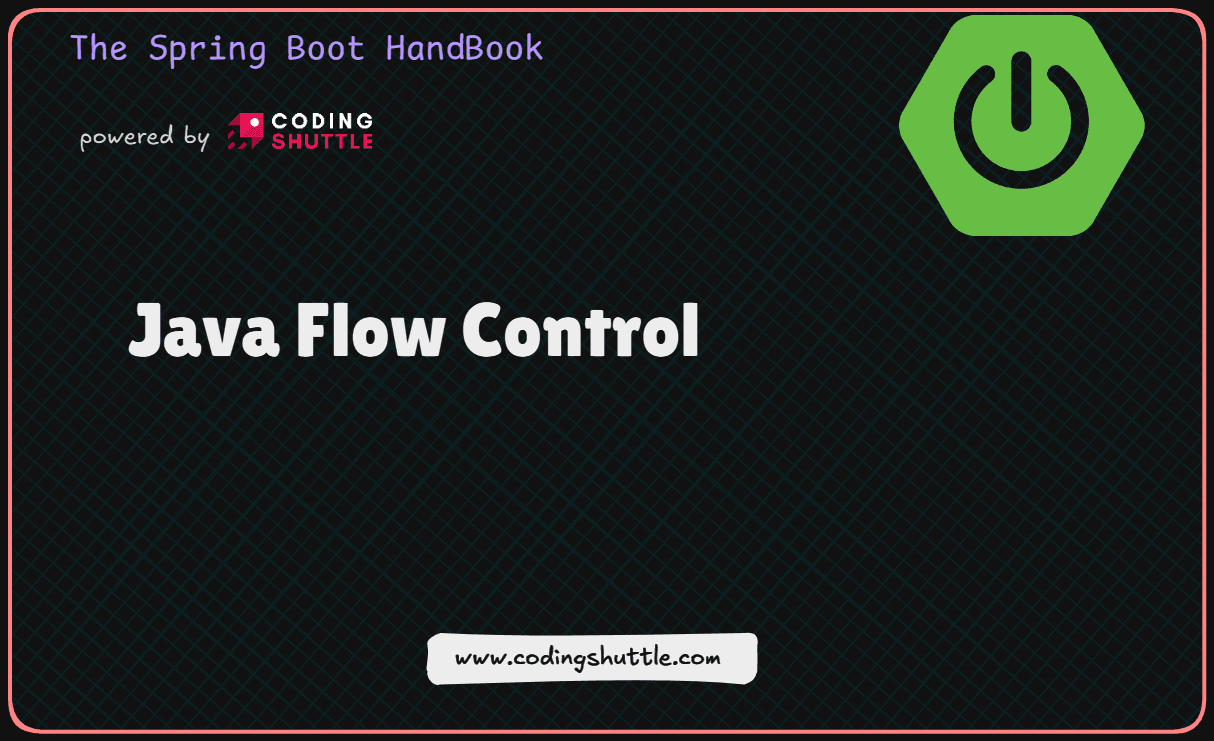
Java Flow Control: A guide to understand the If-else and Loops in Java
This article provides an in-depth exploration of Java flow control statements, covering decision-making, looping, and branching mechanisms. With clear explanations, syntax examples, and practical applications, it serves as a valuable resource for both beginners and experienced developers aiming to enhance their Java programming skills.
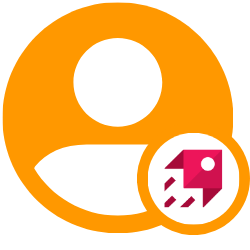
Shreya Adak
February 14, 2025
8 min read
Introduction:#
In Java, flow control statements dictate the order in which instructions are executed, enabling developers to manage the program's execution path based on specific conditions or repetitions. These statements are fundamental for implementing your code's decision-making, looping, and branching mechanisms.
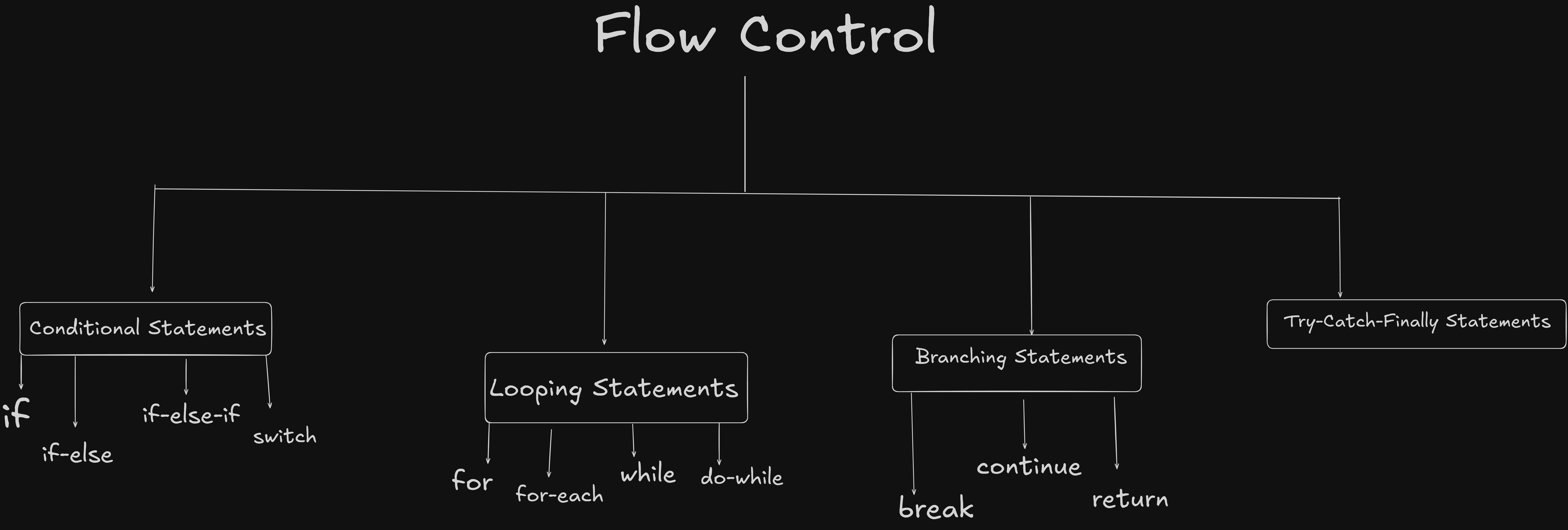
1. Decision-Making Statements (Conditional Statements):#
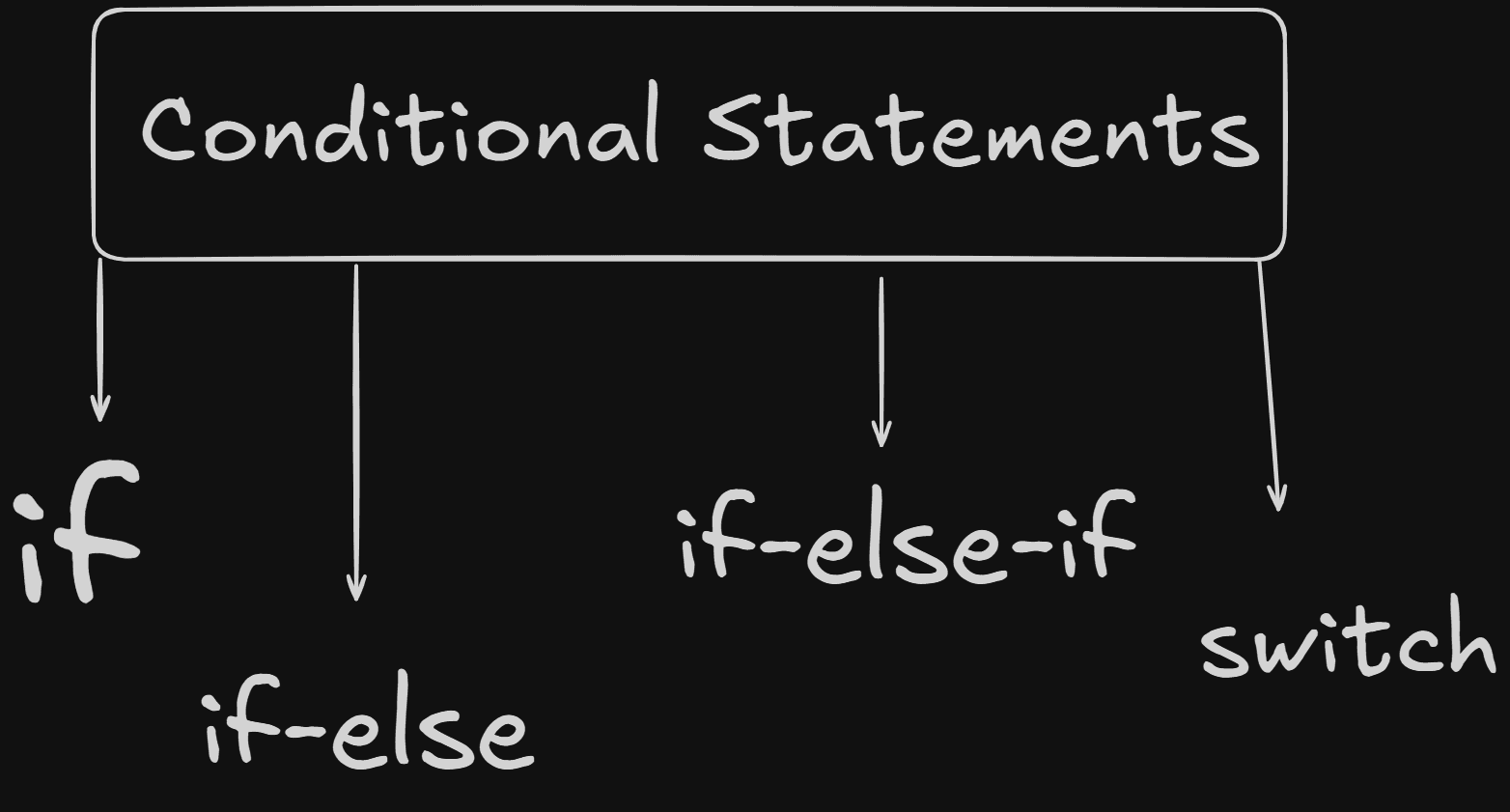
In Java, decision-making statements help to control the flow of execution based on certain conditions. These statements evaluate the expressions and execute the different code blocks depending on whether the conditions are true or false.
