Spring Boot 0 to 100 Course 3.0 [NEW] - With Microservices, Kafka, Docker and Kubernetes
Spring Boot 0 to 100 Course 3.0 [2025] is here — upgraded and better than ever! Master Spring Boot, Microservices, Kafka, Docker, and Kubernetes with cutting-edge content that prepares you for your next dream Job as a Backend Engineer!
What you'll learn
Spring Framework Core Features - Spring IOC Container, Beans, AutoConfigurations
You will master the fundamentals of Spring Framework from scratch, no previous experience is required
Dependency Injection
Learn Spring MVC Concepts
Building projects with System design concepts included
Lombok - Simplify your Java code with Lombok.
Learning SQL and NoSQL databases
Spring Bean Data Validation
Exception Handling in Spring Boot Application
Reformatting the Response Object - Polish those responses until they shine!
Building Spring Boot REST APIs - Create REST APIs with ease
Mini Project: Restful Web Services - Put your skills to the test with a mini project
Master Spring Data JPA from Basics to Custom Queries with MySQL and PostgreSQL
Configuring Hibernate ORM with JDBC
Spring Data JPA Query Methods
Using DTO Pattern and Mapping Libraries
Monitor your applications with Spring Boot Actuator
Spring Documentation with Swagger and Open API
Supercharge your development with Spring Dev Tools
Logging - Keep track of everything with logging
Auditing - Keep track of App's History
REST Template and Third-Party APIs - Integrate third-party APIs with ease
Secure your REST APIs with Spring Security 6 and SQL Database
Create a registration and login system with Spring MVC, Spring Boot, Spring Data JPA, and MySQL
Third-Party Registration System with Google OAuth and JWT Authentication
Implement role-based access control with Spring Boot Authorization and SQL Database
Fine-tune roles and permissions, secure methods, and customize error pages
Secure passwords with encoding. Keep those passwords safe from prying eyes!
Master the User Session Management
Testing with JUnit and Assert Methods
Integration Tests for Reactive CRUD REST APIs
Master Mockito for unit testing
Use Spring Profiles to manage different environments like dev, stage and prod
Learn to creat buildspec to define the build flow
Create Pipelines for CI/CD using AWS Codepipeline
Deploying with CodePipeline and CodeDeploy on Elastic Beanstalk
Aspect-Oriented Programming
Various Advice Types: Before, After, After Throwing Advice
Redis Spring Cache to make your cache smarter and faster!
Implement publish/subscribe messaging with Redis
Using Apache Kafka in Spring Boot
Understanding Kafka's architecture
Kafka Publisher and Kafka Consumer
Spring Boot Messaging with Kafka
Introduction to Microservices
Monolithic vs. Microservice Architecture
Key Microservice Principles and Advantages
Creating REST APIs in Microservice Architecture
Microservices Communication using RestTemplate, WebClient, and Cloud OpenFeign
Service Registry and Discovery using Spring Cloud Netflix Eureka
API Gateway using Spring Cloud Gateway
Auto Refresh Config Changes using Spring Cloud Bus
Secure your microservices with JWT
Distributed Tracing with Spring Cloud Sleuth and Zipkin
Resilience4J: Circuit Breaker, Retry, and RateLimiter Patterns
Centralized Configuration using Spring Cloud Config Server
Centralized Logging using Elasticsearch, Logstash and Kibana Stack
Docker Setup and Commands
Dockering Spring Boot Application Step-by-Step
Learn Docker's role in microservices. Understand how Docker revolutionizes microservice deployment!
Use Docker Compose. Orchestrate multi-container Docker applications with ease!
Understand Kubernetes in Microservices. Master container orchestration with Kubernetes!
Orchestration using Kubernetes
Use the Kubernetes Dashboard. Visualize and manage your Kubernetes clusters with an intuitive UI!
Configure Kubernetes. Set up your Kubernetes clusters for optimal performance!
