Authenticating requests using JWT Part 1
Introduction :#
JWT (JSON Web Token) is a popular method for securely transmitting information between parties as a JSON object. It is commonly used for authenticating and authorizing requests in web applications. Here's an overview of how JWT authentication works:
How JWT Authentication Works:#
- User SignUp:
- User Login:
- Token Generation:
- Token Structure:
- Client-Side Storage:
- Authenticated Requests:
- Token Validation:
- Token Expiration:
- Security Considerations:
Example Workflow#
- User SignUp:
- POST /signUp
- POST /signUp
- User Login:
- POST /login
- Response: JWT
- Access Protected Resource:
- GET /user/profile
- Header:
Authorization: Bearer <JWT>
- Server Validates JWT:
- If valid, the server processes the request and returns the requested resource.
- If invalid, the server responds with a
401 Unauthorized
error.
Using JWT For Authentication:#
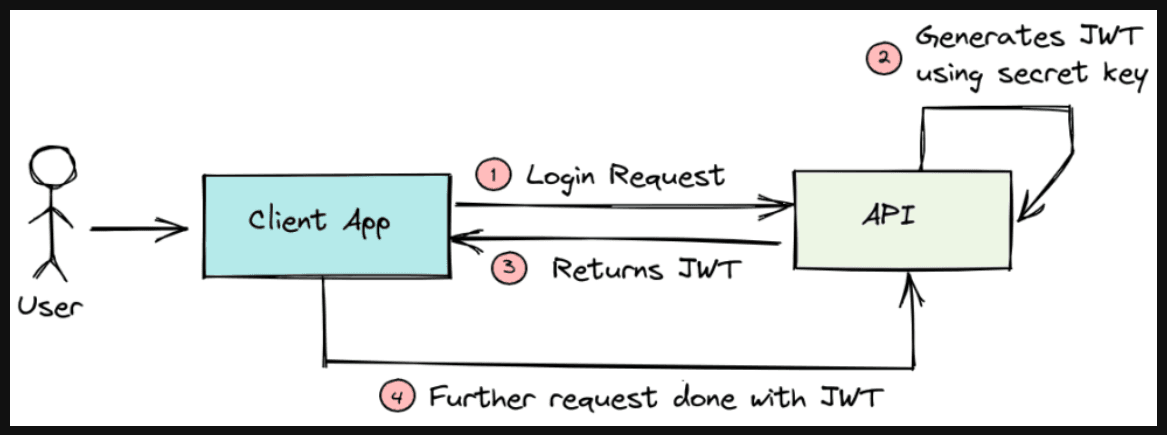
Login Workflow with JWT:#
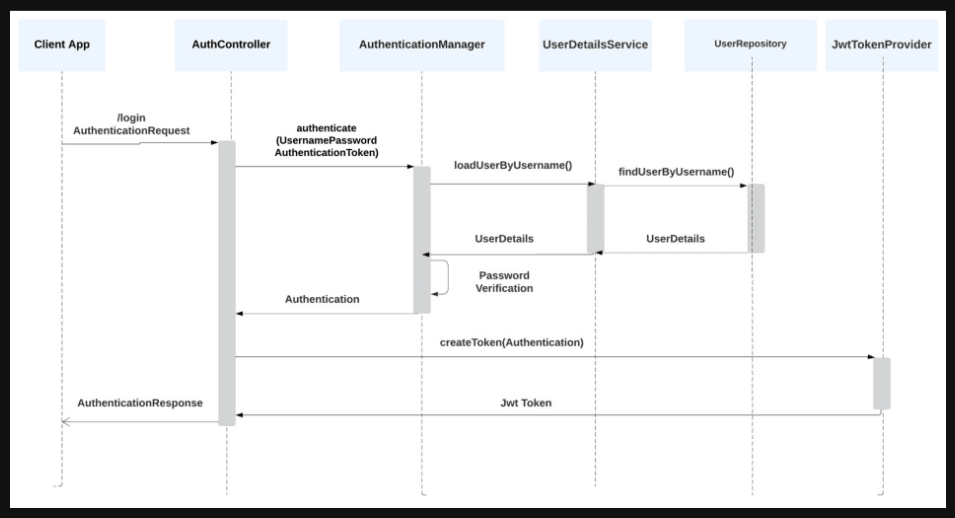
Authentication Workflow with JWT:#
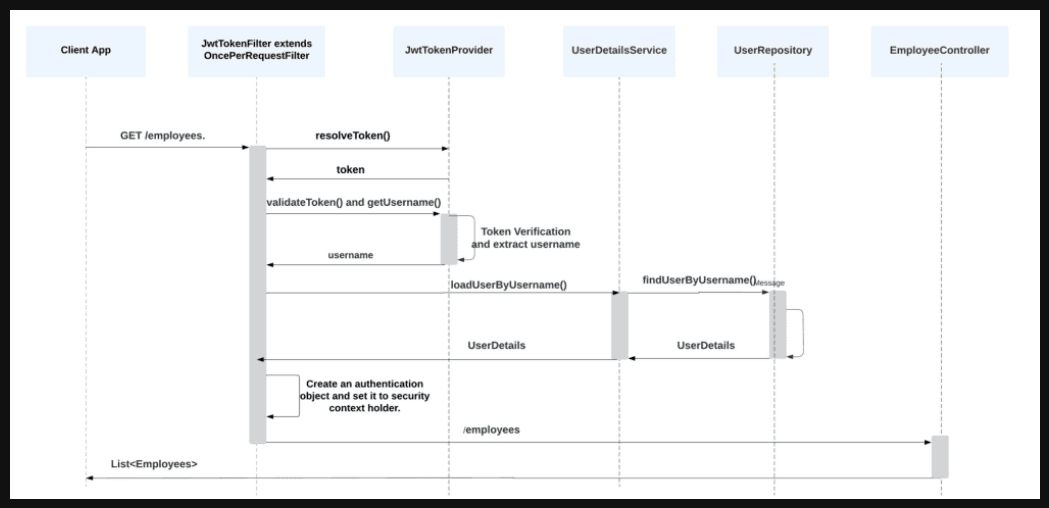
JWTAuthFilter Control Flow:#
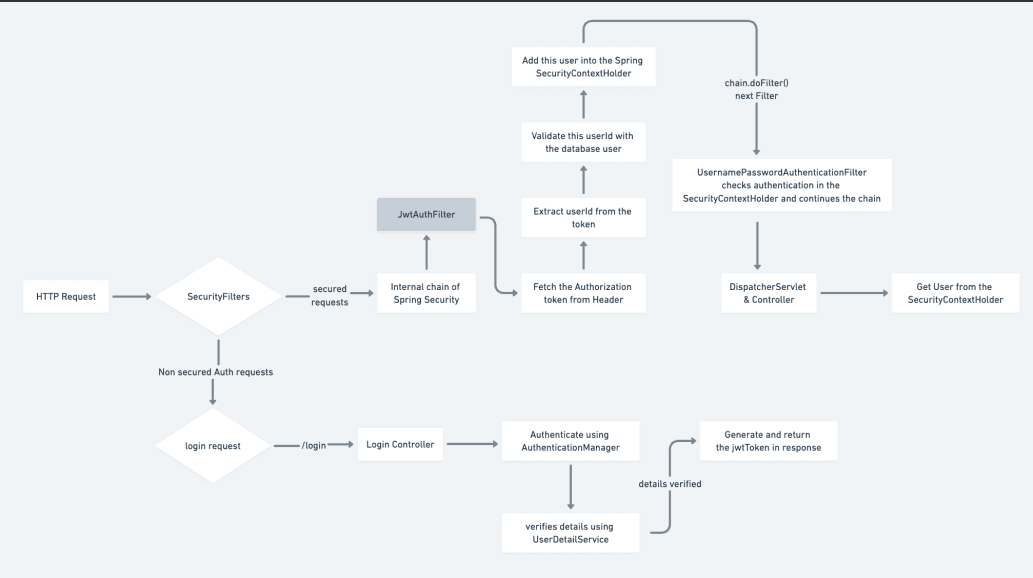
Implementation in Code:#
- How to authenticate future requests that come into the server
- How to authenticate all the requests that contain a token
- The provided token is valid or not
- If it is valid then how to pass the authentication object inside the spring security context holder so that it is available to all the controllers.
let's break down each step:
- Authenticate Future Requests with JWT
When a user logs in, they receive a JWT. This token is included in the Authorization
header of every subsequent request. The server must then authenticate each request using this JWT.
- Authenticate Requests that Contain a Token
You need to intercept every request and check if it has a valid JWT token. If it does, the server will authenticate the user based on the token.
- Check if the Token is Valid
The server will validate the JWT token by checking its signature, expiration date, and possibly other claims.
- Pass the Authentication Object to Spring Security Context Holder
If the token is valid, you'll create an Authentication
object and store it in the SecurityContextHolder
, which makes the authenticated user available throughout the application (e.g., in controllers).
Conclusion#
This article explains how to authenticate requests using JWT in web applications, including user signup, login, token generation, and validation. It highlights the process of securely storing JWT on the client side and authenticating requests through Spring Security. Implementing these steps ensures secure access to protected resources.