React 19 Course 1 To 100 - From Developer To Expert
Learn what no one is teaching — Master advanced React patterns, Performance Optimization, and React Server Components and set youself apart from the competition.
What you'll learn
Unlock the inner workings of React with Babel and Fiber.
Master state management with Context API and Portals.
Boost performance with Code Splitting and Lazy Loading.
Leverage Composition and Compound Components for flexibility.
Explore Async Components and Client-Server integration.
Streamline CI/CD with AWS CodePipeline and S3.
Discover Memoization and useMemo for optimized re-renders.
Understand Race Conditions and how to avoid them.
Master the latest Ref for flexible component management.
Optimize rendering with React Best Practices.
Dive into Windowing to handle large datasets efficiently.
Implement Layout computation for smoother UI rendering.
Explore the power of Bundlers and Babel for production.
Understand the Reconciliation Algorithm for efficient updates.
Optimize React performance with Concurrent Rendering.
Use Props Collection to simplify component APIs.
Get hands-on with Imperative Handles for direct DOM manipulation.
Dive into Slots for creating flexible component structures.
Explore the benefits of Static Exports for optimized sites.
Learn how to use AWS CodeBuild for efficient builds.
Master Pending UI to keep users engaged during fetches.
Explore how Portals allow React to render outside the DOM.
Implement Lazy Loading to improve app load times.
Use State Reducers to manage state transitions effectively.
Get familiar with NodeJS Loader for dynamic module loading.
Learn about Streaming for faster content delivery.
Use Memoization to optimize repeated function calls.
Explore how CloudFront CDN enhances performance.
Understand Code Splitting to reduce initial load time.
Dive into Server Components for more efficient rendering.
Explore Compound Components for complex component structures.
Use State Initializers for smarter state management.
Implement Focus management for seamless user navigation.
Get familiar with AWS S3 for scalable storage solutions.
Understand the concept of Reconciliation for faster updates.
Learn how to handle large datasets efficiently with Windowing.
Master CodePipeline for automated CI/CD workflows.
Leverage Async Components for smoother user interactions.
This course includes:
1:1 Doubts support
LIVE classes every Sunday at 9 PM
40+ hours on-demand video
40+ downloadable resource
1 real-world project
Doubts solving classes
New assignments every week
Certificate of completion
What you'll build
Learn by building. In this course, you will be working on multiple micro-skilling exercises as well as major projects like these:
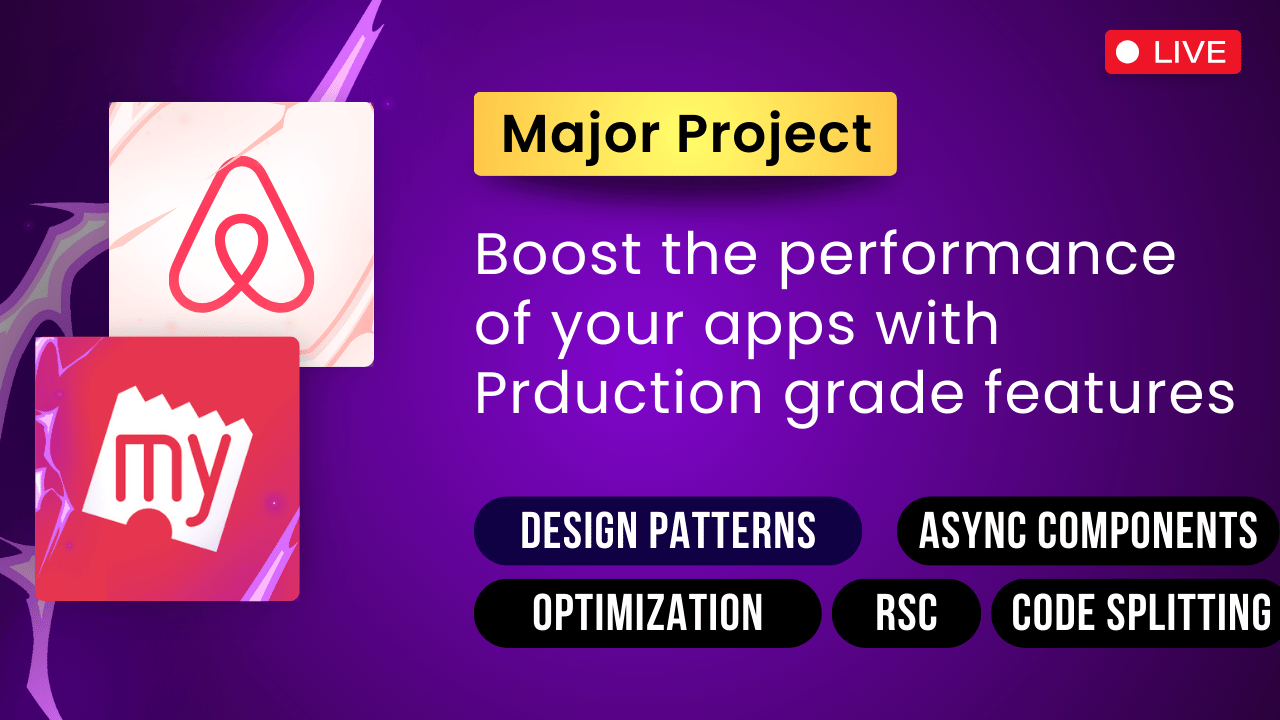
Course content
Cohort Starts from 15 March
Internal working of React
1 Week
Unlock the inner workings of React, from Babel and Fiber to advanced rendering techniques, with performance-boosting strategies for modern React applications.