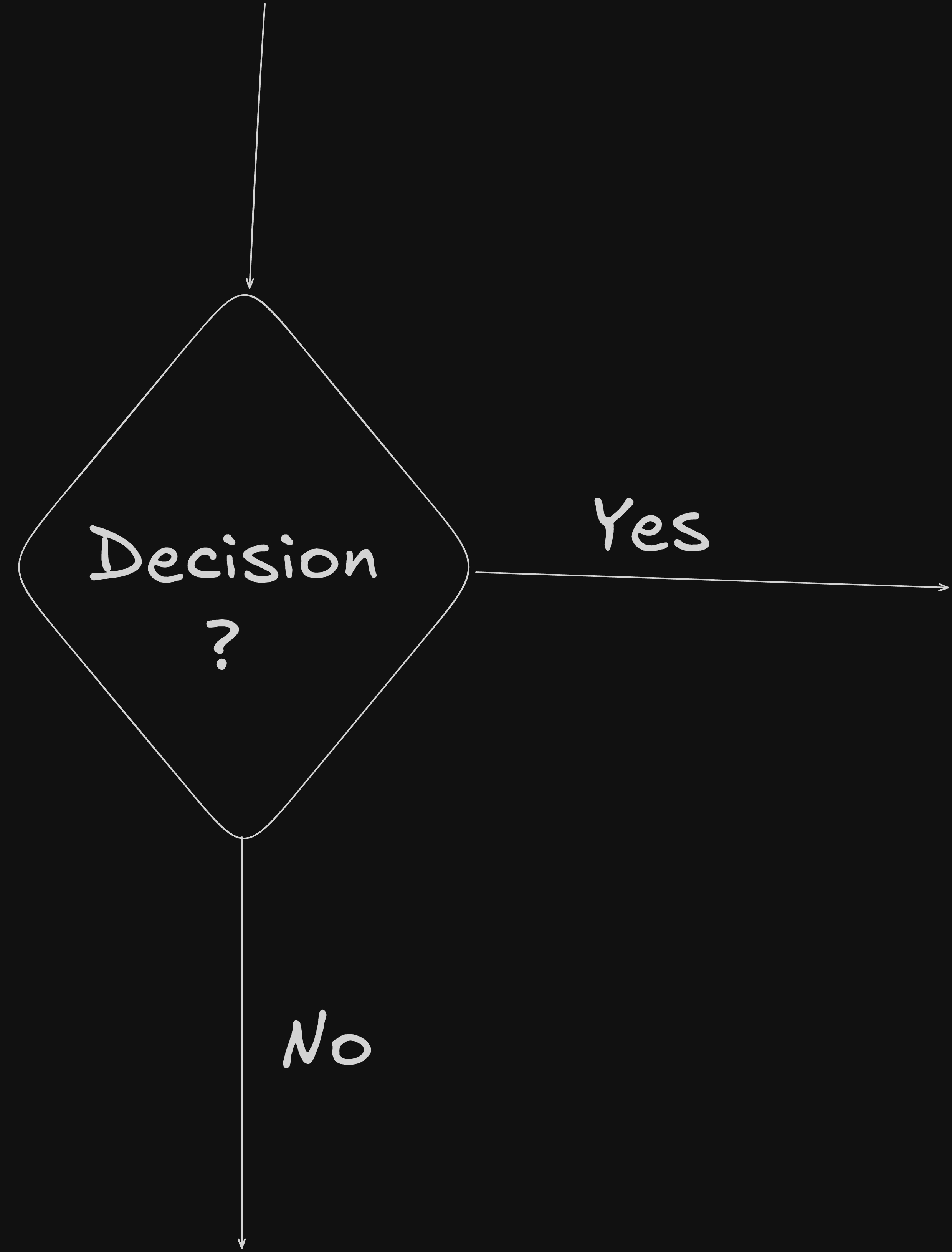
a. if
Statement:#
It evaluates a boolean expression; if true, executes the associated block of code.
b. if-else
Statement:#
Provides an alternative path if the if
condition evaluates to false.
c. if-else-if
Ladder:#
Chains multiple conditions; executes the block where the condition is true.
d.switch
Statement:#
It allows the selection among multiple options based on the value of an expression.
Ternary Operator: It is used to evaluate a test condition and executes a block of code based on the result of the condition. This operator replaces certain types of if-else statements.
Syntax:
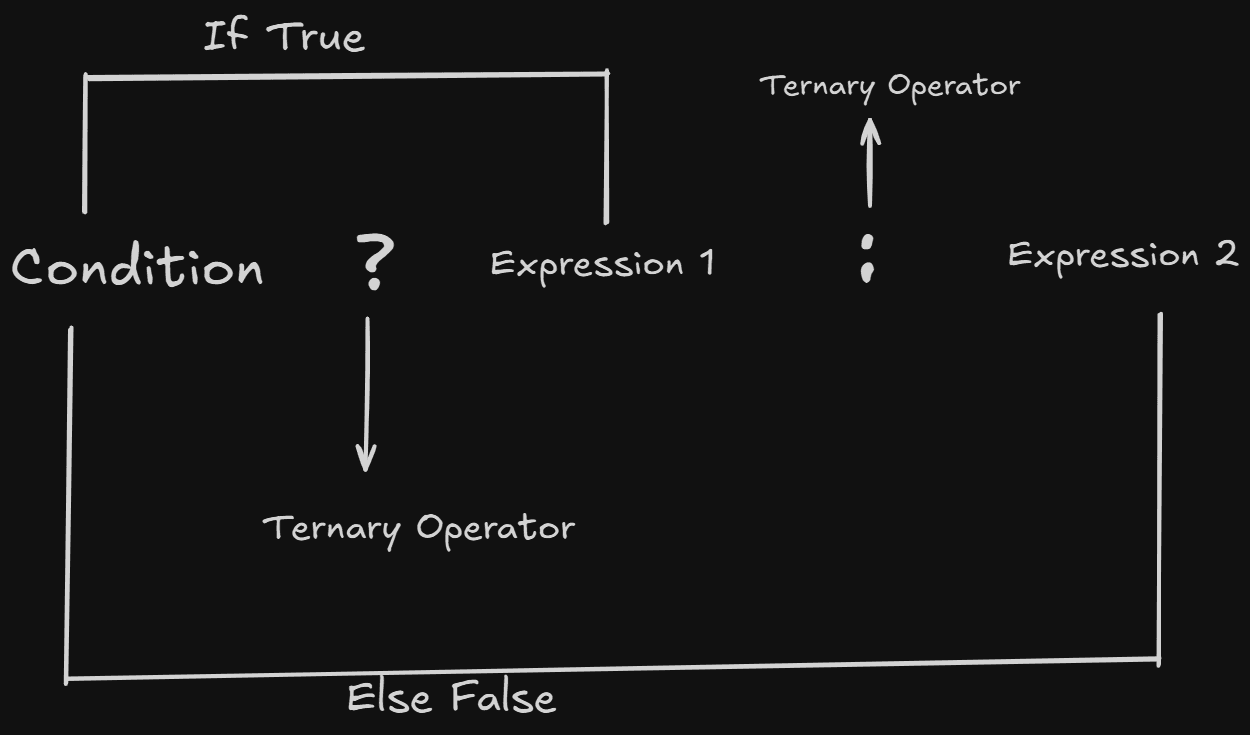
2. Looping Statements (Iteration Statements):#
- Initialization Expression(s)
- Test Expressions (Conditions)
- Body of the Loop
- Update Expressions
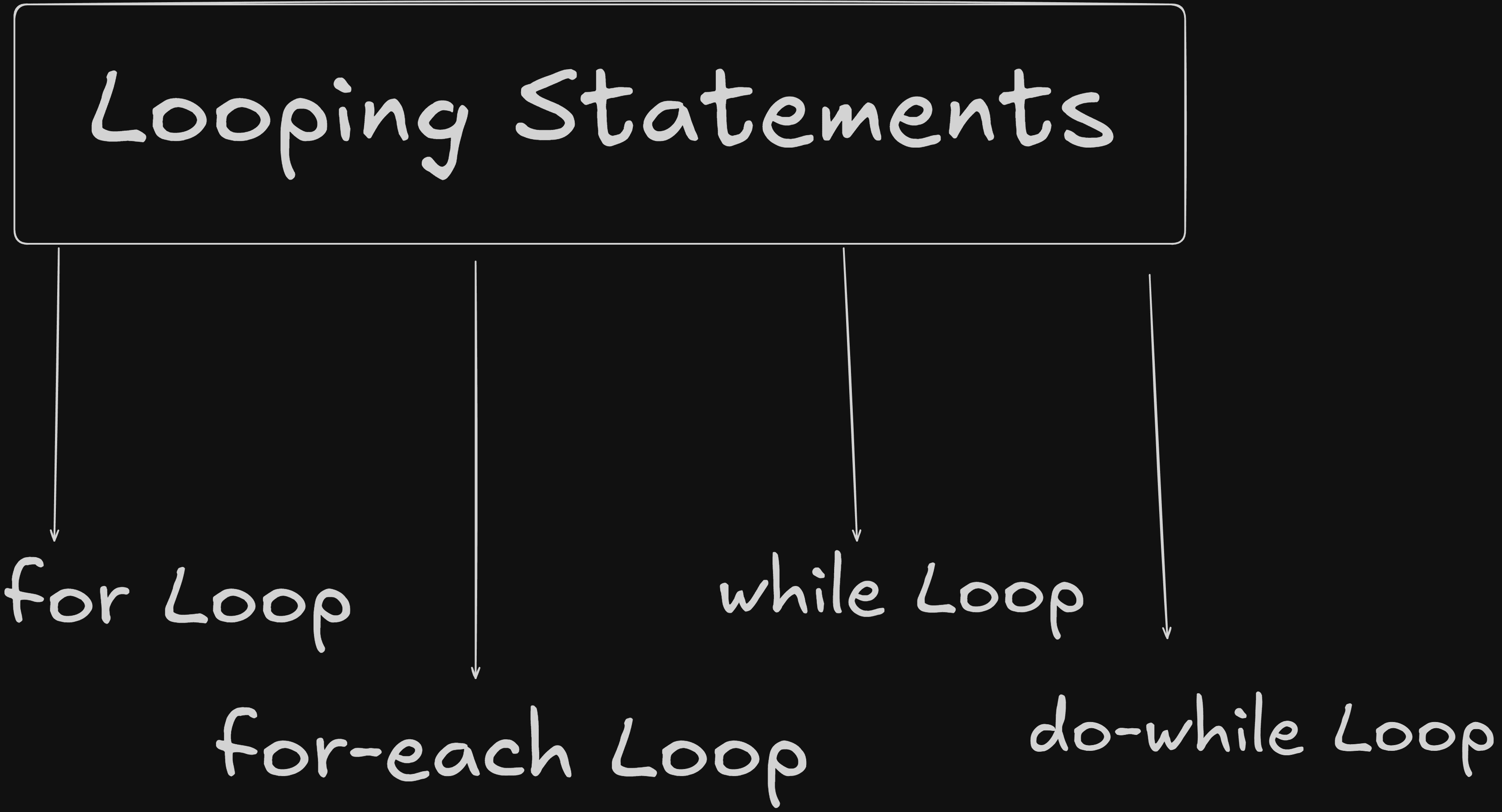
Looping statements in Java allow executing a code block multiple times until a specified condition is met. This helps in reducing redundancy and improving efficiency in programs.
a.for
Loop:#
The traditional for
loop is used when we need explicit control over the loop index. It is useful for iterating over an array or a list where we may need to modify elements or access specific indices.
