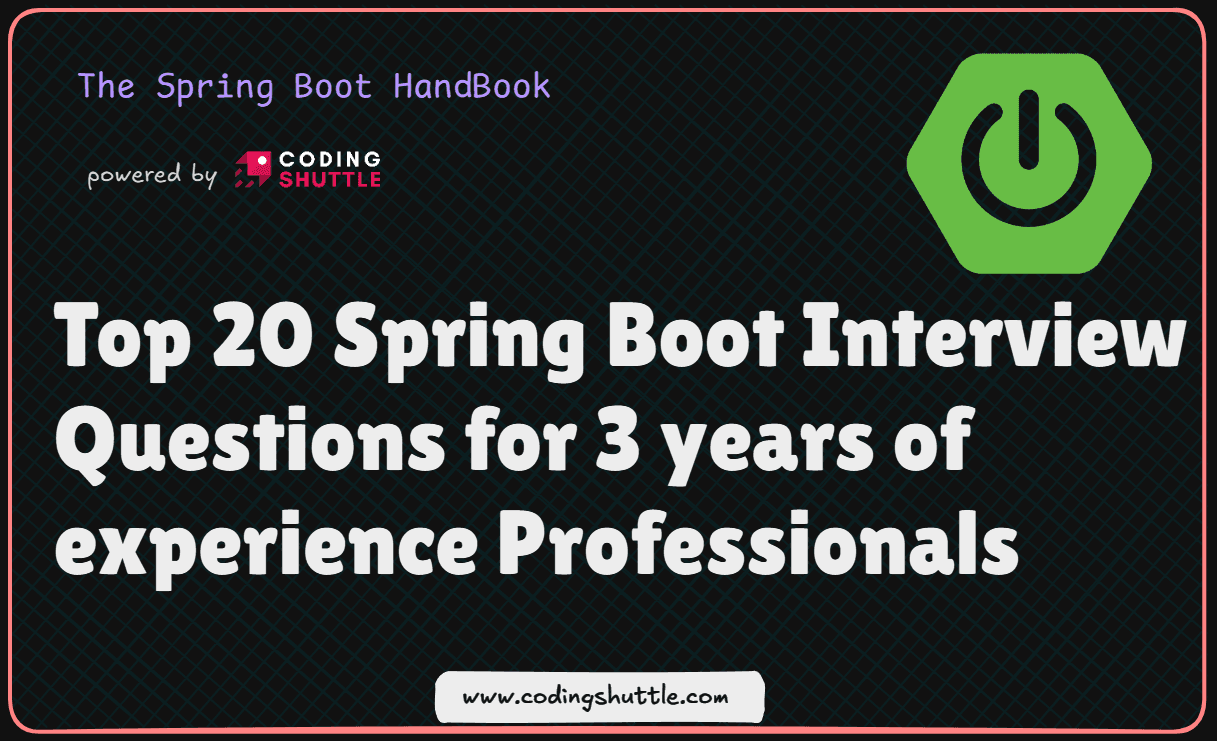
Top 20 Spring Boot Interview Questions for 3 years of experience Professionals
Master your Spring Boot interviews with this comprehensive guide! Designed for professionals with 3 years of experience, this blog covers 20 essential questions and answers
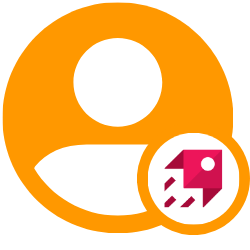
Santosh Mane
December 14, 2024
10 min read
Spring Boot has become the go-to framework for Java developers due to its simplicity and productivity. If you're preparing for a Spring Boot interview with 3 years of experience, here are 20 essential questions and answers to help you ace your interview.
1. What is the purpose of Spring Boot’s @EnableAutoConfiguration annotation?#
Answer: The @EnableAutoConfiguration
annotation is used to enable Spring Boot's auto-configuration mechanism. It attempts to automatically configure your Spring application based on the libraries available on the classpath. For example, if spring-data-jpa
is on the classpath, Spring Boot will configure a JPA EntityManagerFactory.
