Java Stack
The Stack
class in Java is a part of the java.util
package and extends the Vector
class. It represents a Last-In-First-Out (LIFO) data structure, where the last element added is the first one to be removed.
1. What is Stack?#
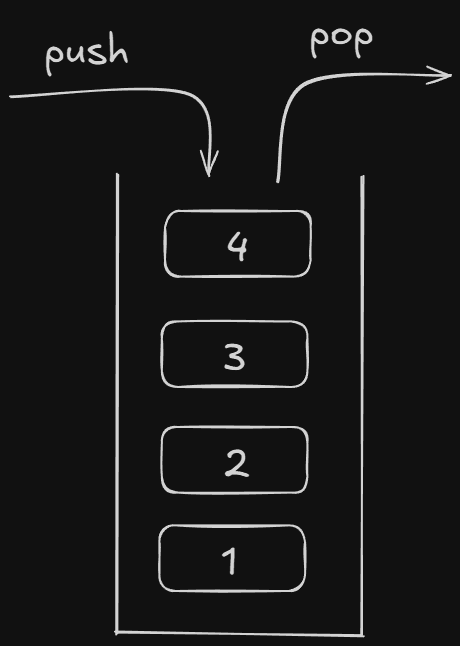
Stack
is a subclass ofVector
.- It works on LIFO (Last in first out )principle. i.e element which is entered last will be removed first.
- It provides five key methods:
push()
,pop()
,peek()
,search()
, andempty()
.
But what is Vector?#
Since Stack
extends Vector
, it's important to understand what a Vector is:
Vector
is a growable array of objects.- It is part of the legacy collection classes and was introduced in JDK 1.0.
- Like
ArrayList
,Vector
uses a dynamic array to store elements. - The key difference:
Vector
is synchronized, which makes it thread-safe, whereasArrayList
is not.
Why Stack extends Vector?#
When Stack
was introduced in Java, Vector
was one of the only dynamic array-based structures that supported synchronization. To build on that functionality, Stack
was created by extending Vector
and adding LIFO-specific methods like push()
, pop()
, and peek()
.
However, note that today Deque
(like ArrayDeque
) is often preferred due to better performance and flexibility.
2. Stack Methods with Examples#
1. push(E item)
– Adds an element to the top#
Output:
2. pop()
– Removes and returns the top element#
Output:
3. peek()
– Returns the top element without removing#
Output:
4. search(Object o)
– Searches the element and returns its 1-based position from the top#
Output:
5. empty()
– Checks if the stack is empty#
Output:
3. Use Cases of Stack#
- Expression Evaluation (Postfix, Prefix)
- Undo Mechanism in editors
- Backtracking Algorithms (e.g., maze solving)
- Browser History Navigation
4. Stack vs Other List Implementations#
Feature | Stack | Vector | ArrayList | LinkedList |
---|---|---|---|---|
Internal Structure | Dynamic Array | Dynamic Array | Dynamic Array | Doubly Linked List |
Thread-Safe | Yes | Yes | No | No |
Order | LIFO | Insertion | Insertion | Insertion |
Special Ops | push, pop, peek | None | None | add/remove first/last |
Conclusion#
The Stack
class is a useful utility for handling LIFO-based data structures in Java. Though it's a legacy class and newer alternatives like Deque
(via ArrayDeque
) are often preferred, it's still important to understand Stack due to its simple usage and appearance in many interview problems. In the next blog, we will explore Queue Interface in Java and its implementations!