Data Binding and Validation in Spring Boot: Using @ModelAttribute, @Valid, and Validation Annotations
In modern web applications, handling user input effectively is crucial for a smooth user experience and data integrity. Spring Boot offers powerful tools for managing data binding and validation through annotations like @ModelAttribute
, @Valid
, and validation annotations. This article will introduce these concepts in a beginner-friendly manner, providing a solid foundation for their application in real-world scenarios.
What is Data Binding?#
Data binding is the process of transferring data between a user interface and the server-side application. In the context of web applications, this means mapping form data from an HTML form to a Java object on the server. Spring Boot simplifies this process through the use of @ModelAttribute
.
Understanding @ModelAttribute
#
The @ModelAttribute
annotation in Spring Boot is used to bind form data to a Java object. This allows you to automatically populate a model object with data from an HTML form submission. Here’s how it works:
Define a Model Class: Create a Java class representing the form data.#
Add Validation Constraints: Use validation annotations from the javax.validation.constraints
package in your model class.
Validation Annotations Explained#
@NotBlank
: Ensures that the field is not empty or null. Useful for strings.@Email
: Validates that the string is a valid email format.@Size
: Validates the length of the field. E.g.,@Size(min = 2, max = 30)
.@Min
: Validates Integer number has to be aleast specified value. and not less than it.
Create a Controller Method: Use @ModelAttribute
to bind form data to the model class.#
Design a Form View: Create an HTML form that binds to the User
object.#
Modify your form view to display validation errors: In this example, Thymeleaf (a popular template engine) is used to display error messages. The th:if
and th:errors
attributes check for and display validation errors associated with each field.
Create result.html to show registered users details#
Run the application and hit the endpoint on browser#
Enter invalid values and in field you will see the errors.
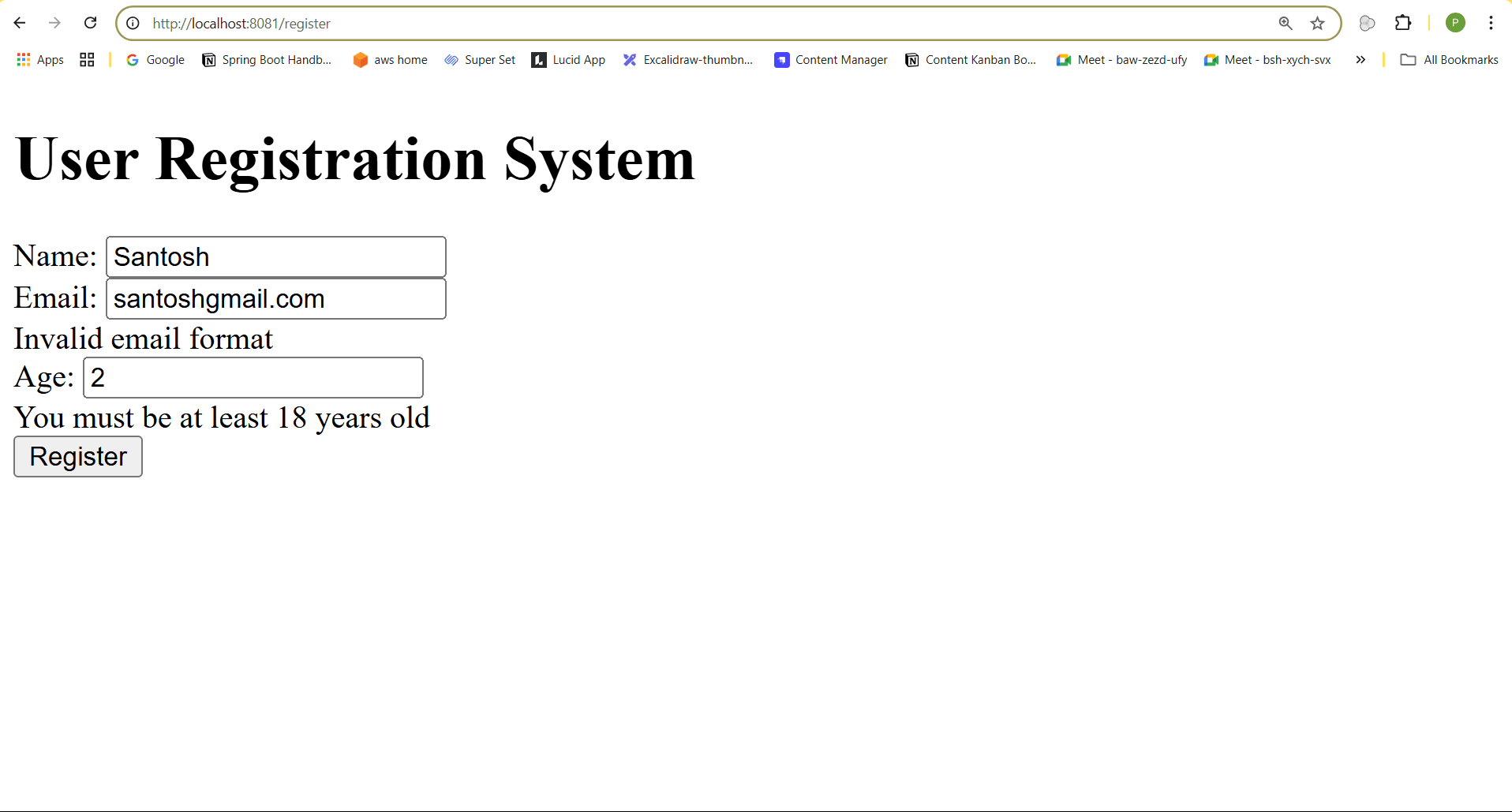
After Entering the valid values in field you will be redirected to result page .