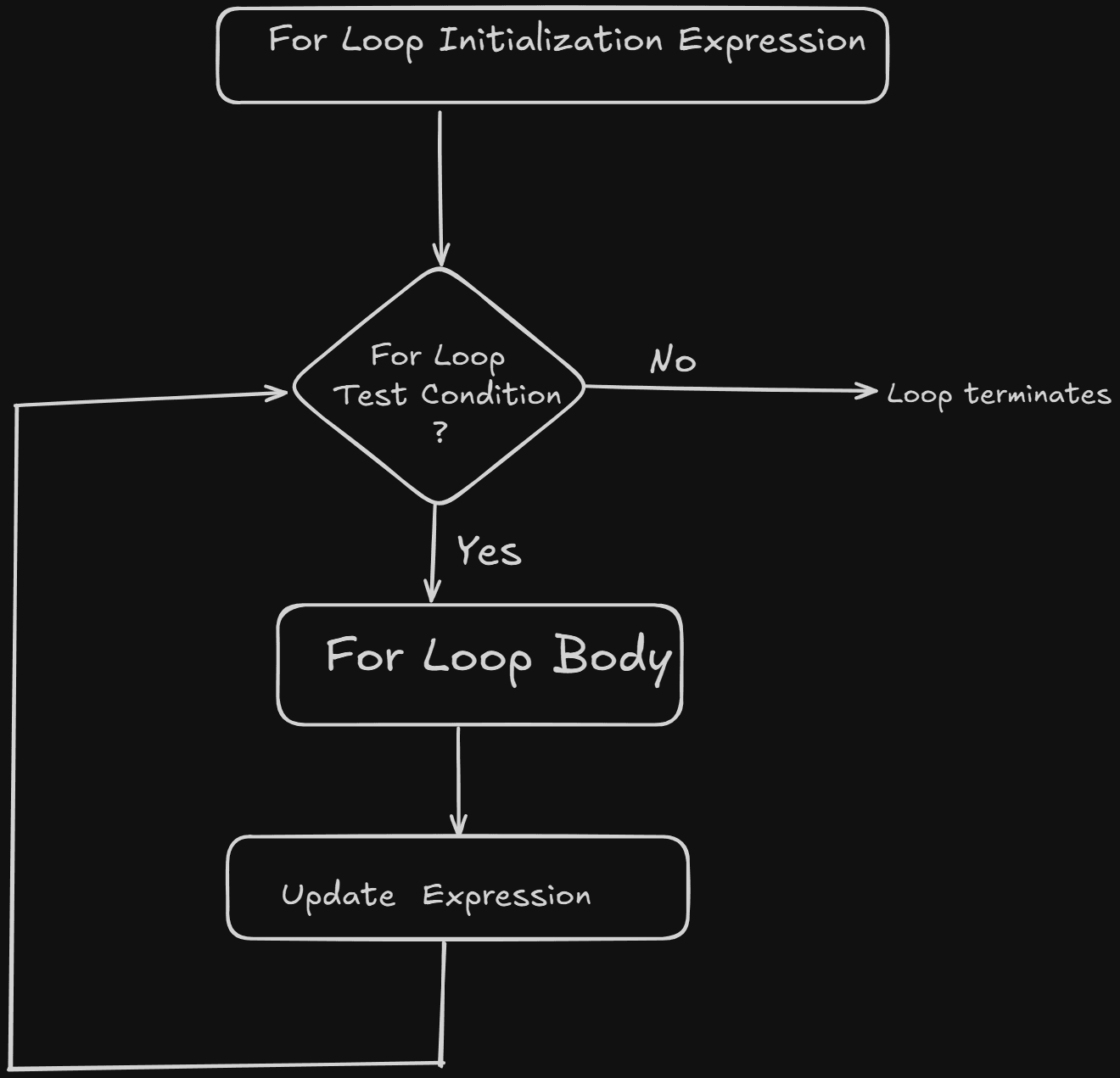
Syntax:
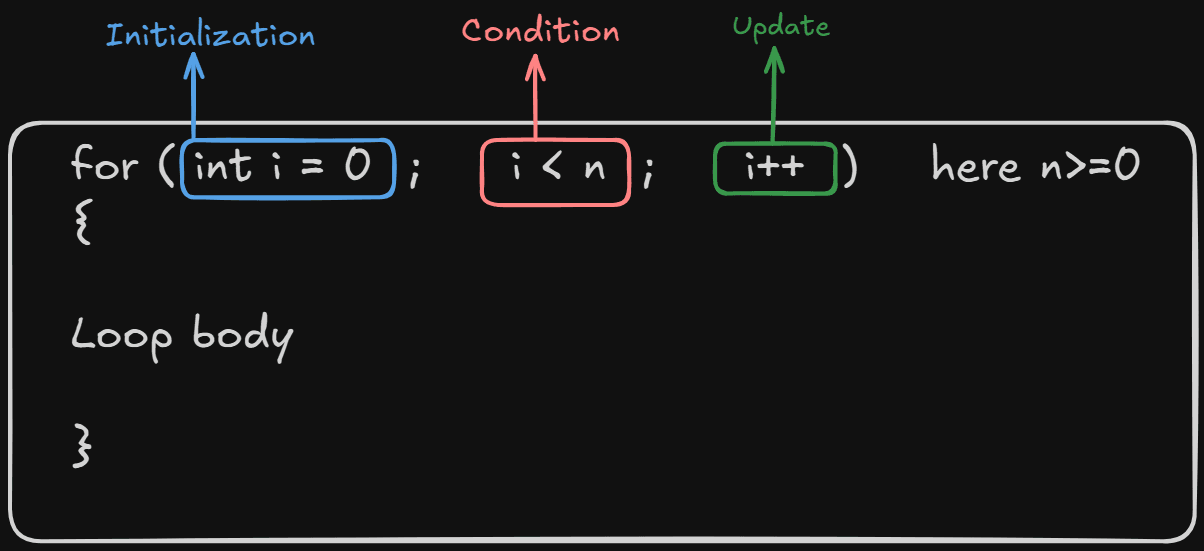
Output:
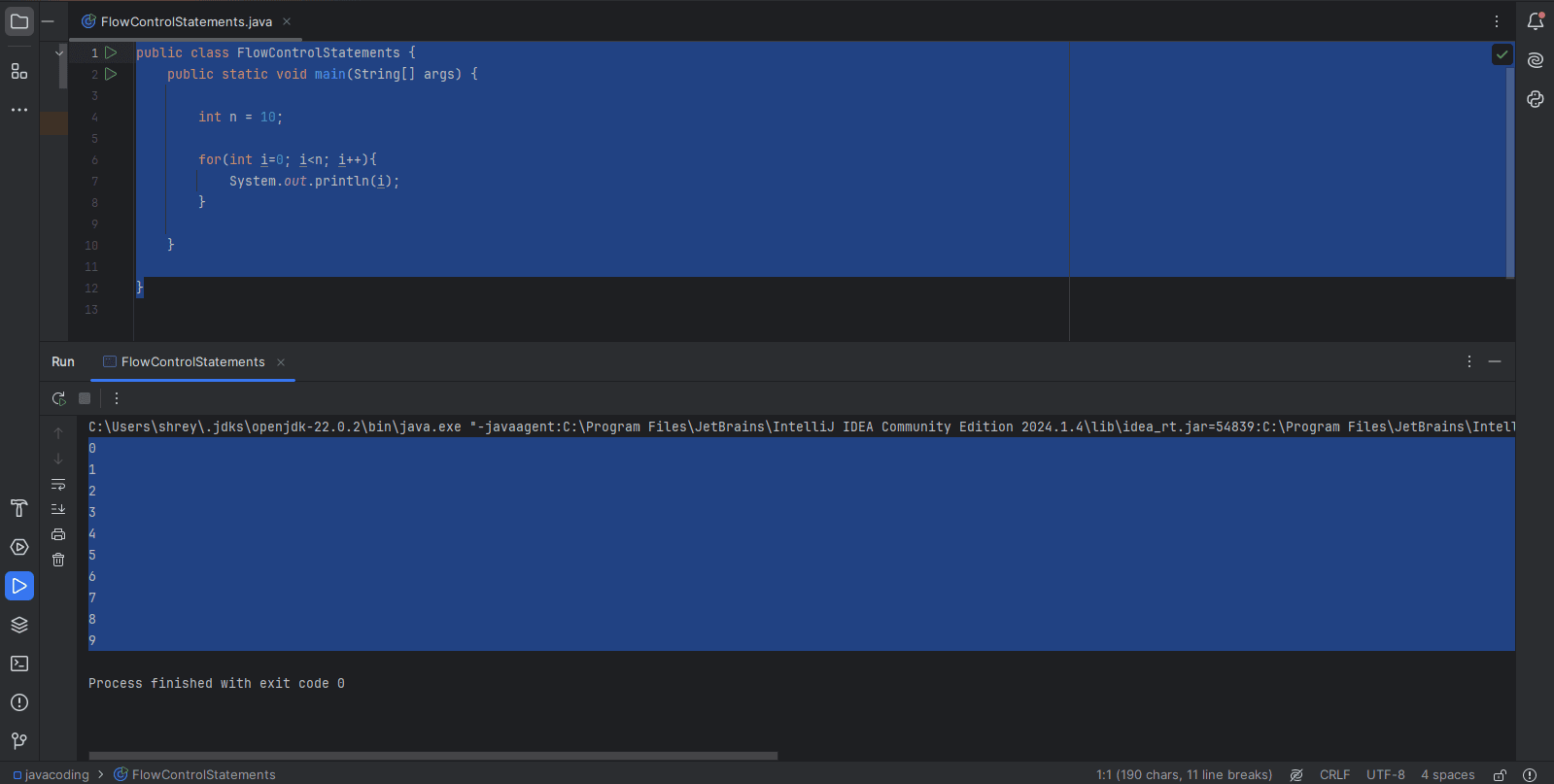
b. Enhanced for
Loop (for-each):#
Introduced in Java 5, the for-each loop is a simplified way of iterating over arrays and collections. It does not require an index and is read-only (modifications to elements are not possible directly).