Deploying Microservices to Kubernetes Cluster
Utilizing Kubernetes services for load balancing and scaling
This course includes:
All Future Updates
Additional Projects-building lectures
90+ hours on-demand video
110+ downloadable resource
2 real-world Capstone projects
1:1 Doubts support by Mentors
Basics of System Design + DevOps
Certificate of completion
What you'll build
Learn by building. In this course, you will be working on multiple micro-skilling exercises as well as major projects like these:
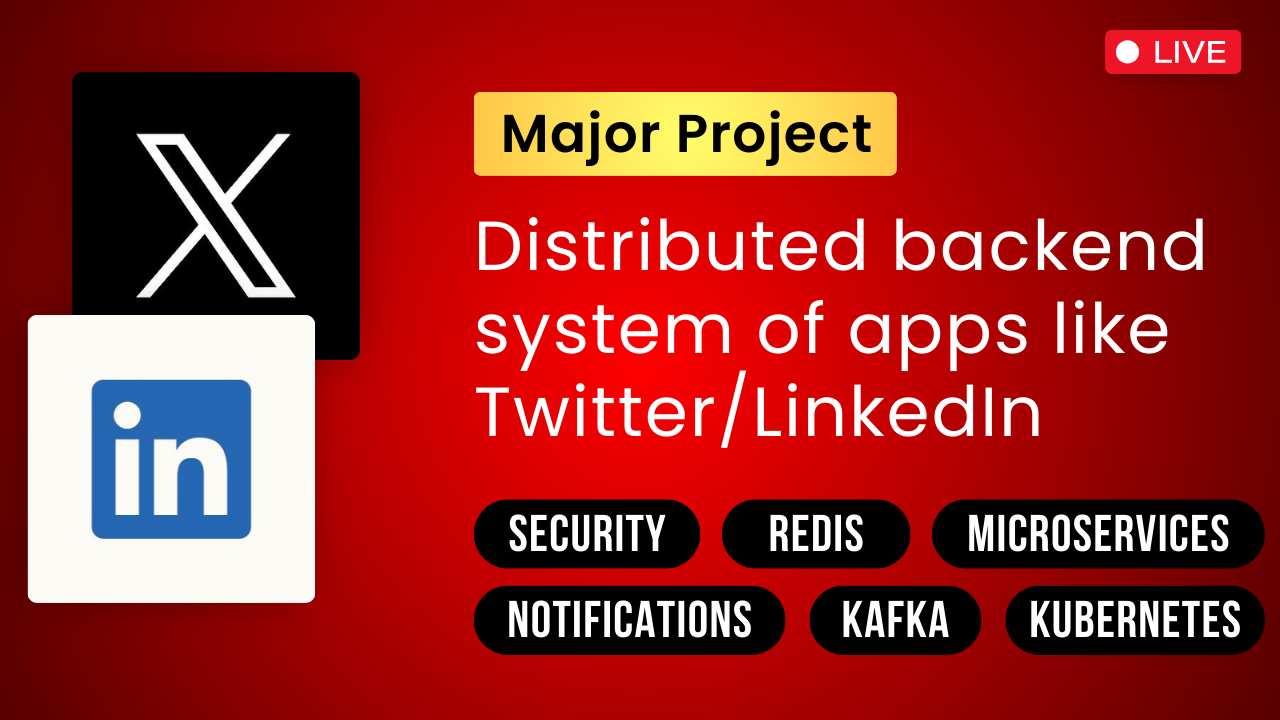
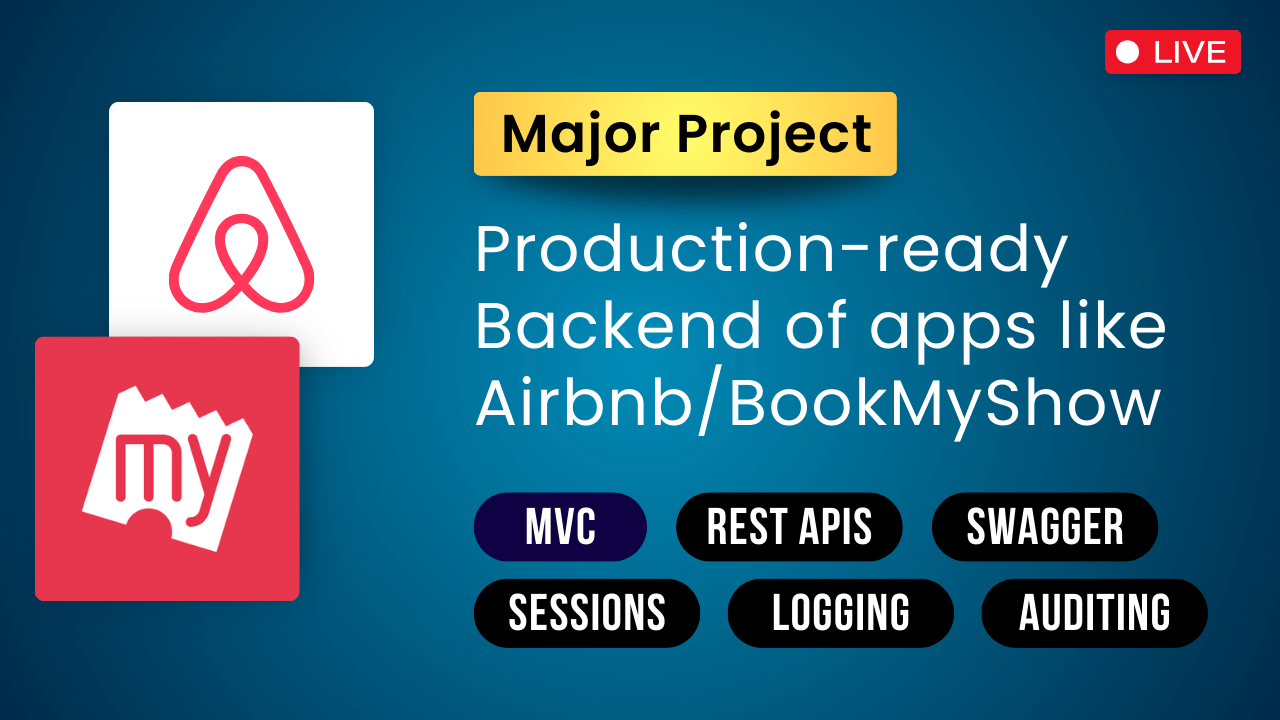
Course content
Cohort Started from 07 April
Introduction To Spring & Spring Boot
1 Week
Explore the core features and fundamentals of the Spring Framework as well as Spring Boot Frameowork
Spring Framework Core Features like Spring IOC Container, Java-based configuration, Annotation-based configuration, Dependency Injection, and more.
Spring Boot Fundamentals and Features - Dive into Spring Boot Internals, Auto Configuration, Spring Initializr, and Starter Projects.
Internal Flow of a Spring Boot Application
Maven Build Tools and Lifecycle goals
Spring Boot MVC and RESTful APIs
1 Week
Dive into Spring MVC and web server configuration, mastering the creation of robust web applications. Learn to integrate Lombok for boilerplate code reduction and build powerful REST APIs.
Spring MVC Concepts - Learn how to create APIs using MVC. Turning your API dreams into reality, one controller at a time!
Lombok - Simplify your Java code with Lombok
Setting up an In-Memory Database with H2 DB
Spring Bean Validation - Ensure your data is always squeaky clean before it even reaches the Service layer
Exception Handling in Spring Boot Application
Spring Boot Data JPA and Database Integration
1 Week
Install and configure essential database tools, then delve into Spring Data JPA fundamentals. Learn to seamlessly integrate Hibernate with MySQL, create custom queries, and explore advanced data mappings through real-world examples.
