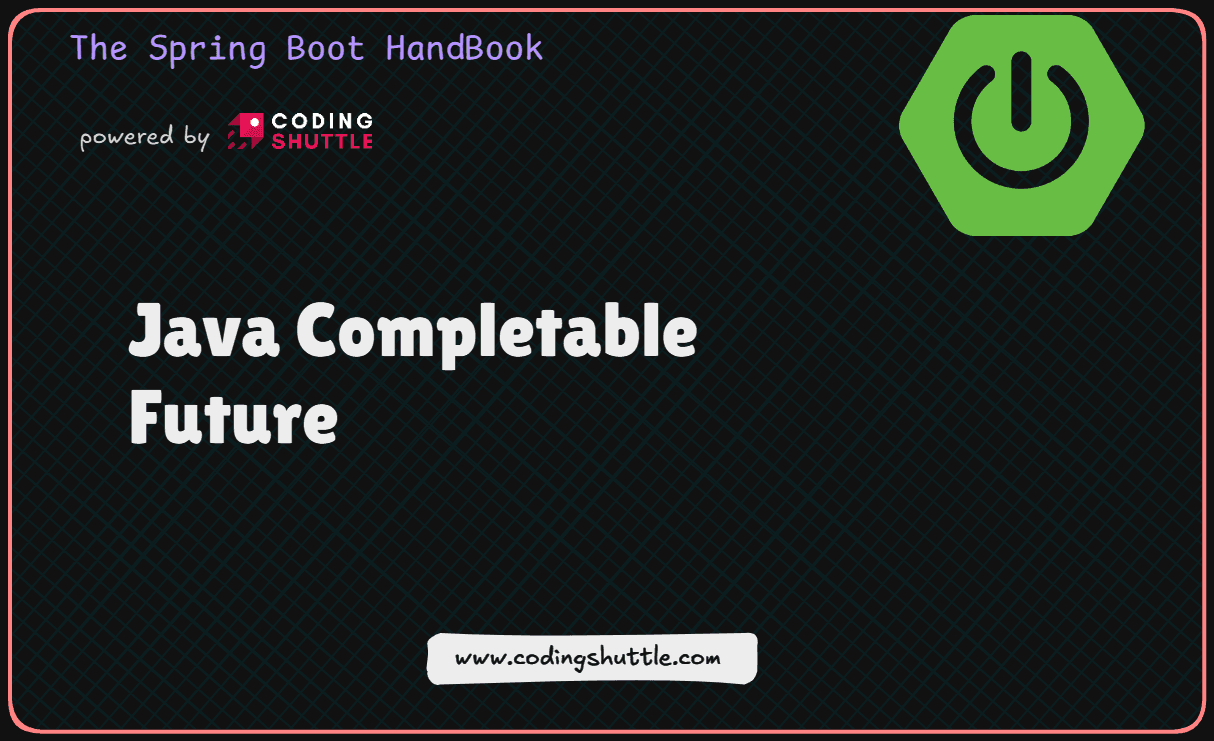
A Comprehensive Guide To Java Completable Future
This article explores CompletableFuture in Java, a key feature introduced in Java 8 for simplifying asynchronous programming. It covers how CompletableFuture enables non-blocking execution, task composition, and parallel task handling, making it ideal for creating scalable, high-performance applications.
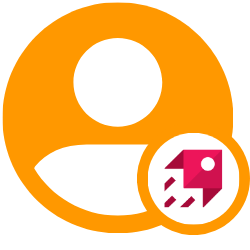
Shreya Adak
February 14, 2025
8 min read
Introduction:#
CompletableFuture is a class in Java's java.util.concurrent
package that Java 8 brought in. This class has an impact on asynchronous programming. It lets you create code that doesn't block, runs at the same time, and responds to events in a way that's easier to read and more effective. You can finish it yourself, or it can finish on its own when the work is done. It gives you a Supplier instance as a lambda expression that does the math and gives back the answer.
In Synchronous programming, each job runs one after the other. This means the program waits for one job to end before it starts the next one.
Asynchronous programming lets tasks run out of order, allowing multiple operations to happen at the same time or without waiting or blocking. This approach boosts productivity, makes systems more responsive, and helps them grow, especially in apps that need to handle many tasks at once. If you're creating web servers, mobile apps, or apps that work with lots of data, asynchronous programming helps you build systems that are effective, quick, and able to handle growth.
Evolution of Asynchronous Programming in Java#
- Pre-Java 5 (Manual Threads & Runnables)
- Used
Thread
andRunnable
to run tasks asynchronously. - Issues: Manual thread management, resource-heavy, no direct return values.
- Used
- Java 5 (
Future
andExecutorService
)- Introduced
Future
for handling async tasks withExecutorService
. - Issues:
get()
blocks execution, no easy chaining, and poor exception handling.
