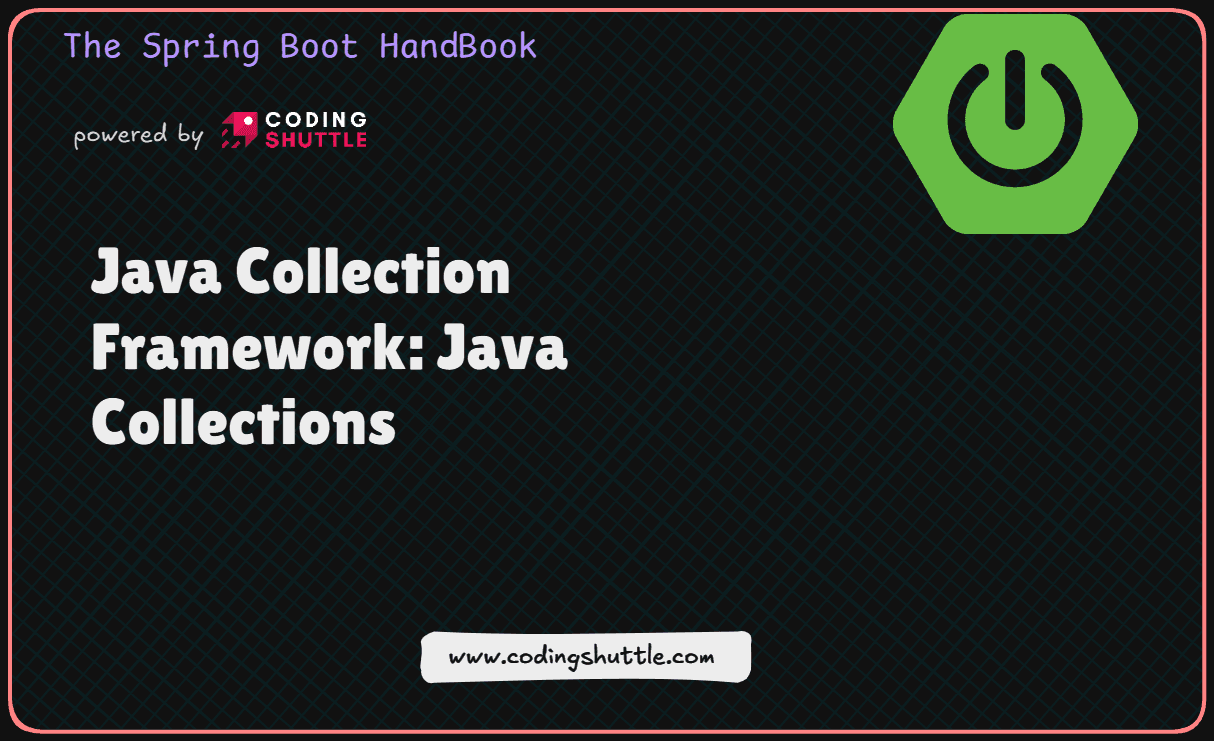
A Complete Guide to Java Collection Framework : Java Tutorial
Let's explore the key collection types like List, Set, Map, and Queue. It covers essential concepts such as iterators, sorting, searching, and thread-safe collections, helping developers efficiently manage and manipulate data in Java applications.
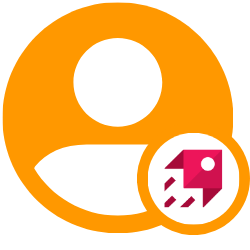
Shreya Adak
February 14, 2025
11 min read
Introduction:#
The Java Collection Framework is a comprehensive set of classes (ArrayList, LinkedList, HashSet, PriorityQueue) and interfaces (List, Set, Queue) and implementations in Java. That provides powerful data structures and algorithms for storing, handling, manipulating, and reusable-efficiently implementing collections of objects. The Java Collections are used fromjava.util.*
package.
Importance and Use Cases Of Java Collection Framework#
- It provides pre-implemented data architecture which helps to reduce programming effort.
- It offers optimized implementations that help to enhance performance.
- It provides consistent APIs across different collection types.
- For sorting (sort()), searching (
binarySearch()
), and iterating () inside the data structure, ****makes these operations easier by providing the necessary methods. - It supports concurrent collections (
ConcurrentHashMap
) for thread-safe operations. - It helps to enable cache implementations (
LinkedHashMap
) for LRU (Least Recently Used) caching. - It gives the facility of graph & tree structures by implementing graph traversal algorithms using
HashMap
andTreeSet
.
