Java For Loop
In Java, a for loop is one of the most commonly used control flow statements. It allows you to repeatedly execute a block of code for a fixed number of iterations. This makes it an essential tool for performing repetitive tasks, especially when you know in advance how many times you need to execute a particular statement or block of code.
In this blog, we’ll explore how the for loop works, its syntax, and provide examples to help you understand its usage clearly.
What is a For Loop?#
The for loop is used when you know the number of iterations you need to execute. It is ideal for iterating through collections, arrays, or performing repetitive tasks.
Syntax of a For Loop#
- initialization: This step is executed once, before the loop starts. It is typically used to initialize loop control variables.
- condition: This is evaluated before each iteration. If the condition evaluates to
true
, the loop body is executed. If it evaluates tofalse
, the loop terminates. - update: After each iteration, the update statement is executed. It is typically used to modify the loop control variable (like incrementing or decrementing).
Flow Chart:
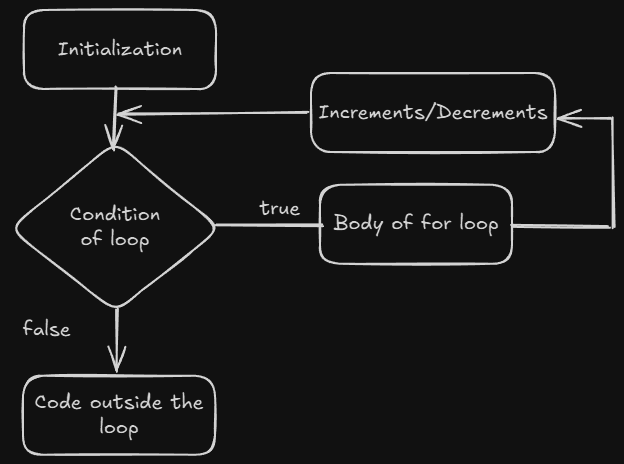
Basic Example of a For Loop#
Let’s start with a simple example to demonstrate the basic structure of a for loop.
Example 1: Print Numbers from 1 to 5#
Explanation:
- Initialization:
int i = 1
sets the starting value ofi
to 1. - Condition:
i <= 5
checks ifi
is less than or equal to 5. - Update:
i++
incrementsi
by 1 after each iteration. - This loop prints the numbers from 1 to 5.
Expected Output:
For Loop with Multiple Statements#
You can also have multiple statements in the initialization, condition, or update sections of a for loop. This is useful when you need to modify more than one variable.
Example 2: Multiple Statements in For Loop#
Explanation:
- Initialization:
int i = 1, j = 10
initializes two variables,i
andj
. - Condition:
i <= 5
checks ifi
is less than or equal to 5. - Update:
i++
incrementsi
by 1, andj -= 2
decrementsj
by 2 after each iteration. - This loop prints the values of
i
andj
in each iteration.
Expected Output:
For Loop with Arrays#
A for loop is frequently used to iterate through arrays. This is useful when you need to perform an operation on each element in the array.
Example 3: Iterate Through an Array#
Explanation:
- Initialization:
int i = 0
starts the loop at the first element of the array. - Condition:
i < numbers.length
ensures the loop continues as long asi
is less than the length of the array. - Update:
i++
incrementsi
by 1 in each iteration. - The loop prints each element in the
numbers
array.
Expected Output:
For Loop with a Decrementing Counter#
A for loop can also be used to count downwards, by using a decrementing counter. This is useful when you need to execute a block of code in reverse order.
Example 4: Count Downwards from 5#
Explanation:
- Initialization:
int i = 5
starts the loop at 5. - Condition:
i > 0
ensures the loop runs whilei
is greater than 0. - Update:
i--
decrementsi
by 1 after each iteration. - The loop prints numbers from 5 to 1 in reverse order.
Expected Output:
Infinite For Loop#
A for loop can be set to run infinitely by leaving out the condition, or setting it to always be true
. This is generally used when you need a loop to run indefinitely until a break condition is met.
Example 5: Infinite For Loop#
Explanation:
- The
for(;;)
creates an infinite loop, since the condition is alwaystrue
. - The
break
statement immediately exits the loop after the first print statement. - Without the
break
, the loop would run forever.
Expected Output:
For-Each Loop (Enhanced For Loop)#
In addition to the traditional for loop
, Java also provides a for-each loop to simplify the iteration over arrays or collections.
Example 6: For-Each Loop#
Explanation:
- The for-each loop simplifies iterating over an array.
- It automatically accesses each element in the array one by one, without needing an index.
Expected Output:
Conclusion#
In this blog, we learned:
- The for loop is a fundamental control flow statement in Java used for iterating over a fixed number of iterations.
- We explored how to use the for loop with initialization, conditions, and updates.
- We also learned how to use the for loop with arrays and collections, as well as for counting upwards or downwards.
- Additionally, we looked at the for-each loop as a simpler way to iterate over arrays.
Mastering the for loop is crucial for efficient coding, especially when you need to perform repetitive tasks in a concise and readable manner.