Java ArrayDeque
The ArrayDeque
class in Java is a resizable-array implementation of the Deque interface. Unlike regular queues or stacks, ArrayDeque
supports insertion and removal at both ends, making it ideal for queue and stack operations.
It is faster than LinkedList
for most queue operations and does not allow null elements.
Key Characteristics#
- Implements Deque, Queue, and Iterable interfaces.
- Backed by a resizable array.
- Faster than
Stack
andLinkedList
for stack/queue operations. - Null elements are not allowed.
- Not thread-safe (must use externally synchronized if needed).
Internal Working#
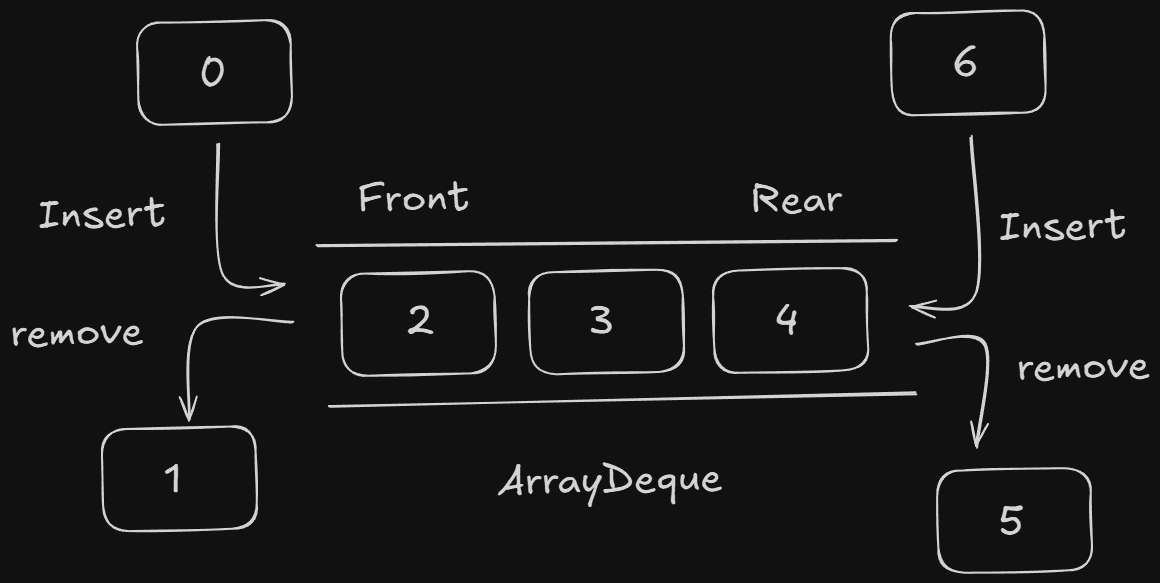
- Backed by a circular array.
- When the array is full, it resizes (doubles capacity).
- Offers amortized constant time for insertions/removals from both ends.
Constructors#
Constructor | Description |
---|---|
ArrayDeque() | Creates an empty deque with default capacity. |
ArrayDeque(int numElements) | Creates a deque with enough space for the given number of elements. |
ArrayDeque(Collection<? extends E> c) | Creates a deque initialized with elements from the specified collection. |
Commonly Used Methods (with Examples)#
1. addFirst(E e)
- Inserts element at the front#
Output:
2. addLast(E e)
- Inserts element at the end#
Output:
3. offerFirst(E e)
- Inserts at front, returns false if capacity restricted#
Output:
4. offerLast(E e)
- Inserts at rear#
Output:
5. removeFirst()
- Removes and returns the first element#
Output:
6. removeLast()
- Removes and returns the last element#
Output:
7. pollFirst()
- Retrieves and removes first element or null if empty#
Output:
8. pollLast()
- Retrieves and removes last element or null if empty#
Output:
9. peekFirst()
- Retrieves but doesn't remove the first element#
Output:
10. peekLast()
- Retrieves but doesn't remove the last element#
Output:
11. contains(Object o)
- Checks if deque contains the element#
Output:
12. size()
- Returns the number of elements#
Output:
13. clear()
- Removes all elements#
Output:
Performance Overview#
Operation | Time Complexity |
---|---|
addFirst() / addLast() | O(1) amortized |
removeFirst() / removeLast() | O(1) amortized |
peekFirst() / peekLast() | O(1) |
contains() | O(n) |
clear() | O(n) |
- Fast queue and stack operations.
- No overhead of node pointers (as in LinkedList).
Limitations#
- Not thread-safe.
- No capacity limit enforcement.
- Cannot store
null
elements (throwsNullPointerException
).
Real-World Use Cases#
- Browser history (forward/backward navigation).
- Undo/Redo functionality.
- Task Scheduling (round-robin execution).
- Palindrome checking (by comparing ends).
Conclusion#
ArrayDeque
is an efficient and flexible choice when you need a double-ended queue or a stack with better performance than Stack
or LinkedList
. It is an excellent general-purpose queue with fast and predictable performance for typical queue and stack use cases.