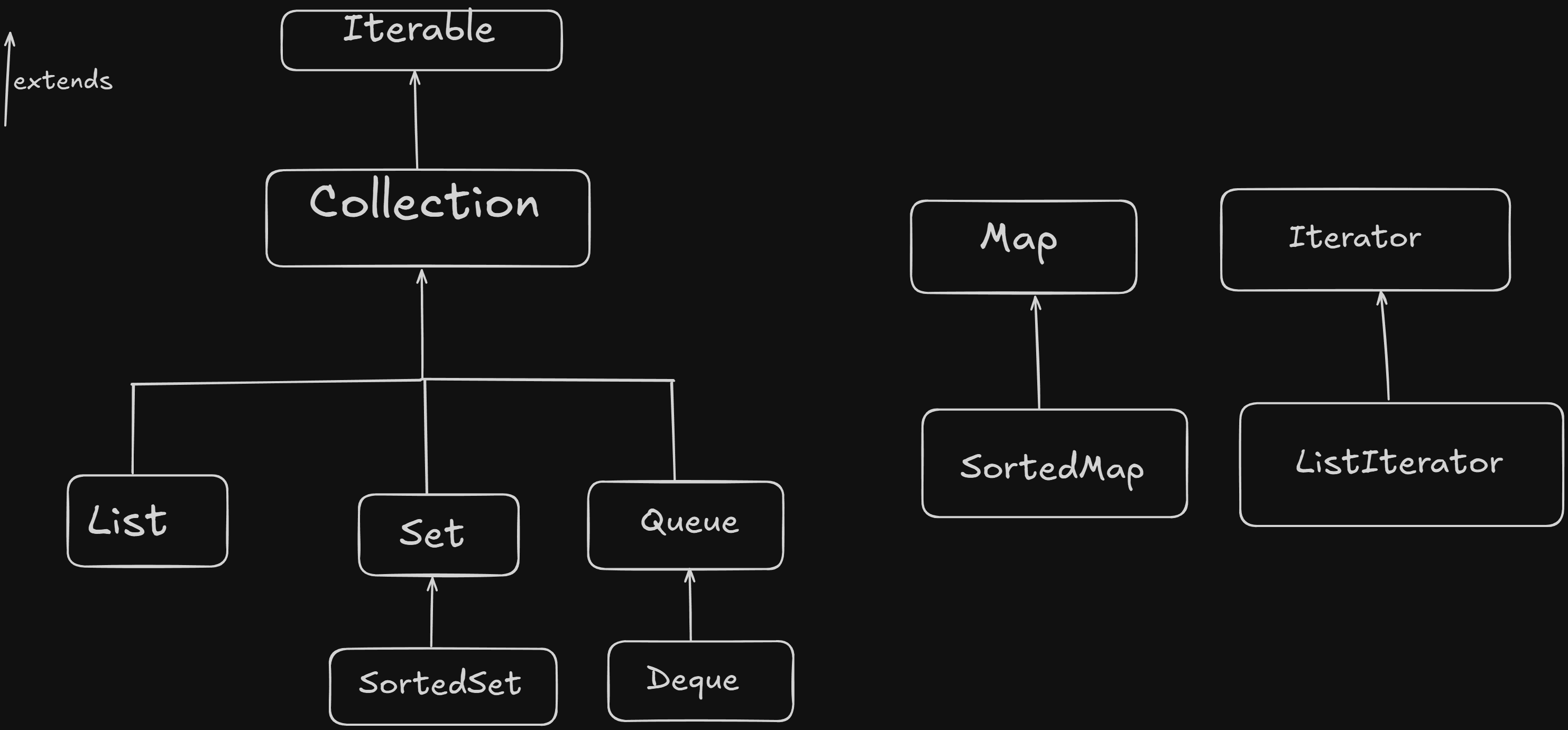
Java Collections: (Oracle_Docs_For_Inbuilt_Methods_Of_Java_Collections_Class)#
The Java Collections is a Java class. This class consists exclusively of static methods that operate on or return collections. It contains polymorphic algorithms that operate on collections, "wrappers", which return a new collection backed by a specified collection, and a few other odds and ends. The methods of this class all throw a NullPointerException if the collections or class objects provided to them are null. This class is a member of the Java Collections Framework.