- Introduced
- Java 7 (Fork/Join Framework)
- Optimized parallel execution for CPU-intensive tasks.
- Issues: Complex API, not ideal for I/O operations.
- Java 8 (
CompletableFuture
)- Fully non-blocking, supports method chaining (
thenApply()
,thenCombine()
). - Built-in exception handling (
exceptionally()
,handle()
). - Allows combining multiple async tasks efficiently.
- Solves all previous limitations, making Java async programming more scalable.
- Fully non-blocking, supports method chaining (
Future in Java: Future<T> is an interface of java from Java's
java.util.concurrent
package which is used to handle asynchronous computations. It checks that the task is done properly or canceled.
Key Features of Completable
:#
Asynchronous Task Management: Allows tasks to run the main program independently without waiting or blocking.
Flexible Completion Handling: Provides methods (e.g. thenRun
, thenApply
, and thenAccept
) to handle task results once completed.
Exception Handling: Enables to manage exceptions (that occur at the time of asynchronous execution).
Task Combination: Supports to combine multiple tasks using methods (e.g. thenCombine
and allOf
).
Implementation of CompletableFuture in Java:#
CompletableFuture
allows for powerful, non-blocking asynchronous programming in Java. It provides:
- Easy chaining of tasks.
- Exception handling for asynchronous operations.
- Handling multiple asynchronous tasks (using
allOf()
,anyOf()
, andthenCombine()
). - Custom execution via Executors.
- Efficient parallel task execution.
By leveraging CompletableFuture
, Java developers can build more responsive, scalable, and efficient applications.
1. Basic CompletableFuture Example using: Creating a CompletableFuture Class#
Create an object/instance of the CompletableFuture class using *supplyAsync*()
which is a supplier. This supplier provides a functional interface that only returns a value.
Output:
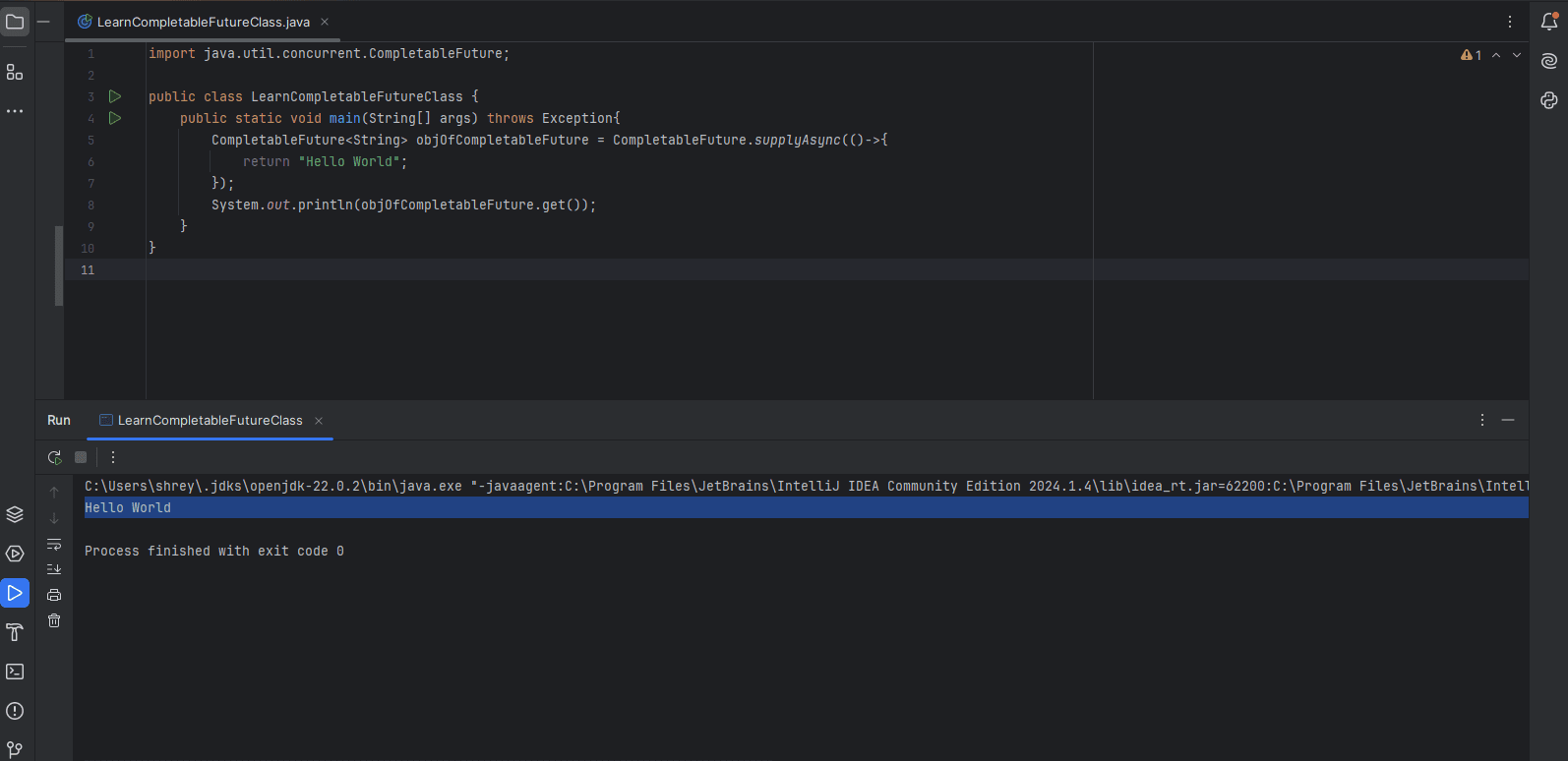
2. Chaining Tasks with CompletableFuture#
One of the most powerful features of CompletableFuture
is chaining multiple tasks together. This is useful when you need to process results step-by-step or handle a series of dependent tasks.
