Auditing and Advance Auditing with Hibernate Evers
Introduction#
Auditing in Spring Boot is a feature that helps track and manage the changes made to your entities, providing insights into who made changes and when. It can automatically populate fields like:
- Creation Timestamp: The date and time when an entity was created.
- Last Modified Timestamp: The date and time when an entity was last updated.
- Created By: The user who created the entity.
- Last Modified By: The user who last modified the entity.
To enable auditing, you typically use the @EnableJpaAuditing
annotation in your configuration class. You also define an AuditorAware
implementation to retrieve the current user, which can be based on the security context or other mechanisms.
A real-life analogy for auditing in an application is a security camera system in a building.
Here’s how it relates:
- Tracking Actions: Just as a security camera records who enters or leaves a building and what activities occur, auditing records who created, updated, or deleted data in your system.
- Time Stamping: Security cameras store the exact time and date of every event, similar to how auditing automatically logs timestamps for when a record was created or modified.
- Accountability: If something goes wrong or is suspicious (like a break-in), you can review the footage to see who was responsible. Similarly, in an application, auditing allows you to see who made changes to data, helping ensure accountability.
- Monitoring and Reviewing: In both cases, whether it's security footage or audit logs, you can go back and review the logs when needed for investigations or compliance.
Just like a building’s security system helps protect and keep track of activity within a building, auditing helps safeguard data and maintain a detailed record of changes within an application.
Benefit of Using Auditing in an Application#
The primary benefit of using auditing in an application is enhanced data tracking and accountability. Here are some key advantages:
- Change History: Auditing allows you to maintain a record of changes made to data, including who made the change and when it occurred. This is crucial for understanding the lifecycle of data and can help with troubleshooting.
- Regulatory Compliance: Many industries have regulations that require tracking changes to sensitive data. Auditing can help meet these compliance requirements.
- Accountability: By recording who performed actions on data, auditing promotes accountability within the organization. This can deter unauthorized changes and help identify issues quickly.
- Data Integrity: Auditing can assist in maintaining the integrity of the data by providing a way to trace back changes, allowing for data recovery or correction if necessary.
- User Activity Monitoring: It provides insights into user behavior and patterns, which can be valuable for security audits and improving user experience.
- Reporting and Analysis: Auditing data can be used for reporting purposes, enabling analysis of how often certain data is accessed or modified.
Overall, auditing enhances the reliability and trustworthiness of your application by providing a transparent view of data changes and user interactions.
Steps to Add Auditing#
Enable JPA Auditing:
Add the @EnableJpaAuditing
annotation to a configuration class to activate the auditing feature.
Create an Auditable Base Entity:
Create a base entity class that will include the auditing fields and annotations. Use @EntityListeners(AuditingEntityListener.class)
to enable auditing.
This way you will get access to @CreatedBy, @CreatedDate, @LastModifiedBy, and @LastModifiedDate annotations.
Here we basically make the ProductEntity as a Audit table. And we add the
@EntityListeners(AuditingEntityListener.class)
.
Create Base Entity Class
In scenarios where we have many entity classes in our application, it is not efficient or maintainable to manually apply auditing annotations to each entity class. Instead, we can create a base auditable entity class that contains common audit fields like createdDate
, lastModifiedDate
, createdBy
, and lastModifiedBy
. Then, we can make all other entities inherit from this base class.
Extend All Entities from the Superclass:
Ensure that all your entities extend this base Auditable
class. After creating the Auditable base entity superclass (AuditableEntity), you need to extend all entities that require auditing from this superclass. This ensures that the common audit fields like createdDate
, lastModifiedDate
, createdBy
, and lastModifiedBy
are automatically included in the entities and managed by Spring Data JPA’s auditing mechanism.
Here we use one entity class that is ‘ProductEnity’ which extend the super class.
Implement the AuditorAware Interface:
Create a class that implements the AuditorAware
interface to retrieve the current authenticated user from Spring Security.
Here, we use a different package ‘auths’ to create the class (AuditorAwareImpl
) that implements the AuditorAware
interface.
Pass the AuditorAware Bean Reference:
The @EnableJpaAuditing
annotation automatically detects the AuditorAware
bean you created, so you don’t need to pass it explicitly. Just ensure that your AuditorAware
implementation is annotated with @Component
.
Here, we use configuration class to create AuditorAware
bean.