Classes are user-defined blueprints for creating objects. They define fields (variables) and methods (functions) but do not occupy memory until an object is created. Objects are instances of a class that represent real-world entities. They are used to access the fields and methods defined in the class.
Java Wrapper Class And Generics:#
Java Wrapper Class:#
The Wrapper class wraps a value of the primitive type (int, long, float) int in an object. An object of type Wrapper Class contains a single field whose type is primitive.
Primitive Data types: The Java programming language is statically typed, meaning that all variables must be declared before being used. There are eight primitive data types in Java: int, float, long, double, char, boolean, byte, and short.
Why we need Wrapper Class:#
- The classes (ArrayList, Vector) of
java.util.*
Packages are mainly worked to handle Objects. - An Object is needed to support synchronization in multithreading.
Wrapper Class | Primitive Data Types |
Integer | int |
Float | float |
Long | long |
Double | double |
Character | char |
Boolean | boolean |
Byte | byte |
Short | short |
Autoboxing: The conversion process of primitive data types to their corresponding wrapper class that is ‘long’ to ‘Long’.
Unboxing: This is the reverse process of the Autoboxing process. The conversion process of a wrapper class to primitive data types that is ‘Long’ to ‘long’.
Generics: Parameterized Typed#
By using generics, it is possible to create classes, interfaces, and methods that work for different data types. Generics are used to prevent ClassCastException
by enforcing type checks at compile-time.
Bounded Generic Types:#
Generic classes can only work with children’s data type of Number
class (e.g. Integer, Float, etc.). You can not use it for primitive data types.
Number
class: The abstract class Number is the superclass of platform classes representing numeric values that are convertible to the primitive types byte, double, float, int, long, and short.
Java Iterable Interface: (Oracle_Docs_Jdk8_For_Inbuilt_Methods_Of_Iterable_Interface)#
