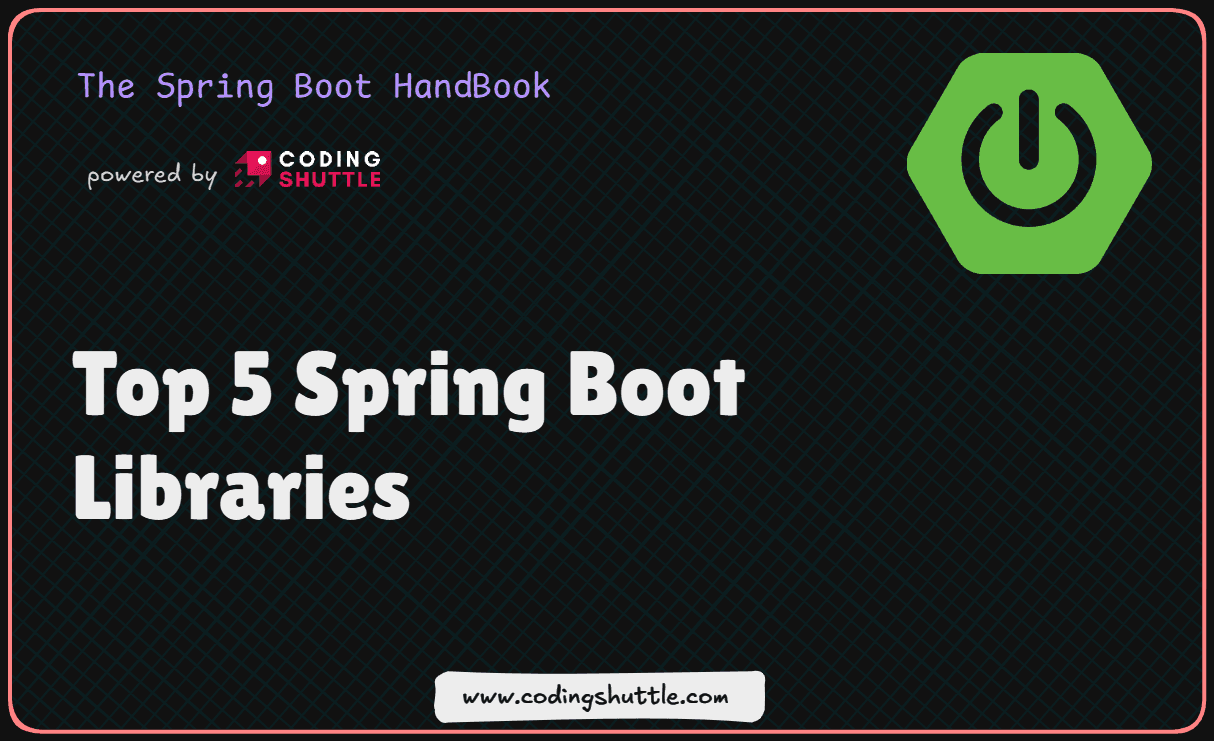
Top 5 Spring Boot Libraries You Need to Know About
This article covers five essential Spring Boot libraries: Starter Web, Data JPA, Security, Lombok, and Validation. It explains their features, benefits, and integration examples for simplified development and enhanced functionality.
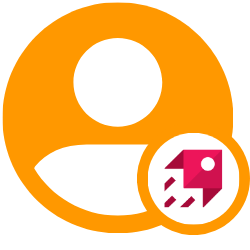
Shreya Adak
December 07, 2024
5 min read
Introduction#
A library is a collection of pre-written code that provides reusable functionality for your project.
It is a type of dependency, but not all dependencies are libraries. Libraries are specific reusable code collections included as part of a dependency. When you add a dependency, it often includes multiple libraries bundled together.
- Libraries focus on specific features or functionality, like handling files, making HTTP requests, or processing data.
- Usually, you directly invoke functions or classes provided by the library in your code.
- Dependency: A toolbox containing various tools (some may be libraries, others might be a power drill or a measurement tool).
- Library: A screwdriver inside the toolbox, designed for a specific job.
Here are the top 5 Spring Boot libraries you should be familiar with to enhance your development experience:
1. Spring Boot Starter Web#
- Purpose: The
spring-boot-starter-web
dependency simplifies web application development by providing essential tools and libraries for building web-based or API-driven applications. It automatically configures everything necessary to develop and deploy web applications with Spring Boot. - Key Features:
- Supports REST API development: Facilitates the creation of RESTful web services.
