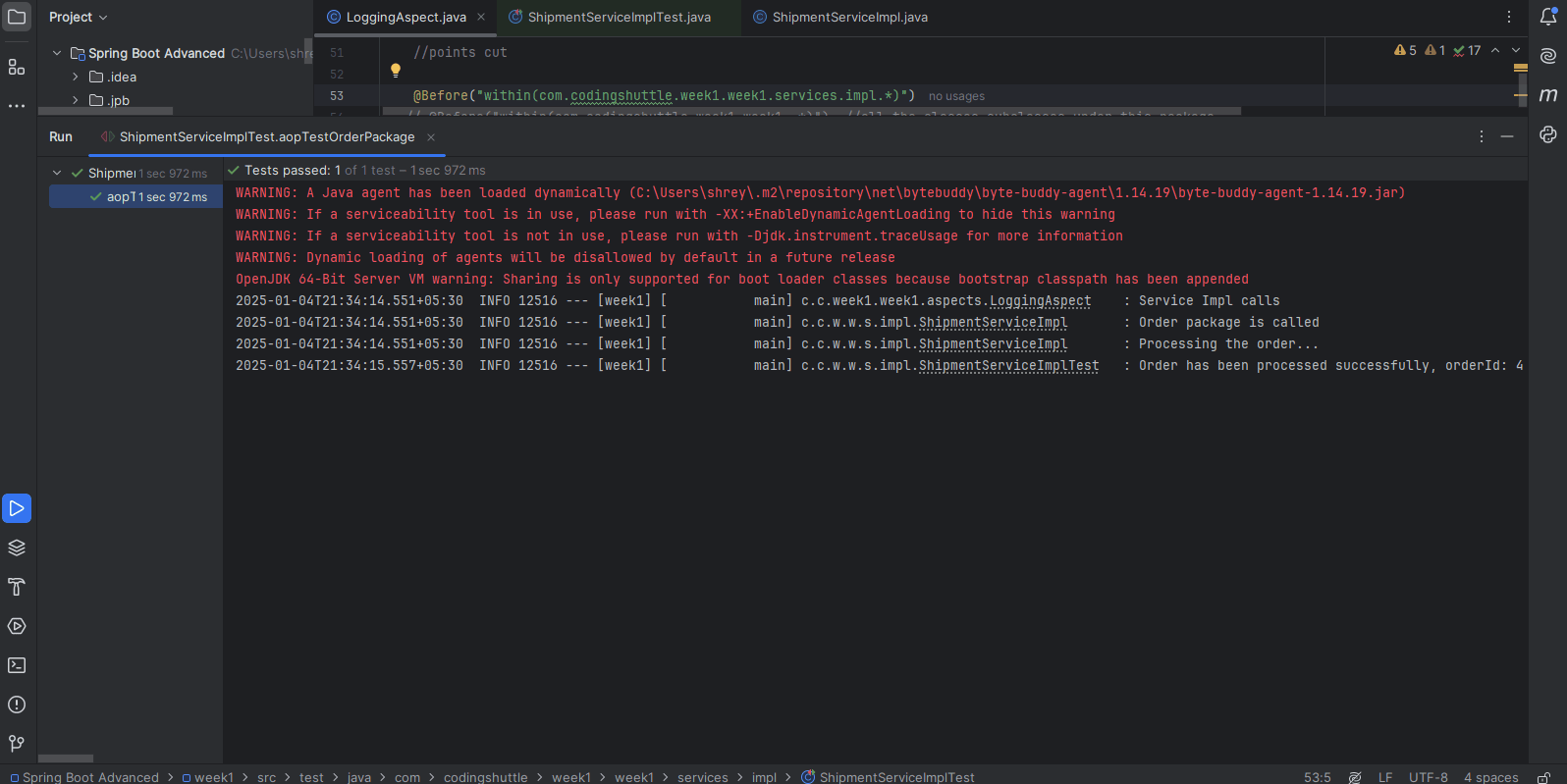
Explanation:#
- within: Specifies the target class or package.
- This will apply advice to all methods within any class inside the specified package.
3. Annotation Kind Pointcut#
Use @annotation()
to apply advice to methods annotated with a particular annotation. This is useful when you want to apply advice only to methods that carry a specific annotation, whether built-in or custom.