Code Implementation of Update Information#
Auditing for updating information involves tracking changes made to an entity by automatically capturing relevant metadata. In this context, fields like @CreatedDate
, @CreatedBy
, @LastModifiedDate
, and @LastModifiedBy
serve specific purposes:
@CreatedDate
: This field records the timestamp when the entity was first created, providing a reference for when the data was added to the system.@CreatedBy
: This field captures the identity of the user who created the entity, enhancing accountability by linking actions to specific users.@LastModifiedDate
: This field tracks the most recent timestamp when the entity was updated, allowing you to know when changes occurred last.@LastModifiedBy
: This field records the identity of the user who last modified the entity, helping to maintain an audit trail of changes over time.
Together, these auditing fields facilitate effective monitoring of data changes, ensuring transparency and accountability within the application. They provide valuable insights for compliance, troubleshooting, and understanding the history of data modifications.
If we want to update anything, such as the title, description, or both, we need to use the @PutMapping
. Here’s the controller and service layer code.
Update Product Endpoint Controller Layer
updateProduct
Method in the Service Layer
Output#
PostMan:
This section can include the expected response or result from a request made via Postman, such as the JSON response body, status code, and any relevant headers.
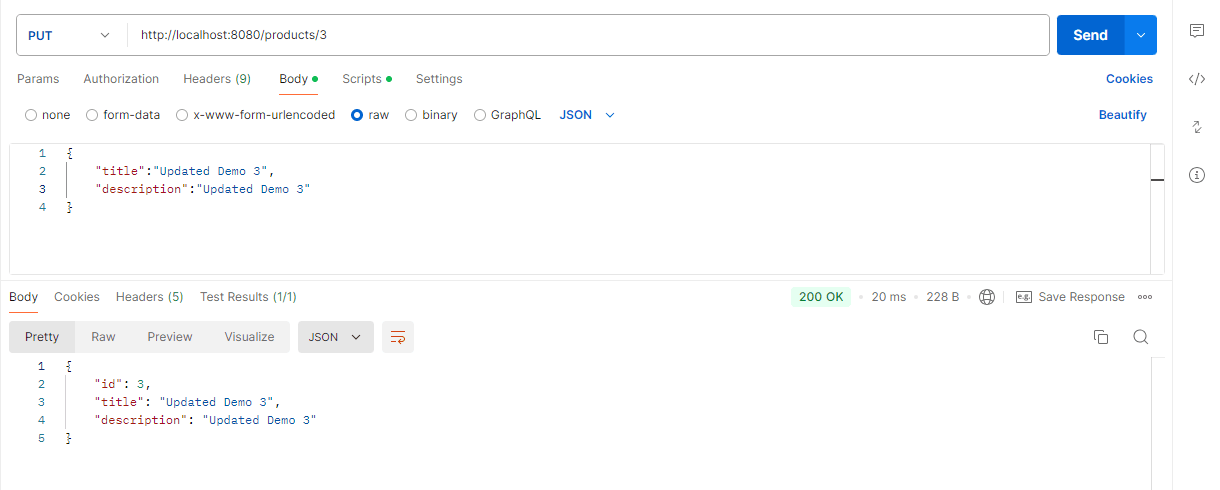
Databse:
Here, you can describe the expected changes in the database after the operation, including updated records, new entries, or any relevant queries to demonstrate the data state.