Implementing this interface allows an object to be the target of the enhanced for statement (sometimes called the "for-each loop" statement). It performs the given action for each element of the Iterable until all elements have been processed or the action throws an exception.
Interfaces are introduced for achieving abstraction and multiple inheritance. It provides full abstraction. Objects cannot be created directly from interfaces; at least one class must implement them.
- Abstract Methods – Implicitly
public
andabstract
, declared without an implementation.- Default Methods (Java 8+) – Have an implementation and can be overridden.
- Static Methods (Java 8+) – Have an implementation but cannot be overridden.
- Private Methods (Java 9+) – Used for code reuse within the interface but not accessible outside.
Java Collection Interfaces: (Oracle_Docs_Jdk8_For_InBuilt_Methods_Of_ Java_Collection_Interface)#
The root interface in the collection hierarchy. A collection represents a group of objects known as its elements. Some collections allow duplicate elements, and others do not. Some are ordered, and others are unordered. Collections that have a defined encounter order are generally subtypes of the SequencedCollection interface. The JDK does not provide any direct implementations of this interface; it provides implementations of more specific sub-interfaces like Set and List. This interface is typically used to pass collections around and manipulate them where maximum generality is desired. This interface is a member of the Java Collections Framework.
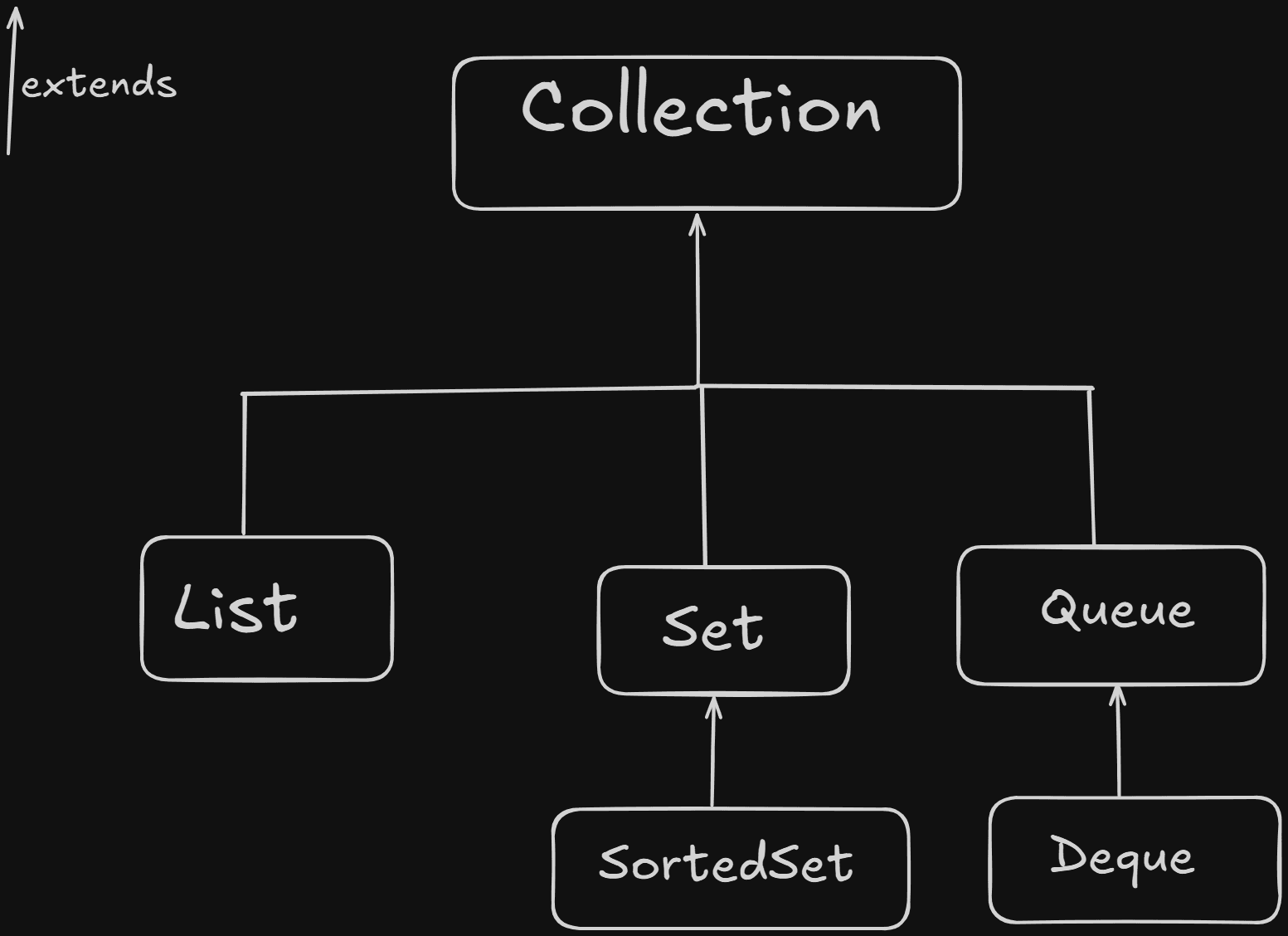
Java List Interface: (Oracle_Docs_Jdk8_For_In_Built_Methods_Of_List_Interface)#
An ordered collection, where the user has precise control over where in the list each element is inserted. Unlike sets, lists typically allow duplicate elements. The List interface provides four methods for positional (indexed) access to list elements. Lists (like Java arrays) are zero-based.
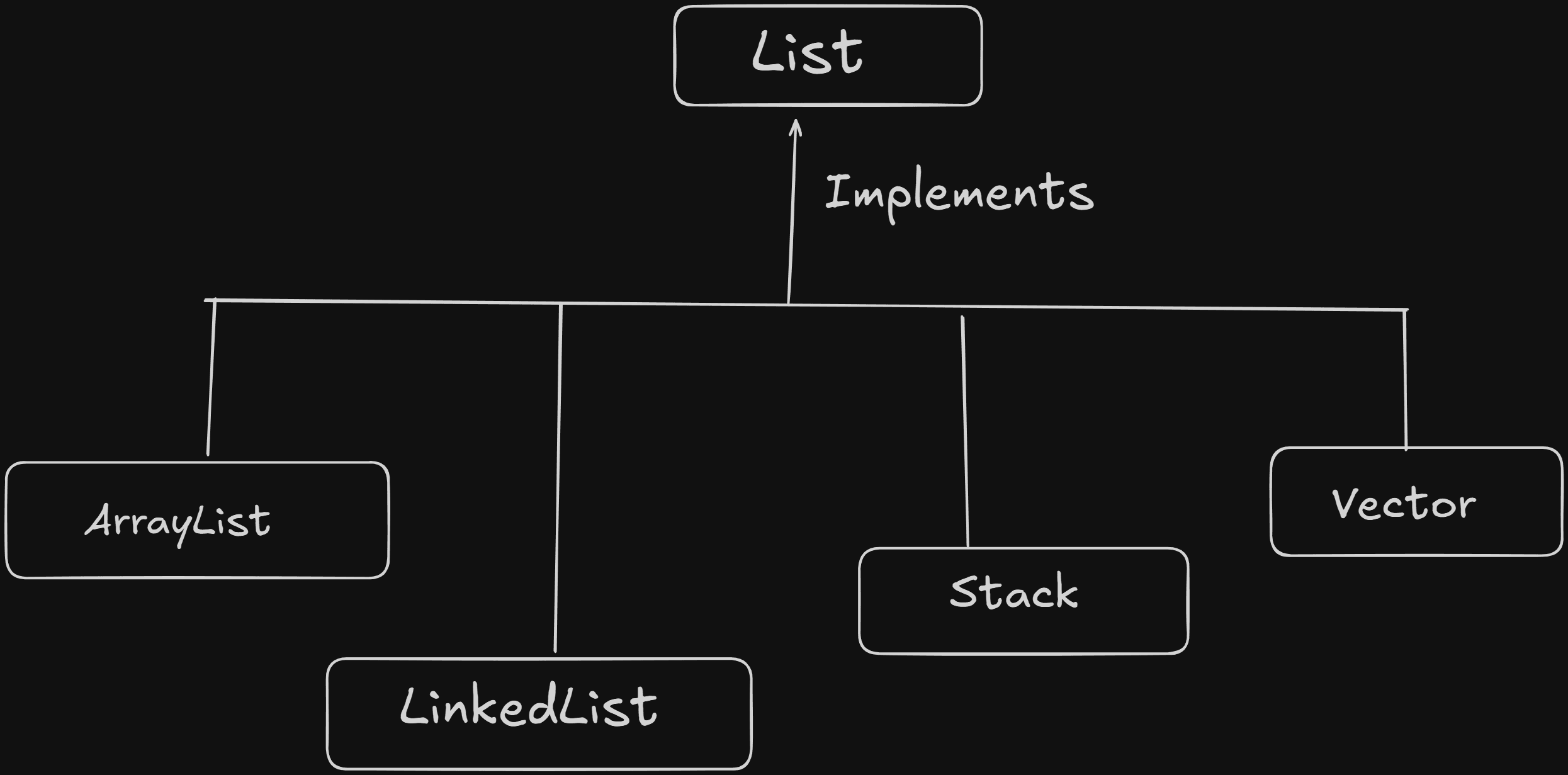