Dive into React's Reconciliation for efficient UI updates.
Uncover the secrets of React Rendering with React Fiber and performance boosts.
Understand how compilers translate code into machine-ready language.
Discover how bundlers and Babel optimize your code for production.
Peek behind React’s internal workings for performance mastery.
Advanced React 19 APIs
1 Week
Master React state management, optimization techniques, and advanced tools like Context API, Portals, useMemo, and Imperative Handles for seamless and performant UI experiences.
Explore powerful State management techniques in React using Context API and Redux
Uncover strategies for smart State Optimization.
Learn how Portals bring React outside the DOM tree.
Master the Memoization concept to avoid unnecessary re-renders with useMemo
Understand Layout computation for smooth UI rendering.
React Performance and Optimisation
1 Week
Boost React performance with best practices, Context optimization, Code Splitting, Concurrent Rendering, Lazy Loading, and efficient rendering strategies for faster, scalable apps.
Explore React Best Practices for cleaner, faster code.
Uncover the benefits of Code Splitting to boost performance and smooth rendering
Discover Windowing to handle large data sets efficiently.
Master Lazy Loading for faster app load times.
React Design Patterns
1 Week
Master the art of Thinking in React by leveraging Composition, Compound Components, and advanced patterns like Props Collection, State Initializers, and Reducers for flexible, maintainable design.
Explore the mindset of Thinking in React for better app design.
Master Composition and Compound Components to build reusable, flexible components.
Dive into Slots and latest Ref for flexible component structures.
Discover State Initializers for smarter state management.
Utilize State Reducers for more controlled state transitions.
React 19 Server Components
1 Week
Unlock the power of Async Components, Streaming, and Client-Server integration while mastering dynamic loading, Pending UI handling, and preventing race conditions for seamless user experiences.
Explore Async Components for smoother user interactions.
Uncover the Client and Server Components.
Learn how the NodeJS Loader handles dynamic module loading.
Master Pending UI to keep users engaged during data fetches.
Avoid Race Conditions with smart async handling techniques.
React CICD
1 Week
Streamline your production workflow with essential tools like AWS CodePipeline, CodeBuild, S3, and CloudFront for optimized deployment and flawless performance.
Master Static Exports for optimized, static site generation.
Dive into AWS CodePipeline and CodeDeploy for seamless CI/CD automation.
Learn how to leverage AWS S3 for secure, scalable storage for static exports
Boost performance with CloudFront CDN for faster content delivery.
Used by learners at
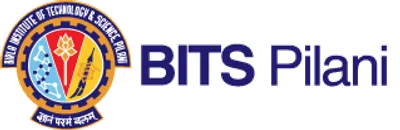

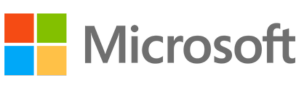

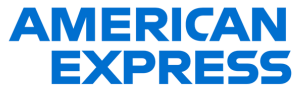
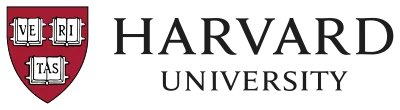
Student Reviews
Sanjaya Tripathi
CTO at Sarvanam Software
“The course is pretty awesome. Anuj really knows his stuff and has put together a course that's great for getting you ready for the industry. ”
Akash Panse
“The Course is totally worth it because the course is so indepth that anyone can understand the concepts easily. The language used in the course is too easy to understand. The structure of the course is the part of attraction for me.”
Jyoti Bharti
Software Developer at Johnson Controls
“I particularly appreciate the concept of project-based learning, which allows me to engage deeply with the material by applying it to projects I am passionate about. Additionally, the weekly homework assignments are an excellent component of the program.”
