Java While and Do-While Loop
In Java, loops are used to execute a block of code repeatedly based on a given condition. While loops and do-while loops are two types of loops in Java that allow you to do this.
In this blog, we’ll explore the while loop and do-while loop, explain their syntax, and provide examples to help you understand when and how to use them.
What is a While Loop?#
The while loop repeatedly executes a block of code as long as a given condition is true
. It’s often used when you don’t know exactly how many times you need to loop, but you know the condition to stop.
Syntax of a While Loop#
- condition: This is the test condition that’s evaluated before each iteration. If the condition evaluates to
true
, the loop body is executed. If the condition evaluates tofalse
, the loop terminates. - The loop keeps running until the condition becomes
false
.
Basic Example of a While Loop#
Let’s start with a simple example to demonstrate how a while loop works.
Example 1: Print Numbers from 1 to 5#
Explanation:
- Initialization:
int i = 1
sets the initial value ofi
. - Condition:
i <= 5
ensures the loop runs as long asi
is less than or equal to 5. - Update:
i++
incrementsi
by 1 after each iteration. - The loop prints the numbers from 1 to 5.
Expected Output:
What is a Do-While Loop?#
The do-while loop is similar to the while loop, but with one key difference: the condition is checked after the loop’s body is executed. This guarantees that the loop body will run at least once, even if the condition is false.
Syntax of a Do-While Loop#
- The condition is checked after the loop executes the code block.
- The loop body executes at least once, regardless of the condition.
Flow chart:
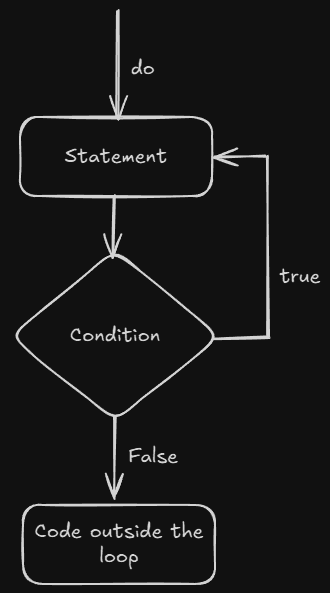
Basic Example of a Do-While Loop#
Let’s now see a simple example to understand how the do-while loop works.
Example 2: Print Numbers from 1 to 5 using Do-While Loop#
Explanation:
- Initialization:
int i = 1
sets the initial value ofi
. - Condition:
i <= 5
ensures the loop runs as long asi
is less than or equal to 5. - Update:
i++
incrementsi
by 1 after each iteration. - The loop prints the numbers from 1 to 5, but it will always execute the body at least once, even if
i
were initially greater than 5.
Expected Output:
Difference Between While and Do-While Loop#
The primary difference between the while loop and the do-while loop is when the condition is checked:
- In the while loop, the condition is checked before the loop starts executing, which means the code inside the loop may never execute if the condition is initially false.
- In the do-while loop, the condition is checked after the loop has executed, ensuring that the loop body runs at least once, even if the condition is false initially.
Example 3: Do-While Loop Always Runs at Least Once#
Explanation:
- Even though the condition
i <= 5
is false from the start, the loop executes the block of code at least once because it’s a do-while loop.
Expected Output:
Infinite Loops#
Both the while loop and do-while loop can be used to create an infinite loop if the condition is always true
. This is useful when you need the program to keep running until some external condition occurs (like user input or a system event).
Example 4: Infinite While Loop#
Explanation:
- The condition
true
makes the while loop run indefinitely. - The
break
statement is used to exit the loop after one iteration.
Expected Output:
Example 5: Infinite Do-While Loop#
Explanation:
- The condition
true
makes the do-while loop run indefinitely. - The
break
statement is used to exit the loop after one iteration.
Expected Output:
Conclusion#
In this blog, we’ve learned:
- The while loop executes as long as a condition is true, and the condition is checked before each iteration.
- The do-while loop is similar to the while loop, but it guarantees the loop body will execute at least once because the condition is checked after the loop executes.
- We explored the differences between these loops, infinite loops, and practical examples to solidify your understanding.
Understanding while and do-while loops is important as they allow you to handle situations where the number of iterations is unknown or when the loop must run at least once.