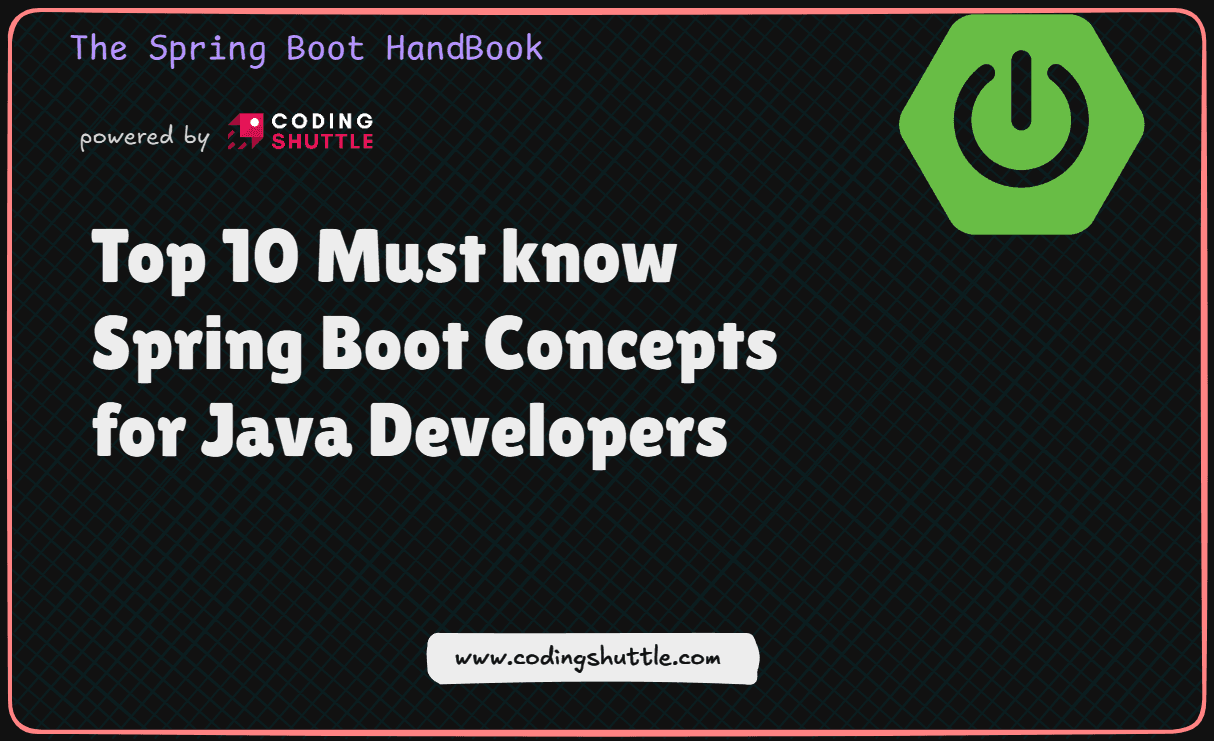
Top 10 Must-Know Spring Boot Concepts for Java Developers
Want to become a Java Developer? Here are the top 10 Spring Boot concepts you must know in 2025.
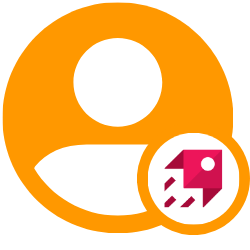
Santosh Mane
January 31, 2025
6 min read
Introduction#
If you're just starting out with Spring Boot, you might feel like there’s a lot to take in especially because it’s such a powerful tool for building Java applications. But don’t worry, I’m here to walk you through some of the key concepts you should definitely get familiar with. Think of this as the “Spring Boot essentials” that you’ll want under your belt, whether you're just beginning or have been working with it for a little while.
1. Spring Boot Starter Projects#
One of the first things that will probably blow your mind when working with Spring Boot is starter projects. They’re like pre-packaged templates that Spring Boot provides for common app setups. It’s like a shortcut. For example, if you want to create a web app, you can just pull in spring-boot-starter-web
, and boom you get everything set up, including Spring MVC, Tomcat, and Jackson (the JSON library). No need to manually configure those things one by one. You save time, and you get a reliable, working setup right away.
2. Auto-Configuration#
This is one of Spring Boot’s coolest features. Auto-configuration means that Spring Boot will automatically configure your application based on the libraries you’ve added to your project. So, let’s say you add spring-boot-starter-data-jpa
to your project for database interactions. Spring Boot will automatically configure Hibernate, JPA, and even data source connections without you lifting a finger. It’s like Spring Boot is looking at your dependencies and thinking, "Oh, you need this? I’ve got you covered." That’s one of the reasons why Spring Boot speeds up development so much.