- ArrayList or Resizable-array implementation of the List interface. Each ArrayList instance has a capacity. The capacity is the size of the array used to store the elements in the list. It is always at least as large as the list size. As elements are added to an ArrayList, its capacity grows automatically. This implementation is not synchronized. Internal working of ArrayList: Initially, Array has a certain capacity, and elements are added and filled up. When the capacity is reached, the ArrayList creates a new larger array and copies the elements from the old array to the new one. This process of resizing and coping is transparent to the user.
- LinkedList (Doubly-linked list) implementation of the List and Deque interfaces. This implementation is not synchronized.
- Stack represents a last-in-first-out (LIFO) stack of objects.
- Vector implements a growable array of objects. Like an array, it contains components that can be accessed using an integer index. However, the size of a Vector can grow or shrink as needed to accommodate adding and removing items after the Vector has been created.
Java Set Interface:#
(Oracle_Docs_Jdk8_For_Inbuilt_Methods_Of_Set_Interface)
A collection that contains no duplicate elements. All basic operations execute in constant time.
- HashSet: This class implements the Set interface, backed by a hash table (actually a HashMap instance). It makes no guarantees as to the iteration order of the set; in particular, it does not guarantee that the order will remain constant over time.
- EnumSet: A specialized Set implementation for use with enum types. All of the elements in an enum set must come from a single enum type that is specified, explicitly or implicitly, when the set is created.
- LinkedHashSet: Hash table and linked list implementation of the Set interface, with well-defined encounter order.
- TreeSet: A NavigableSet implementation based on a TreeMap. The elements are ordered using their natural ordering.
Java Queue Interface: (Oracle_Docs_Jdk8_For_Inbuilt_Methods_Of_Queue_Interface)#
A collection designed for holding elements before processing in order elements in a FIFO (first-in-first-out) manner.