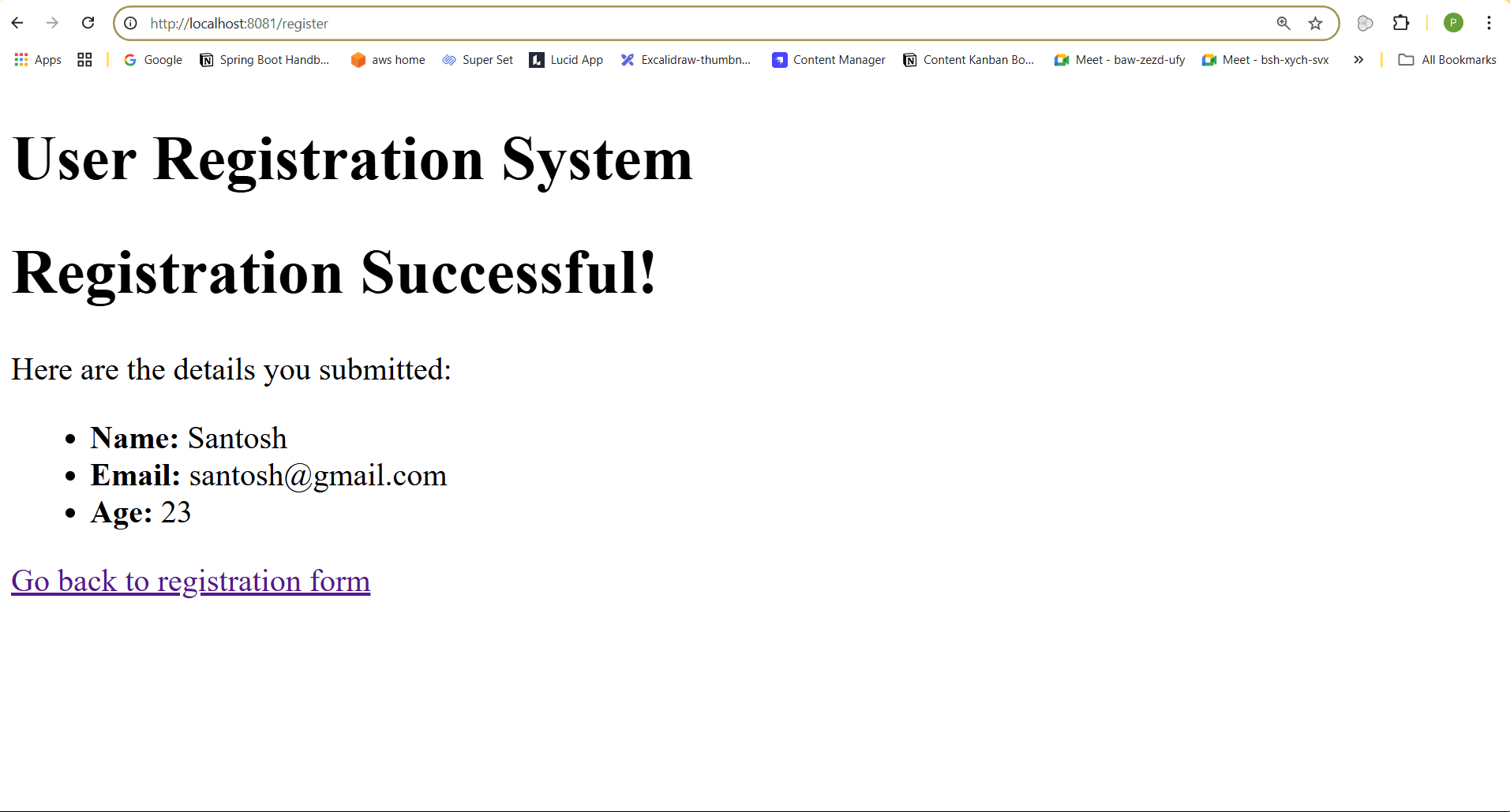
In this article, we explored the crucial concepts of data binding and validation in Spring Boot using annotations like @ModelAttribute
, @Valid
, and various validation annotations. We demonstrated how to bind form data to a Java object seamlessly and validate user input effectively to ensure data integrity and a smooth user experience. With practical examples and a step-by-step approach, you now have a solid foundation to implement these techniques in your own web applications.