thenApplyAsync()
: Allows chaining additional computations.thenAccept()
: Consumes the final result after all computations.
Output:
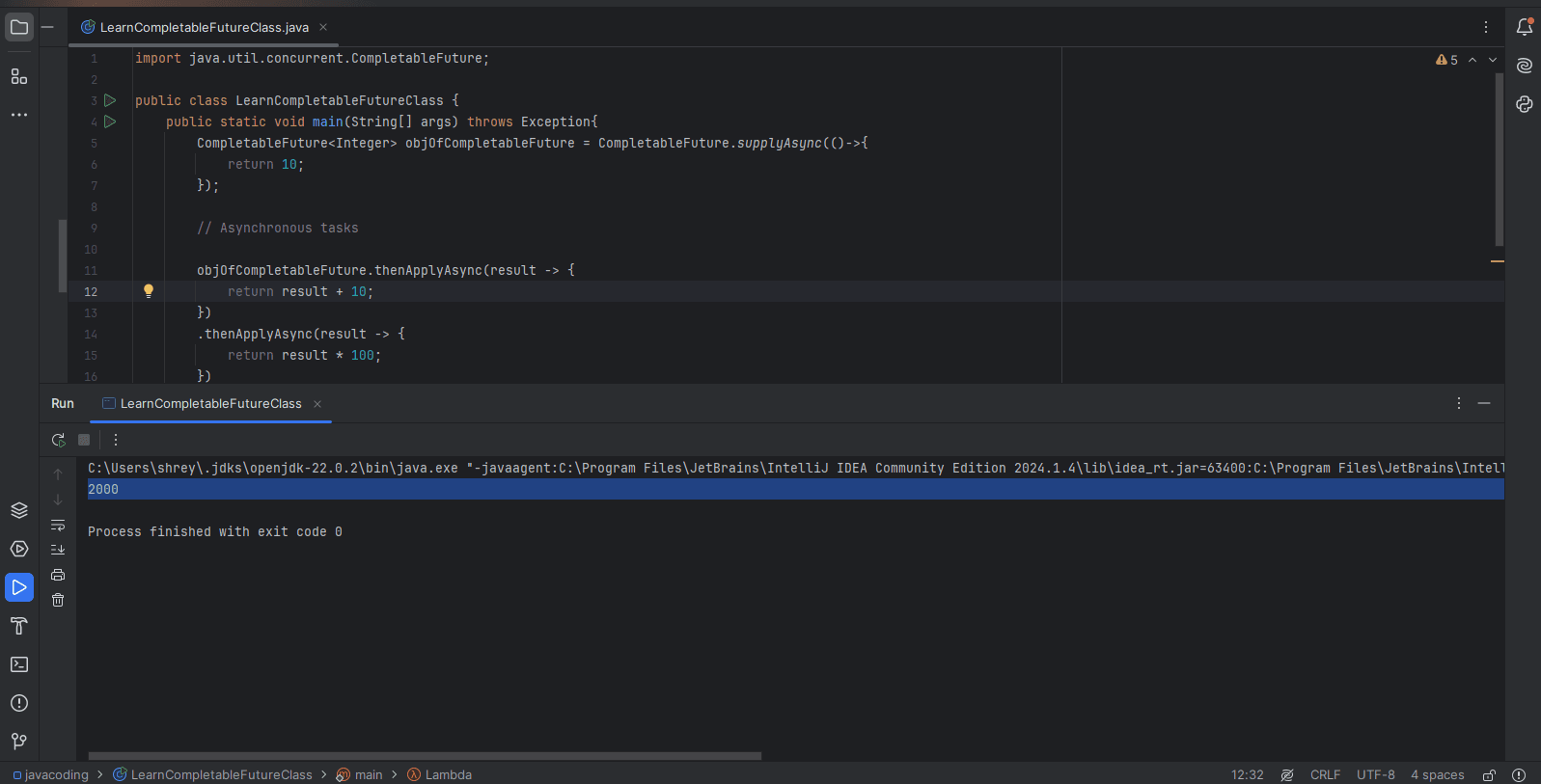
3. Handling Multiple CompletableFutures with allOf()
, anyOf()
#
Multiple CompletableFuture tasks are executed parallelly. You can use anyOf()
or allOf()
to wait for the completion of multiple futures.