Syntax:
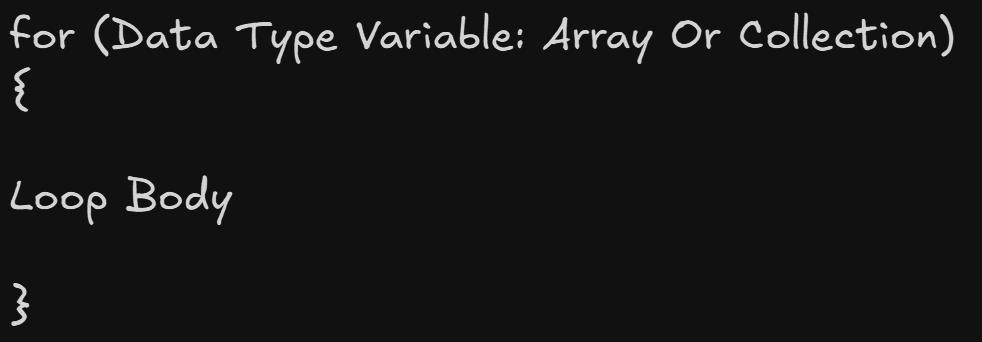
Output:
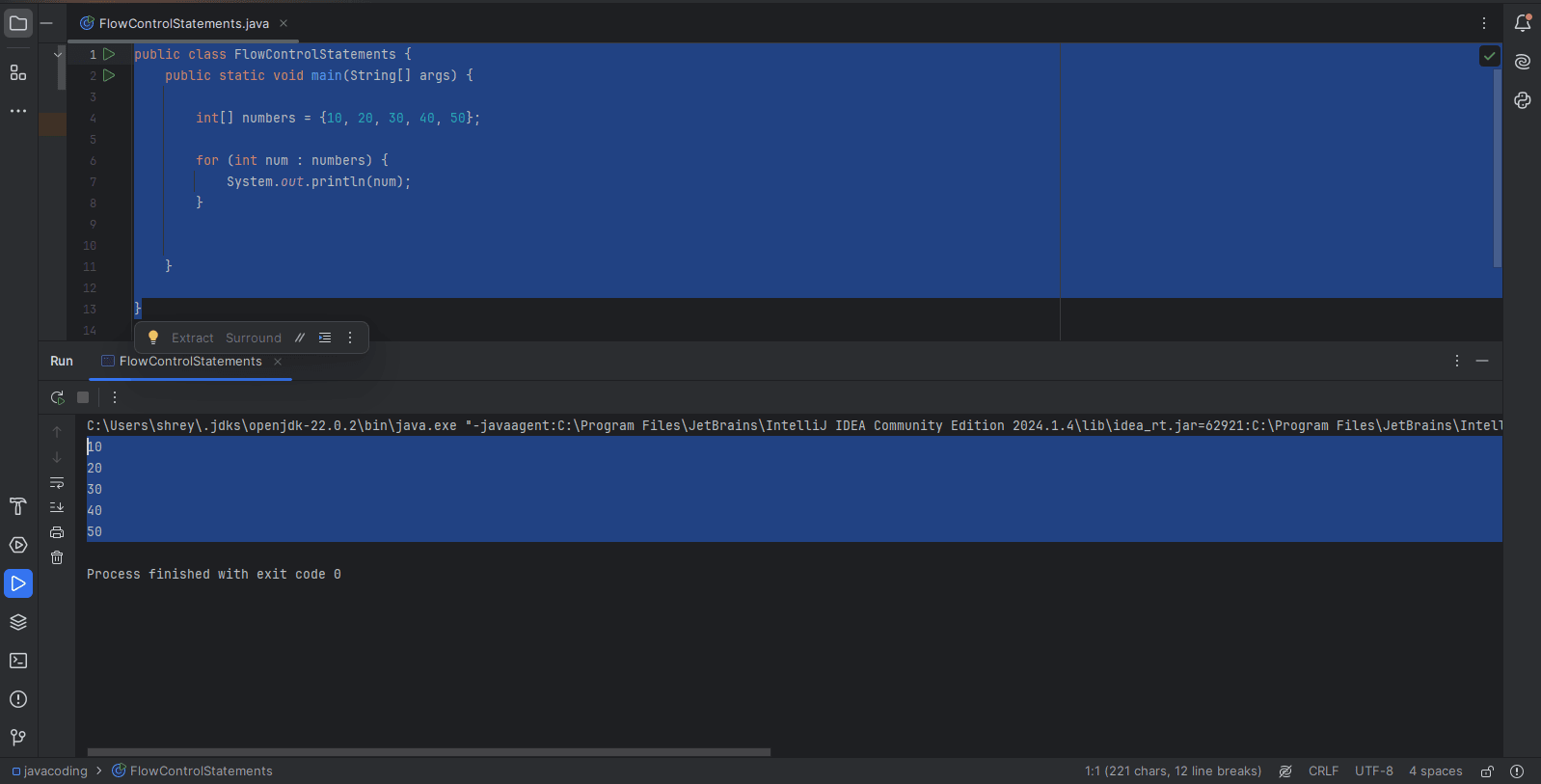
c. while
Loop:#
The condition is checked first before executing the loop body. If the condition is false initially, the loop body will not execute even once. It is an entry-controlled loop (checks condition before execution).
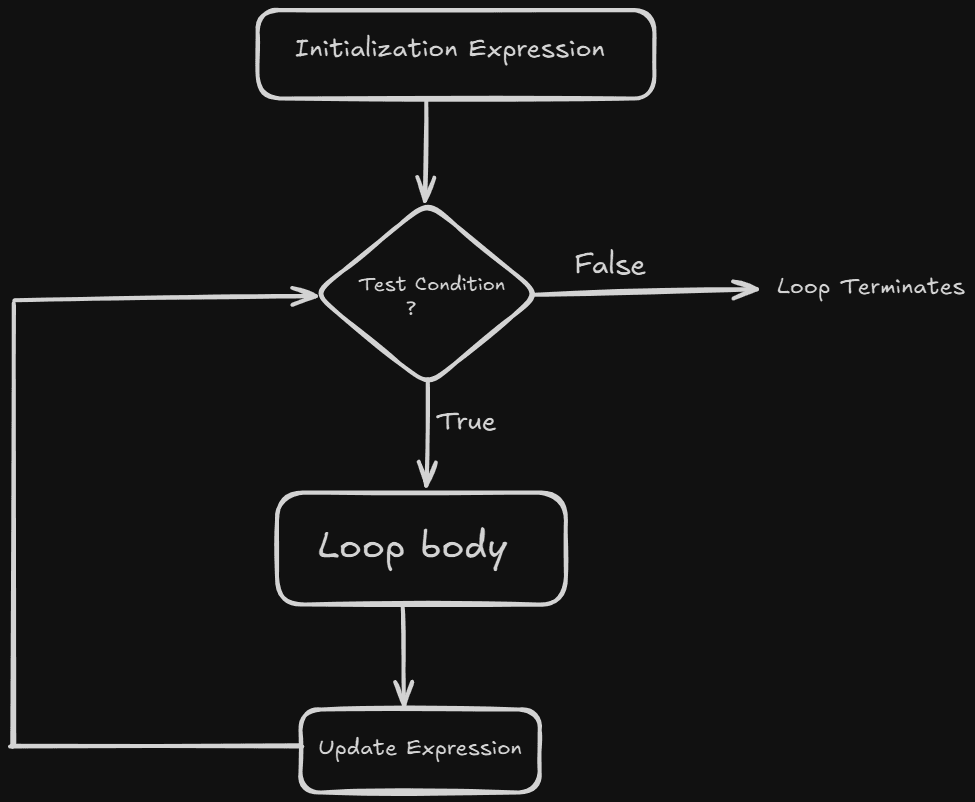
Syntax:
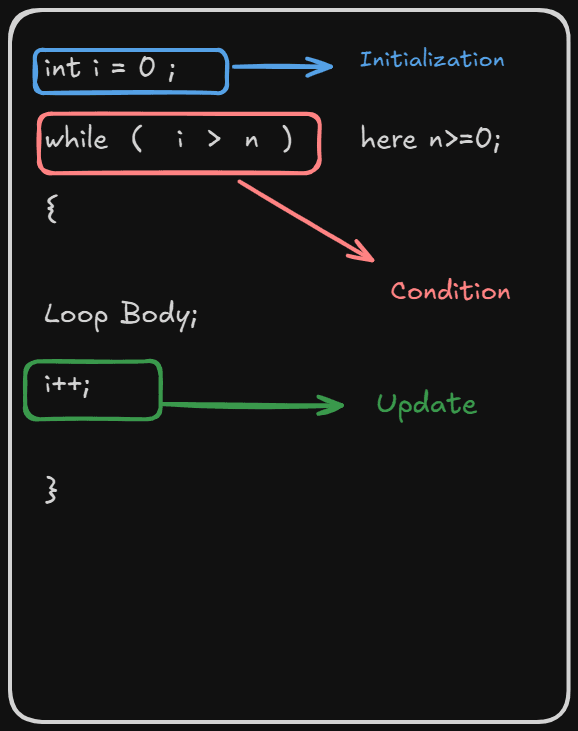
Output:
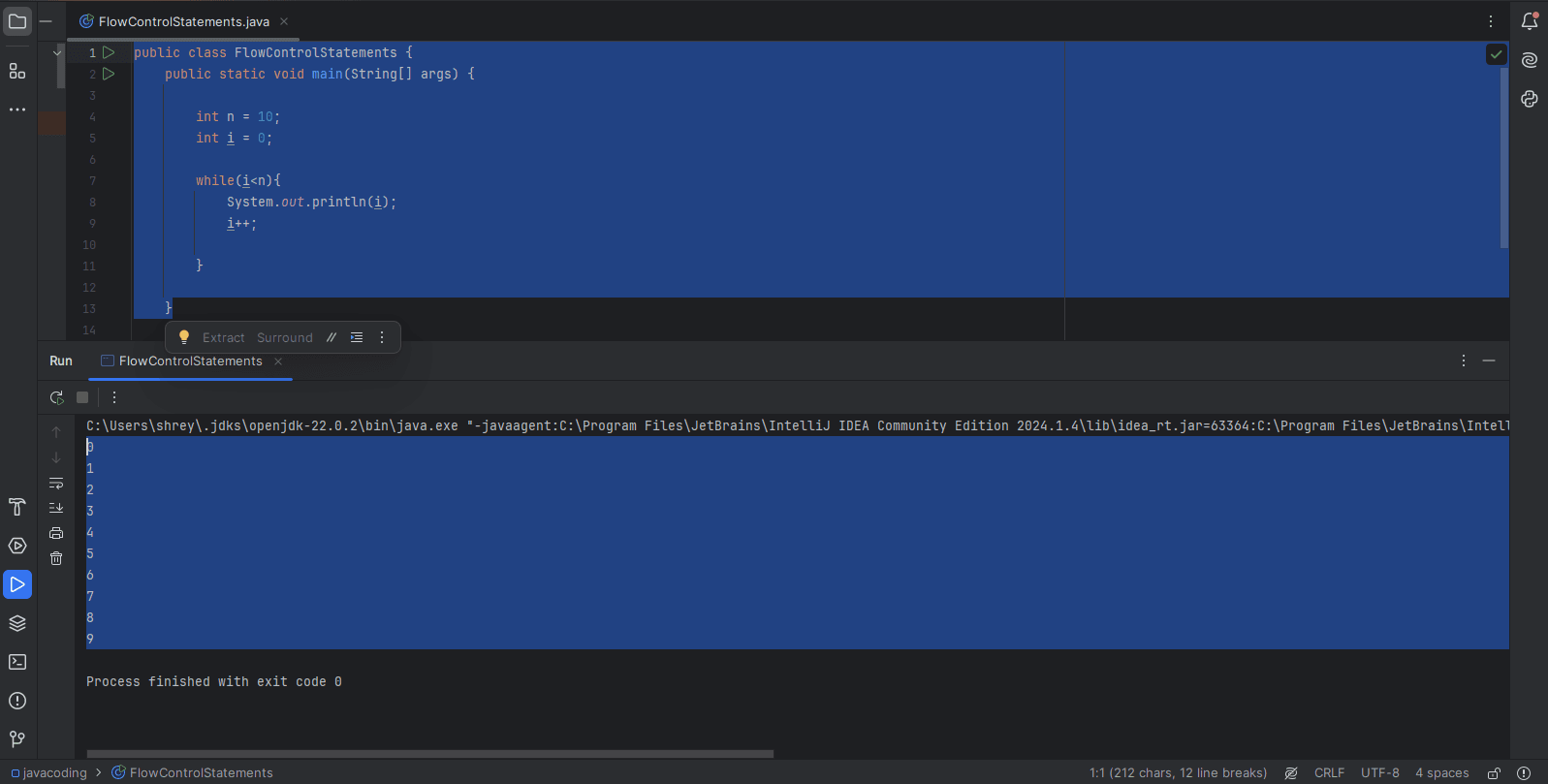
d. do-while
Loop: (Executes Once Even if Condition is False)#