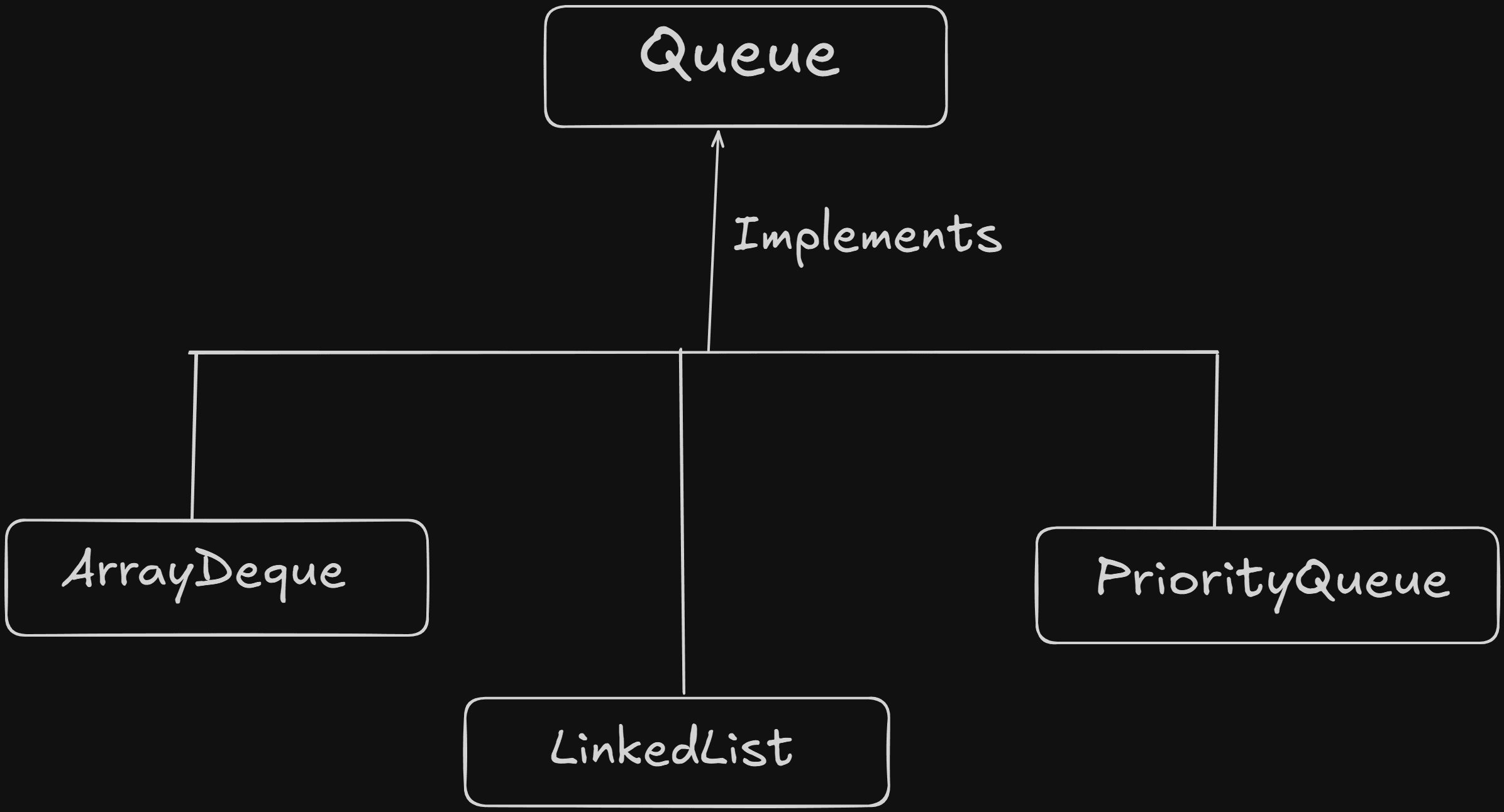
- ArrayDeque: Resizable-array implementation of the Deque interface. Array deques have no capacity restrictions; they grow as necessary to support usage.
- LinkedList: Doubly-linked list implementation of the List and Deque interfaces. Implements all optional list operations, and permits all elements (including null).
- PriorityQueue: An unbounded priority queue based on a priority heap. The elements of the priority queue are ordered according to their natural ordering, or by a Comparator provided at queue construction time,
Java Map Interface:(Oracle_Docs_Jdk8_For_Inbuilt_Methods_Of_Map_Interface)#
An object that maps keys to values. A map cannot contain duplicate keys; each key can map to at most one value. The Map interface provides three collection views, which allow a map's contents to be viewed as a set of keys, a collection of values, or set of key-value mappings.