2. How@SpringBootApplication
and @EnableAutoConfiguration
related?#
Answer:
Relationship between @SpringBootApplication
and @EnableAutoConfiguration
:
@SpringBootApplication
includes@EnableAutoConfiguration
as part of its functionality. Therefore, when you use@SpringBootApplication
in your main application class, you're automatically enabling auto-configuration without needing to explicitly annotate your class with@EnableAutoConfiguration
.- The key difference is that
@SpringBootApplication
is a higher-level annotation combining multiple annotations (@Configuration, @EnableAutoConfiguration, @ComponentScan), whereas@EnableAutoConfiguration
is focused solely on auto-configuration. Using@SpringBootApplication
simplifies the setup process, while@EnableAutoConfiguration
can be used independently when you want to enable auto-configuration in a class without requiring component scanning or bean definitions.
3. What is the difference between @Component, @Repository, and @Service annotations in Spring Boot?#
Answer:
@Component
: A generic stereotype for any Spring-managed component.@Repository
: A specialization of@Component
for data access layers; it also provides additional capabilities like exception translation.@Service
: A specialization of@Component
for service layers to indicate business logic.
4. How does Spring Boot support asynchronous processing?#
Answer: Spring Boot supports asynchronous processing using the @Async
annotation. To enable it, you need to add @EnableAsync
in a configuration class. Methods annotated with @Async
run in a separate thread pool and return a Future
or CompletableFuture
.
5. What is the significance of Spring Boot’s @Conditional annotations?#
Answer: Spring Boot’s @Conditional
annotations allow beans to be loaded conditionally based on:
- The presence/absence of a class (
@ConditionalOnClass
or@ConditionalOnMissingClass
). - A specific property value (
@ConditionalOnProperty
). - A particular bean being defined (
@ConditionalOnBean
). - A custom condition (
@Conditional
).
6. How does Spring Boot handle logging?#
Answer: Spring Boot uses SLF4J as the default logging API and Logback as the default implementation. Logging levels can be configured in application.properties
or application.yml
. Example:
You can also customize Logback by providing a logback-spring.xml
file in the resources
folder.
7. What is CSRF protection in Spring Security and how can you disable it ?#
Answer:
CSRF (Cross-Site Request Forgery) is an attack where a user is tricked into performing unintended actions on a web application they are authenticated to. Spring Security provides protection against CSRF attacks by requiring a CSRF token for sensitive requests (like POST
, PUT
, DELETE
), which ensures the request is coming from the legitimate user.
How CSRF Protection Works:#
- Spring Security generates a CSRF token for each session.
- The client must send this token in each sensitive request.
- If the token doesn't match the server's stored token, the request is rejected.
Disabling CSRF Protection:#
For stateless applications (e.g., APIs using JWT), you can disable CSRF as it's not necessary. You can disable it with the following configuration:
When to Disable CSRF:#
- When Using stateless authentication (like JWT) or APIs.
- Not needed if you're not using session-based authentication.
8. What is the purpose of the SecurityContextHolder
in Spring Security?#
Answer:
SecurityContextHolder
holds the security context of the current user, which contains the authentication information. This context is typically stored in a thread-local variable and is accessible throughout the request cycle. It provides access to the Authentication
object, which contains details about the authenticated user.
9. What are the benefits of Spring Boot’s actuator endpoints?#
Answer: Spring Boot Actuator provides endpoints to monitor and manage your application. Benefits include:
- Real-time application monitoring.
- Health checks with
/actuator/health
. - Metrics collection with
/actuator/metrics
. - Thread dump analysis with
/actuator/threaddump
. - Easy integration with tools like Prometheus and Grafana.
10. How does Spring Boot’s @Value annotation work?#
Answer: The @Value
annotation is used to inject values from properties files, system environment variables, or command-line arguments. Example:
If app.name
is defined in application.properties
, its value will be injected into appName
.
11. What is Spring Boot’s WebClient, and how does it differ from RestTemplate?#
Answer:
- RestTemplate is blocking and synchronous, which means the calling thread waits for the HTTP response before moving on. RestTemplate is more straightforward for simpler, traditional applications that don't require reactive features.
- WebClient is non-blocking, meaning it allows asynchronous, non-blocking calls, and is more suitable for high-performance, scalable applications. WebClient is ideal for reactive applications and environments where performance is crucial, particularly when making multiple concurrent HTTP requests.
12. How does Spring manage dependency injection for circular dependencies?#
Answer: Circular dependencies occur when two or more beans depend on each other directly or indirectly.