Gautam Batra
“It is a really nice course, as always it was very well taught by Anuj bhaiya.”
Shlok Singh
“This course is totally worthy, it prepares you at industry level. I would like to suggest all serious motivated students to pursue this course.”
Rushikesh Chavan
System Engineer @ TCS
“One of the best courses for developers, students, and working professionals. The course is very well structured and completes every concept required to work with any IT organization.”
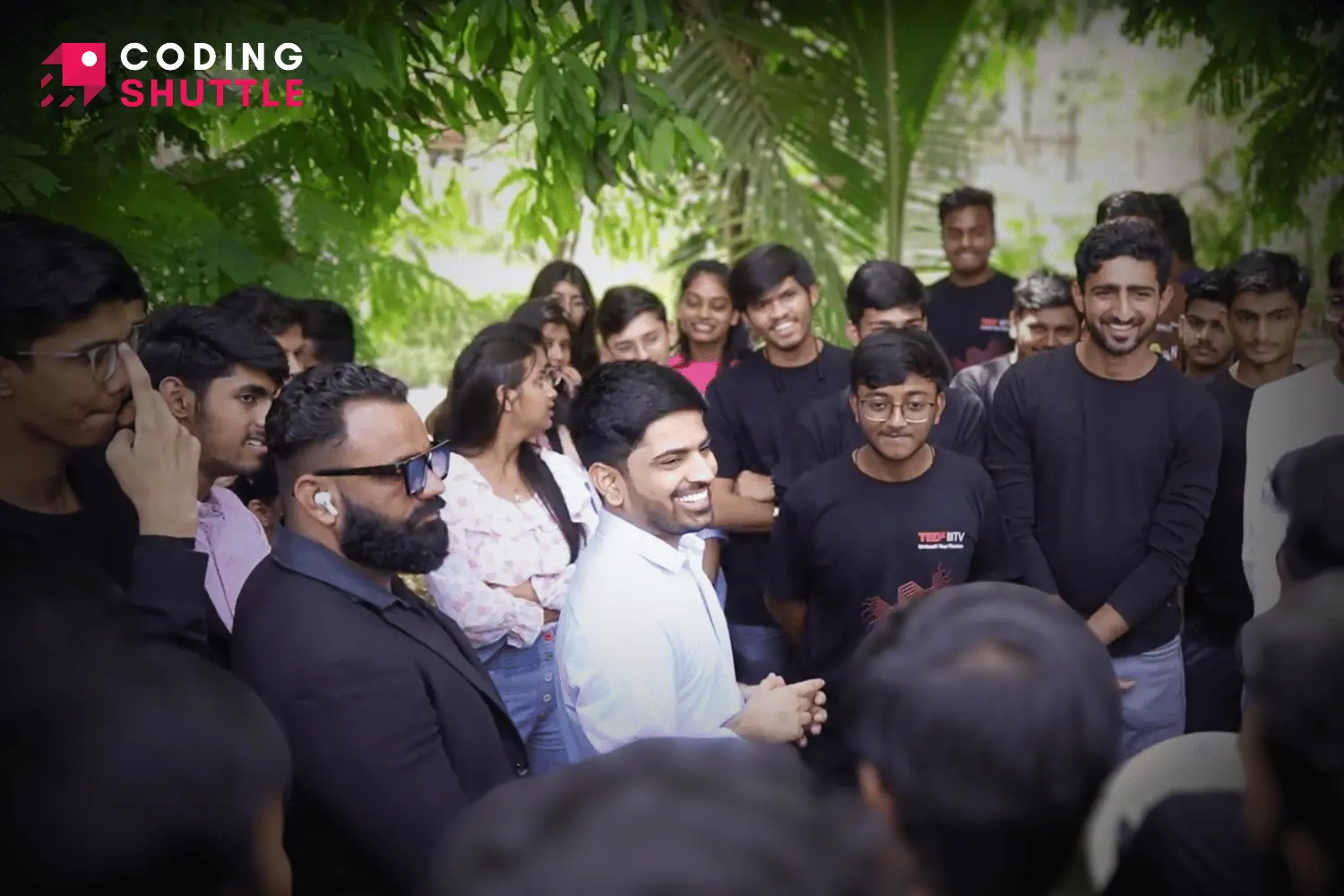
Meet the Instructor - Mr. Anuj Kumar Sharma
Anuj is a Software Engineer and has worked with Top Product based companies like Amazon and Urban Company in the past. He manages his personal YouTube channel named Anuj Bhaiya with a subscriber base of more than 5 Lakh students. After leaving his job at Amazon, Anuj has devoted his life to helping students to learn coding. "Anyone can learn how to code with the right training and support. That's why I created Coding Shuttle to provide comprehensive programs designed to help students at all levels of expertise, from complete beginners to advanced coders looking to enhance their skills." - from Anuj
faqs
Frequently Asked Questions
What is the React 19 Course 1 to 100?
