Java HashMap
Introduction#
The HashMap
class is one of the most commonly used data structures in Java, designed to store key-value pairs efficiently. It is a part of the java.util
package and implements the Map
interface.
This blog will guide you through everything you need to know about HashMap
, including its working mechanism, key methods, examples, internal performance considerations, and a conclusion.
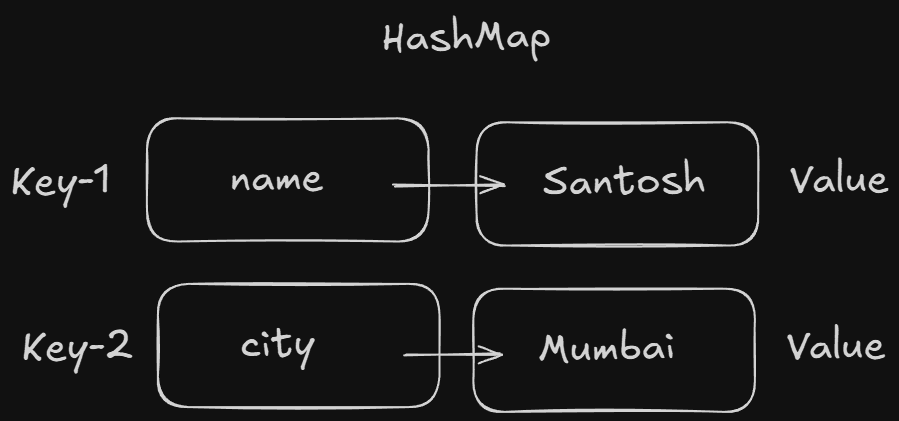
Key Characteristics#
- Implements the
Map
interface. - Stores data as key-value pairs.
- Keys must be unique.
- Allows one
null
key and multiplenull
values. - Does not maintain any insertion or sorted order.
- Not synchronized (not thread-safe).
HashMap Syntax#
Example:
Commonly Used Methods in HashMap (With Examples and Output)#
1. put(K key, V value)
– Add or update key-value pair#
Note:- Insertion order is not maintained here, To maintain insertion order use LinkedHashMap which we will see in our next blog.
Output:
2. get(Object key)
– Retrieve value for a key#
Output:
3. remove(Object key)
– Remove mapping by key#
Output:
4. containsKey(Object key)
– Check if key exists#
Output:
5. containsValue(Object value)
– Check if value exists#
Output:
6. size()
– Total number of key-value pairs#
Output:
7. isEmpty()
– Check if map is empty#
Output:
8. clear()
– Remove all key-value pairs#
Output:
9. keySet()
– Get all keys as a Set
#
Output:
10. values()
– Get all values as a Collection
#
Output:
11. entrySet()
– Get all key-value pairs as a Set
of Map.Entry
#
Output:
Example: Basic HashMap Usage#
Output:
Example: Iterating through HashMap#
Output:
Internal Working of HashMap#
- Internally, HashMap uses an array of Node objects (buckets).
- When you insert a key, it computes the hash code, which determines the bucket index.
- If multiple keys map to the same index (collision), the entries are stored in a linked list or balanced tree (Java 8+).
Performance and Time Complexity#
Operation | Time Complexity |
---|---|
put() | O(1) average, O(n) worst (due to collisions) |
get() | O(1) average, O(n) worst |
remove() | O(1) average, O(n) worst |
Note: From Java 8, when a bucket has more than 8 entries, the linked list is replaced by a balanced tree, improving worst-case time complexity to O(log n).
When to Use HashMap#
- When you need fast lookup and insertion.
- When order of elements doesn’t matter.
- When you can ensure only one thread is accessing the map (or use
Collections.synchronizedMap()
orConcurrentHashMap
).
Conclusion#
In this blog, we explored the HashMap
class in Java in detail. We covered how it stores data in key-value pairs, its key methods with examples and outputs, how it works internally, performance characteristics, and when to use it in real-world applications.
In the next blog, we will explore LinkedHashMap
, which maintains the insertion order of key-value pairs while offering similar performance characteristics.