Install MySQL database server and DBeaver analytics tool
Spring Data JPA Fundamentals like database, drivers, JDBC, ORM, Spring Data JPA Repositories, etc.
Configuring Hibernate ORM with MySQL Database
Build custom queries effortlessly with Spring Data JPA Query Methods
JPQL and Named Query - Master JPQL and Named Queries.
One to One, One to Many, Many to One and Many to Many Mappings with real-world examples
Production ready Spring Boot Features
1 Week
Equip your Spring Boot applications with production-ready features. Add comprehensive documentation, implement metrics and health checks, enhance logging, and integrate third-party APIs seamlessly for a robust and reliable application.
Spring Boot Actuator - Monitor your application's health with Spring Boot Actuator.
Spring Documentation with Swagger and Open API
Spring Dev Tools - Supercharge your development with Spring Dev Tools.
Logging - Keep track of everything with logging.
REST Template and Third-Party APIs - Integrate third-party APIs with ease. Bring the power of the internet to your app!
Authentication & Authorisation with Spring Security
2 Weeks
Secure your REST APIs with Spring Security 6, Spring Boot 3, and SQL Database. Add various types of authentications and implment role-based authorisation.
Create a secure Login and user registration system
Add request filters to secure your REST endpoints
Security against CSRF (Cross-Site Request Forgery) and XSS (Cross-Site Scripting) attacks
Third-party registration system with Google OAuth and JWT authentication
Implement role-based access control with Spring Boot Authorization and SQL Database.
Password Security with Encoding - Secure passwords with encoding. Keep those passwords safe from prying eyes!
Session Management - Manage user sessions effectively. Keep your users logged in and happy!