The React 19 Course 1 to 100 is an advanced course for developers who already have experience with React and want to gain in-depth knowledge. It covers advanced topics such as React Server Components, advanced React hooks, design patterns, and optimization techniques to help you build scalable and high-performance React applications.
What prerequisites are needed for this course?
This is an advanced course in React 19. A fundamental understanding of React JS is required to follow this course.
What is the React 19 Course 0 to 1?
The React 19 Course 0 to 1 is a beginner-friendly course designed to introduce you to React 19 web development. It's an ideal course for newcomers eager to get started with React and build a strong foundation.
What is the React 19 Course 0 to 100?
The React 19 Course 0 to 100 combines both the 0 to 1 and 1 to 100 courses. It covers everything from fundamental React concepts such as state, props, hooks, and Redux, to advanced topics like React design patterns and optimization techniques. This course is perfect for both beginners and professionals looking to master React and accelerate their careers.
Will I get homework or assignments to practice?
Yes, throughout the course, you will be assigned practical exercises and a major real-world project. These activities will help reinforce your learning and provide hands-on experience with the concepts covered.
How will my doubts be resolved?
You will have access to a dedicated support system where you can ask questions, seek clarification, and interact with both mentors and fellow learners throughout the course.
Do I really need to learn advanced React concepts if I already know the basics?
Many courses cover basic React concepts, but companies are looking for experts who understand React inside and out. This course goes beyond the basics, teaching advanced concepts that are crucial for building scalable React applications and preparing you for real-world challenges. This makes you stand out in a crowd where not many people know abou the advanced concepts, thus improve your chances of gettign shortlisted.
How is this course different from other React courses?
This course stands out in both its design and philosophy. It covers all the essential concepts for becoming a proficient React developer, offering a comprehensive curriculum and robust support system. Additionally, this course goes beyond just teaching React concepts – it focuses on building strong intuition for React and developing major projects, which is far more valuable than simply skimming through the concepts.
How well are Coding Shuttle alumni doing?
Over 5,000 Coding Shuttle alumni from various colleges and companies are now working at leading tech firms. At last count, more than 100 Coding Shuttle alumni are employed at FAANG companies, and over 60% are working at major tech MNCs and unicorn startups.
In which language is the course taught?
The course is conducted entirely in English and will be taught by Mr. Anuj Kumar Sharma, a renowned Java and React expert with experience working at major tech companies such as Amazon and Urban Company.
How do the classes take place?
Classes are delivered in both pre-recorded and LIVE formats. You can access recorded lessons anytime and anywhere, while LIVE sessions are conducted every Sunday at 9 PM.
Can I get the full recordings after purchasing the course?
This is a cohort-based course where all students in a batch progress at the same pace. You will not receive the full recordings immediately upon purchase. New content is unlocked each week, and we also host weekly LIVE project sessions and doubt-solving sessions.
Can I pay using EMIs?
Yes, you can pay using EMIs. We also offer no-cost EMI options on major credit cards as well as select debit cards.
What if I miss any of the LIVE classes?
Don’t worry! Recordings of all LIVE sessions will be shared on the platform within 24 hours of the class ending, so you can catch up anytime.
How many projects are covered in this course?
The course includes one major project build using the industry standards and multiple mini-projects. The major project will start from day one, with new content being unlocked weekly. At the end of each week, we will use the newly learned concepts in that week to build the major project in the LIVE sessions, fostering a comprehensive and flexible learning environment.
I don't have any experience with React. Is this course for me?
If you do not have any experience in React, you might find this course hard to follow. We recommend you opt for the React 19 Course 0 to 1, which is designed for absolute beginners in React JS.
Official and Verified Certificate of Completion
Add the certificate to your CV or your resume or post it directly on Linkedin to enhance your professional credibility.