allOf()
: Returns a new CompletableFuture when all tasks are done successfully otherwise if any of task fails then it throws an exception.anyOf()
: Returns when any one of the provided futures completes.
Output:
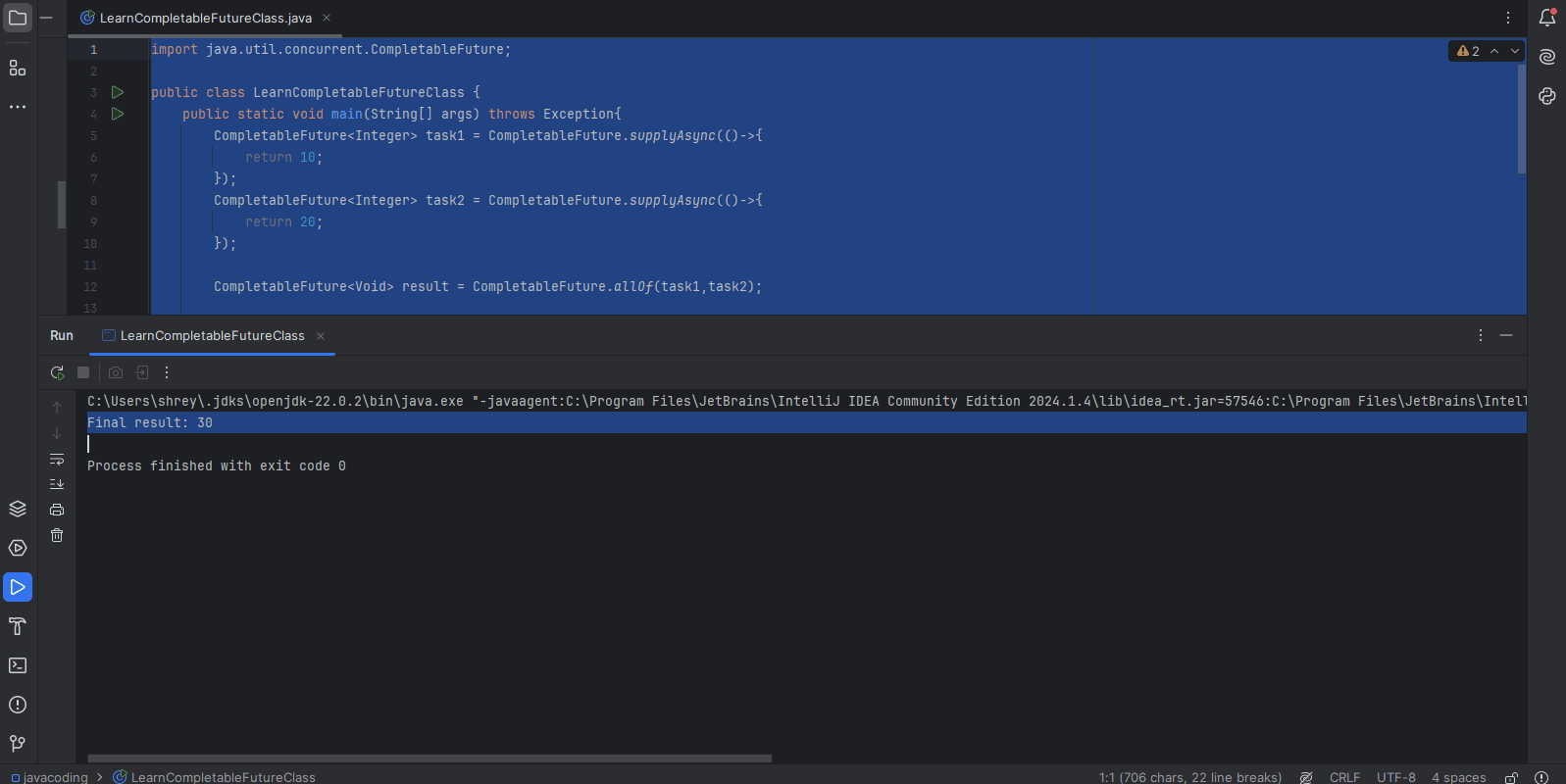
allOf()
, anyOf()
4. Handling Exceptions in CompletableFuture#
Exception handling in asynchronous tasks is easier with CompletableFuture
. You can use methods like exceptionally()
, handle()
, or whenComplete()
to deal with errors gracefully.
exceptionally()
: Handles exceptions by providing a fallback value.handle()
: Handles both success and failure, allowing transformation of results.whenComplete()
: Executes a callback after completion without modifying the result.
