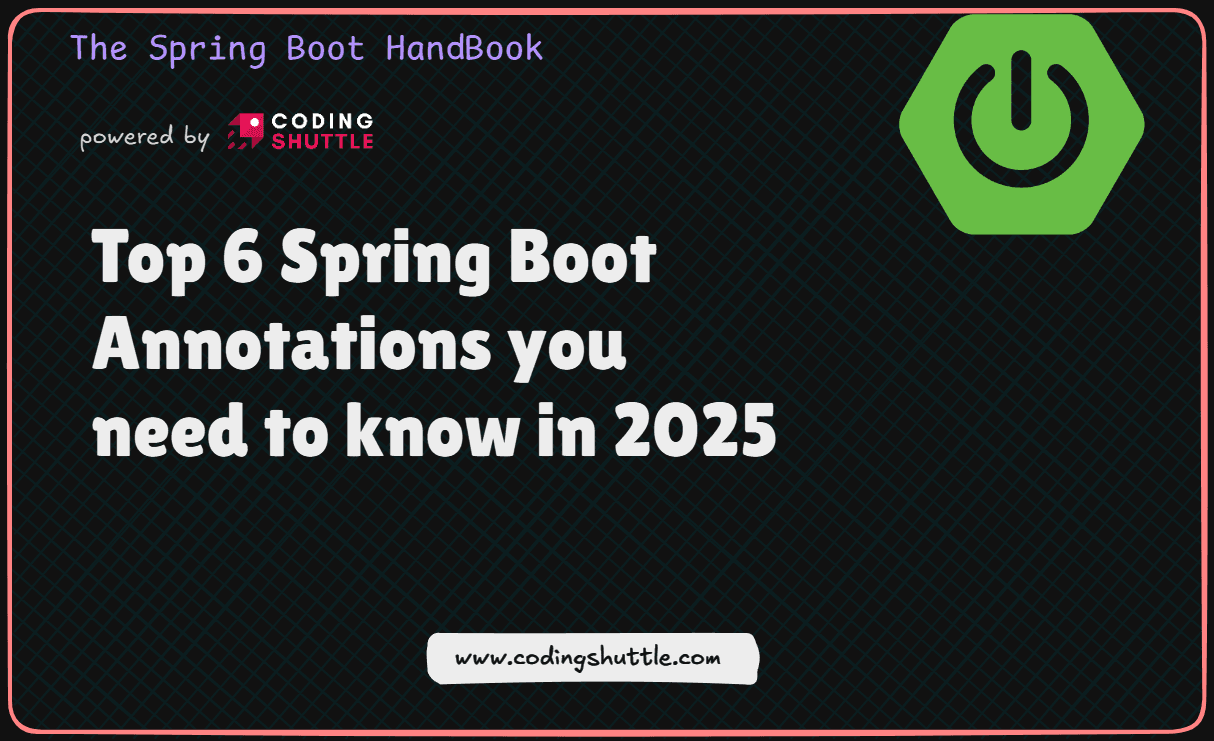
Top 6 Spring Boot Annotations Every Spring Boot Developer Need to Know in 2025
In this blog I have listed six essential Spring Boot annotations every developer should know in 2025. It covers key annotations like @SpringBootApplication, @RestController, and @Async and many more, helping you streamline development and improve application efficiency.
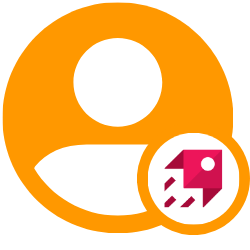
Santosh Mane
January 31, 2025
5 min read
Introduction#
What makes Spring Boot such a favorite among developers? For me, it’s how it simplifies the whole process. You don’t have to wrestle with a ton of configuration or waste time on repetitive tasks it just works, and it lets you focus on the actual coding. But honestly, what really stands out are the annotations. They save so much time by cutting out unnecessary boilerplate code and letting you zero in on what matters most.
