Understanding Collection Interface in Java
The Collection
interface is the root interface of the Java Collection Framework and defines the fundamental methods used by all collection types. In this blog, we will explore the Collection
interface in detail, understand its importance, and learn how to use it effectively with examples.
What is the Collection Interface?#
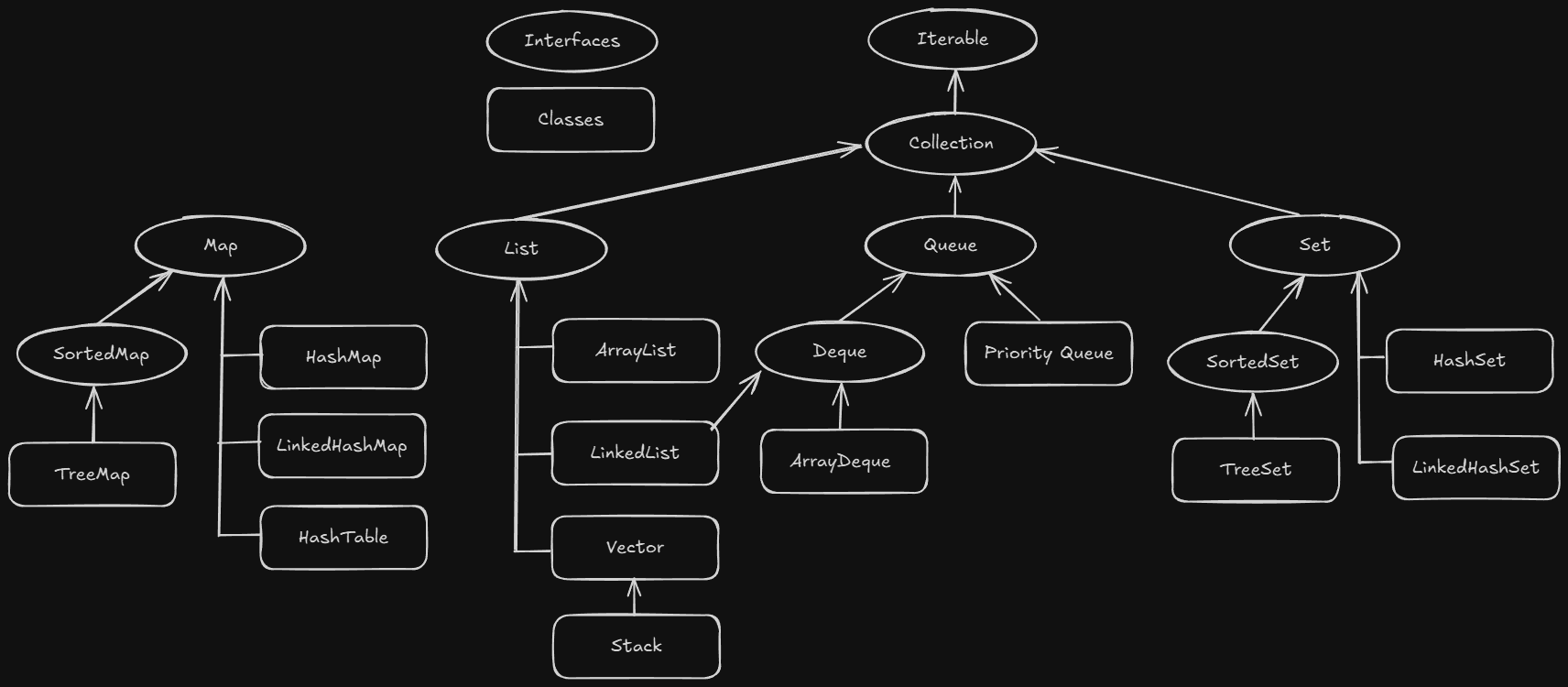
The Collection
interface is part of java.util
package and is the base interface for all collections like List, Set, and Queue. It extends the Iterable<T>
interface, allowing collections to be iterated using an iterator or enhanced for-loop.
Why is the Collection Interface Important?#
- Provides a common structure: All collections follow a standard set of methods.
- Encourages abstraction: Developers can write generic code that works with any collection type.
- Supports Polymorphism: A method can accept
Collection<?>
as a parameter, making it flexible.
Key Methods of the Collection Interface#
The Collection
interface provides several useful methods that all collections must implement.
1. Adding Elements#
Method | Description |
---|---|
add(E e) | Adds a single element to the collection. |
addAll(Collection<? extends E> c) | Adds all elements from another collection. |
Example:#
Output:
2. Removing Elements#
Method | Description |
---|---|
remove(Object o) | Removes a single instance of the specified element. |
removeAll(Collection<?> c) | Removes all elements present in another collection. |
clear() | Removes all elements from the collection. |
Example:#
Output:
3. Checking Elements#
Method | Description |
---|---|
contains(Object o) | Checks if the collection contains the specified element. |
containsAll(Collection<?> c) | Checks if all elements of another collection exist in this collection. |
Example:#
Output:
4. Size and Empty Check#
Method | Description |
---|---|
size() | Returns the number of elements in the collection. |
isEmpty() | Checks if the collection is empty. |
Example:#
Output:
5. Iterating Over Elements#
Method | Description |
---|---|
iterator() | Returns an iterator to traverse elements. |
Example:#
Output:
Conclusion#
In this blog, we explored the Collection
interface, its importance, and how to use its methods. This interface forms the foundation for Lists, Sets, and Queues, allowing flexible and efficient data handling. In the next blogs, we will dive deeper into specific collection types like List, Set, Queue, and Map!