Output:
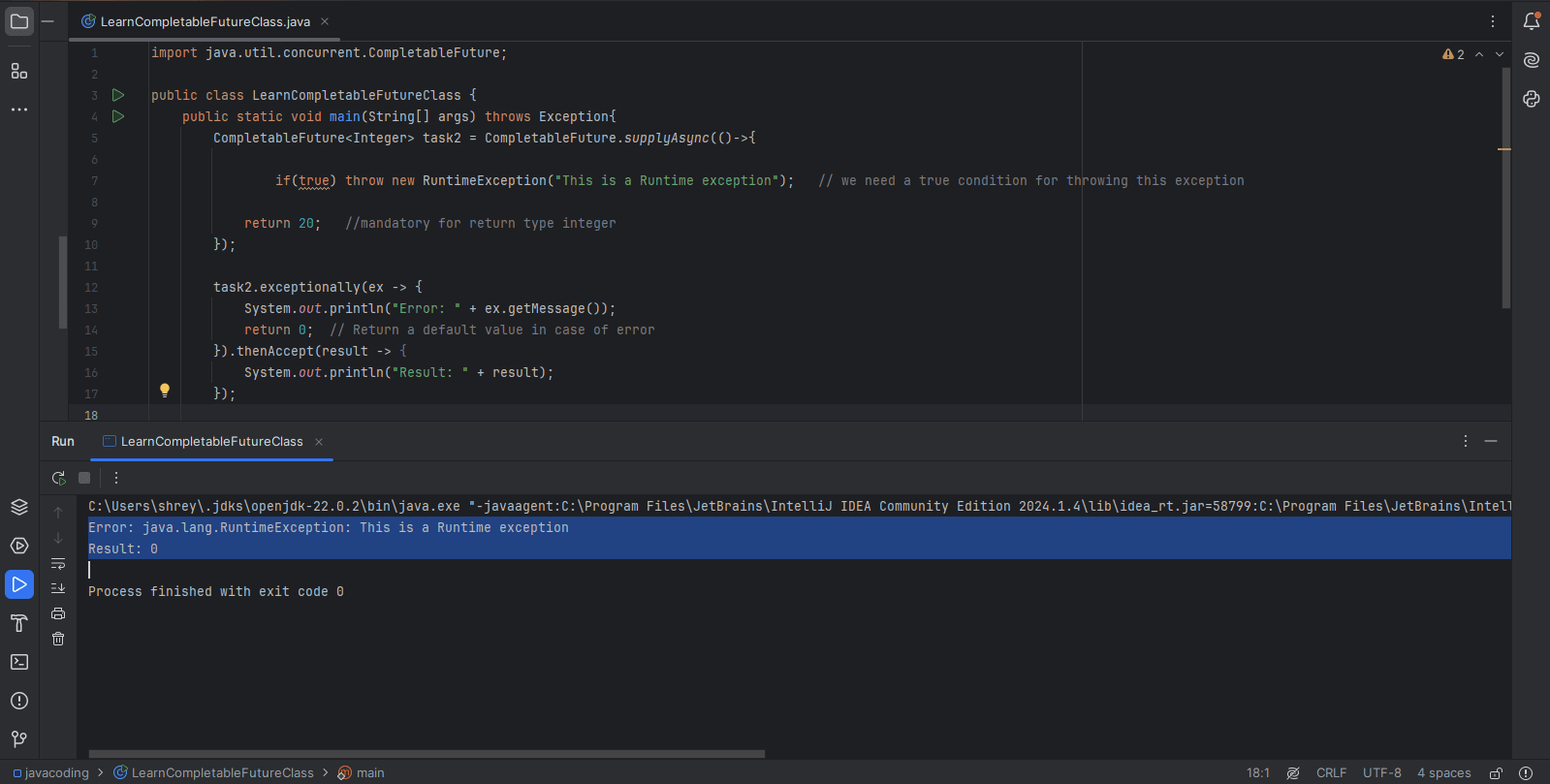
5. Combining Results from Multiple Futures Using thenCombine()
,thenCompose()
, thenApply()
: Composing CompletableFuture#
CompletableFuture can compose multiple asynchronous tasks.
thenCombine()
: Combines results of two independent futures when both completethenCompose()
: Chains dependent futures, using the result of the first to create a new future.thenApply()
: Transforms the result of a completed future into another value.
