Asynchronous Task Scheduling in Spring Boot
Introduction#
It should be noted that there are various performance degradation areas associated with a long-running or time-sequenced task during synchronization. Their asynchronous execution capability places them among all other tasks in the application context and, finally, leads to their efficient execution without blocking the application thread.
Spring Boot has built-in asynchronous task execution support with:#
@Async
Annotation: Runs the method in a separate thread.TaskScheduler
and@Scheduled
Annotation: Automatically execute periodic tasks with fixed intervals.- Executor Configuration: Controls the configuration of thread pools for the concurrent running of tasks.
All these techniques help to achieve effective performance optimization while keeping the application running smoothly.
Default @Scheduled#
@Scheduled
tasks in Spring Boot run single-threaded by default through a single-thread executor. A delay occurs on the next execution when the task goes beyond the scheduled interval.
Use for concurrent execution:
@EnableAsync
along with@EnableScheduling
.@Async
is a method annotated with@Scheduled
to run the task on a different thread.
Scheduling tasks on a particular custom thread pool executor will ensure that the scheduled tasks run without blocking each other, resulting in a good performance boost for the application itself.
Async Task Scheduling#
To run long-residing jobs independently so that they do not block other jobs, use an @Async
with @Scheduled
one.
Steps:#
Enable async execution: Put @EnableAsync
and @EnableScheduling
.
Mark methods with@Async
: Each scheduled task runs in a separate thread.
Benefits:#
task1()
andtask2()
are independent.- No long execution will affect blocking.
- Great application performance.
Custom Executor#
By default, Spring Boot creates unlimited threads for its @Async
; memory leaks, therefore, could result.
Solution: Use a Custom Executor#
- Define an
Executor
Bean with a fixed thread pool.
- Specify the Executor in
@Async("customTaskExecutor")
.
Output:#
@Async()
(Default Executor)- Uses Spring Boot's default task executor (
SimpleAsyncTaskExecutor
). - Creates a new thread per task, leading to unlimited thread creation.
- Output will show dynamically generated thread names like:
- Uses Spring Boot's default task executor (
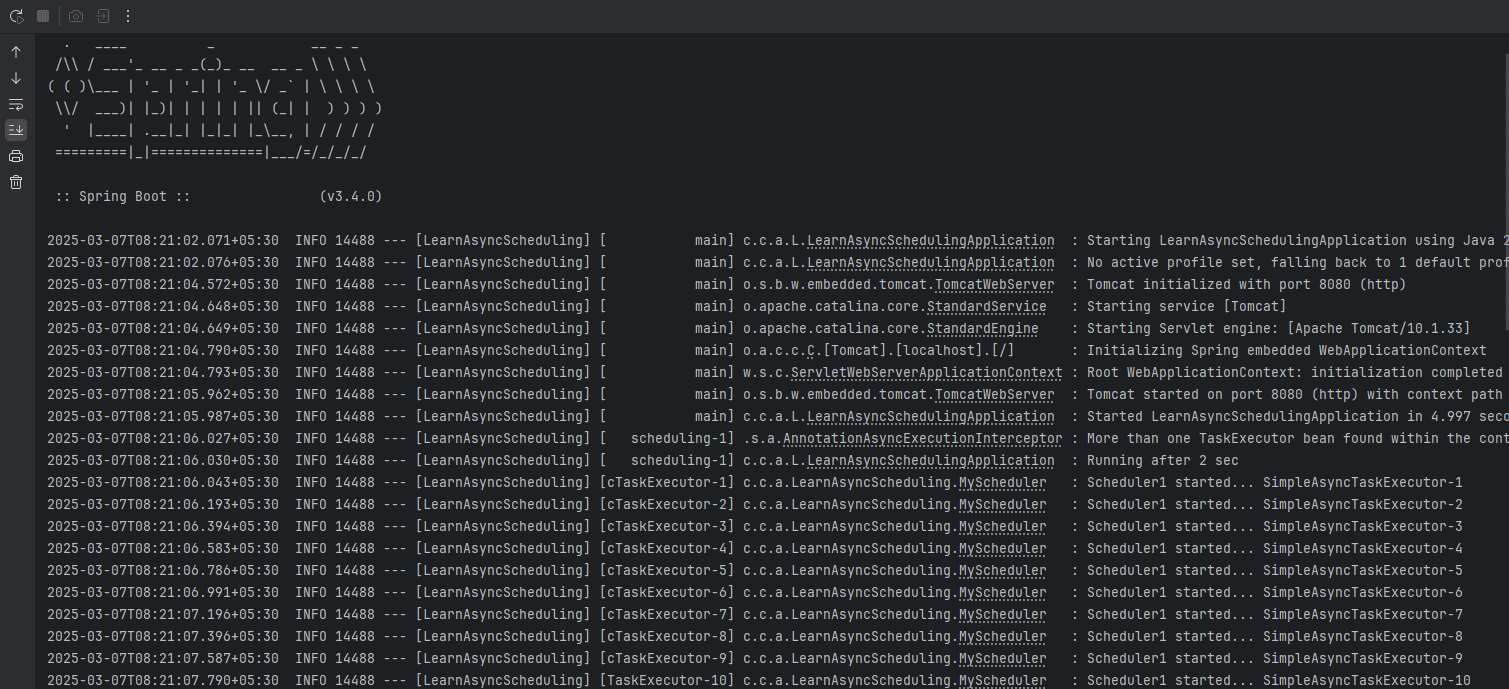
@Async("jobExecutor")
(Custom Executor)- Uses a customly defined thread pool (e.g.,
ThreadPoolTaskExecutor
). - Thread count is limited based on pool size settings.