In this blog, I’ll share six Spring Boot annotations that I think are absolute must-knows as we head into 2025. Let’s get started!
1. @SpringBootApplication#
What It Does:#
The @SpringBootApplication
annotation is the backbone of any Spring Boot application. It's essentially a combination of three crucial annotations: @Configuration
, @EnableAutoConfiguration
, and @ComponentScan
.
- @Configuration tells Spring that this class contains bean definitions.
- @EnableAutoConfiguration enables Spring Boot's auto-configuration magic, automatically setting up the beans based on the project's dependencies.
- @ComponentScan makes Spring Boot automatically detect and register spring-managed beans in the classpath. (@Component, @RestController, @Service, @Repository).
Why You Need It:#
You only need this one annotation at the entry point of your application (usually in the main
class), and Spring Boot will handle the rest. It significantly reduces the need for configuration and makes your life easier.
2. @RestController#
What It Does:#
The @RestController
annotation is used to define a controller in a Spring Boot application that handles HTTP requests and sends back HTTP responses. It's a convenience annotation that combines @Controller
and @ResponseBody
, meaning you don't need to annotate each method with @ResponseBody
individually.
Why You Need It:#
If you’re building a RESTful web service, @RestController
is a must-have. It simplifies your code by eliminating the need to return views. Instead, it automatically serializes the response body into JSON or XML, making it ideal for APIs.
3. @Autowired#
What It Does:#
The @Autowired
annotation is used for dependency injection in Spring Boot. It tells Spring to automatically inject the required bean into your class at runtime.
Why You Need It:#
Instead of manually creating instances of your dependencies, @Autowired
lets Spring handle it for you. This is especially useful in large applications where managing dependencies manually would be tedious.
In the example above, @Autowired
tells Spring to inject an instance of MyRepository
into MyService
at runtime.
4. @Value#
What It Does:#
The @Value
annotation is used to inject values into Spring beans from external sources like application.properties
or application.yml
.
Why You Need It:#
This annotation is perfect for configuring your application with properties without hardcoding values into your code. It makes your app more flexible and easier to configure for different environments (development, production, etc.).
In the example above, Spring Boot will inject the value of spring.application.name
from the application.properties
file into the appName
field.
5. @Async#
What It Does:#
The @Async
annotation is used to run methods asynchronously in Spring Boot. When you mark a method with @Async
, Spring will execute it in a separate thread, allowing the main thread to continue executing without waiting for the method to complete.
Why You Need It:#
Applications are expected to handle a growing number of concurrent tasks. By using @Async
, you can keep your app responsive and efficient, especially when dealing with time-consuming tasks like I/O operations or external API calls.
This method will run in the background, letting the main thread keep doing other tasks.
6. @Entity#
What It Does:#
The @Entity
annotation marks a class as an entity bean that should be mapped to a database table. It’s part of JPA (Java Persistence API) and tells Spring Boot that this class should be managed by the persistence context.
Why You Need It:#
If you’re working with a relational database, @Entity
is essential for defining the structure of your database tables and letting Spring Boot automatically handle CRUD (Create, Read, Update, Delete) operations through repositories.
In the example above, @Entity
maps the User
class to a database table, and Spring Boot will automatically manage it using JPA repositories.
Conclusion#
Spring Boot has a bunch of annotations that can seriously simplify your coding life. Once you start using them, you’ll notice how much easier it is to get things done without worrying about writing a ton of repetitive code. As web applications keep getting more demanding and complex, knowing how and when to use these annotations can make a big difference in how efficient and scalable your projects are.
So, to wrap it up, the six annotations we talked about—@SpringBootApplication
, @RestController
, @Autowired
, @Value
, @Async
, and @Entity
are tools you’ll find yourself reaching for almost every day. Get comfortable with them, and you’ll be better equipped to build robust, modern Spring Boot applications that stand the test of time.