The loop body executes first, then the condition is checked. Ensures that the loop executes at least once, even if the condition is false initially. It is an exit-controlled loop (checks condition after execution).
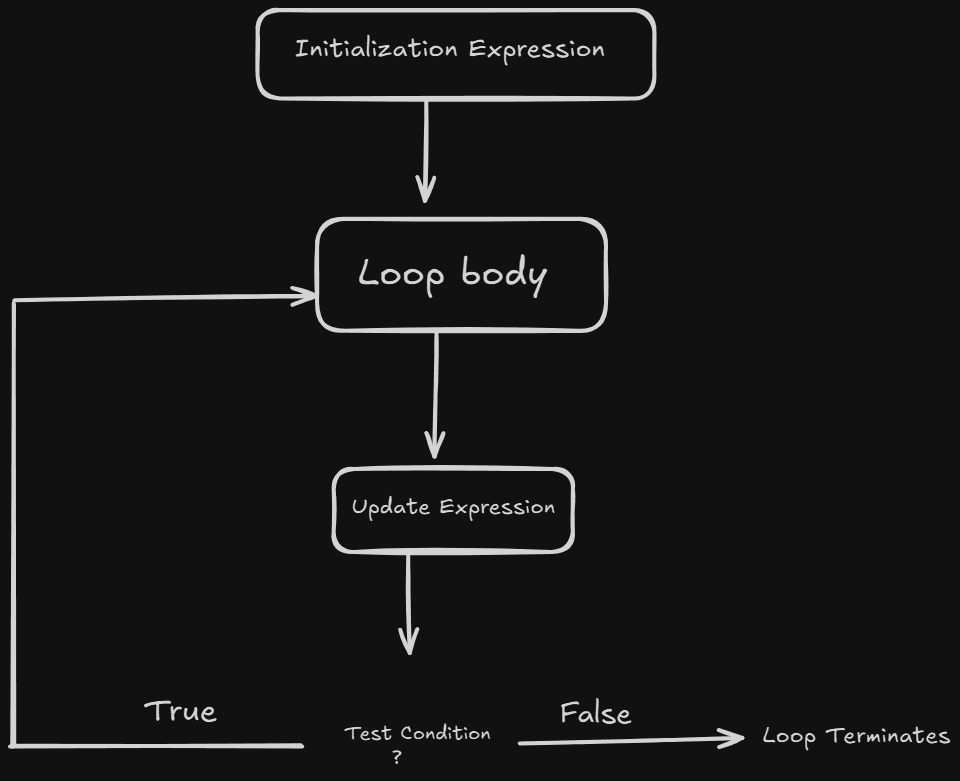
Syntax:
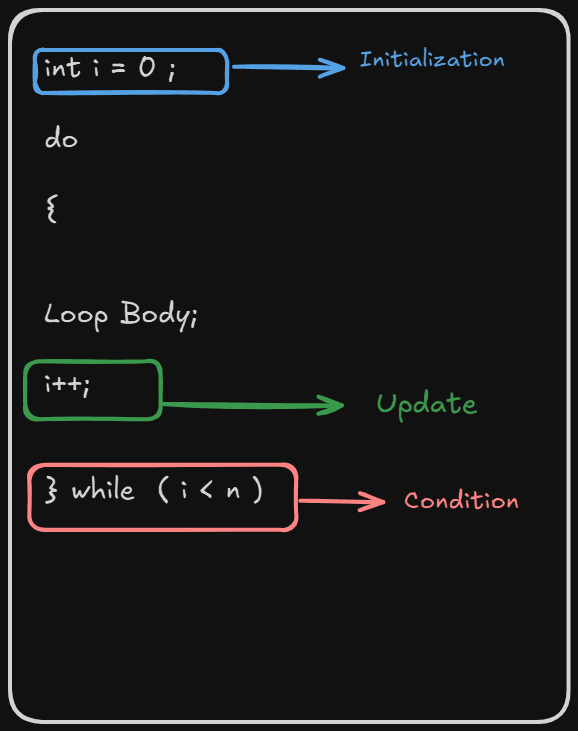
Output:
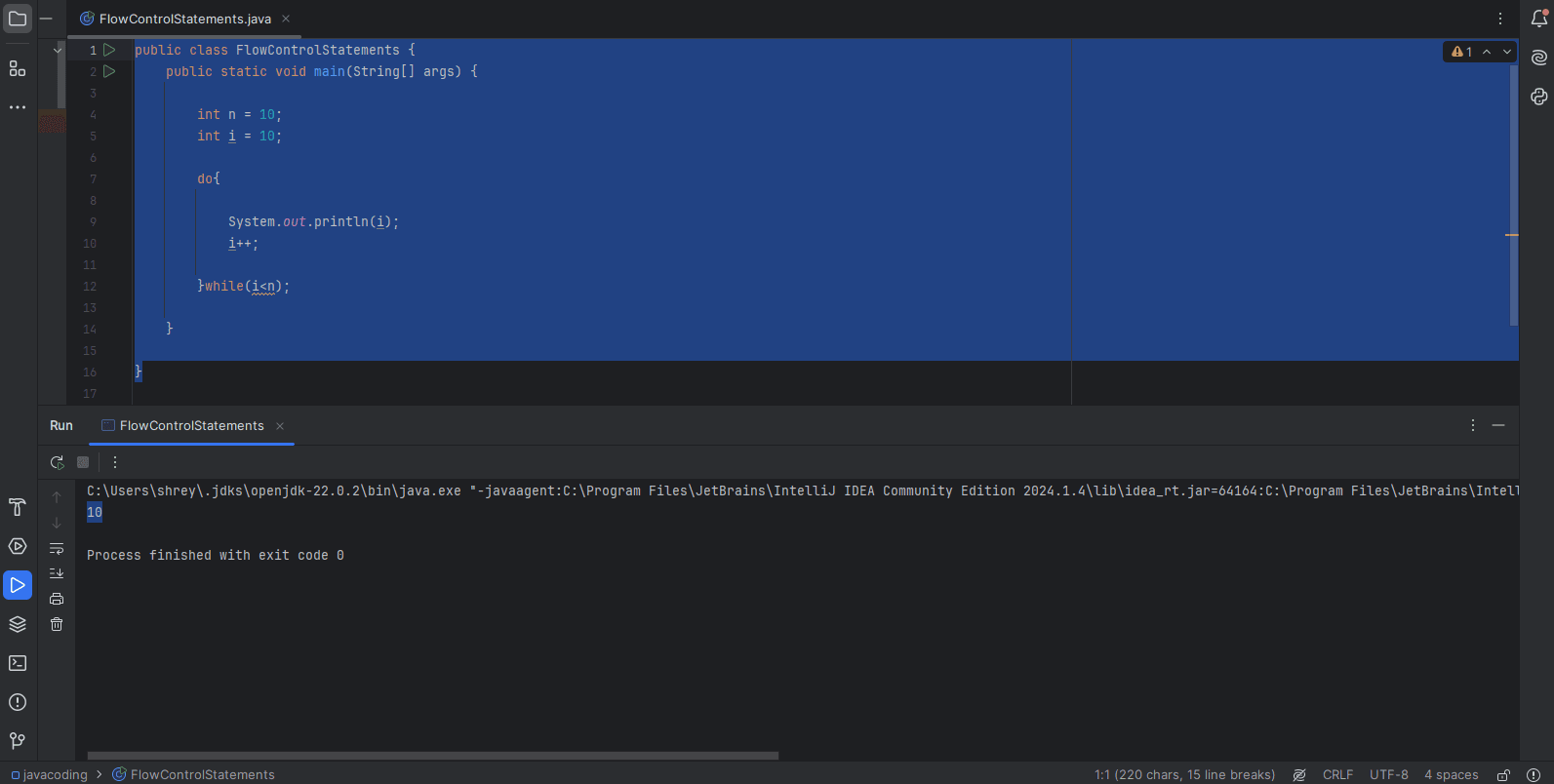
Logical Operator: “AND” (&&), “OR”(||) and “NOT”(!) are ****used to perform logical operations. Relational Operator: “==”, “!=”, “>”, “<”, “>=”, “<=”, are ****used to check the relation between two operands.