- Output will show controlled thread names like:
- Uses a customly defined thread pool (e.g.,
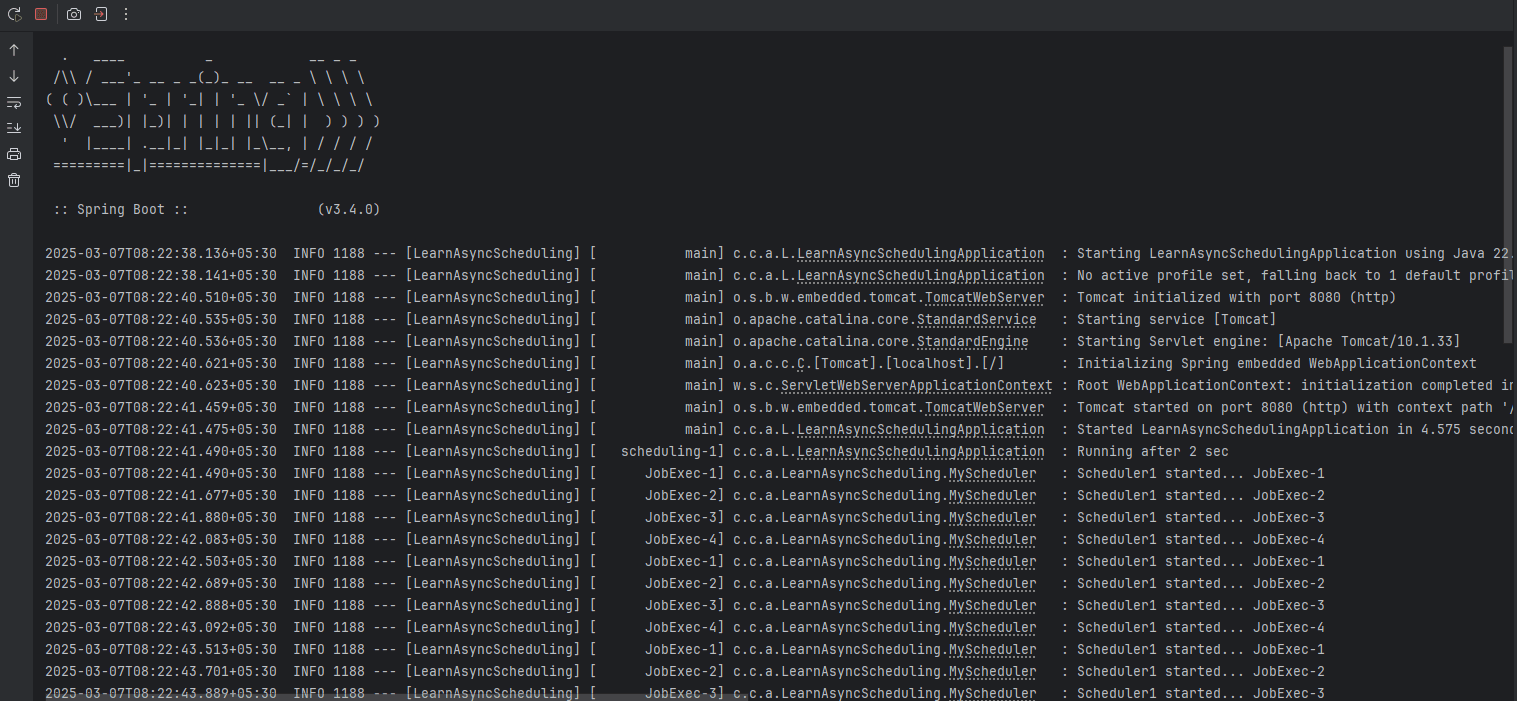
Benefits:#
- Prevents excessive thread creation.
- Optimizes performance with controlled concurrency.
- Avoids memory leaks by limiting threads.
Conclusion#
This article introduces the Spring Boot asynchronous task-scheduling framework through @Scheduled
and @Async
to run tasks in parallel. It comments on the limitations of the default executor and the possibility of running out of control while creating threads. To enhance performance and avert memory leaks, it proposes that a custom thread-pool executor be opted for so that concurrency can be controlled.