- Before Update id 3
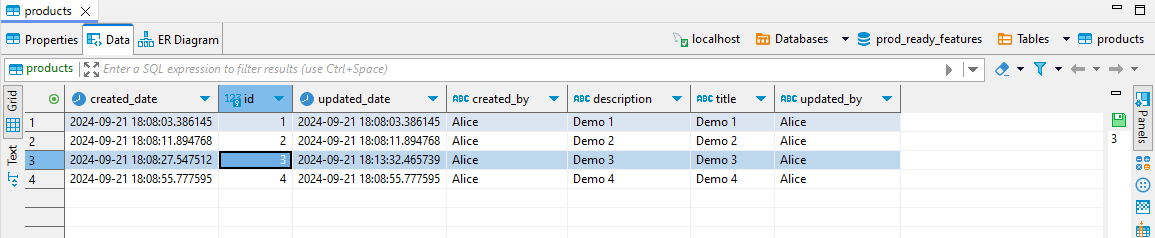
- After Update id 3
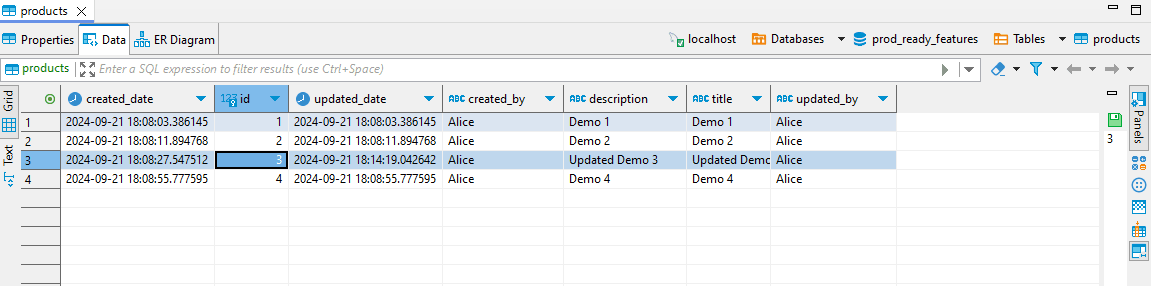
Internal working of Auditing#
The auditing feature in Spring Boot automates the tracking of entity changes by leveraging lifecycle event listeners and the AuditorAware
interface to capture user and timestamp information seamlessly. This approach enhances data integrity and accountability in your application without requiring additional boilerplate code.
Entity Lifecycle Events:
- When an entity is persisted or updated, the
AuditingEntityListener
is triggered. - This listener handles the following lifecycle events:
@PrePersist
: This method is called before an entity is saved for the first time. During this phase, the listener populates the fields annotated with@CreatedDate
and@CreatedBy
.@PreUpdate
: This method is invoked before an entity is updated. It sets the fields annotated with@LastModifiedDate
and@LastModifiedBy
.@PreRemove
: This method is called before the entity manager executes theremove()
operation on the entity. It is an appropriate place for implementing any necessary cleanup or validation logic.
AuditorAware Interface:
- The
AuditorAware
interface is crucial for providing the current user’s information during the auditing process. - When the auditing listener is triggered, it calls the
getCurrentAuditor()
method of theAuditorAware
implementation you created. - This method typically retrieves the current authenticated user's information, often using Spring Security's context to ensure that the correct user is logged in.
Hibernate Envers#
Hibernate Envers is a module of Hibernate that provides auditing and versioning capabilities for entity classes. It allows you to track changes to your entities over time by creating a history of their states. Here’s a brief overview of its features and how it works:
Key Features of Hibernate Envers:#
- Audit History: Envers automatically creates audit tables for your entities, storing historical data each time an entity is created, modified, or deleted.
- Versioning: It keeps track of all changes, allowing you to retrieve past versions of an entity, making it useful for data recovery and compliance.
- Simple Integration: Envers integrates seamlessly with Hibernate and requires minimal configuration to get started.
- Customizable: You can customize which fields to audit and how to handle specific actions (like ignoring certain fields or excluding certain entities).
- Querying Historical Data: It provides APIs to easily query historical data, making it straightforward to access previous states of entities.
How Hibernate Envers Works:#
Add Dependency
Entity Configuration:
We annotate our entity classes with @Audited
. This indicates that Hibernate should track changes to these entities. Here, we add @PrePersist
, @PreUpdate
, @PreRemove
. The @Audited
annotation in Hibernate Envers is used to mark an entity class that you want to track for auditing purposes. When applied, it tells Envers to create an audit table for that entity, where it will store historical versions of the entity whenever changes are made.
Field Configuration:
We can apply @NotAudited
at the field level within an entity class or at the class level for an entire entity. The @NotAudited
annotation in Hibernate Envers is used to indicate that specific fields or entities should not be tracked or included in the audit history. When applied, any changes to the annotated field or entity will not be recorded in the corresponding audit tables.
Output
After running the application, we can observe that the relevant tables, including the audit tables created by Hibernate Envers, appear in our database. This confirms that the auditing features have been successfully integrated.