3. Jump Statements (Branching Statements):#
Jump statements (also called branching statements) in Java control the flow of execution by transferring it to another part of the program.
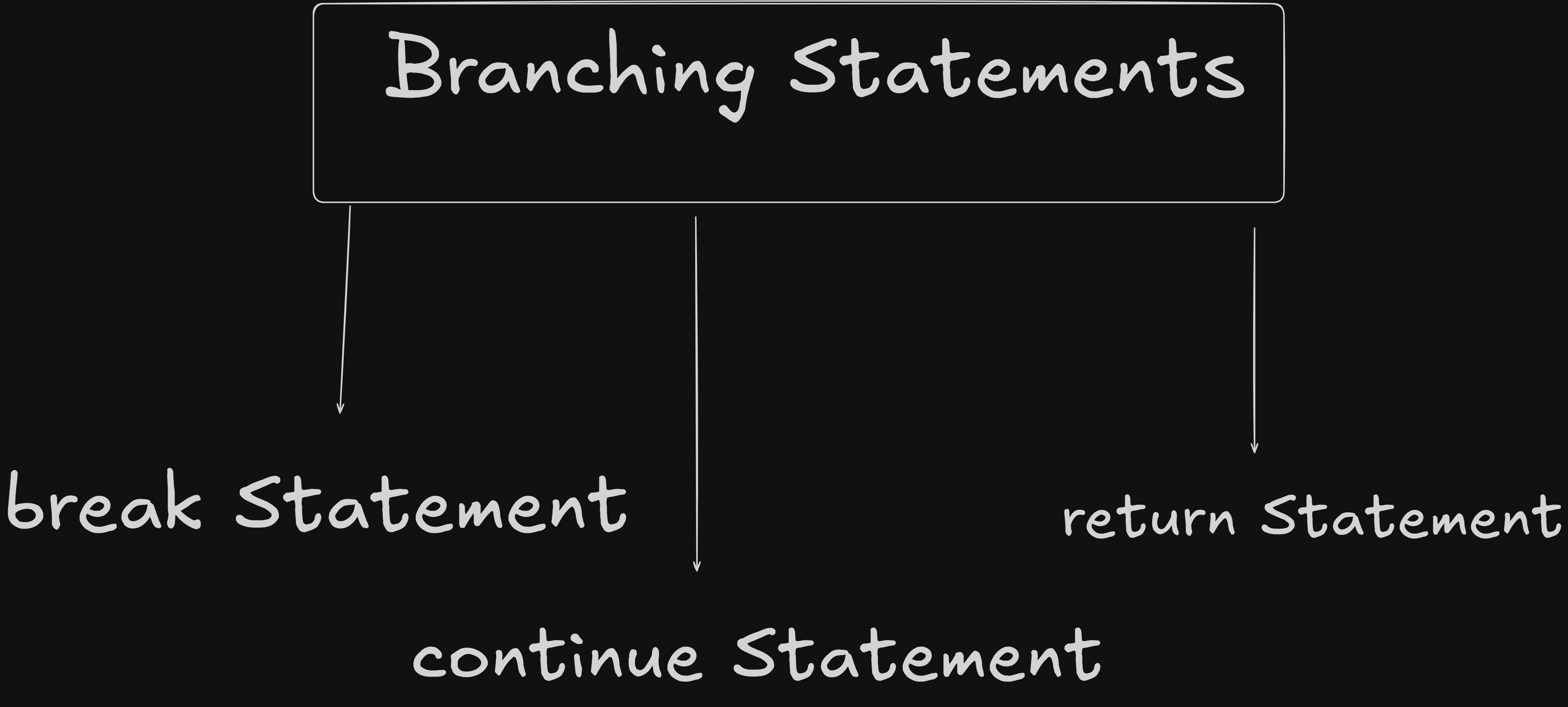
a. break
Statement (Exiting a Loop or Switch Case):#
The break
statement terminates the loop. (switch
statement immediately).
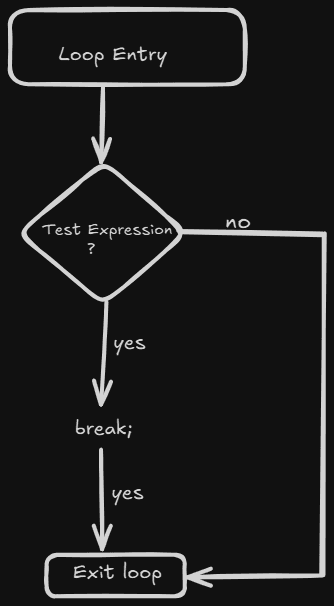
Syntax:

Output:
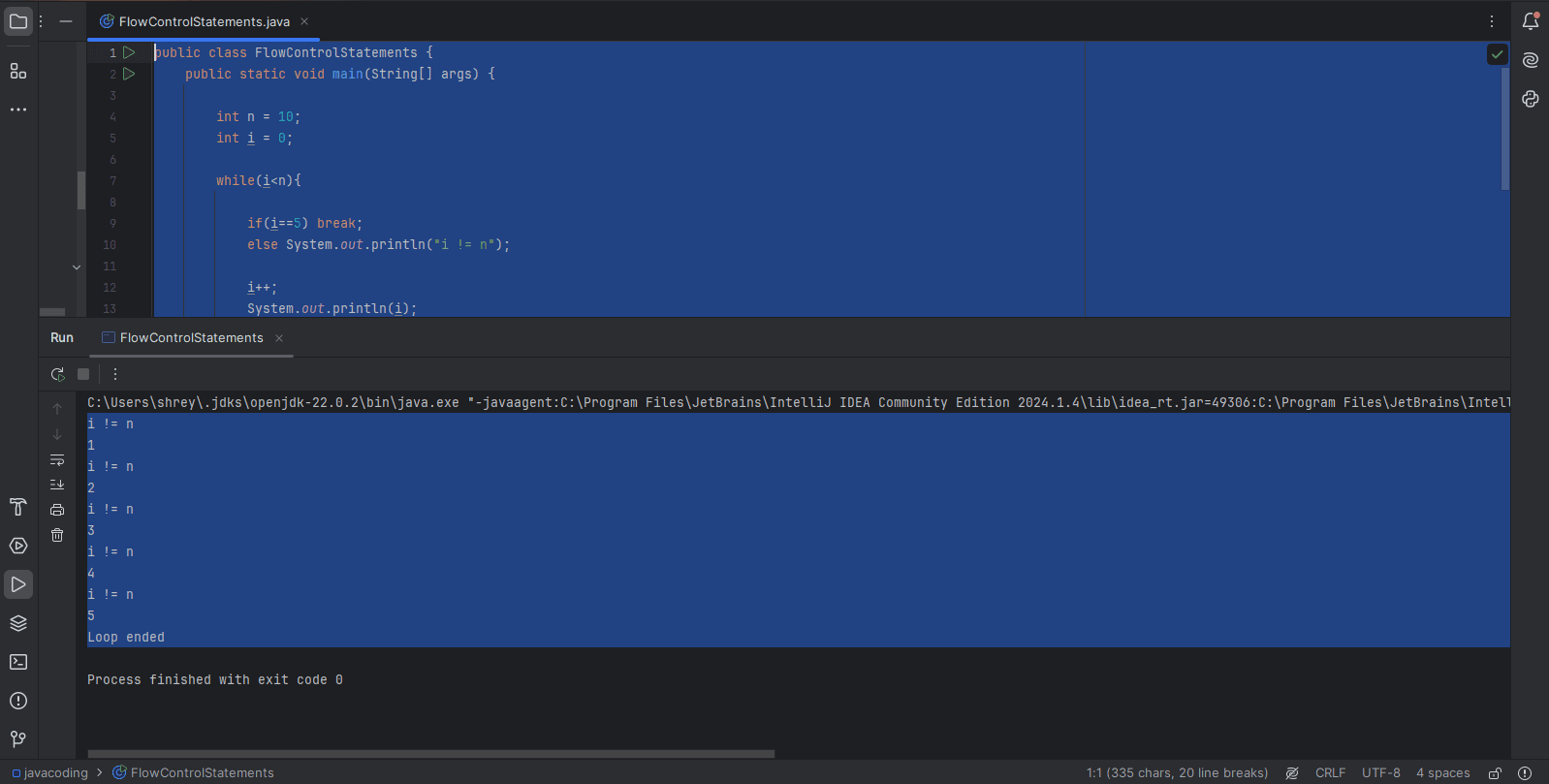
b. continue
Statement (Skip Current Iteration):#
The continue
statement skips the current iteration and moves to the next iteration of the loop.