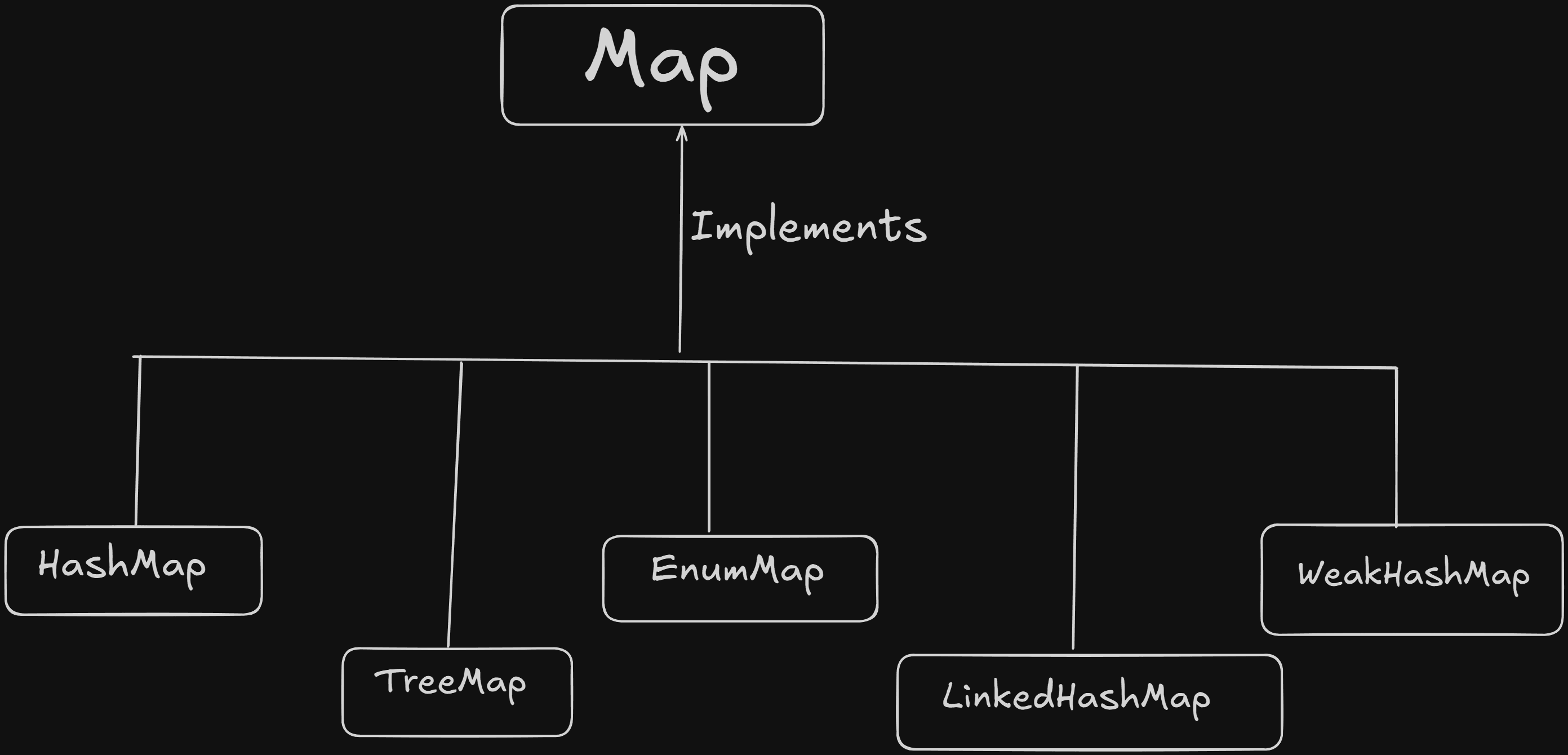
- HashMap: Hash table-based implementation of the Map interface. This implementation provides all of the optional map operations and permits null values and the null key.
- TreeMap: A Red-Black tree-based NavigableMap implementation. The map is sorted according to the natural ordering of its keys.
- EnumMap: A specialized Map implementation for use with enum type keys. All of the keys in an enum map must come from a single enum type that is specified, explicitly or implicitly, when the map is created.
- LinkedHashMap: Hash table and linked list implementation of the Map interface, with well-defined encounter order. This implementation differs from HashMap in that it maintains a doubly-linked list running through all of its entries.
