Java LinkedList
The LinkedList
class in Java is part of the Java Collections Framework and implements both the List
and Deque
interfaces. It provides a doubly-linked list data structure.
1. What is a LinkedList?#
LinkedList
is a doubly-linked list.- It allows elements to be added or removed from both ends.
- It can store duplicate and
null
elements.
2. Internal Working#
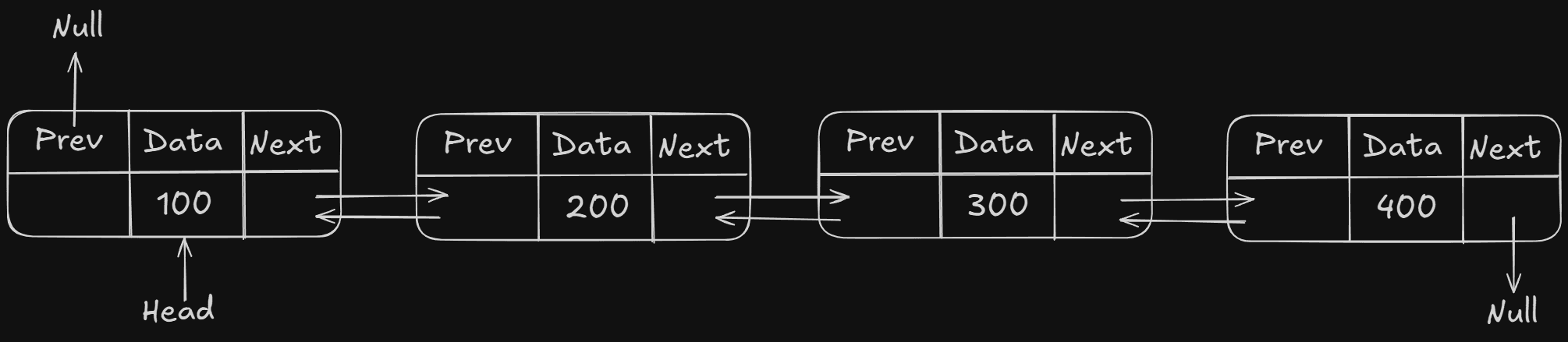
- Each node contains a data element, a reference to the previous node, and a reference to the next node.
- Enables constant-time insertions and removals using iterators.
3. Constructors#
4. Commonly Used Methods with Examples#
1. add(E e)
– Adds element at the end#
Output:
2. add(int index, E element)
– Inserts at a specific index#
Output:
3. addFirst(E e)
– Adds to the beginning#
Output:
4. addLast(E e)
– Adds to the end#
Output:
5. get(int index)
– Retrieves element at a given index#
Output:
6. getFirst()
– Gets the first element#
Output:
7. getLast()
– Gets the last element#
Output:
8. remove()
– Removes and returns the first element#
Output:
9. remove(int index)
– Removes element at a given index#
Output:
10. removeFirst()
– Removes the first element#
Output:
11. removeLast()
– Removes the last element#
Output:
12. contains(Object o)
– Checks if an element exists#
Output:
13. size()
– Returns the number of elements in the list#
Output:
14. isEmpty()
– Checks if the list is empty#
Output:
15. clear()
– Removes all elements#
Output:
16. offer(E e)
– Adds element at the end (queue style)#
Output:
17. peek()
– Retrieves the head element without removing it#
Output:
18. poll()
– Retrieves and removes the head element#
Output:
19. descendingIterator()
– Iterates in reverse order#
Output:
5. Performance#
Operation | Time Complexity |
---|---|
Access by index | O(n) |
Add/remove at ends | O(1) |
Add/remove in middle | O(n) |
6. Thread Safety#
LinkedList
is not synchronized.- For a thread-safe version:
7. When to Use LinkedList?#
- When frequent insertion or removal is required.
- When random access is not a priority.
- For implementing queues, stacks, and deques.
8. Best Practices#
- Prefer using the
List
interface:
- Avoid frequent index-based access when performance is critical.
Conclusion#
The LinkedList
is a versatile structure for dynamic data handling, especially where frequent modifications are needed. It shines in scenarios requiring sequential access and frequent insertions or deletions.