Output:
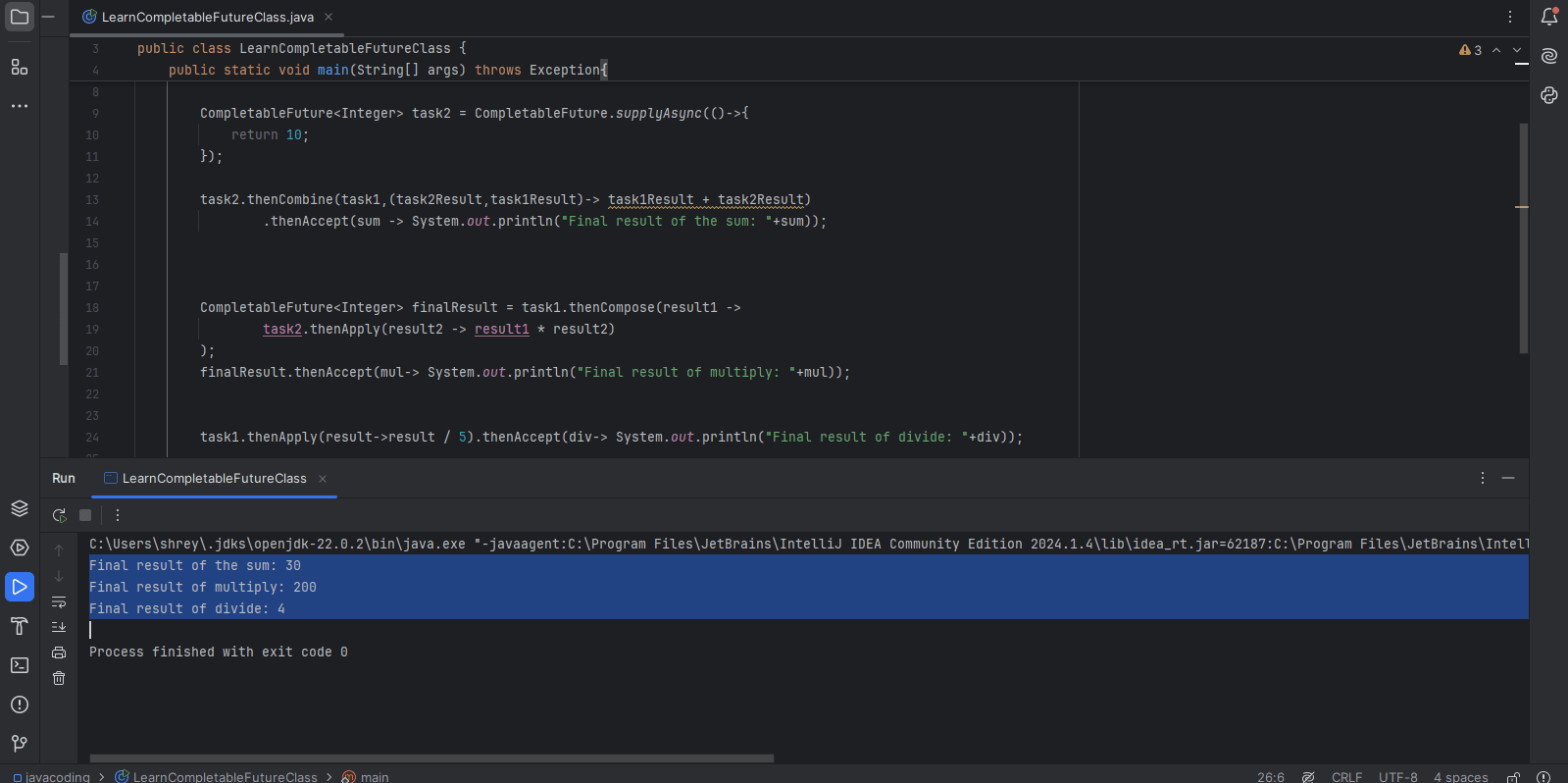
thenCombine()
,thenCompose()
, thenApply()
: Composing CompletableFuture6. Using runAsync()
for Non-returning Tasks#
Use runAsync()
for tasks that do not return a value, such as logging or background operations.