Enroll Now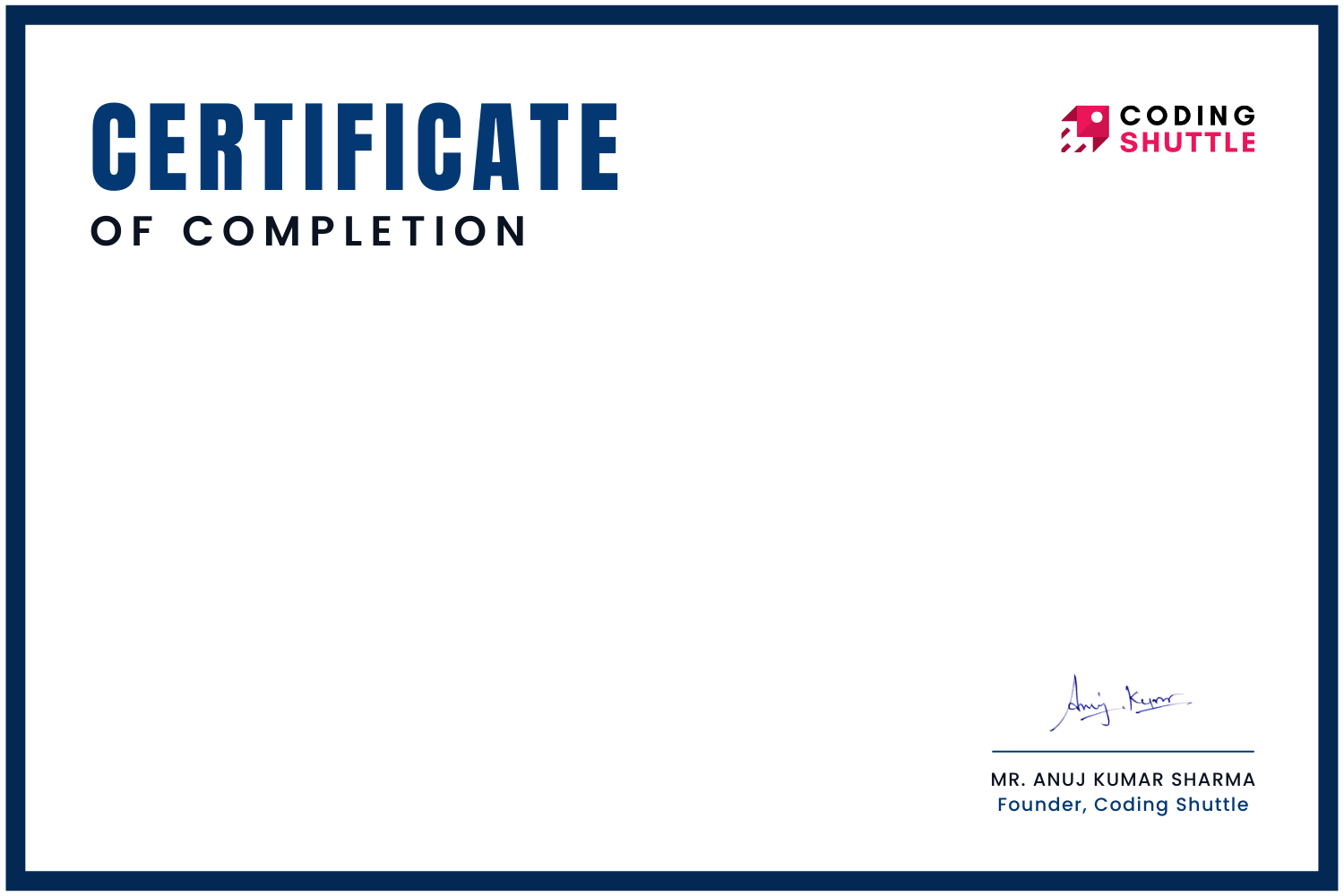
This is to certify that
Your NameHas completed the React 19 Course 1 To 100 from Coding Shuttle
Date of Issuance : DD/MM/YYYY
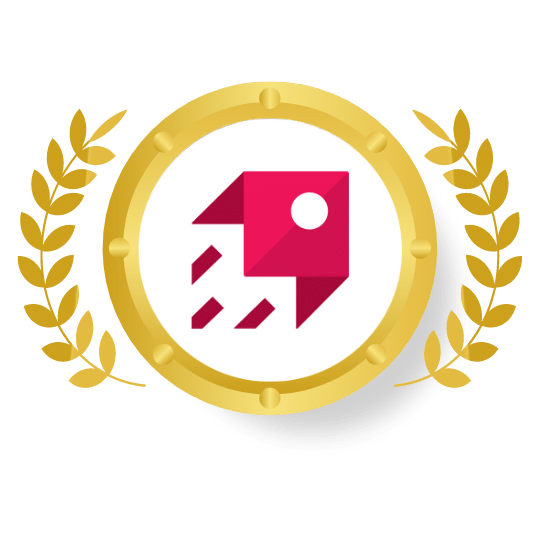
SCAN & ENROLL NOW