Configuring SecurityFilterChain
Introduction :#
To configure a SecurityFilterChain
in a Spring Security-based application, you'll typically use the SecurityFilterChain
interface to define how security should be applied to HTTP requests.
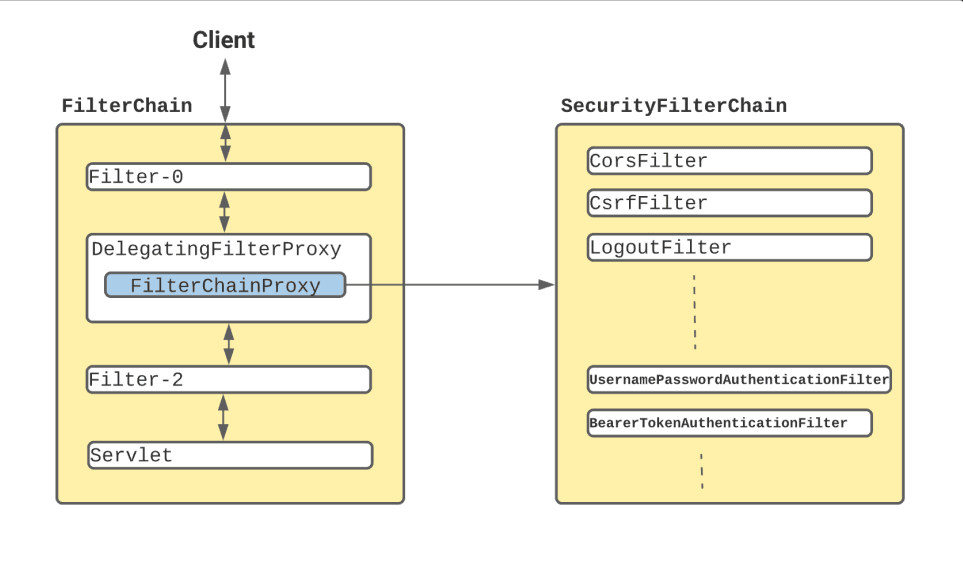
SecurityFilterChain
Here's how you can set up and customize a SecurityFilterChain
in your Spring Boot application:
- Add Dependencies
Make sure you have the necessary dependencies in yourpom.xml
file. For Spring Boot, this usually includes:
- Create a Security Configuration Class
You can define aSecurityFilterChain
in a configuration class. Here’s an example:
- Explanation of Key Components
- HttpSecurity Configuration: The
HttpSecurity
object is used to customize security settings. In the example, CSRF is disabled (not recommended for production) and HTTP requests are configured so that any request matching/<any endpoint>/**
is permitted without authentication, while all other requests require authentication. - Form Login: Form-based login is enabled with a custom login page (
/login
). - Logout: A custom logout URL (
/logout
) is provided. - UserDetailsService: This example uses an in-memory user store for simplicity, but in a real application, you would likely connect to a database or another user management system.
- HttpSecurity Configuration: The
- Running the Application
When you run the application, any request to paths like/<any end point>/xyz
will be accessible without logging in, while other paths will require authentication. The login form will be available at/login
.
- Customization
- Custom Authentication Providers: If you need custom authentication logic, you can create and configure an
AuthenticationProvider
bean. If you were developing a simple REST API where you wanted to protect certain endpoints and only allow access to users with specific roles, but you didn't want to set up a database just for this, you might use in-memory authentication as shown above. - Password Encoding: Use a proper
PasswordEncoder
for production environments. - CSRF Protection: For most applications, you should enable and properly configure CSRF protection.
- Custom Authentication Providers: If you need custom authentication logic, you can create and configure an
Default SecurityFilterChain Config:#
- authorizeRequests() restricts access based on RequestMatcher implementations.
- authenticated() requires that all endpoints called be authenticated before proceeding in the filter chain.
- formLogin() calls the default FormLoginConfigurer class that loads the login page to authenticate via username-password and accordingly redirects to corresponding failure or success handlers.
- csrf() to cofigure the csrf protection.
- sessionManagement() to configure session management for your application.
Conclusion#
This article explains how to configure SecurityFilterChain
in a Spring Boot application using Spring Security. It covers setting up authentication, authorization, session management, and password encoding. It also highlights key best practices like enabling CSRF protection and using password encoders for production.