Output:
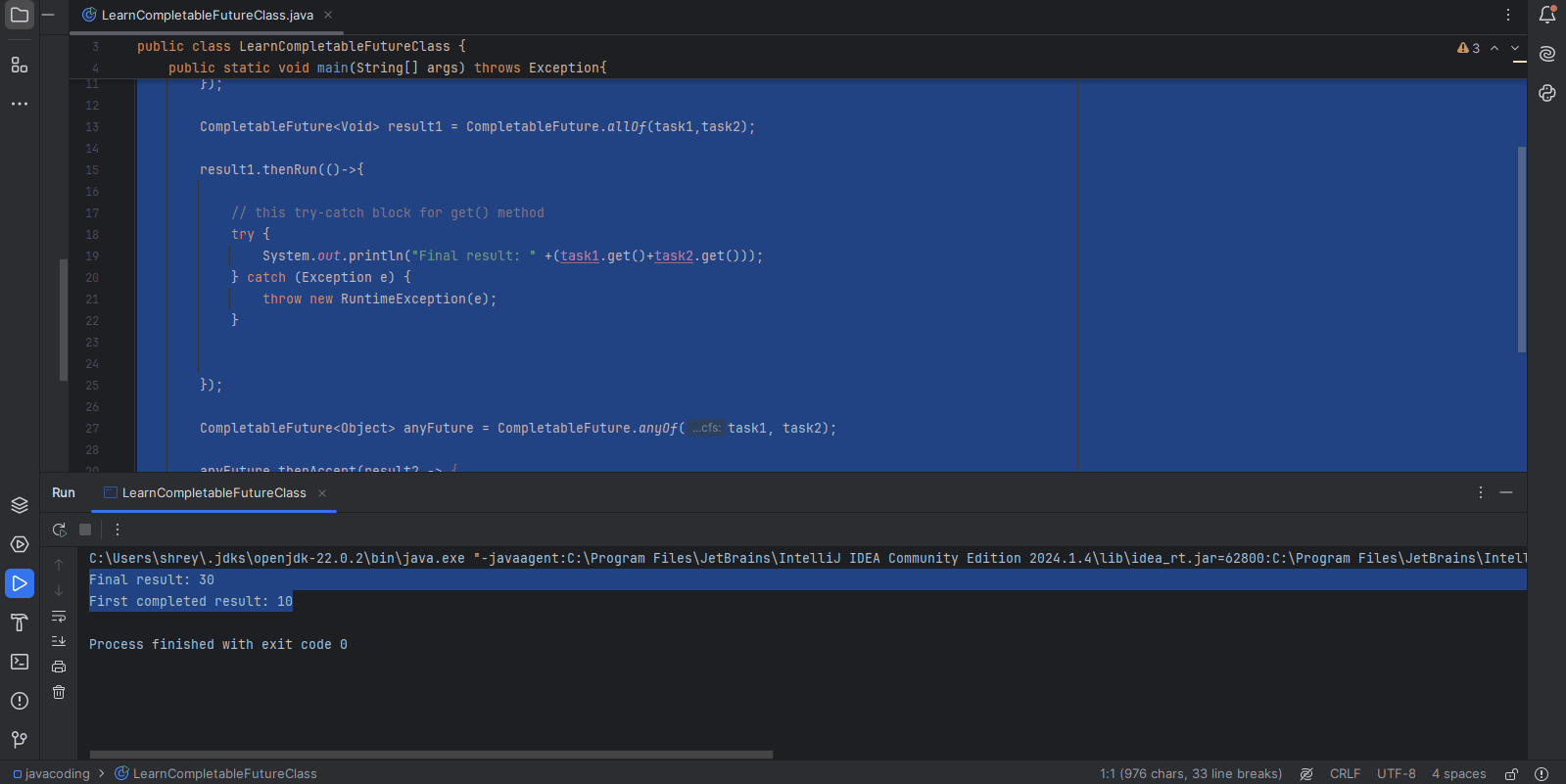
runAsync()
for Non-returning Tasks7. Using a Custom Executor with CompletableFuture#
To control the concurrency and manage the number of threads, you can provide a custom executor for asynchronous tasks.
ExecutorService
manages asynchronous tasks by providing thread pooling and lifecycle control. It simplifies concurrent task execution, improving performance and resource management.