3. Embedded Servers#
So, if you’ve worked with Java in the past, you probably remember the whole hassle of setting up an external server, like Tomcat, right? You had to install it, configure it, and get everything just right before even running your app. Well, Spring Boot makes all of that way simpler with its embedded servers. Basically, Spring Boot comes with a server built right into the framework—Tomcat, Jetty, or whatever you prefer—so you don’t need to bother with setting up anything externally. You just run your application directly from the command line, and the server fires up automatically. This is a game-changer because it makes your life easier and cuts down on all the extra steps when you’re just trying to get your app up and running.
4. Spring Boot Annotations#
If you’ve started using Spring Boot, you’ve probably noticed that annotations are everywhere and for good reason. They make things way easier. Let me break down a few that you’ll use all the time:
@SpringBootApplication
: Think of this one as your app's main entry point. It’s the big, important annotation that marks the class where everything starts. It’s kind of like a shortcut because it combines a few other important annotations for you (like@Configuration
,@EnableAutoConfiguration
, and@ComponentScan
).@RestController
: If you’re building a REST API, this one’s essential. It combines@Controller
and@ResponseBody
, making it easy to handle web requests and automatically send responses in various formats like JSON, XML, or even custom formats, depending on the request. This eliminates a lot of the boilerplate code you’d otherwise need to handle different response types.@Autowired
: This one is a huge time-saver. It’s all about dependency injection basically, it allows Spring Boot to automatically wire up your beans. Instead of having to manually set things up yourself, Spring Boot does the heavy lifting, so your code stays cleaner and more maintainable.
Once you get comfortable with these, you’ll realize just how much they streamline the development process. You won’t have to worry about a ton of configuration, and your code will stay neat and easy to follow.
5. Application Properties#
Configuring your Spring Boot app is usually done in application.properties (or application.yml) files. This is where you’ll set things like the database URL, logging levels, and server ports.
For instance, you might have something like this to configure your database:
It’s a pretty straightforward way to externalize your configuration. Plus, Spring Boot makes it easy to have different properties for different environments—like dev, test, and prod—just by creating different profiles.
6. Spring Boot Actuator#
Once your app is up and running in production, you’ll want to know how it’s doing. That’s where Spring Boot Actuator comes in. It’s a set of tools that helps you monitor your app’s health, performance, and various metrics. For example, you can check the /actuator/health
endpoint to see if your app is running smoothly, or /actuator/metrics
to get a look at things like memory usage and request counts.
It’s a lifesaver when you need to keep an eye on your app in production without setting up tons of extra monitoring tools.
7. Spring Data JPA#
If you're working with databases, you need to know about Spring Data JPA. This library helps you interact with your database using JPA (Java Persistence API) and makes life a lot easier. It takes care of all the boring CRUD (Create, Read, Update, Delete) operations for you. Just create an interface that extends JpaRepository
, and Spring Boot will automatically implement it for you.
Here’s an example:
The best part? You don’t have to write any implementation code. Spring Boot does all that under the hood.
8. Spring Security#
Security is always a top priority in backend development. Spring Security is Spring’s framework for handling authentication and authorization. It allows you to secure your application with minimal effort. You can set up authentication methods, restrict access to certain parts of your app, and even integrate with things like JWT for stateless authentication.
While it may seem a bit overwhelming at first, once you get a feel for it, Spring Security will become an essential tool for any project.
9. Spring Boot DevTools#
Want to speed up your development process? Spring Boot DevTools is here to help. It comes with features like automatic restarts and live reload—which means when you make changes to your code, the app automatically restarts, so you don’t have to manually restart it yourself.
It’s a small thing, but it can make a big difference in your workflow.
10. Testing in Spring Boot#
Testing is one of those things that you can’t skip if you want a solid app. With Spring Boot, it’s pretty easy to get started. For full integration tests, you’ve got @SpringBootTest
. But let’s say you just need to test a specific part, like your web controllers or repositories—use @WebMvcTest
or @DataJpaTest
.
What I love is how much Spring Boot takes care of for you. It sets up a lot of the testing environment, so you don’t have to waste time on all that boilerplate. You can just focus on writing the tests that actually matter to your app.
Conclusion#
Once you get a good grip on these Spring Boot concepts, you'll start noticing how much smoother your development process becomes. They’re the stuff that really makes building applications easier, faster, and more manageable. Don’t stress about knowing everything perfectly from the start—just keep playing around with the features and practicing. Over time, you’ll get more comfortable with it all, and before long, it’ll just feel like part of your regular workflow.