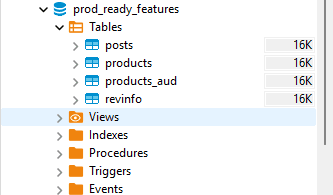
- PostMan
- Post Request
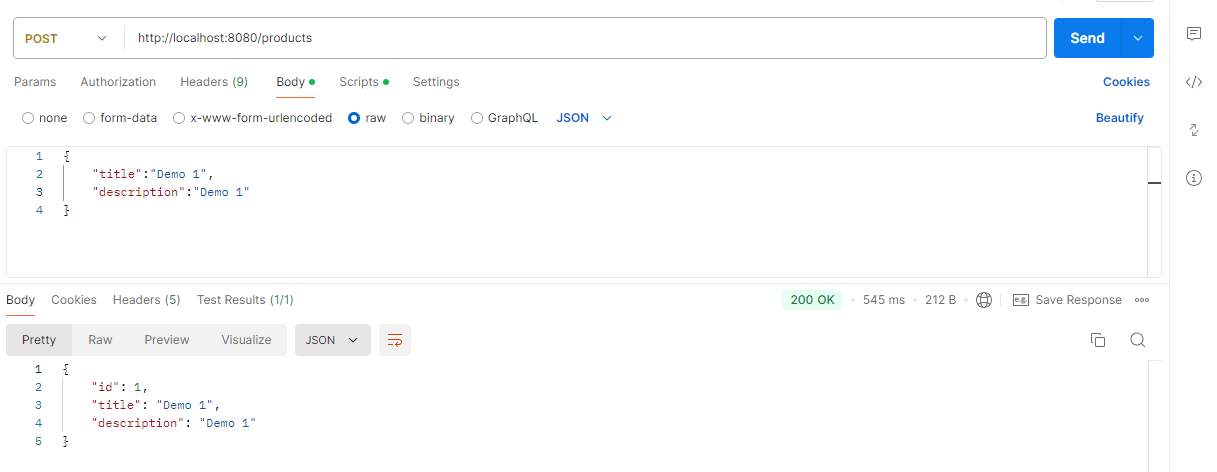
- Put Request
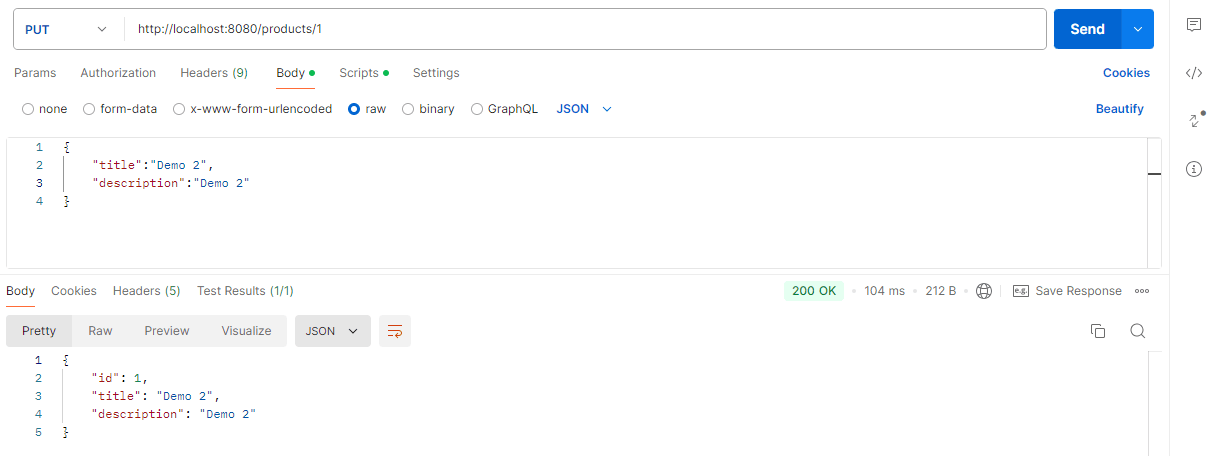
- Database
Products with createdDat, createdBy and updatedDate, updatedBy.
