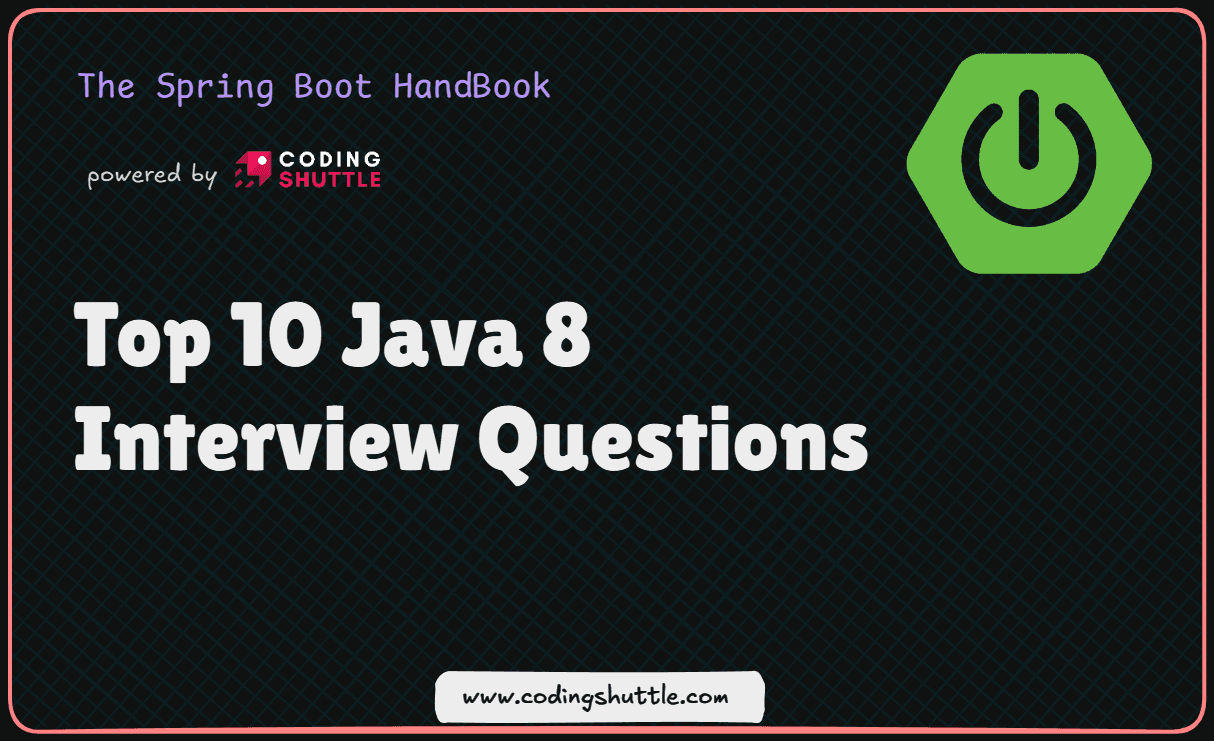
Top 10 Java 8 Interview Questions
Prepare for your next Java interview with this comprehensive guide to the top Java 8 features, including Lambda Expressions, Streams API, Optional Class, Default Methods, and more
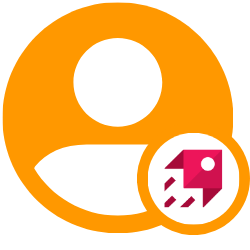
Santosh Mane
December 14, 2024
4 min read
Java 8 introduced several powerful features that revolutionized the way Java is used for programming. These features are frequently discussed during interviews. Here, we’ll explore the Top 10 Java 8 Interview Questions along with comprehensive answers to help you ace your interview.
1. What are the key features introduced in Java 8?#
Answer:
Java 8 introduced several groundbreaking features, including:
- Lambda Expressions: Enables functional programming by allowing concise syntax for implementing functional interfaces.
- Streams API: Facilitates functional-style operations on collections and sequences of elements.
- Functional Interfaces: Interfaces with a single abstract method, like
Runnable
andCallable
. Annotated with@FunctionalInterface
. - Optional Class: Provides a way to handle null values gracefully and avoid
NullPointerException
. - Default Methods: Allows interfaces to have methods with implementations.
- New Date and Time API: Introduced
java.time
package for better date-time handling. - Method References: Simplifies referring to methods by their names instead of invoking them.
- Nashorn JavaScript Engine: Allows integration of JavaScript with Java.
2. What are Lambda Expressions, and why are they useful?#
Answer:
Lambda Expressions are a way to represent anonymous functions in Java. They enable functional programming and concise code. The syntax is:
Example:
Benefits:
- Reduces boilerplate code.
- Improves readability.
- Enables functional-style programming.
3. What is the Streams API, and how is it used?#
Answer:
The Streams API is used for processing collections of data in a functional style. Streams support operations like filtering, mapping, and reducing.
Key Characteristics:
- Lazy Evaluation: Operations are not performed until a terminal operation is invoked.
- Stateless: Intermediate operations don’t store state.
- Parallelism: Simplifies parallel execution with
parallelStream()
.
Example:
4. What is a Functional Interface? Give examples.#
Answer:
A Functional Interface is an interface with exactly one abstract method. It can have any number of default or static methods.
Examples:
- Built-in Functional Interfaces:
Predicate<T>
: Accepts a single input and returns a boolean.Consumer<T>
: Accepts a single input and performs an operation.Supplier<T>
: Provides a result without taking any input.Function<T, R>
: Accepts an input and produces an output.
Example Code:
5. What are Method References in Java 8?#
Answer:
Method References provide a shorthand for referring to methods by their names. Syntax:
Types:
- Static Method Reference:
ClassName::staticMethod
- Instance Method Reference:
object::instanceMethod
- Constructor Reference:
ClassName::new
Example:
6. What is the Optional class in Java 8? Why is it useful?#
Answer:
The Optional class is a container for holding nullable values and helps avoid NullPointerException
.
Example:
Benefits:
- Encourages writing cleaner and safer code.
- Eliminates explicit null checks.
7. What are Default Methods in Interfaces?#
Answer:
Default Methods are methods with implementations inside interfaces. They were introduced to provide backward compatibility to existing interfaces.
Example:
8. How does Java 8 handle Date and Time?#
Answer:
Java 8 introduced the java.time package to replace the outdated java.util.Date
and Calendar
classes.
Key Classes:
LocalDate
: Represents a date without a time zone.LocalTime
: Represents a time without a date.LocalDateTime
: Combines date and time.ZonedDateTime
: Handles time zones.
Example:
9. What are Streams’ Terminal and Intermediate Operations?#
Answer:
- Intermediate Operations: Return another stream. Examples:
filter()
,map()
,sorted()
. - Terminal Operations: Trigger the processing of streams. Examples:
collect()
,forEach()
,reduce()
.
Example:
10. What are the differences between a Stream and a Collection?#
Answer:
Feature | Stream | Collection |
---|---|---|
Processing | Lazy (performed only on terminal ops). | Eager (performed on element addition). |
Iteration | Functional-style iteration. | External iteration. |
Modifiability | Immutable. | Mutable. |
Parallelism | Built-in support via parallelStream() . | Manual setup required. |
Conclusion#
These questions represent some of the most important Java 8 concepts frequently asked in interviews. Mastering these will not only help you in interviews but also enhance your coding skills for real-world applications. Good luck!