Spring Boot Junit Testings
1 Week
Embark on your testing journey with JUnit and Mockito. Dive deep into JUnit annotations and assert methods, and master Mockito for effective unit testing.
Get started with testing and JUnit. Test early, test often, and conquer bugs!
Dive deep into JUnit annotations and assert methods.
Master Mockito for unit testing.
Spring Boot Deployment with CI/CD
1 Week
Dive into AWS CodePipeline to construct robust CI/CD pipelines and deploy effortlessly with CodeDeploy on Elastic Beanstalk.
Use Spring Profiles to manage different environments like development, staging and production
Learn to creat buildspec to define the build flow
Create Pipelines for CI/CD using AWS Codepipeline
Deploying with CodePipeline and CodeDeploy on Elastic Beanstalk
Aspect-Oriented Programming
1 Week
Learn to organize your code better with Aspect-Oriented Programming. It helps keep your software neat and flexible by separating different concerns.
Learn the fundamentals of Aspect-Oriented Programming (AOP) and how it improves software modularity.
Explore different advice types (before, after, around) to manage the behavior of your application at specified join points.
Discover the weaving process and how aspects are integrated with the main code during compile time, load time, or runtime.
Caching and Database Transactions
1 Week
Learn about Redis Cache and Database Transactions. Understand how to implement caching with ease using Spring Boot and enhance scalability and performance.
Learn how to integrate Redis for high-performance caching in your Spring Boot applications.
Spring Cache: Understand the Spring Cache abstraction and how to implement caching with ease using Spring Boot.
Distributed Caching: Explore the concepts and implementation of distributed caching to enhance scalability and performance.
Apache Kafka in Spring Boot
1 Week
Explore how to use Kafka with Spring Boot for real-time communication, making your apps smarter and more responsive.
Discover how to leverage Apache Kafka for building robust and scalable messaging systems in Spring Boot.
Learn about the messaging capabilities in Spring Boot and how to integrate them seamlessly with Kafka
Explore the differences and similarities between Kafka and RabbitMQ, and learn how to choose the right messaging solution for your Spring Boot application
Microservices Architecture
2 Weeks
Dive into building microservices with Spring Boot, creating apps that are easier to manage and scale.
Learn how to use Eureka Registry for service discovery in a microservices architecture with Spring Boot.
Explore strategies and best practices for securing your microservices in a Spring Boot environment.
Learn how to use Resilient4J to build resilient microservices with fault tolerance and recovery mechanisms.
Explore centralized logging solutions to aggregate and manage logs from all your microservices.
Master the ELK Stack (Elasticsearch, Logstash, Kibana) for powerful log analysis and visualization in a microservices environment.
Discover how Spring Cloud Sleuth adds tracing capabilities to your microservices, integrating seamlessly with Zipkin.
Docker
1 Week
Learn to package your Spring Boot apps with Docker, making them portable and easy to deploy.
Learn how to set up Docker on your development environment for efficient containerization.
Understand the usage of Docker Compose to manage multi-container applications with ease.
Gain insights into building, managing, and deploying Docker images for consistent application environments.
Kubernetes Deployment for Spring Boot Applications
2 Weeks
Discover how to deploy your Spring Boot apps in Kubernetes, a powerful tool for managing large-scale applications.
Learn the essentials of managing Kubernetes clusters, including setting up, scaling, and maintaining cluster resources effectively.
Dive into the core concepts and functionalities of Kubernetes, such as Pods, Deployments, Services, and more, to orchestrate and manage containerized applications at scale.
Explore the Kubernetes Dashboard, a web-based user interface for managing and monitoring Kubernetes clusters, to visualize and interact with cluster resources efficiently.
Java Executor Framework & Scheduling
1 Week
Learn how to build cron jobs, and add task scheduling functionalities to your Spring Boot applications.