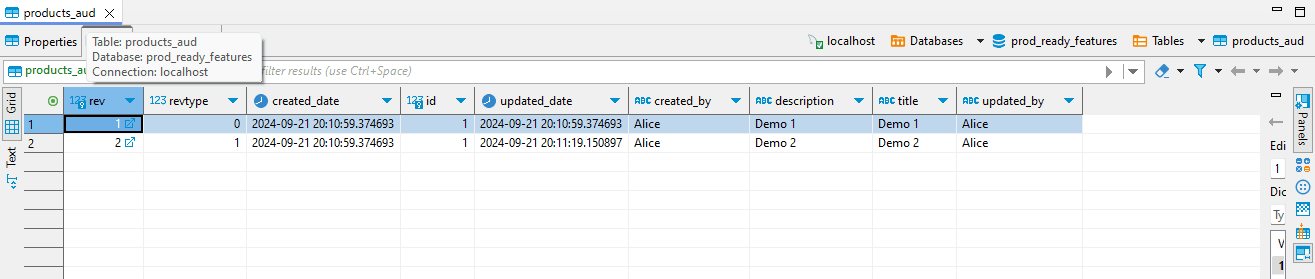
Table of revinfo.
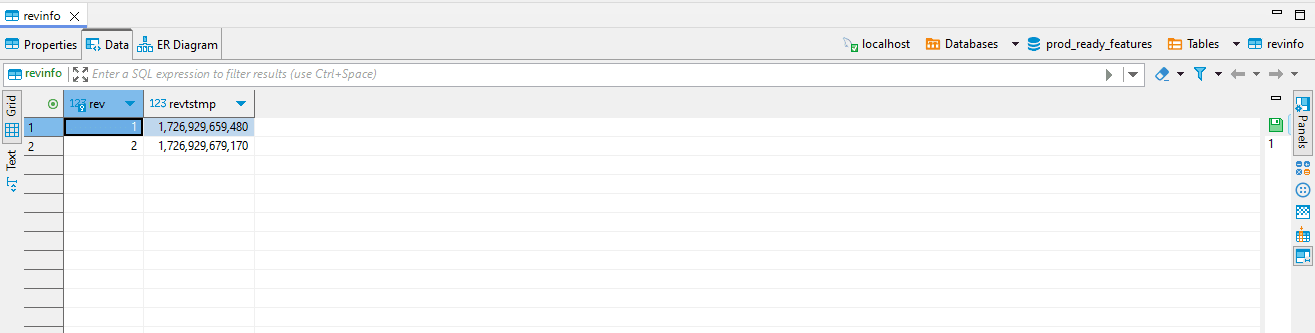
When to Use Hibernate Envers:#
- Data Auditing:
- When your application requires a history of changes made to entity data, such as in financial applications, content management systems, or any data-driven application where accountability is important.
- Regulatory Compliance:
- If you need to comply with legal or regulatory requirements that mandate tracking changes to data (e.g., healthcare, finance), Envers provides a straightforward way to maintain an audit trail.
- Version Control:
- When you need to keep track of different versions of an entity. This is useful for applications that need to provide the ability to roll back to previous states or review historical data.
- Data Recovery:
- In scenarios where data might be accidentally deleted or modified, having a historical record allows for easy restoration of previous states.
- Change Tracking:
- When you want to monitor who changed what and when, providing accountability and traceability for user actions in the application.
Why Use Hibernate Envers:#
- Automatic Change Tracking:
- Envers automates the process of auditing by creating and managing audit tables, reducing the manual overhead associated with tracking changes.
- Minimal Configuration:
- Integrating Envers into your Hibernate application requires minimal setup, making it easy to add auditing features without extensive code changes.
- Query Historical Data:
- Envers provides a convenient API to query past versions of entities, allowing developers to easily access historical data without complex queries.
- Customizable Auditing:
- You can customize which fields to audit, ignore specific changes, and define custom revision entities to capture additional metadata.
- Seamless Integration:
- Envers integrates seamlessly with Hibernate, so you can continue using your existing entity model without significant alterations.
Limitation#
- Complexity and Overhead: It introduces additional complexity and a learning curve, and may impact performance in high-transaction environments.
- Limited Flexibility: Some projects require highly customized auditing solutions that Envers may not provide.
- Not Always Necessary: For simpler applications, the added complexity of Envers may be unnecessary, especially if existing solutions are in place.
- Database Schema Changes: Adding audit tables can complicate database migrations and management.
- Development and Maintenance: Maintaining audit tables can create operational overhead and manage data volume.
- Compatibility Issues: Potential integration challenges with other frameworks may lead developers to seek alternative solutions.
Create Some APIs for Admin#
Controller
Output
After running the application.