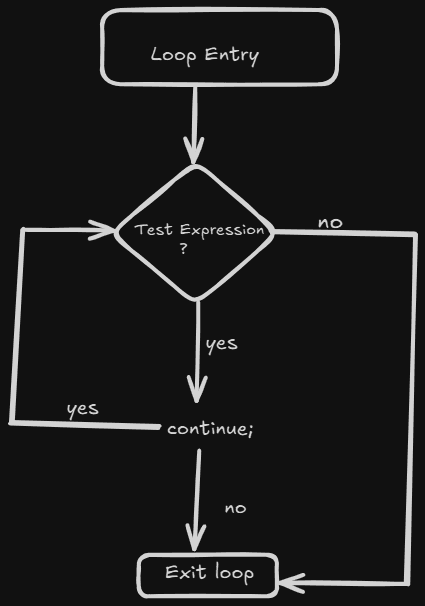
Syntax:
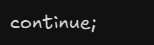
Output:
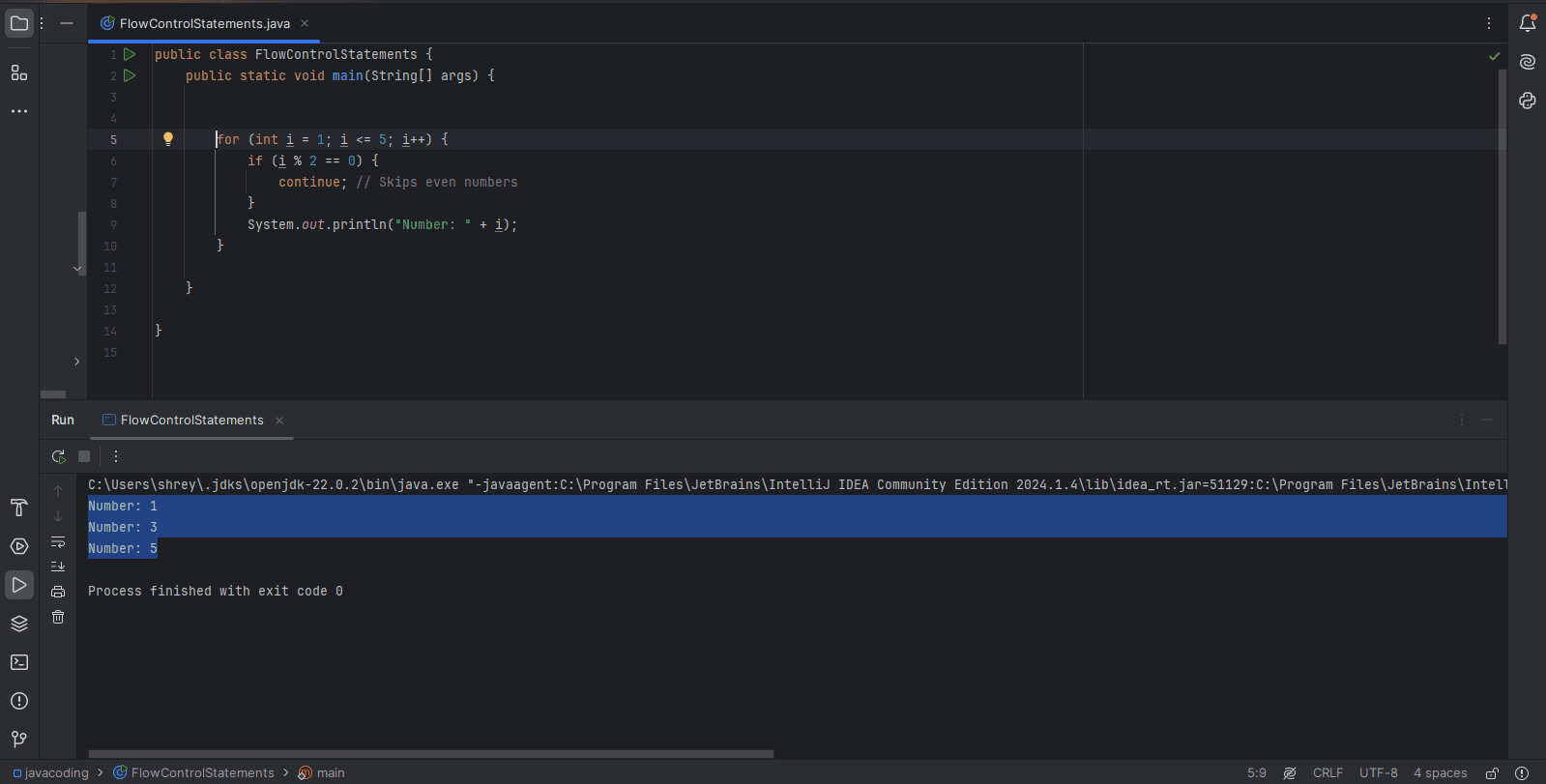
Labeled Blocks (Labeled Break and Labeled Continue):#
Labeled blocks in Java are advanced control flow techniques that allow breaking out of or continuing a specific loop when dealing with nested loops. A label in Java is an identifier followed by a colon (:
) placed before a loop. It helps in controlling which loop should be affected by break
or continue
.
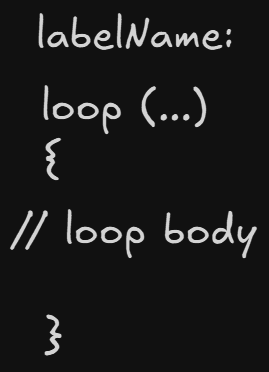
Labeled continue
Statement: Used to skip the current iteration of a specific outer loop instead of the nearest one. Regular continue
only affects the inner loop, but labeled continue
affects the outer loop.
Output:
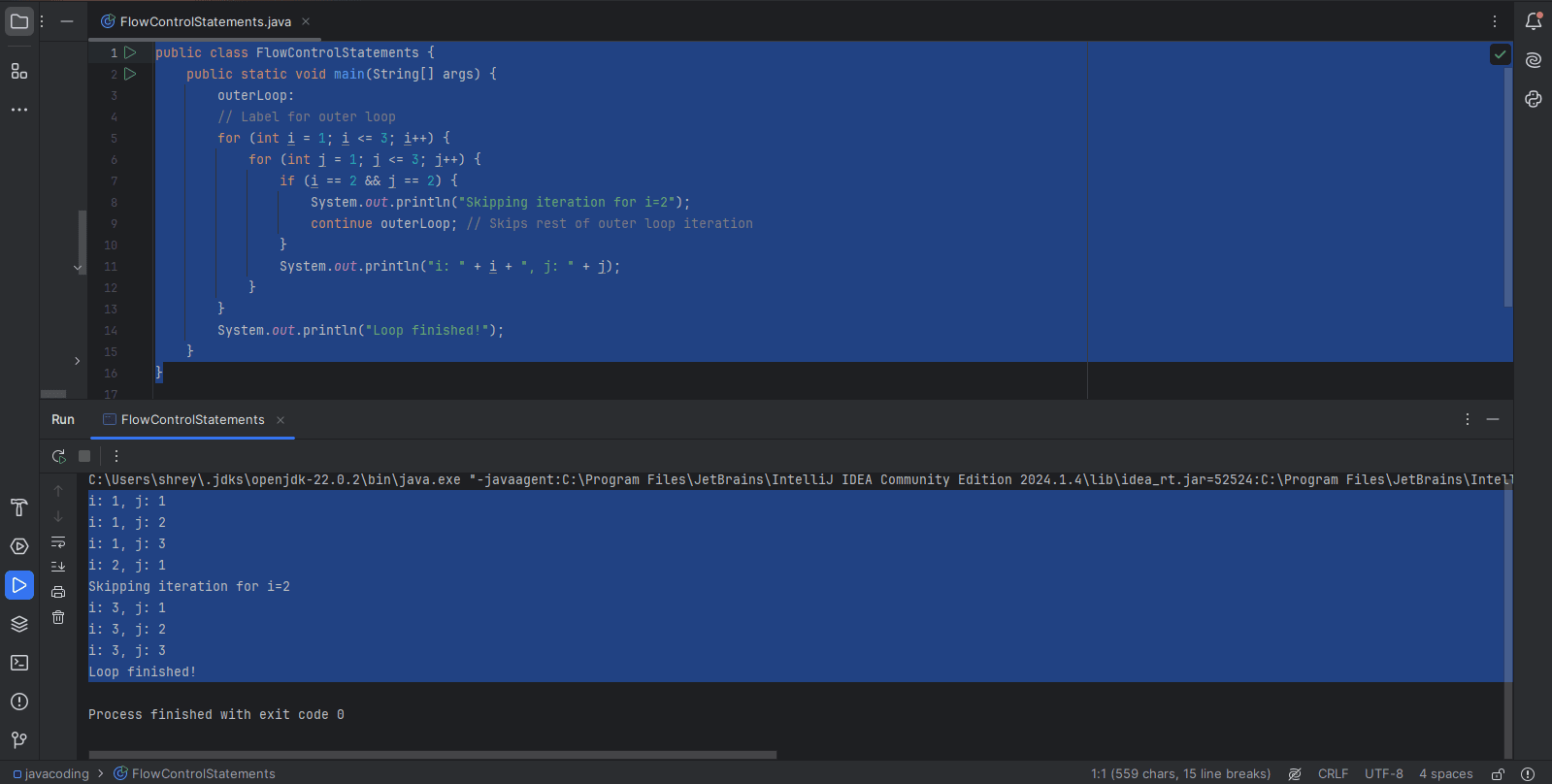
continue
outputLabeled break
Statement: Used to terminate a specific outer loop instead of just the inner loop. Regular break
only exits the nearest loop, but labeled break
exits the labeled loop.