Learn Java Executor Framework to manage asynchronous operations effectively
Build Cron Jobs and Schedulers to manage asynchronous Operations
Add Task Scheduling functionalities to handle background tasks in your Spring Boot Apps
Used by learners at
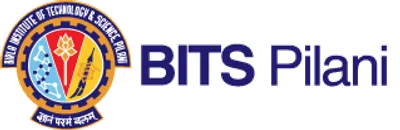

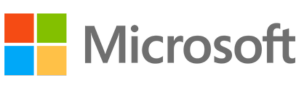

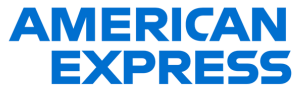
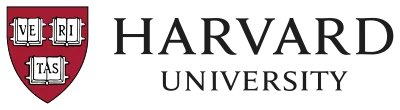
Student Reviews
Om Singh
“ I used to think, that I was good at Spring Boot, but after enrolling into this course I found that my basics were not strong, and I was not even aware of advanced concepts & practices, that were actually used in the industry, which I learnt in this course. ”
Sanjaya Tripathi
CTO at Sarvanam Software
“ The course is pretty awesome. Anuj really knows his stuff and has put together a course that's great for getting you ready for the industry. ”
Aiman Shakeel
Senior Software Engineer at Congnizant
“ Very elaborate course and instructor explained every concept in very simple way. Also the project created in the course is covering every aspect of a production grade application. ”
Vansh Trivedi
“ Each week's lectures were so engaging that, if you're a curious learner, you'll want to finish them all in one go and then eagerly wait for the next week's lessons. ”
Ayush Raje
“ This course not only teaches you Spring Boot but also expose you to industry practices like how to design an application, how to write scalable and optimized code etc. ”
Hindol Roy
SDE at JPMC
“ I tried learning SpringBoot through youtube, initially I found it similar to the course , but now I realise this course actually covers a lot of concepts which are not available on Youtube. ”
Poornima Medar
Software Engineer at Encora Inc
“ The inclusion of weekly assignments helps reinforce learning, and the live projects provide valuable hands-on experience. ”
Parth Thakkar
Software Engineer at NetWeb Software
“ From the beginning, I found the content very useful for anyone looking to develop their skills in Spring Boot. This course is way beyond my expectations. Best course in SpringBoot. 👍😄 ”
Yash Girhe
SDE at JPMC
“ This is a total worth it course. There are many videos available for spring boot on the internet but none of them are created in depth as this. ”
Manoj S
“ Anuj Bhaiya has not only coved most of the topics but has explained it really very well, it does seem to be a sea of topics with a lot of time to digest, investing a good amount of time in the course gives you all the necessary “spring” knowledge you desire. ”
Honey Mehra
“ I have found the explanations of key Spring Boot concepts to be much clearer and more effective compared to other platforms. ”
Sudipta Khotel
“ Best thing is this is a project oriented course where we make good complex backend project to implement the concept and get hands on experience . ”
Santosh Mane
“ A key highlight was the weekly recorded sessions, followed by live classes every Saturday, where we applied the week’s learnings directly to these projects. ”
Akash Panse