- PostMan: If you create a new product and update its information by hitting the GET
/audit/products/{id}
endpoint, you can view all the related data, including the updated product details.
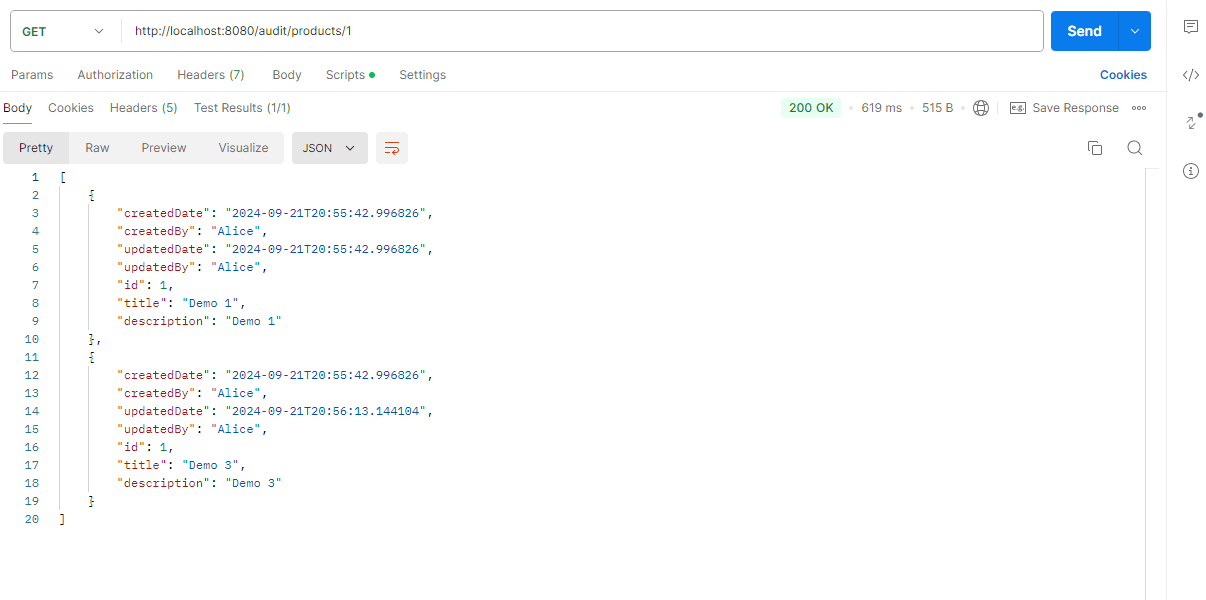
- Database:
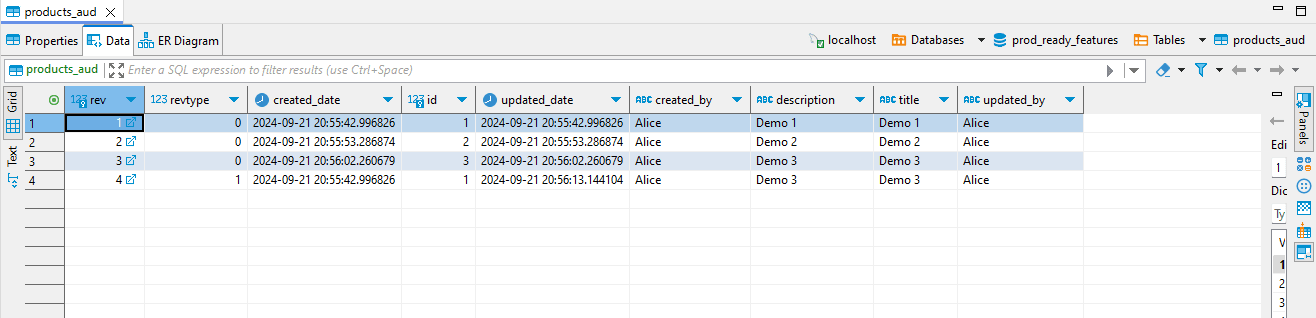
Conclusion#
This article explains how to implement auditing and advanced auditing using Hibernate Envers in Spring Boot to track changes in data and maintain accountability. It covers enabling JPA auditing, using AuditorAware for user tracking, and integrating Hibernate Envers for entity versioning. The focus is on improving security, compliance, and traceability within applications.