Output:
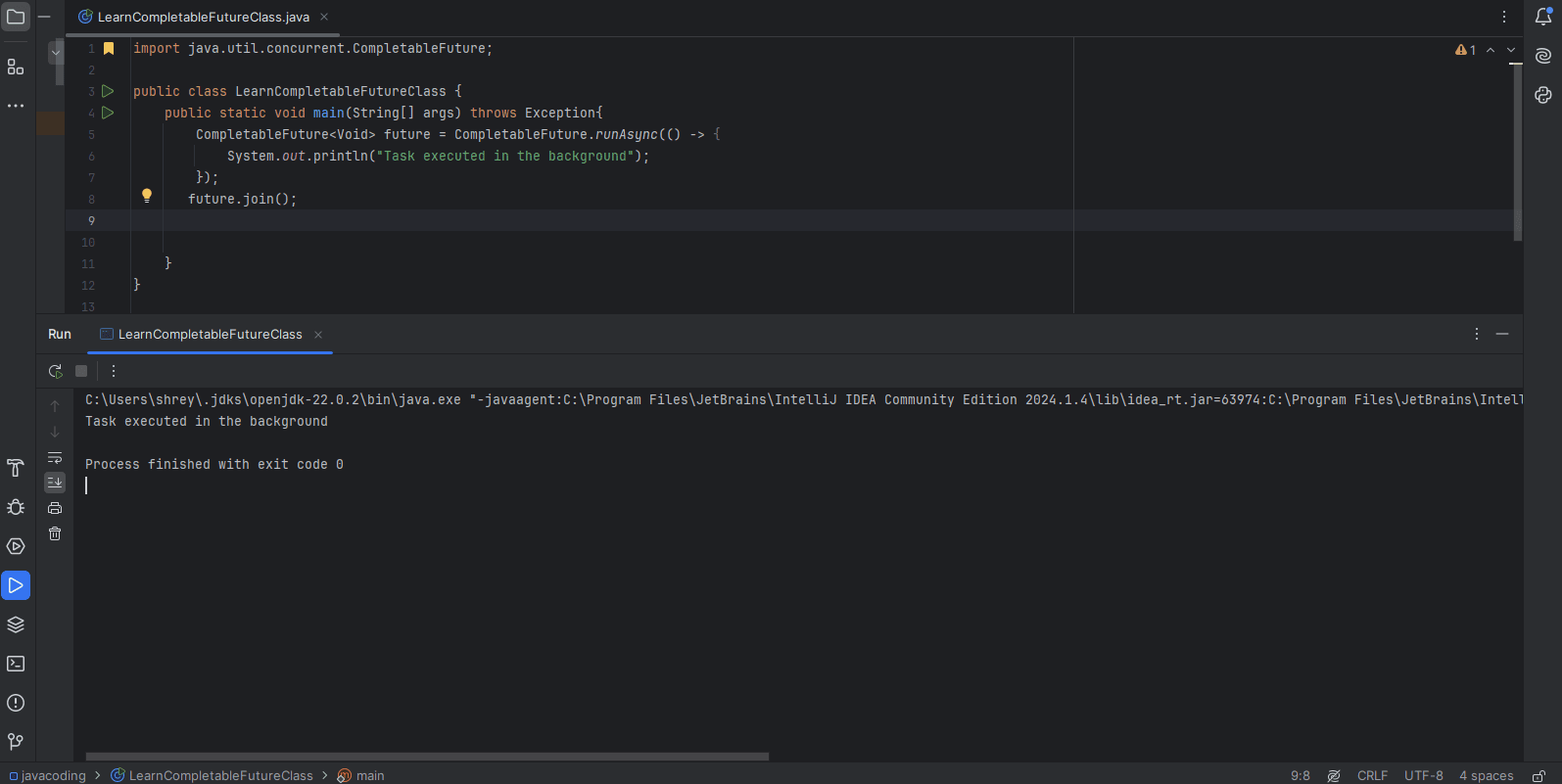
Note: If you don't call future.get() or wait for the task to complete, the program might terminate before the background task finishes executing, depending on how the JVM handles the thread.
Core Difference between Future and CompletableFuture: #
Future
is a simpler interface to asynchronous task management: with methods like get() that require the blocking of the current thread. Conversely, CompletableFuture
is much more sophisticated and allows non-blocking operations for chaining tasks and better handling of exceptions. Use Future for straightforward, once-off tasks that can afford to block. Use CompletableFuture for more complex and chained non-blocking task workflows with good exception handling.
Features | Future<T> In Java | CompletableFuture<T> In Java |
Introduced | In Java 5 | In Java 8 |
Asynchronous Execution | Yes, but limited | Yes, but without any limitation |
Non-blocking Calls | No, must use get() which blocks | Yes, supports non-blocking methods like thenApply() , thenAccept() |
Manual Completion | No, only the computation can set the result | Yes, can manually complete with complete() or completeExceptionally() |
Chaining Operations | No, requires additional handling | Yes, allows method chaining (thenApply() , thenCompose() ) |
Exception Handling | No built-in exception handling | Provides exceptionally() , handle() for error handling |
Multiple Futures Combination | Not supported directly | Supports combining multiple futures (thenCombine() , allOf() , anyOf() ) |
Thread Pool | Uses ExecutorService.submit() | Uses ForkJoinPool but can accept custom executors |
Conclusion:#
We explored the CompletableFuture in Java, and how it is designed to simplify asynchronous programming. Non-blocking execution allows for the chaining of tasks and handling concurrent tasks. The exception handling, timeout management, and task interruption offered by CompletableFuture are simple and make it suitable for writing scalable and fast applications. Top Ranking Keywords: CompletableFuture Java, Asynchronous programming Java, Non-blocking execution, Java task composition, Scalable applications.