Java Multidimensional Arrays
In Java, arrays can have multiple dimensions. A multidimensional array is an array of arrays, meaning each element of the main array can be another array. This is useful when dealing with tabular data, matrices, or grids.
In this blog, we will cover:
- What is a Multidimensional Array?
- How to Declare a Multidimensional Array
- How to Initialize a Multidimensional Array
- Accessing and Modifying Elements
- Looping Through a Multidimensional Array
- Example Programs with Expected Outputs
- Limitations of Multidimensional Arrays
What is a Multidimensional Array?#
A multidimensional array is an array where each element is itself an array. The most commonly used multidimensional array is a two-dimensional array (2D array).
Example of a 2D array:
Here, each row is an array containing multiple elements.
How to Declare a Multidimensional Array#
In Java, we declare a 2D array as follows:
For example:
We can also declare higher-dimensional arrays (3D, 4D, etc.), but 2D is the most commonly used.
How to Initialize a Multidimensional Array#
We can initialize a 2D array in multiple ways.
1. Using new
Keyword#
This creates a 3x3 array with default values (0
for integers).
2. Direct Initialization#
Here:
numbers[0] = {1, 2, 3}
numbers[1] = {4, 5, 6}
Accessing and Modifying Elements#
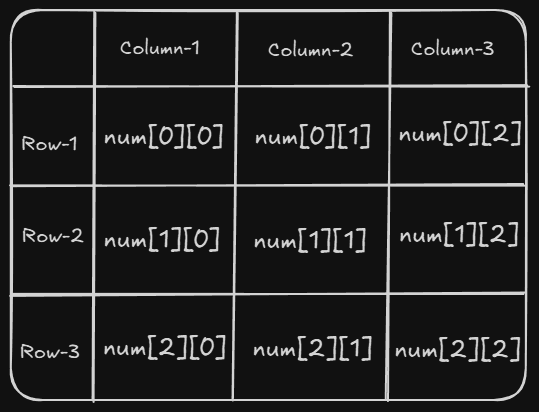
To access elements, we use row index and column index.
Output: 25
Looping Through a Multidimensional Array#
We can iterate through a 2D array using nested loops.
1. Using for
Loop#
Output#
2. Using Enhanced for
Loop#
Output#
Example Programs#
Example 1: Sum of All Elements in a 2D Array#
Output#
Example 2: Find Maximum Element in a 2D Array#
Output#
Jagged Arrays in Java#
A jagged array is a multidimensional array where each row can have a different number of columns.
Example:
Output#
Limitations of Multidimensional Arrays#
- Increased Memory Usage: Multidimensional arrays require more memory.
- Difficult to Manage: Nested loops make it more complex to work with.
- Fixed Size: Once declared, the size cannot be changed.
Conclusion#
In this blog, we learned:
- What multidimensional arrays are and why they are useful.
- How to declare, initialize, and access elements in a 2D array.
- How to loop through a multidimensional array using
for
andfor-each
loops. - Example programs: Finding sum and maximum elements in a 2D array.
- Introduction to jagged arrays.
- Limitations of using multidimensional arrays.
Multidimensional arrays are useful in cases like matrices, graphs, and gaming applications. However, for dynamic data structures, ArrayLists or HashMaps are preferred.