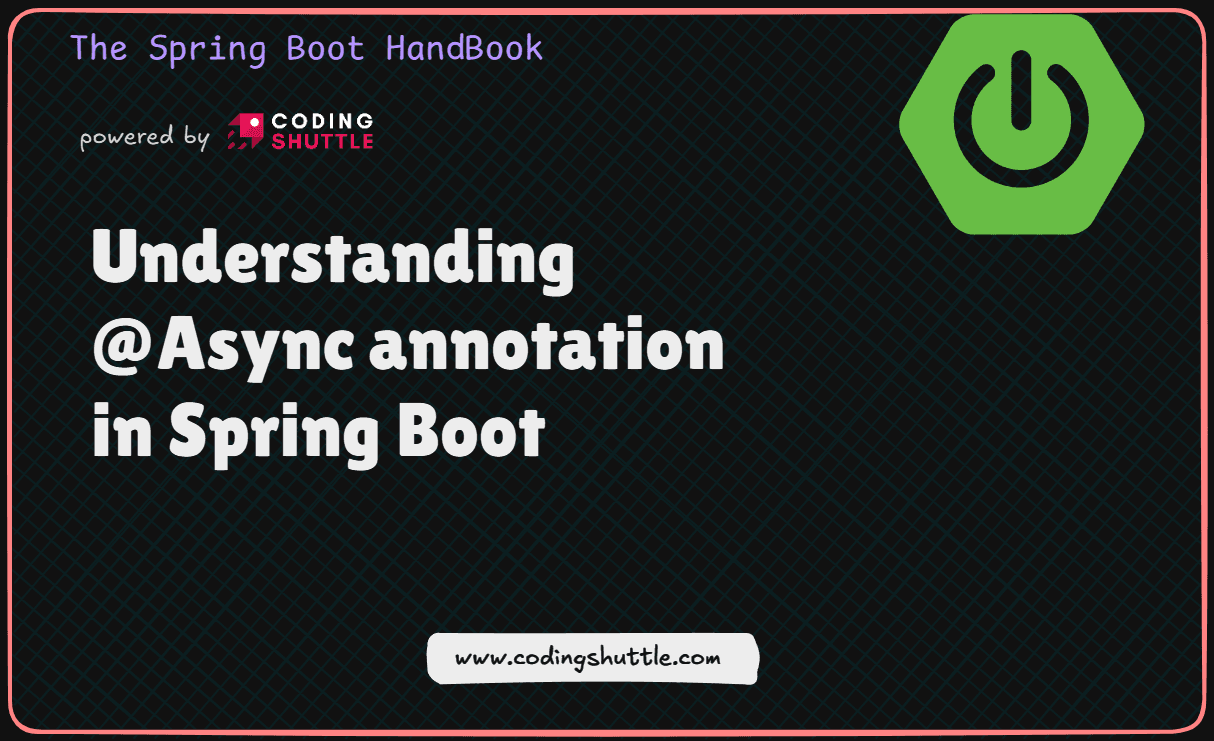
Understanding The @async Annotation In Spring Boot
In this blog, I'll provide a simple guide to using the @Async annotation in Spring Boot, helping you improve application performance by running tasks asynchronously. I will also cover setup, usage, and best practices for efficient thread management and error handling.
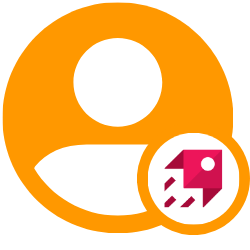
Santosh Mane
January 31, 2025
7 min read
Introduction#
If you’re diving into web development, you’ve probably heard that speed and responsiveness are key, right? That’s where asynchronous programming comes into play. It’s one of those tools you didn’t know you needed until you do. If you’re using Spring Boot, you’ve probably bumped into the @Async
annotation at some point, but what does it actually do, and why should you care? Let’s break it down in a way that actually makes sense, without all the jargon.
So, What’s @Async
All About?#
@Async
is like telling Spring, “Hey, this task? Let it run in the background while I get on with other stuff.” When you throw @Async
on a method, Spring takes that method and hands it off to a different thread, leaving your main thread free to keep doing its thing. It’s like delegating a long-running task to someone else so you don’t have to sit there twiddling your thumbs waiting for it to finish. So, whether it’s pulling data from an API or doing some heavy lifting in your code, @Async
helps keep things moving without holding up the rest of your app.