“ The structure of the course is the part of attraction for me. The video quality is quit good. The way Anuj bhaiya explain the project is remarkable ✨✨ ”
Avijit Patra
Software Engineer at LTIMindtree
“ What sets this course apart is its unique structure. It combines both self-paced learning for core concepts and live classes for major projects. If there are any concepts you don’t understand, you can request a live class to clarify them by reaching out to the team via Discord. ”
Sakshi Mhetre
“ It is well designed course with such a good platform. Anuj Sir gives the best experience in all live sessions while developing Uber Application Backend project. ”
Lokesh Kumar
Founder Manya Technologies
“ Anuj’s teaching style is awesome. He casts a magical spell on his students so that they truly learn what they are taught. I can say this honestly because I have taken multiple courses of his. ”
Akhil Giri
System Engineer @ TCS
“ Anuj Bhaiya's course seems to excel at blending theory with real-world application, providing a strong foundation in Spring Boot while focusing on industry-relevant knowledge that can be applied in professional settings. ”
Virender Kumar
“ After watching the course curriculum, I bought it because it was very reasonably priced with so much offerings that are hard to find in place. The best thing about this course is that it has doubt support and if any doubt came, it would be solved quickly. ”
Rakhi Yadav
“ I purchased this course by my first stipend. As beginner i want to start back-end Development after learning JAVA programming language. Spring Boot 0 to 100 has in-depth core topics with easy communication. ”
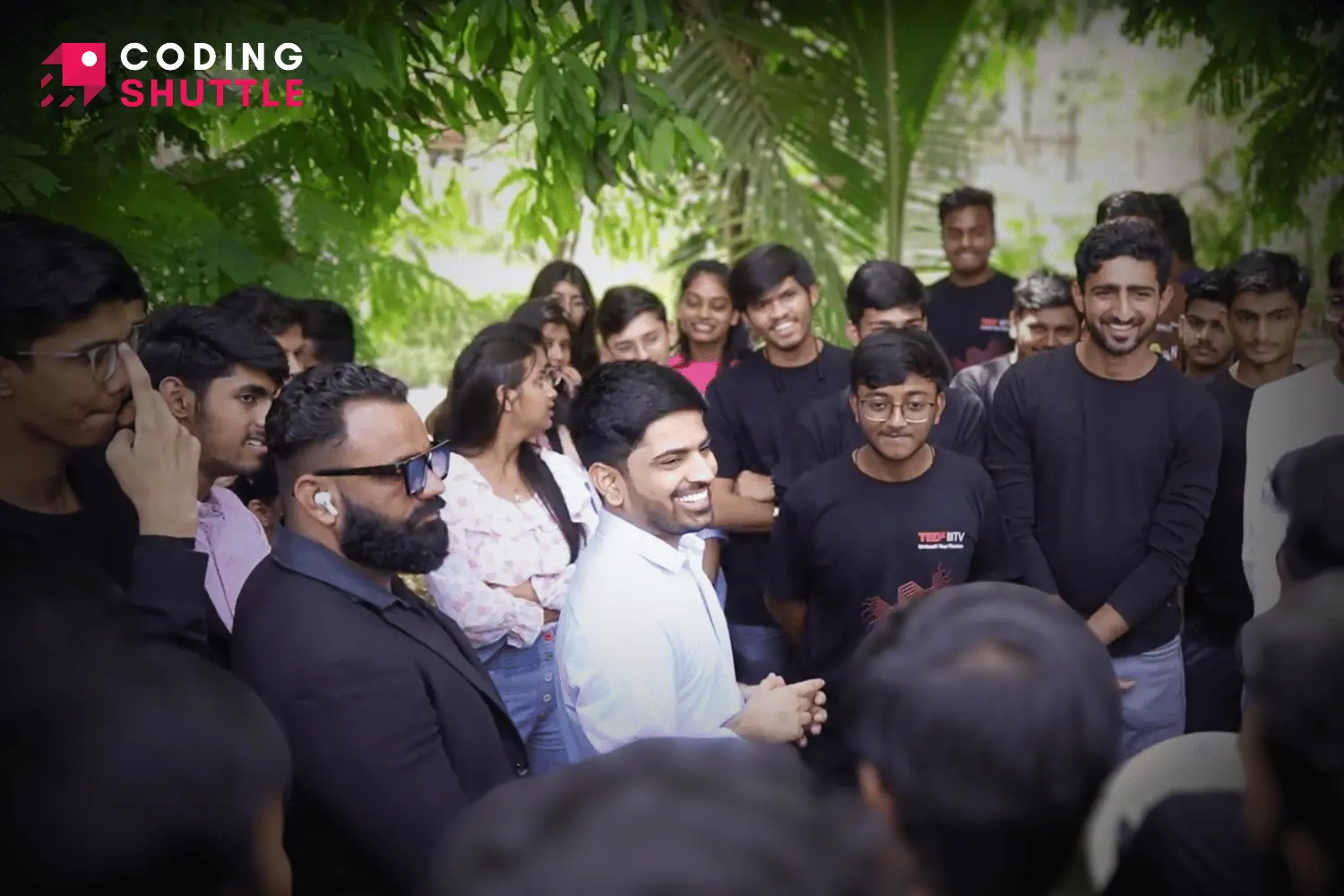
Meet the Instructor - Mr. Anuj Kumar Sharma
Anuj is a Software Engineer and has worked with Top Product based companies like Amazon and Urban Company in the past. He manages his personal YouTube channel named Anuj Bhaiya with a subscriber base of more than 5 Lakh students. After leaving his job at Amazon, Anuj has devoted his life to helping students to learn coding. "Anyone can learn how to code with the right training and support. That's why I created Coding Shuttle to provide comprehensive programs designed to help students at all levels of expertise, from complete beginners to advanced coders looking to enhance their skills." - from Anuj
faqs
Frequently Asked Questions
What is the Spring Boot 0 to 100 Cohort 3.0?
Spring Boot 0 to 100 Cohort 3.0 is a self-paced, all-in-one course designed for graduated and working professionals with basic Java knowledge. It takes you from beginner to expert in Spring Boot, covering core features, security, testing, database integration, deployment, and advanced topics like microservices, Spring Cloud, Kubernetes, and Kafka—with dedicated doubt support.
Who is this Spring Boot course for?
This course is perfect for college graduated students and working individuals with basic Java skills who are looking to land high-paying tech jobs. No prior Spring Boot experience is needed—just a desire to advance your career.
Is this Spring Boot course self-paced?