Syntax:#
Applying Advice to Methods with a Custom Annotation:#
- Custom Annotation:
- Aspect to Apply Advice:
- Using the Annotation:
- Output:
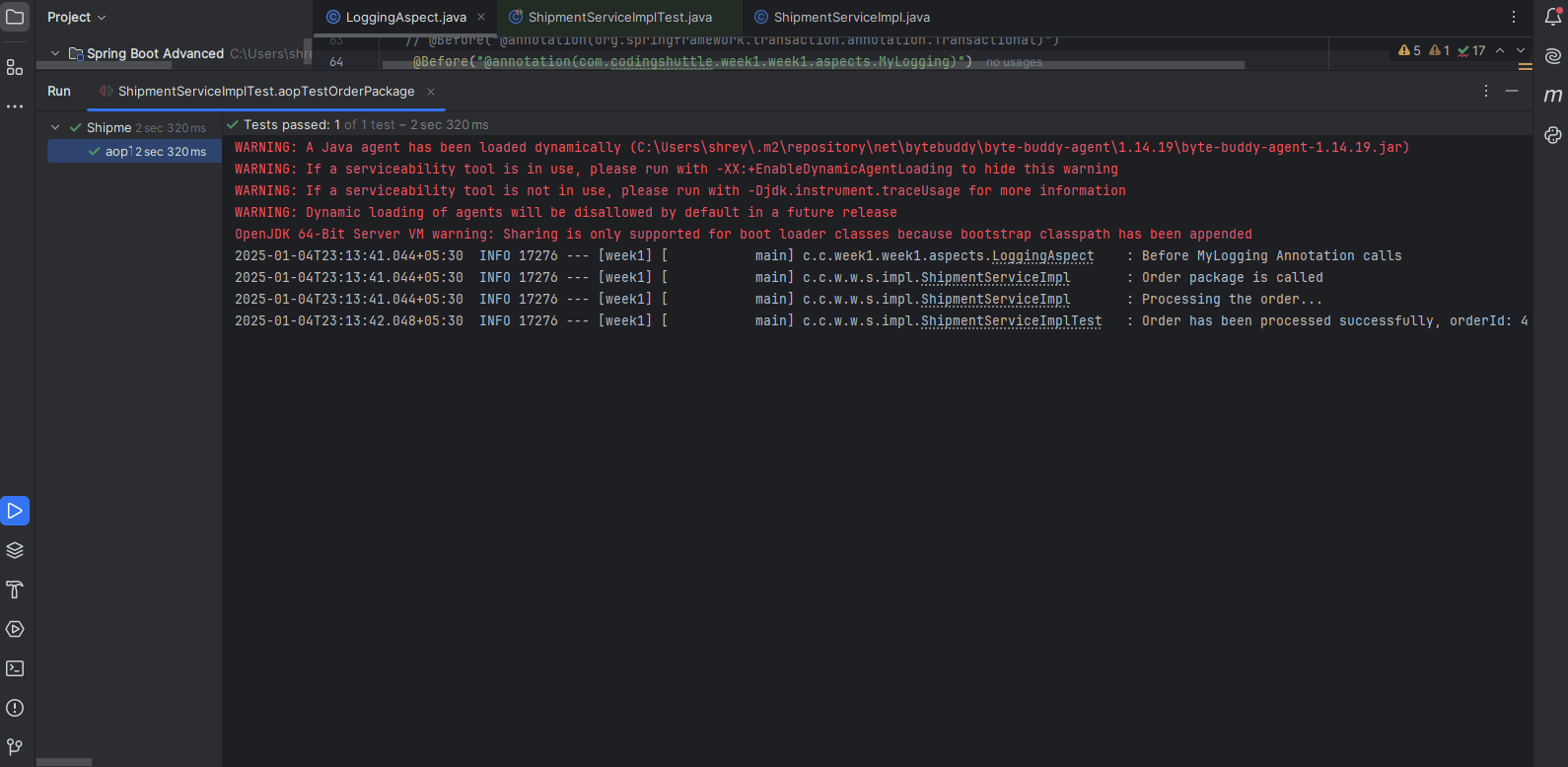
Applying Advice to Methods with Built-in Annotations#
- Using the Transactional Annotation:
- Aspect to Apply Advice:
- Output:
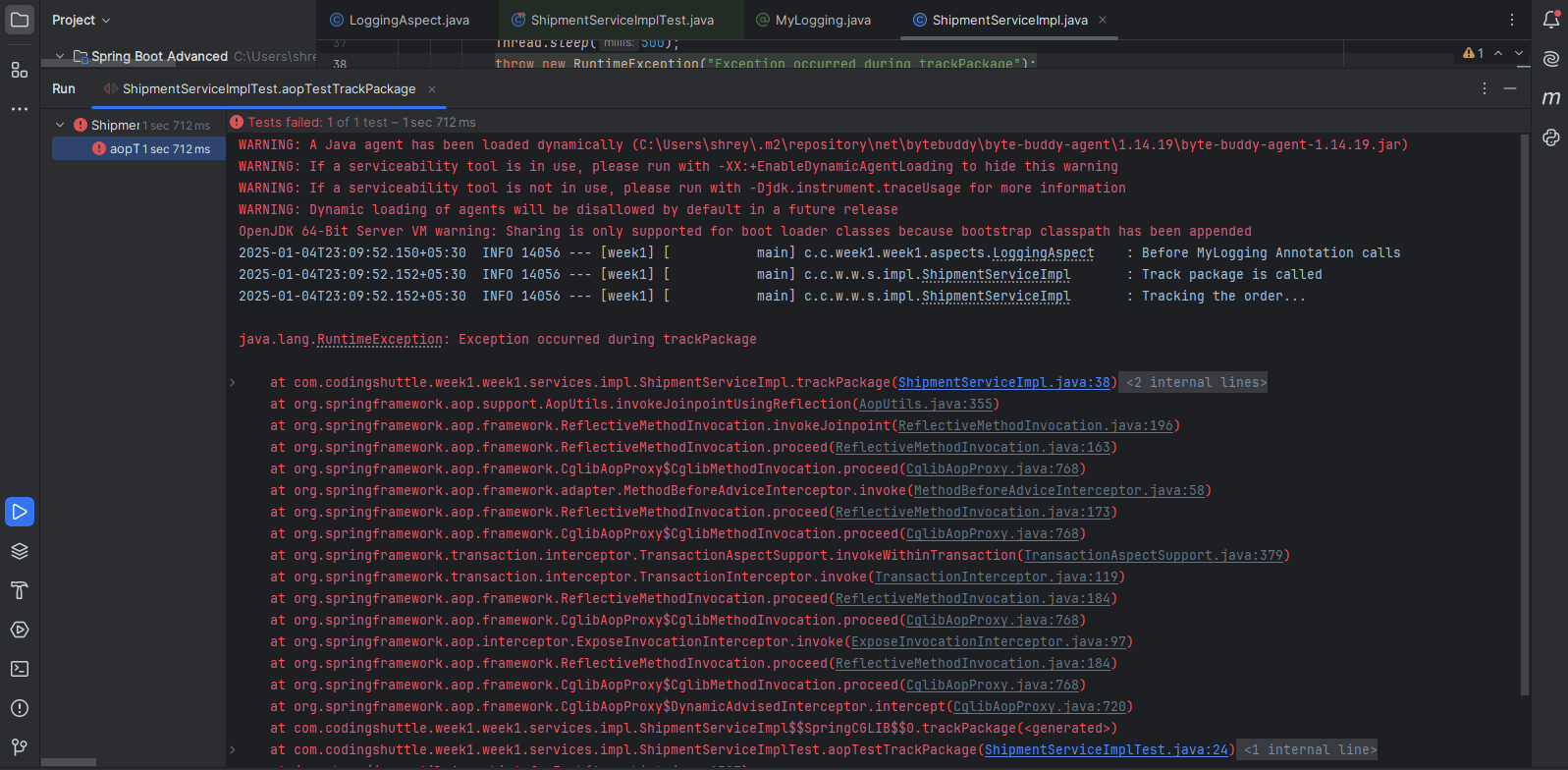
Explanation:#
@annotation
: Specifies that the advice should be applied to methods that are annotated with a given annotation.- The pointcut expression matches methods that carry a specific annotation, such as
@MyLogging
,@Transactional
, or any custom annotations you define.
4. Pointcut Declaration#
A pointcut can be declared separately using the @Pointcut
annotation and then referenced in the advice annotations like @Before
, @After
, @Around
, etc.
Syntax:#
Reusable Pointcut for a Package#
- Declaring the Pointcut:
- Sing the Pointcut in Advice:
Combining Pointcuts:#
You can combine pointcuts using logical operators (&&
, ||
, !
).
- Declare Multiple Pointcuts:
- Combine Pointcuts:
- Apply Combined Pointcut:
By defining pointcuts separately and referencing them in the corresponding advice, Spring AOP allows you to modularly apply cross-cutting concerns such as logging, transaction management, and security.
Key Notes
- The
(..)
pattern in the pointcut allows matching methods with any number or type of arguments.- The
*
wildcard can be used to match any return type, class name, or method name.- Combining multiple pointcuts using
&&
,||
, or!
provides more flexibility.
Conclusion#
This article provides an overview of pointcuts in Aspect-Oriented Programming (AOP) within Spring Boot, highlighting their role in separating cross-cutting concerns like logging, security, and transactions. It explains different types of pointcuts (execution, within, annotation, and reusable) and their syntax. Using pointcuts improves application modularity, scalability, and maintainability by decoupling concerns from core business logic.