How Does It Actually Work?#
So, how does Spring make all this magic happen? Well, it uses something called a task executor. This is just a fancy way of saying it manages a pool of threads to handle asynchronous methods. When you mark a method with @Async
, Spring grabs an available thread from this pool and runs the method on it.
If you don’t specify a custom executor, Spring will use a default one. But, if you’ve got more specific needs (like controlling the number of threads or tweaking how tasks are handled), you can easily create a custom executor. It gives you a lot of flexibility to fine-tune how your app runs.
Steps to Use the @Async
Annotation#
1. Enable Asynchronous Processing#
Before you can start using the @Async
annotation, you need to enable asynchronous processing in your Spring Boot application. You can do this by adding the @EnableAsync
annotation to one of your configuration classes.
The @EnableAsync
annotation tells Spring to look for methods annotated with @Async
and handle them asynchronously.
2. Annotate Your Method#
Once asynchronous processing is enabled, you can annotate any method you want to run asynchronously with @Async
.
In this case, the processData
method will run in a separate thread, allowing the main thread to continue executing other code.
3. Return Type Consideration#
The return type of an asynchronous method is important. The method can either return void
, Future
, or CompletableFuture
. If you need to get a result from the asynchronous task, you’ll want to use Future
or CompletableFuture
.
Here’s an example using CompletableFuture
:
With CompletableFuture
, you can even chain multiple asynchronous tasks together or apply transformations to the result once the task completes.
4. Calling an Asynchronous Method#
When calling an asynchronous method, it behaves just like any other method, but the difference is that it runs in the background. Here’s how you would call the fetchData()
method from another component:
Handling Exceptions#
One of the challenges with asynchronous methods is handling exceptions. Since asynchronous methods run in a separate thread, exceptions thrown inside those methods won’t be caught in the usual try-catch blocks. To deal with this, you can use the @Async
method’s Future
or CompletableFuture
to handle exceptions.
For example, you could use CompletableFuture.exceptionally()
to manage errors:
Customizing the Task Executor#
While Spring Boot provides a default task executor, you may need more control over the execution of your asynchronous methods. You can define a custom task executor by adding a bean to your configuration:
This custom executor will control how many threads Spring uses to handle asynchronous tasks, ensuring you don’t overload your system.
Things to Keep in Mind#
- Thread Management: Make sure the thread pool size is configured properly. Too many threads can cause resource exhaustion, while too few can lead to tasks queuing up.
- Asynchronous Method Visibility: Only methods in Spring-managed beans can be asynchronous. If you try to mark a method in a non-Spring bean with
@Async
, it won’t work. - Non-blocking Operations:
@Async
is ideal for non-blocking operations. If the task involves waiting on I/O or external services, asynchronous processing can make a big difference in performance.
Example with Output:#
Let’s say you have a service that fetches user data asynchronously. We’ll mock up an example with @Async
and CompletableFuture
to simulate the process of fetching user data and processing something else in the meantime.
Service Class (MyService.java
)#
Controller or Another Class Where You Call the Service (MyController.java
)#
Breakdown of What Happens:#
- When you hit the
/process
endpoint, theprocess()
method inMyController
is triggered. - It starts the
fetchData()
method asynchronously. The method begins to run on a separate thread, simulating a 2-second delay (like waiting for an API response). - While the async task is running, the main thread continues doing other work. In this case, it prints
"Doing other work..."
5 times, with a 0.5-second delay between each print. - After the async task finishes (after 2 seconds), we call
result.join()
to get the result from theCompletableFuture
. Once that’s done, the final message is returned.
Output:#
When you hit the /process
endpoint, the output will look something like this in the console:
Here’s what happens step-by-step:
- The
"Doing other work..."
messages are printed while the async task is running in the background. - After the background task finishes, you get the
"Finished processing. User data fetched successfully!"
message returned to the user.
Conclusion#
The @Async
annotation in Spring Boot is a powerful tool for making your application more responsive by executing tasks asynchronously. By offloading time-consuming tasks to background threads, you improve the overall user experience and system efficiency. Whether you're fetching data from a remote server or processing complex calculations, asynchronous methods can significantly boost performance. Just be mindful of thread management and exception handling to ensure everything runs smoothly.