Output:
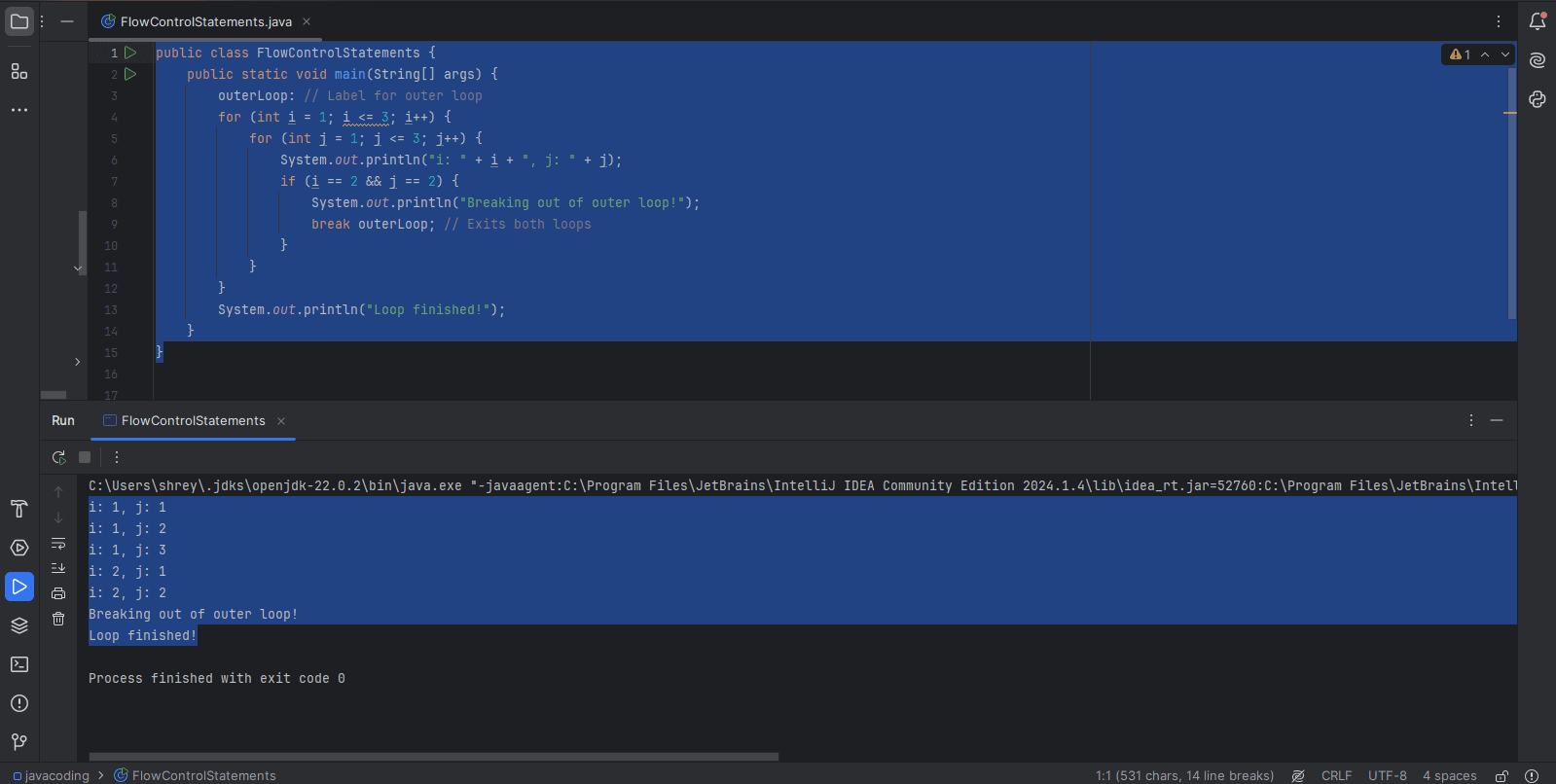
break
outputc. return
Statement (Exit from a Method):#
The return
statement terminates a method and optionally returns a value.
Output:
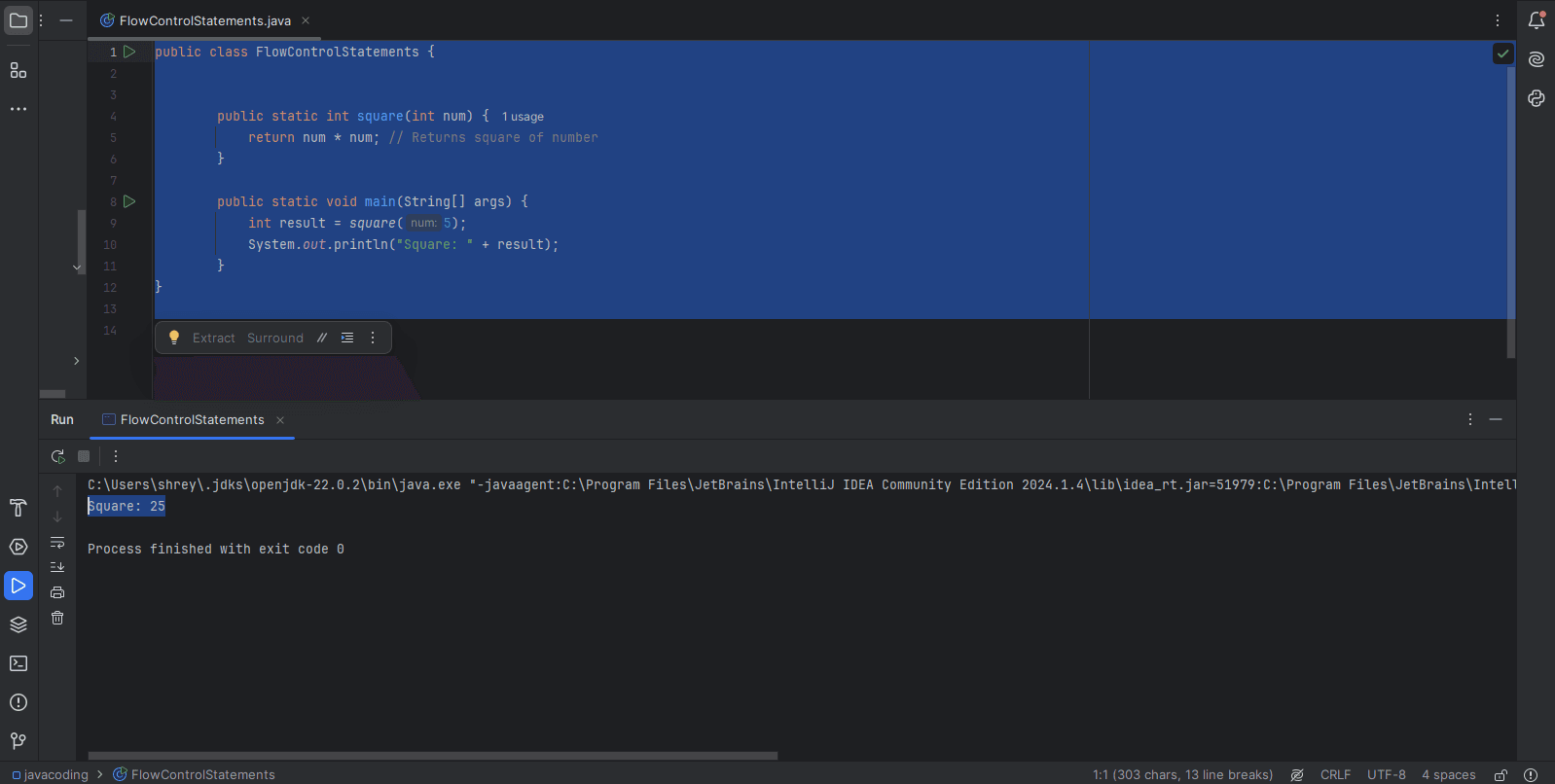
return
Statement output4. Exception Handling (Try-Catch-Finally):#
try
Block: Contains code that may throw an exception.
catch
Block: Handles specific exceptions that occur in the **try
**block.
finally
Block: Executes always, whether an exception occurs or not.
Output:
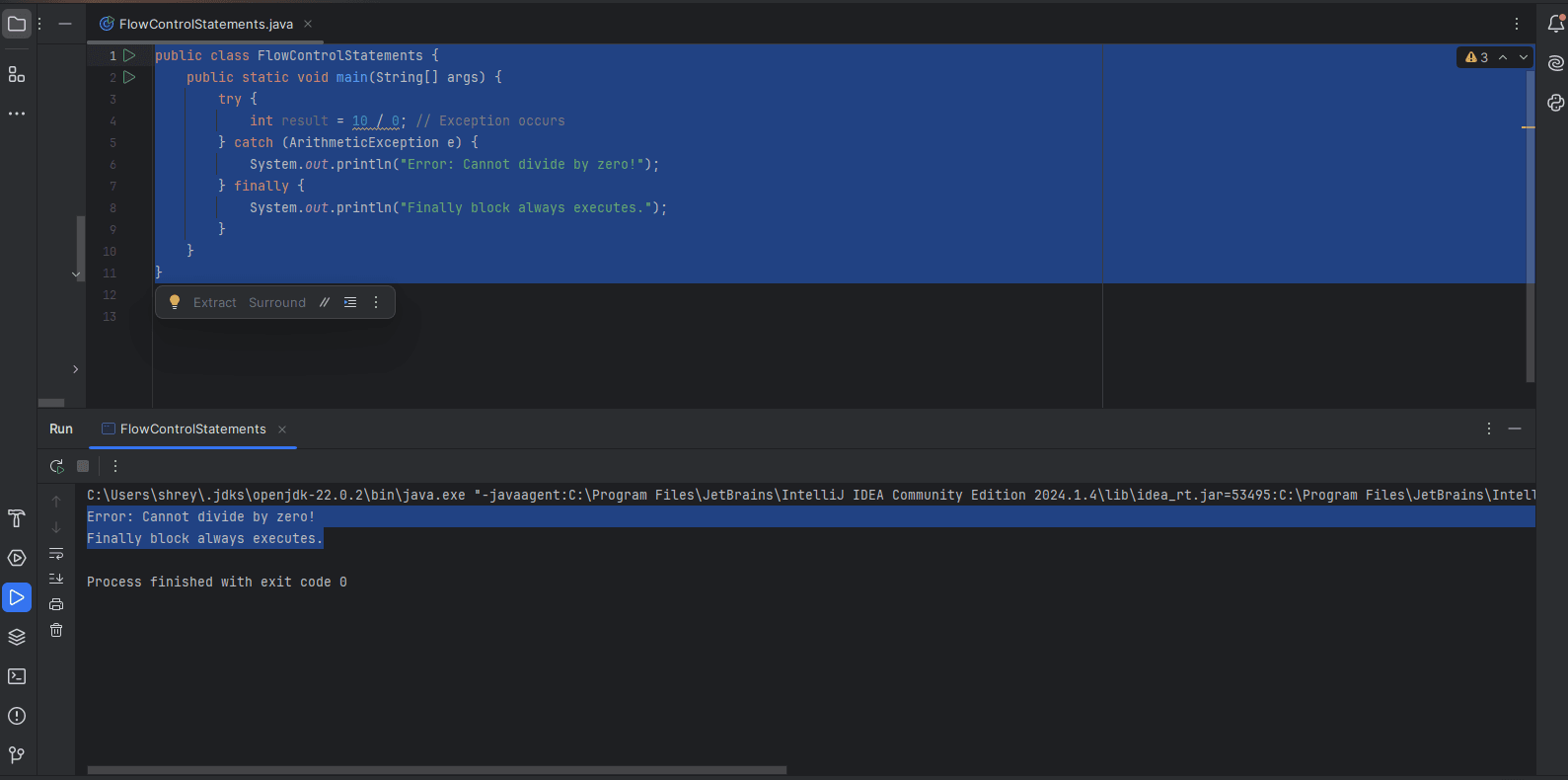
Conclusion:#
This article uses examples and syntax to explore Java flow control statements, including decision-making, looping, and branching mechanisms. Mastering these concepts enhances code efficiency, readability, and logical structuring in Java programming.