- Built-in HTTP endpoint support: Automatically configures Spring MVC for handling HTTP requests.
- Embedded server configuration: Includes Tomcat (or other servers) for running the app without external setup.
- Automatic JSON handling: Uses Jackson for easy JSON serialization and deserialization.
- Template engine support: Integrates Thymeleaf for rendering dynamic HTML.
- Importance: Core for building web-based or API applications, reducing setup time and complexity, and enabling quick deployment with embedded servers.
- Adding the Dependency (
pom.xml
for Maven):
- Building a Simple REST API: Here's a basic example of a Spring Boot REST controller using
spring-boot-starter-web
.
- When you run the application, it will start an embedded Tomcat server on port 8080 by default.
- You can access the API by visiting
http://localhost:8080/hello
in your browser or making a GET request.
2. Spring Data JPA#
- Purpose: The primary purpose of Spring Data JPA is to simplify database interaction by utilizing JPA (Java Persistence API). It abstracts the complexities of database access, allowing developers to interact with relational databases in an object-oriented way without the need to write complex SQL queries or boilerplate code.
- Key Features:
- CRUD Repository Support: Provides a set of predefined methods for common database operations (e.g.,
save()
,findById()
,delete()
) through theCrudRepository
interface. - Eliminates Boilerplate Code: Reduces the need for writing repetitive SQL or JDBC code by using JPA annotations and repository methods.
- Seamless Integration with Databases: Works effortlessly with popular relational databases (e.g., MySQL, PostgreSQL, H2) via JPA implementations like Hibernate.
- CRUD Repository Support: Provides a set of predefined methods for common database operations (e.g.,
- Importance: It's the go-to library for interacting with relational databases in Spring Boot. It abstracts database access, promotes clean code, and enhances productivity by automating CRUD operations.
- Adding the Dependency (
pom.xml
for Maven):
- Define an Entity:
- Create a Repository:
- Use the Repository in a Service:
- Expose the Service in a Controller:
- Database Configuration:
In the application.properties
file, you need to configure the database connection. For example, with an in-memory H2 database:
3. Spring Security#
- Purpose: Spring Security is a powerful framework that provides authentication (who you are) and authorization (what you're allowed to do) for Java applications, making sure that your application is secure from common vulnerabilities.
- Key Features:
- Out-of-the-Box Security: Automatically protects against common vulnerabilities like CSRF and XSS.
- OAuth2 and JWT: Supports modern authentication methods such as OAuth2 and JWT (for API security).
- Customizable: Easily extend and customize to meet specific security needs.
- Importance: Security is essential for any application. Spring Security provides robust tools to protect your application from unauthorized access and vulnerabilities.
- Add Spring Security Dependency:
- Basic Authentication Example:
- Form-Based Authentication Example:
- JWT (JSON Web Token) Authentication Example:
4. Lombok#
- Purpose: Lombok reduces boilerplate code in Java by automatically generating commonly used methods like getters, setters, constructors, and more during compile time. This helps streamline code, making it cleaner and more maintainable.
- Key Features:
@Getter
/@Setter
: Automatically generates getter and setter methods.@NoArgsConstructor
,@AllArgsConstructor
: Generates constructors.@Builder
: Provides a builder pattern for object creation.@Data
: Combines common annotations like@Getter
,@Setter
, and@ToString
.@Slf4j
: Is a Lombok annotation that automatically generates a logger field for your class. It simplifies logging by removing the need to explicitly define a logger in your class.
- Importance: It simplifies your code and improves readability by removing repetitive boilerplate code.
- Adding the Dependency (
pom.xml
for Maven):
5. Validation#
- Purpose: Hibernate Validator is the reference implementation of the Bean Validation (JSR 380) specification. It provides a powerful and extensible framework to validate user inputs and ensure data consistency in your application.
- Key Features:
- Bean validation using annotations like
@NotNull
,@Size
,@Email
. - Custom validators for specific requirements.
- Integration with Spring Boot for automatic validation.
- Bean validation using annotations like
- Importance: Validating request payloads in REST APIs or ensuring data integrity before persisting in a database.
- Adding the Dependency (
pom.xml
for Maven):
Conclusion#
This article emphasizes essential Spring Boot libraries, such as Spring Boot Starter Web and Spring Security, that simplify web development and enhance application security. Tools like Lombok and Hibernate Validator improve productivity by reducing boilerplate code and ensuring data validation. These libraries help developers build efficient, scalable, and secure systems.