Yes, Spring Boot 0 to 100 Cohort 3.0 is fully self-paced, allowing busy professionals to learn at their own speed. Access pre-recorded lessons anytime, anywhere, while still getting expert doubt support.
How does doubt support work for working professionals?
Our dedicated doubt resolution platform lets you post questions anytime and get answers from mentors and peers—perfect for professionals balancing work and learning.
Are there hands-on projects in this Spring Boot course?
Yes, you’ll work on practical exercises and 2 real-world projects to build job-ready skills. First Project is a clone of AirBnb Hotel Booking and Management System. Second Project is LinkedIn Microservice System with Kafka, Neo4J Graph DB and Spring Cloud. These projects have impressed many recruiters.
How will this course help me get a job?
This course offers resume building, LinkedIn optimization, resume review webinars, and hackathons—alongside advanced Spring Boot skills—to help you stand out to recruiters and land roles like Backend Developer or Software Engineer.
Why choose this Spring Boot course for my career?
Tailored for job-seeking professionals, this self-paced course delivers in-demand Spring Boot expertise, real-world projects, and career-boosting extras like resume reviews and hackathons—all at your own pace.
Is Spring Boot easy to learn with a Java background?
Yes, with basic Java knowledge, Spring Boot is straightforward to master. Our structured lessons and doubt support make it even easier for professionals to upskill quickly.
Is Spring Boot in demand for jobs in 2025?
Absolutely. Companies like Amazon, Google, Netflix, TCS, Infosys, and startups like Cred and Paytm are hiring Spring Boot developers in 2025—making it a top skill for job seekers.
What salary can I expect as a Spring Boot developer?
In India, Spring Boot developers earn an average of ₹12 lakhs per annum, with a range of ₹5–22 lakhs. Senior roles at top firms can pay over ₹1 crore annually.
How can Spring Boot advance my career?
Mastering Spring Boot can lead to roles like Senior Developer, Solution Architect, or Technical Lead. Our career-focused tools like LinkedIn optimization and resume webinars accelerate your progress.
What job roles can I target with Spring Boot skills?
You can aim for roles like Backend Developer, Full Stack Developer, Software Engineer, or Junior Spring Boot Developer—positions in high demand at MNCs and startups.
What makes this Spring Boot course stand out?
Designed for professionals, this course combines self-paced learning, advanced topics like microservices, hands-on projects, and career support—resume building, LinkedIn optimization, and hackathons—to get you job-ready.
How successful are Coding Shuttle alumni?
Over 6,000 alumni are thriving at top firms, with 200+ in FAANG and around 60% of students are in Big Tech MNCs, our training delivers career success.
Who teaches this Spring Boot course?
The course is taught by Mr. Anuj Kumar Sharma, a Java expert with experience at Amazon and Urban Company, ensuring you learn industry-relevant skills.
How is the course content delivered?
All lessons are pre-recorded for self-paced access, with 2 years of updates included. We have also included additional Projects building LIVE classes recordings from previous batch in this course. Learn whenever it fits your schedule, and use our doubt support platform for help.
Can I pay for this course in EMIs?
Yes, we offer flexible EMI options, including no-cost EMIs on major credit and debit cards, making it affordable for working professionals to invest in their future.
What career support does this course offer?
You’ll get resume building sessions, LinkedIn optimization guidance, resume review webinars, and hackathons—tools to help you land your dream tech job.
Will I receive a certificate after completing this course?
Yes, you’ll earn a certificate from Coding Shuttle upon successful course completion, showcasing your Spring Boot skills to employers and enhancing your job prospects.
How soon can I start upskilling with this course?
Start immediately after enrolling! With instant access to pre-recorded lessons, you can begin mastering Spring Boot and preparing for your next job right away.
Official and Verified Certificate of Completion
Add the certificate to your CV or your resume or post it directly on Linkedin to enhance your professional credibility.