- WeakHAshMap: Hash table based implementation of the Map interface, with weak keys. An entry in a WeakHashMap will automatically be removed when its key is no longer in ordinary use.
Java Iterator Interface: (Oracle_Docs_Jdk8_For_Inbuilt_Methods_Of_Iterator_Interface)#
An iterator over a collection. Iterator takes the place of Enumeration in the Java Collections Framework. Iterators allow the caller to remove elements from the underlying collection during the iteration with well-defined semantics.
Concurrent Collections in Java:#
Concurrent collections in Java (java.util.concurrent
) provide thread-safe alternatives to traditional collections, preventing issues like ConcurrentModificationException
while improving performance in multi-threaded environments.
Key Concurrent Collections:#
CopyOnWriteArrayList
– A thread-safeArrayList
that creates a new copy on modification, ideal for read-heavy scenarios.ConcurrentHashMap
– A thread-safeHashMap
that uses segmentation for better performance.ConcurrentLinkedQueue
– A non-blocking, thread-safe queue based on linked nodes.BlockingQueue
(ArrayBlockingQueue
,LinkedBlockingQueue
) – Used in producer-consumer patterns, blocks when full/empty.ConcurrentSkipListMap
– A thread-safe, sortedTreeMap
alternative using a skip list algorithm.
Comparable & Comparator: (Java 8)#
Comparator: A comparison function, which imposes a total ordering on some collection of objects. Comparators can be passed to a sort method (such as Collections. sort or Arrays. sort) to allow precise control over the sort order. Comparators can also be used to control the order of certain data structures (such as sorted sets or sorted maps) or to provide an ordering for collections of objects that don't have a natural ordering. The ordering imposed by a comparator c on a set of elements S is said to be consistent with equals if and only if c. compare(e1, e2)==0 has the same boolean value as e1.equals(e2) for every e1 and e2 in S.
Usage: Collections.*sort*(list, new NameComparator());
Comparable: This interface imposes a total ordering on the objects of each class that implements it. This ordering is referred to as the class's natural ordering, and the class's compareTo method is referred to as its natural comparison method. Lists (and arrays) of objects that implement this interface can be sorted automatically by Collections. sort (and Arrays. sort). Objects that implement this interface can be used as keys in a sorted map or as elements in a sorted set, without the need to specify a comparator. The natural ordering for a class C is said to be consistent with equals if and only if e1.compareTo(e2) == 0 has the same boolean value as e1.equals(e2) for every e1 and e2 of class C. Note that null is not an instance of any class, and e. compareTo(null) should throw a NullPointerException even though e. equals(null) returns false.
- Usage:
List<Student> list = new ArrayList<>();
list.add(new Student(2, "Alice"));
list.add(new Student(1, "Bob"));
Collections.*sort*(list);
Java Collection Performance Optimization:#
- Choose the Right Collection Type – Use
ArrayList
for fast lookups,LinkedList
for frequent insertions,HashMap
for fast key-value access, andTreeSet/TreeMap
for sorted data. - Optimize Initial Capacity & Load Factor – Avoid frequent resizing by setting an initial capacity (
new ArrayList<>(1000)
,new HashMap<>(16, 0.75f)
). - Use Efficient Iteration – Prefer enhanced for-loops or iterators for modifications.
- Use Concurrent Collections – For multi-threading, use
ConcurrentHashMap
,CopyOnWriteArrayList
, andConcurrentLinkedQueue
. - Use Unmodifiable Collections – Reduce memory usage with
Collections.unmodifiableList()
. - Leverage Java 8 Streams & Parallel Processing – Use
parallelStream()
for large collections but avoid for small ones. - Avoid Auto-Boxing – Use primitive arrays (
int[]
) instead ofArrayList<Integer>
. - Use Efficient Sorting – Utilize
Collections.sort()
orArrays.parallelSort()
for large datasets. - Remove Elements Efficiently – Use
filter()
instead ofremoveIf()
to avoid performance overhead. - Avoid Redundant Collections – Use
Set
to remove duplicates efficiently.
Conclusion:#
This article provides an overview of the Java Collection Framework, which offers pre-implemented data structures like List
, Set
, Queue
, and Map
to store and manipulate collections of objects. It highlights key components such as interfaces, classes, and wrapper classes, along with the benefits of using generics, autoboxing, and thread-safe collections. The framework simplifies working with collections, ensuring optimized performance and consistency in Java applications.