Example:
Spring resolves them by using:
- Setter Injection: Defers bean initialization until all dependencies are available. This allows Spring to create the beans and then set their dependencies afterward.
@Lazy
Annotation: Defers initialization of one of the beans.
- Refactoring to Break the Dependency If possible, refactor the design to remove the circular dependency. For instance, introduce a third bean (a mediator) that acts as a bridge between
A
andB
.
13. Explain the role of @Primary
, @Qualifier
, and @Profile
in bean selection.#
Answer:
@Primary
: Marks a bean as the default for autowiring when multiple candidates are available.@Qualifier
: Used to specify the exact bean to inject when ambiguity exists.@Profile
: Activates beans only in specific environments based on the active profile (e.g.,dev
,prod
).
14. What is the difference between @Bean
and @Component
?#
Answer:
@Component
: Used for automatic bean detection and registration during classpath scanning.@Bean
: Used to explicitly declare a bean in a@Configuration
annotated class, typically for third-party library objects or complex initialization logic. It is used when you need more control over the bean instantiation process
15. What is a Circuit Breaker, and how do you implement it in Spring Boot?#
Answer: A circuit breaker prevents a system from making repeated failed calls to a service. In Spring Boot, it can be implemented using Resilience4j:
- Add the
resilience4j-spring-boot2
dependency. - Annotate methods with
@CircuitBreaker
.
Example:
16. How do you secure Spring Boot REST APIs using OAuth2 for login with a provider like Google?#
Answer:
Add Dependency:
Configure Client Registration: Add the client ID and secret in application.yml
:
Implement Security Configuration:
Use .oauth2Login()
in your HttpSecurity
setup:
Handle OAuth2 Success: Implement a custom success handler:
Process Tokens: Use your JwtService
to generate and validate access and refresh tokens for secured API access.
17. What is the purpose of @DataJpaTest
in Spring Boot?#
Answer: @DataJpaTest
is used for testing the JPA repository layer. It configures an in-memory database and loads the repository beans but excludes other Spring components like the service or controller layer. It is primarily used for testing database interactions in isolation. Example:
18. What are @ConfigurationProperties in Spring Boot?#
Answer:
@ConfigurationProperties
binds hierarchical configuration properties to Java objects.
Example:
@ConfigurationProperties(prefix = "app")
#
- Maps properties with the prefix
app
to the fields of this class. For example:
If yourapplication.yml
has:
These values will automatically be set to the name
and version
fields of the AppProperties
class.
Example Usage:
You can now inject AppProperties
into any other Spring component using @Autowired
:
19. How does Spring Boot handle caching, and what are the different annotations available for caching?#
Answer: Spring Boot handles caching through its @Cacheable
, @CachePut
, and @CacheEvict
annotations.
@Cacheable
: Caches the result of a method. If the method is called with the same parameters, the cached result is returned instead of executing the method again.@CachePut
: Updates the cache with the method’s result, even if the result already exists in the cache.@CacheEvict
: Removes data from the cache. It can be used for clearing caches after an update.
Example:
20. What are the different scopes of Spring Beans?#
Answer:
Spring supports the following bean scopes:
singleton
(default):- A single shared instance of the bean is created and used throughout the Spring IoC container. This is the default scope if no scope is specified.
- Every request for this bean will return the same instance.
prototype
:- A new instance of the bean is created each time it is requested from the Spring container.
- This scope is suitable for beans that need to be stateless or have a short lifecycle.
request
(Web applications only):- A new instance is created for each HTTP request.
- The bean is only available during the lifecycle of the HTTP request and is discarded after the request is completed.
session
(Web applications only):- A new instance is created for each HTTP session.
- The bean is tied to the HTTP session and remains active for the duration of that session.
application
(Web applications only):- A single instance of the bean is created for the entire ServletContext (the entire web application).
- The bean is shared across all HTTP requests and sessions in the application.
websocket
(Web applications only):- A new instance is created for each WebSocket connection.
- The bean is tied to the lifecycle of a WebSocket connection and is discarded once the connection is closed.
Conclusion#
Preparing for a Spring Boot interview as a professional with 3 years of experience requires a solid grasp of the framework’s key concepts, annotations, and practical implementations. This blog has covered some of the most important interview questions to help you build confidence and showcase your expertise during the interview.