Enroll Now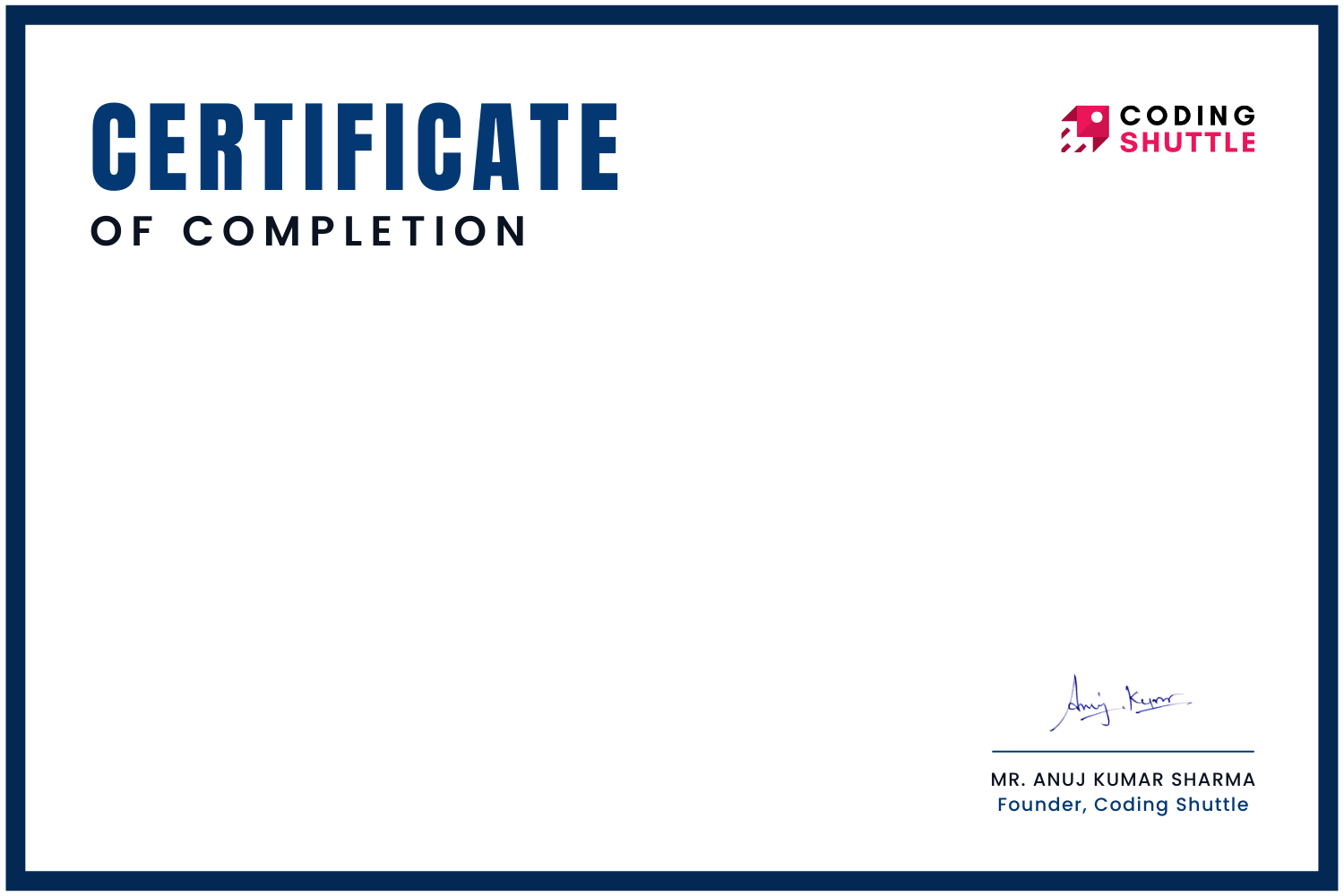
This is to certify that
Your NameHas completed the Spring Boot 0 to 100 Cohort 3.0 [NEW] from Coding Shuttle
Date of Issuance : DD/MM/YYYY
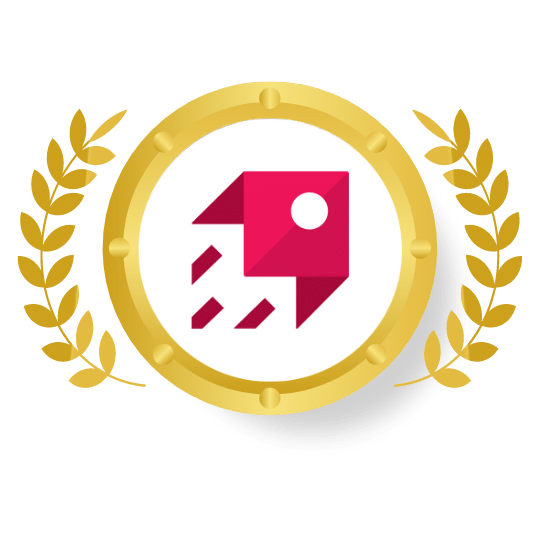
SCAN & ENROLL NOW